The read_excel()
method in Pandas allows us to import data from excel files into the Python environment.
Example
import pandas as pd
# read data from an excel file
df = pd.read_excel('data.xlsx')
print(df)
read_excel() Syntax
The syntax of the read_excel()
method in Pandas is:
pd.read_excel(io, sheet_name=0, header=0, names=None, index_col=None, usecols=None, dtype=None, converters=None)
read_excel() Arguments
The read_excel()
method has the following arguments:
io
: specifies the path representing the excel file to be readsheet_name
(optional): the name or index of the sheet to read from the excel fileheader
(optional): the row to use as the column namesnames
(optional): a list of column names to use instead of the header rowindex_col
(optional): the column to use as the indexusecols
(optional): a list of column names or indices to readdtype
(optional): data type to force on the columnsconverters
(optional): a dictionary specifying functions to apply to specific columns for data conversion
read_excel() Return Value
The read_excel()
method returns a DataFrame containing the data from the Excel file.
Example 1: Basic Excel File Reading
Let's take a excel file with the following data:
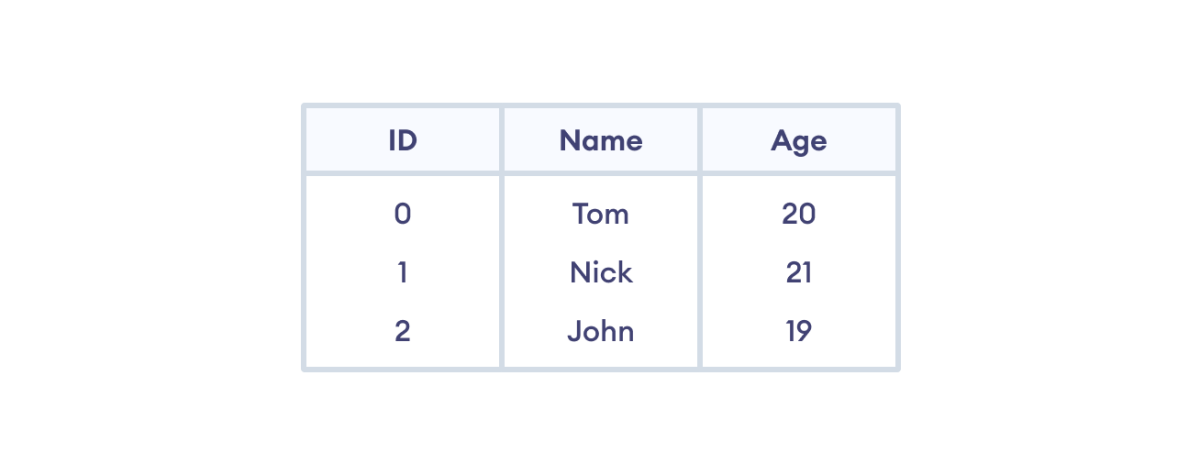
Now, let's import the above file using read_excel()
.
import pandas as pd
# read data from an excel file into a DataFrame
df = pd.read_excel('data.xlsx')
print(df)
Output
ID Name Age 0 0 Tom 20 1 1 Nick 21 2 2 John 19
In this example, we read data from an excel file named data.xlsx
and displayed the resulting DataFrame.
Example 2: Reading Specific Sheet
Let's take another sheet in data.xlsx
named Address
with the following data:
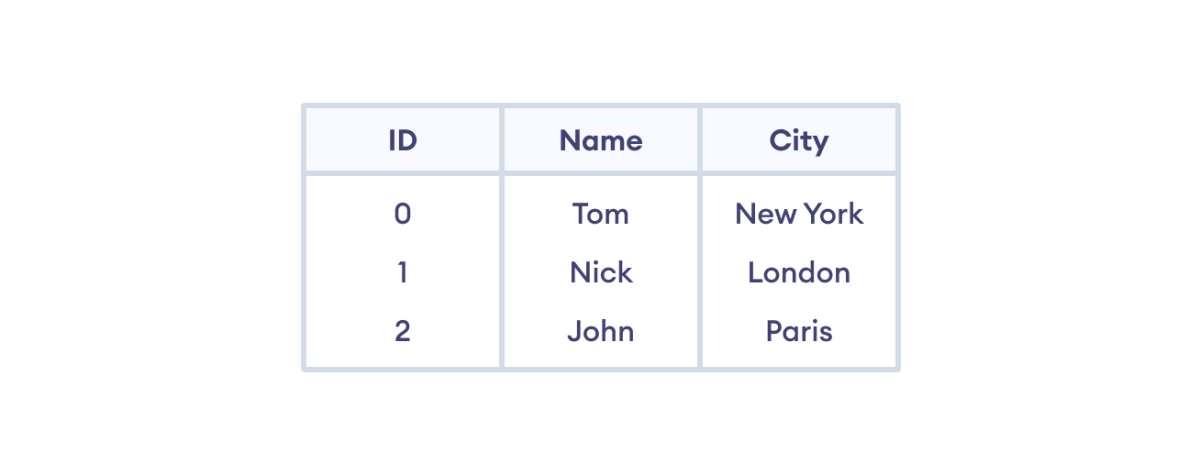
import pandas as pd
# read data from a specific sheet in an excel file
df = pd.read_excel('data.xlsx', sheet_name='Address')
print(df)
Output
ID Name City 0 0 Tom New York 1 1 Nick London 2 2 John Paris
In this example, we read data from the sheet named Address
in an excel file named data.xlsx
.
Example 3: Custom Column Names and Index Column
For this example, let's take the same file as in example 1.
import pandas as pd
# read data from an excel file
# with custom column names and index column
df = pd.read_excel('data.xlsx', header=0, names=['ID','First_Name', 'Age'], index_col='ID')
print(df)
Output
First_Name Age ID 0 Tom 20 1 Nick 21 2 John 19
In this example, we read data from an excel file and specified custom column names and an index column.