In programming, loops are used to repeat a block of code until a specified condition is met.
C programming has three types of loops.
- for loop
- while loop
- do...while loop
In the previous tutorial, we learned about for
loop. In this tutorial, we will learn about while
and do..while
loop.
while loop
The syntax of the while
loop is:
while (testExpression) {
// the body of the loop
}
How while loop works?
- The
while
loop evaluates thetestExpression
inside the parentheses()
. - If
testExpression
is true, statements inside the body ofwhile
loop are executed. Then,testExpression
is evaluated again. - The process goes on until
testExpression
is evaluated to false. - If
testExpression
is false, the loop terminates (ends).
To learn more about test expressions (when testExpression
is evaluated to true and false), check out relational and logical operators.
Flowchart of while loop
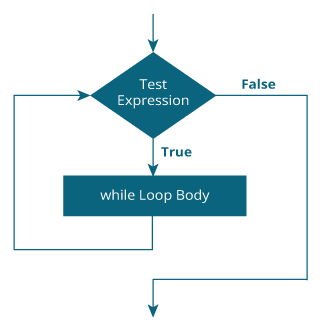
Example 1: while loop
// Print numbers from 1 to 5
#include <stdio.h>
int main() {
int i = 1;
while (i <= 5) {
printf("%d\n", i);
++i;
}
return 0;
}
Output
1 2 3 4 5
Here, we have initialized i to 1.
- When
i = 1
, the test expressioni <= 5
is true. Hence, the body of thewhile
loop is executed. This prints1
on the screen and the value of i is increased to2
. - Now,
i = 2
, the test expressioni <= 5
is again true. The body of thewhile
loop is executed again. This prints2
on the screen and the value of i is increased to3
. - This process goes on until i becomes 6. Then, the test expression
i <= 5
will be false and the loop terminates.
do...while loop
The do..while
loop is similar to the while
loop with one important difference. The body of do...while
loop is executed at least once. Only then, the test expression is evaluated.
The syntax of the do...while
loop is:
do {
// the body of the loop
}
while (testExpression);
How do...while loop works?
- The body of
do...while
loop is executed once. Only then, thetestExpression
is evaluated. - If
testExpression
is true, the body of the loop is executed again andtestExpression
is evaluated once more. - This process goes on until
testExpression
becomes false. - If
testExpression
is false, the loop ends.
Flowchart of do...while Loop
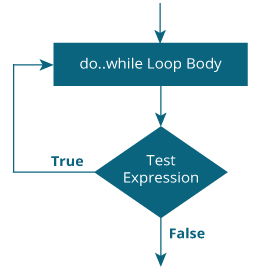
Example 2: do...while loop
// Program to add numbers until the user enters zero
#include <stdio.h>
int main() {
double number, sum = 0;
// the body of the loop is executed at least once
do {
printf("Enter a number: ");
scanf("%lf", &number);
sum += number;
}
while(number != 0.0);
printf("Sum = %.2lf",sum);
return 0;
}
Output
Enter a number: 1.5 Enter a number: 2.4 Enter a number: -3.4 Enter a number: 4.2 Enter a number: 0 Sum = 4.70
Here, we have used a do...while
loop to prompt the user to enter a number. The loop works as long as the input number is not 0
.
The do...while
loop executes at least once i.e. the first iteration runs without checking the condition. The condition is checked only after the first iteration has been executed.
do {
printf("Enter a number: ");
scanf("%lf", &number);
sum += number;
}
while(number != 0.0);
So, if the first input is a non-zero number, that number is added to the sum variable and the loop continues to the next iteration. This process is repeated until the user enters 0
.
But if the first input is 0, there will be no second iteration of the loop and sum becomes 0.0
.
Outside the loop, we print the value of sum.