The switch statement allows us to execute one code block among many alternatives.
You can do the same thing with the if...else..if
ladder. However, the syntax of the switch
statement is much easier to read and write.
Syntax of switch...case
switch (expression)
{
case constant1:
// statements
break;
case constant2:
// statements
break;
.
.
.
default:
// default statements
}
How does the switch statement work?
The expression is evaluated once and compared with the values of each case label.
- If there is a match, the corresponding statements after the matching label are executed. For example, if the value of the expression is equal to constant2, statements after
case constant2:
are executed untilbreak
is encountered. - If there is no match, the default statements are executed.
Notes:
- If we do not use the
break
statement, all statements after the matching label are also executed. - The
default
clause inside theswitch
statement is optional.
switch Statement Flowchart
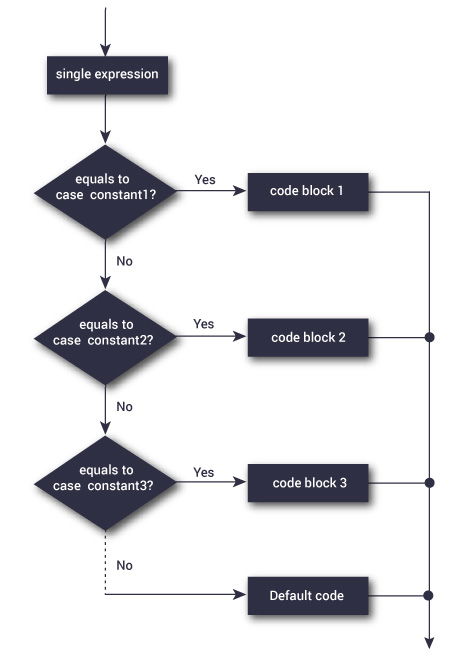
Example: Simple Calculator
// Program to create a simple calculator
#include <stdio.h>
int main() {
char operation;
double n1, n2;
printf("Enter an operator (+, -, *, /): ");
scanf("%c", &operation);
printf("Enter two operands: ");
scanf("%lf %lf",&n1, &n2);
switch(operation)
{
case '+':
printf("%.1lf + %.1lf = %.1lf",n1, n2, n1+n2);
break;
case '-':
printf("%.1lf - %.1lf = %.1lf",n1, n2, n1-n2);
break;
case '*':
printf("%.1lf * %.1lf = %.1lf",n1, n2, n1*n2);
break;
case '/':
printf("%.1lf / %.1lf = %.1lf",n1, n2, n1/n2);
break;
// operator doesn't match any case constant +, -, *, /
default:
printf("Error! operator is not correct");
}
return 0;
}
Output
Enter an operator (+, -, *, /): - Enter two operands: 32.5 12.4 32.5 - 12.4 = 20.1
The - operator entered by the user is stored in the operation variable. And, two operands 32.5 and 12.4 are stored in variables n1 and n2 respectively.
Since the operation is -
, the control of the program jumps to
printf("%.1lf - %.1lf = %.1lf", n1, n2, n1-n2);
Finally, the break statement terminates the switch
statement.