In NumPy, interpolation estimates the value of a function at points where the value is not known.
Let's suppose we have two arrays: day representing the day of the week and gold_price representing the price of gold per gram.
day = np.array([2, 4, 7])
gold_price = np.array([55, 58, 65])
With the given data set, we can say the price of the gold on day 2 is 55. But we do not know the price of gold on day 3.
In such a case, we use interpolation to estimate the price of the gold at any day within the data points.
NumPy Interpolation
NumPy provides an interp()
function to work with interpolation. Let's see an example.
import numpy as np
day = np.array([2, 4, 7])
gold_price = np.array([55, 58, 65])
# find the value of gold on day 3
day3_value = np.interp(3, day, gold_price)
print("The value of gold on day 3 is", day3_value)
Output
The value of gold on day 3 is 56.5
Here, we used the interp()
function to find the value of gold on day 3.
We have sent 3 arguments to the interp()
function:
- 3: coordinate whose value needs to be interpolated
- day: x-ordinate of the data points that represent days of the week
- gold_price: y-coordinates of the data points that represent the gold price
Example: NumPy Interpolation
import numpy as np
day = np.array([2, 4, 7, 10])
gold_price = np.array([55, 58, 65, 70])
# days whose value is to be interpolated
interpolate_days = np.array([1, 3, 5, 6, 8, 9])
interpolated_price= np.interp(interpolate_days, day, gold_price)
print(interpolated_price)
Output
[55. 56.5 60.33333333 62.66666667 66.66666667 68.33333333]
In this example, we have used the interp()
function to interpolate the values in the array interpolate_days.
The resulting array is the estimated price of gold on day 1, 3, 5, 6, 8, and 9.
Graph the Interpolated Values
To plot the graph, we need to import the pyplot
from the matplotlib
module. Let's see an example.
import numpy as np
import matplotlib.pyplot as plt
# arrays with random data points
x = np.array([0, 1, 2, 3, 4, 5])
y = np.array([0, 3, 4, 5, 7, 8])
# generate evenly spaced values between the minimum and maximum x values
x_interp = np.linspace(x.min(), x.max(), 100)
# interp() to interpolate y values
y_interp = np.interp(x_interp, x, y)
# plot the original data points and the interpolated values
plt.plot(x, y, 'bo')
plt.plot(x_interp, y_interp, 'r-')
plt.show()
Output
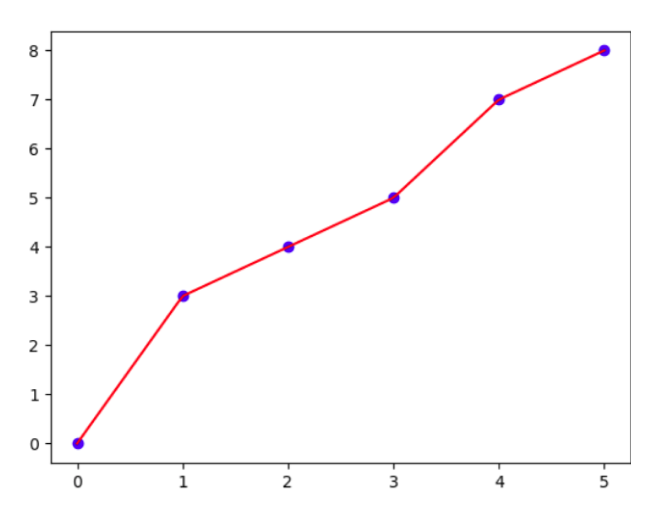
In this example, we have plotted the graph of the interpolated values in y_interp.
First, we generated 100 evenly spaced values between the minimum and maximum of x using the linspace()
function.
Then we used the interp()
function to interpolate the y values and plotted the interpolated values using plot()
function.