In C programming, we can convert the value of one data type (int,
float
, double
, etc.) to another. This process is known as type conversion. Let's see an example,
#include <stdio.h>
int main() {
int number = 34.78;
printf("%d", number);
return 0;
}
// Output: 34
Here, we are assigning the double value 34.78 to the integer variable number. In this case, the double value is automatically converted to integer value 34.
This type of conversion is known as implicit type conversion. In C, there are two types of type conversion:
- Implicit Conversion
- Explicit Conversion
Implicit Type Conversion In C
As mentioned earlier, in implicit type conversion, the value of one type is automatically converted to the value of another type. For example,
#include<stdio.h>
int main() {
// create a double variable
double value = 4150.12;
printf("Double Value: %.2lf\n", value);
// convert double value to integer
int number = value;
printf("Integer Value: %d", number);
return 0;
}
Output
Double Value: 4150.12 Integer Value: 4150
The above example has a double variable with a value 4150.12. Notice that we have assigned the double value to an integer variable.
int number = value;
Here, the C compiler automatically converts the double value 4150.12 to integer value 4150.
Since the conversion is happening automatically, this type of conversion is called implicit type conversion.
Example: Implicit Type Conversion
#include<stdio.h>
int main() {
// character variable
char alphabet = 'a';
printf("Character Value: %c\n", alphabet);
// assign character value to integer variable
int number = alphabet;
printf("Integer Value: %d", number);
return 0;
}
Output
Character Value: a Integer Value: 97
The code above has created a character variable alphabet with the value 'a'
. Notice that we are assigning alphabet to an integer variable.
int number = alphabet;
Here, the C compiler automatically converts the character 'a'
to integer 97. This is because, in C programming, characters are internally stored as integer values known as ASCII Values.
ASCII defines a set of characters for encoding text in computers. In ASCII code, the character 'a'
has integer value 97, that's why the character 'a'
is automatically converted to integer 97.
If you want to learn more about finding ASCII values, visit find ASCII value of characters in C.
Explicit Type Conversion In C
In explicit type conversion, we manually convert values of one data type to another type. For example,
#include<stdio.h>
int main() {
// create an integer variable
int number = 35;
printf("Integer Value: %d\n", number);
// explicit type conversion
double value = (double) number;
printf("Double Value: %.2lf", value);
return 0;
}
Output
Integer Value: 35 Double Value: 35.00
We have created an integer variable named number with the value 35 in the above program. Notice the code,
// explicit type conversion
double value = (double) number;
Here,
(double)
- represents the data type to which number is to be convertednumber
- value that is to be converted todouble
type
Example: Explicit Type Conversion
#include<stdio.h>
int main() {
// create an integer variable
int number = 97;
printf("Integer Value: %d\n", number);
// (char) converts number to character
char alphabet = (char) number;
printf("Character Value: %c", alphabet);
return 0;
}
Output
Integer Value: 97 Character Value: a
We have created a variable number
with the value 97 in the code above. Notice that we are converting this integer to character.
char alphabet = (char) number;
Here,
(char)
- explicitly converts number into characternumber
- value that is to be converted tochar
type
Data Loss In Type Conversion
In our earlier examples, when we converted a double type value to an integer type, the data after decimal was lost.
#include<stdio.h>
int main() {
// create a double variable
double value = 4150.12;
printf("Double Value: %.2lf\n", value);
// convert double value to integer
int number = value;
printf("Integer Value: %d", number);
return 0;
}
Output
Double Value: 4150.12 Integer Value: 4150
Here, the data 4150.12 is converted to 4150. In this conversion, data after the decimal, .12 is lost.
This is because double
is a larger data type (8 bytes) than int
(4 bytes), and when we convert data from larger type to smaller, there will be data loss..
Similarly, there is a hierarchy of data types in C programming. Based on the hierarchy, if a higher data type is converted to lower type, data is lost, and if lower data type is converted to higher type, no data is lost.
