The to_excel()
method in Pandas is used to write a DataFrame to an Excel file.
Example
import pandas as pd
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'San Francisco', 'Los Angeles']
}
df = pd.DataFrame(data)
# write df to an excel file
df.to_excel('output.xlsx')
to_excel() Syntax
The syntax of the to_excel()
method in Pandas is:
df.to_excel(excel_writer, sheet_name='Sheet1', na_rep='', float_format=None, columns=None, header=True, index=True, index_label=None, startrow=0, startcol=0, merge_cells=True, encoding=None, freeze_panes=None)
to_excel() Arguments
The to_excel()
method has the following arguments:
excel_writer
: the file path or existingExcelWriter
object to target, or a file-like objectsheet_name
(optional): name of the sheet which will contain the DataFramena_rep
(optional): string representation ofNaN
to usefloat_format
(optional): format string for floating point numberscolumns
(optional): columns to writeheader
(optional): whether to write out the column namesindex
(optional): whether to write out the row names (index)index_label
(optional): column label for index column(s) if desiredstartrow
(optional): upper left cell row to dump data framestartcol
(optional): upper left cell column to dump data framemerge_cells
(optional): writeMultiIndex
and hierarchical rows as merged cellsencoding
(optional): encoding for the output filefreeze_panes
(optional): top-left cell where to freeze the frame
to_excel() Return Value
The return value of to_excel()
is None
. The method writes the DataFrame to an Excel file without returning any value.
Example 1: Specifying Sheet Name and Starting Row
import pandas as pd
# create DataFrame
data = {'Name': ['Tom', 'Nick', 'John'],
'Age': [20, 21, 19],
'City': ['New York', 'London', 'Paris']}
df = pd.DataFrame(data)
# write DataFrame to Excel file with a specific sheet name and starting row
df.to_excel('output.xlsx', sheet_name='People', startrow=2)
output.xlsx
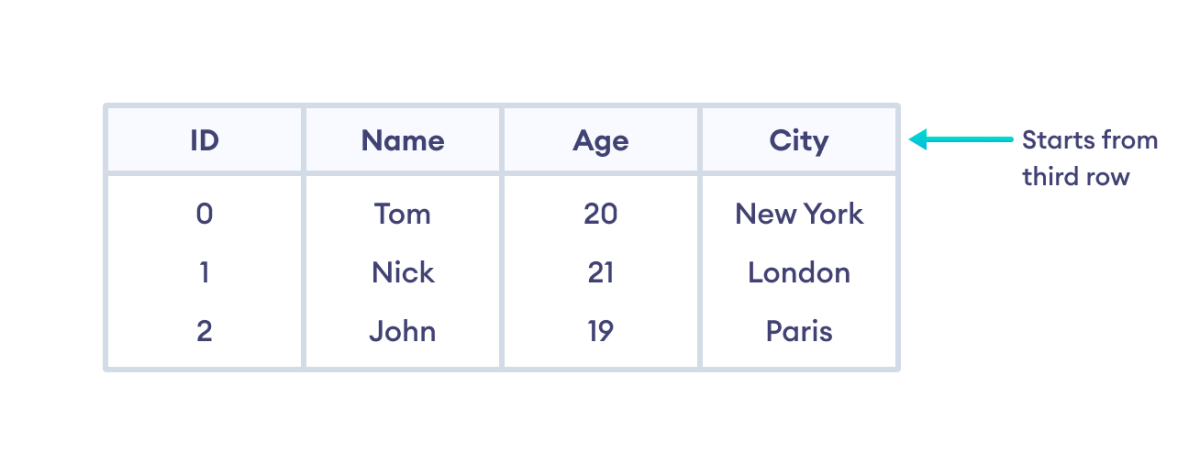
In the example above, we wrote the DataFrame to an Excel file with a specific sheet name People
and started the data from the third row of the sheet.
