We use the ternary operator in C to run one code when the condition is true
and another code when the condition is false
. For example,
(age >= 18) ? printf("Can Vote") : printf("Cannot Vote");
Here, when the age is greater than or equal to 18, Can Vote
is printed. Otherwise, Cannot Vote
is printed.
Syntax of Ternary Operator
The syntax of ternary operator is:
testCondition ? expression1 : expression 2;
The testCondition
is a boolean expression that results in either true or false. If the condition is
true
- expression1 (before the colon) is executedfalse
- expression2 (after the colon) is executed
The ternary operator takes 3 operands (condition, expression1 and expression2)
. Hence, the name ternary operator.
Example: C Ternary Operator
#include <stdio.h>
int main() {
int age;
// take input from users
printf("Enter your age: ");
scanf("%d", &age);
// ternary operator to find if a person can vote or not
(age >= 18) ? printf("You can vote") : printf("You cannot vote");
return 0;
}
Output 1
Enter your age: 12 You cannot vote
In the above example, we have used a ternary operator that checks whether a user can vote or not based on the input value. Here,
age >= 18
- test condition that checks if input value is greater or equal to 18printf("You can vote")
- expression1 that is executed if condition is trueprintf("You cannot vote")
- expression2 that is executed if condition is false
Here, the user inputs 12, so the condition becomes false. Hence, we get You cannot vote
as output.
Output 2
Enter your age: 24 You can vote
This time the input value is 24 which is greater than 18. Hence, we get You can vote
as output.
Assign the ternary operator to a variable
In C programming, we can also assign the expression of the ternary operator to a variable. For example,
variable = condition ? expression1 : expression2;
Here, if the test condition is true
, expression1 will be assigned to the variable. Otherwise, expression2 will be assigned.
Let's see an example.
#include <stdio.h>
int main() {
// create variables
char operator = '+';
int num1 = 8;
int num2 = 7;
// using variables in ternary operator
int result = (operator == '+') ? (num1 + num2) : (num1 - num2);
printf("%d", result);
return 0;
}
// Output: 15
In the above example, the test condition (operator == '+')
will always be true. So, the first expression before the colon i.e the summation of two integers num1 and num2 is assigned to the result variable.
And, finally the result variable is printed as an output giving out the summation of 8 and 7. i.e 15.
Ternary Operator Vs. if...else Statement in C
In some of the cases, we can replace the if...else
statement with a ternary operator. This will make our code cleaner and shorter.
Let's see an example:
#include <stdio.h>
int main() {
int number = 3;
if (number % 2 == 0) {
printf("Even Number");
}
else {
printf("Odd Number");
}
return 0;
}
We can replace this code with the following code using the ternary operator.
#include <stdio.h>
int main() {
int number = 3;
(number % 2 == 0) ? printf("Even Number") : printf("Odd Number");
return 0;
}
Here, both the programs are doing the same task, checking even/odd numbers. However, the code using the ternary operator looks clean and concise.
In such cases, where there is only one statement inside the if...else
block, we can replace it with a ternary operator
.
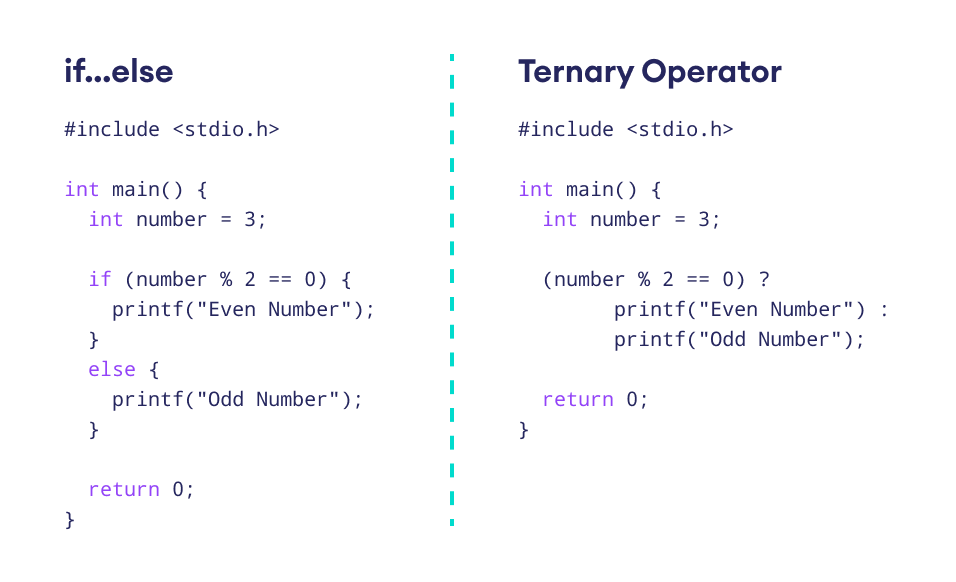