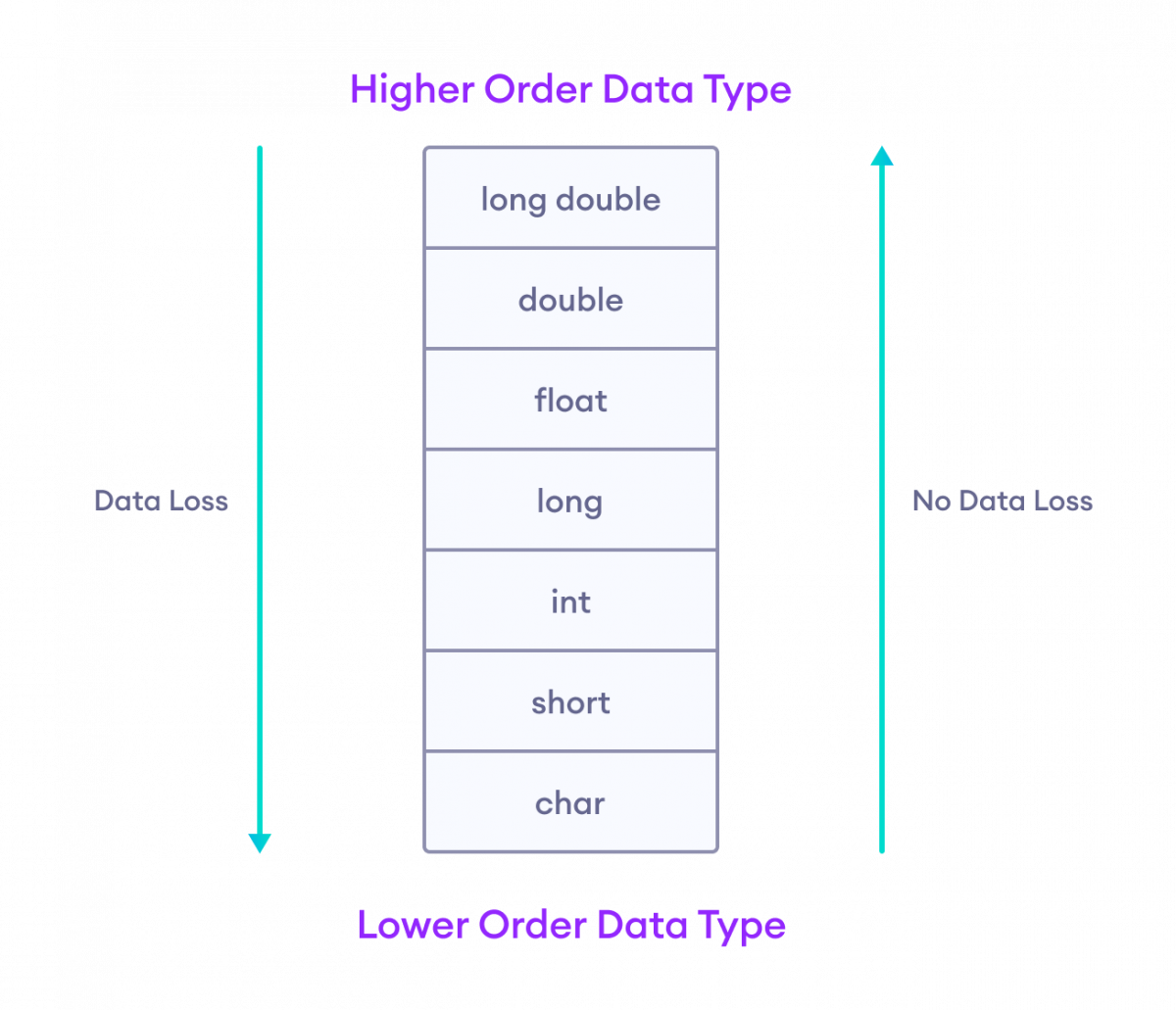
Here,
- data loss - if
long double
type is converted todouble
type - no data loss - if
char
is converted toint