Example 2: Writing Only Specific Columns to Excel
import pandas as pd
# create DataFrame
data = {'Name': ['Tom', 'Nick', 'John'],
'Age': [20, 21, 19],
'City': ['New York', 'London', 'Paris'],
'Salary': [50000, 60000, 55000]}
df = pd.DataFrame(data)
# save only specific columns to Excel
df.to_excel('output.xlsx', columns=['Name', 'Age'])
output.xlsx
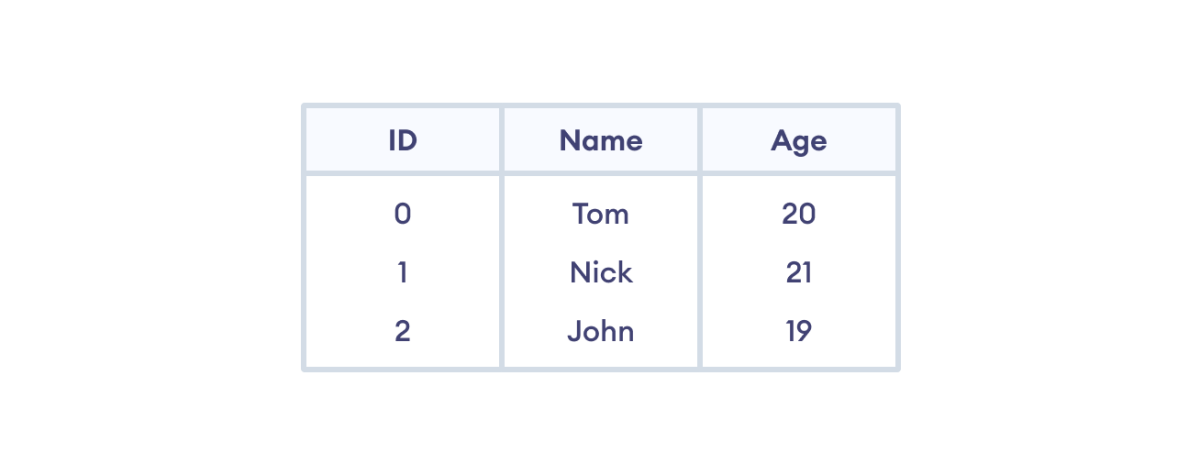
In the example above, we selectively exported only the Name
and Age
columns of our DataFrame to the output.xlsx
Excel file.
Example 3: Using Index Label and Freeze Panes
import pandas as pd
# create DataFrame
data = {'Name': ['Tom', 'Nick', 'John'],
'Age': [20, 21, 19]}
df = pd.DataFrame(data)
# write to Excel with index label and freeze the top row
df.to_excel('output.xlsx', index_label='ID', freeze_panes=(1,0))
output.xlsx
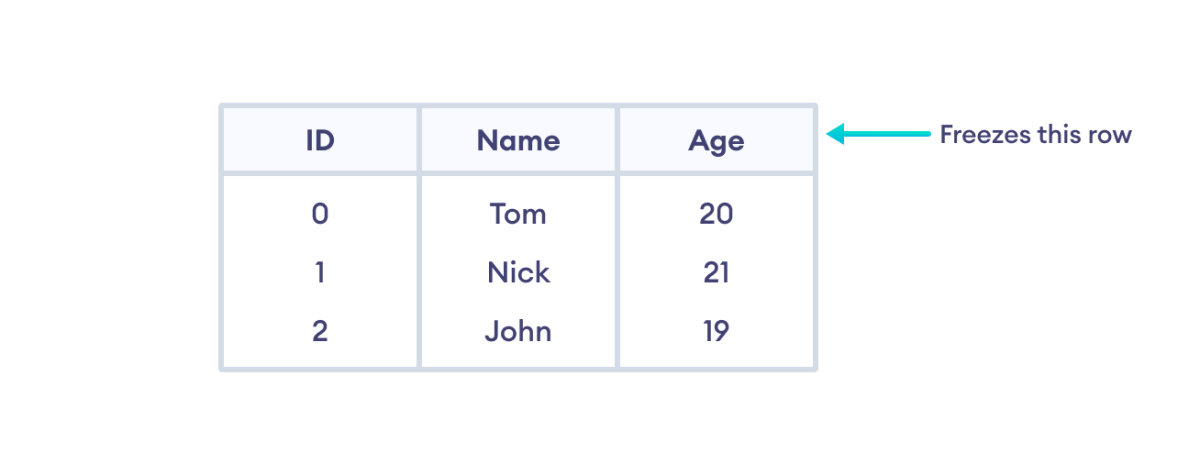
In the example above, we wrote the DataFrame to an Excel file and labeled the index column as ID
. We also froze the top row by using the freeze_panes
option.
Here,
index_label ='ID'
: This argument adds a column labelID
to the index column in the Excel file. If the DataFrame has an index, this will be the heading of the first column in the Excel spreadsheet.
freeze_panes = (1,0)
: This argument freezes the top row of the Excel worksheet. In Excel, freezing panes keeps a set of rows or columns visible while scrolling through the rest of the worksheet.