Suppose you are working with loops. It is sometimes desirable to skip the current iteration of the loop.
In such case, continue
is used. The continue
construct skips the current iteration of the enclosing loop, and the control of the program jumps to the end of the loop body.
How continue works?
It is almost always used with if...else construct. For example,
while (testExpression1) { // codes1 if (testExpression2) { continue } // codes2 }
If the testExpression2 is evaluated to true
, continue
is executed which skips all the codes inside while
loop after it for that iteration.
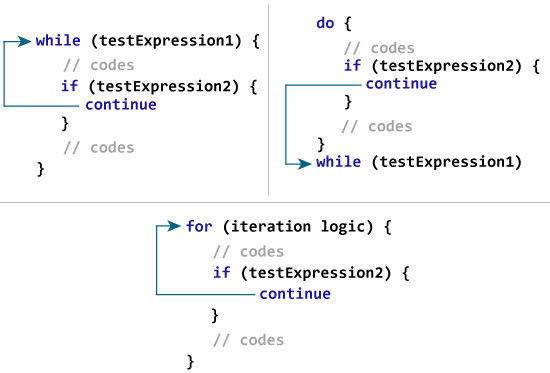
Example: Kotlin continue
fun main(args: Array<String>) {
for (i in 1..5) {
println("$i Always printed.")
if (i > 1 && i < 5) {
continue
}
println("$i Not always printed.")
}
}
When you run the program, the output will be:
1 Always printed. 1 Not always printed. 2 Always printed. 3 Always printed. 4 Always printed. 5 Always printed. 5 Not always printed.
When the value of i is greater than 1 and less than 5, continue
is executed, which skips the execution of
println("$i Not always printed.")
statement.
However, the statement
println("$i Always printed.")
is executed in each iteration of the loop because this statement exists before the continue
construct.
Example: Calculate Sum of Positive Numbers Only
The program below calculates the sum of maximum of 6 positive numbers entered by the user. If the user enters negative number or zero, it is skipped from calculation.
Visit Kotlin Basic Input Output to learn more on how to take input from the user.
fun main(args: Array<String>) {
var number: Int
var sum = 0
for (i in 1..6) {
print("Enter an integer: ")
number = readLine()!!.toInt()
if (number <= 0)
continue
sum += number
}
println("sum = $sum")
}
When you run the program, the output will be:
Enter an integer: 4 Enter an integer: 5 Enter an integer: -50 Enter an integer: 10 Enter an integer: 0 Enter an integer: 12 sum = 31
Kotlin Labeled continue
What you have learned till now is unlabeled form of continue
, which skips current iteration of the nearest enclosing loop. continue
can also be used to skip the iteration of the desired loop (can be outer loop) by using continue
labels.
How labeled continue works?
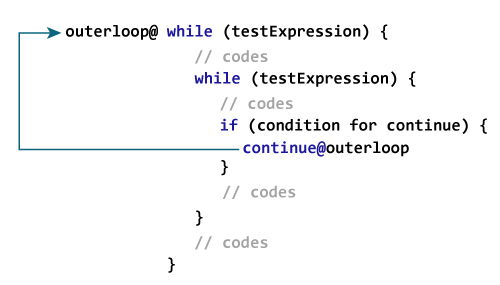
Label in Kotlin starts with an identifier which is followed by @
.
Here, outerloop@ is a label marked at outer while loop. Now, by using continue
with the label (continue@outerloop
in this case), you can skip the execution of codes of the specific loop for that iteration.
Example: labeled continue
fun main(args: Array<String>) {
here@ for (i in 1..5) {
for (j in 1..4) {
if (i == 3 || j == 2)
continue@here
println("i = $i; j = $j")
}
}
}
When you run the program, the output will be:
i = 1; j = 1 i = 2; j = 1 i = 4; j = 1 i = 5; j = 1
The use of labeled continue
is often discouraged as it makes your code hard to understand. If you are in a situation where you have to use labeled continue
, refactor your code and try to solve it in a different way to make it more readable.
There are 3 structural jump expressions in Kotlin: break
, continue
and return
. To learn about break
and return
expression, visit:
- Kotlin break
- Kotlin function