Suppose, you need to extend a class with new functionalities. In most programming languages, you either derive a new class or use some kind of design pattern to do this.
However, in Koltin, you can also use extension function to extend a class with new functionalities. Basically, an extension function is a member function of a class that is defined outside the class.
For example, you need to use a method to the String class that returns a new string with first and last character removed; this method is not already available in String
class. You can use extension function to accomplish this task.
Example: Remove First and Last Character of String
fun String.removeFirstLastChar(): String = this.substring(1, this.length - 1)
fun main(args: Array<String>) {
val myString= "Hello Everyone"
val result = myString.removeFirstLastChar()
println("First character is: $result")
}
When you run the program, the output will be:
First character is: ello Everyon
Here, an extension function removeFirstLastChar()
is added to the String
class.
The class name is the receiver type (String
class in our example). The this
keyword inside the extension function refers the receiver object.
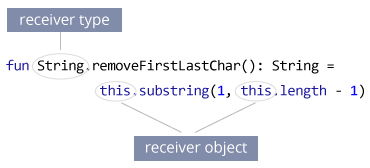
If you need to integrate Kotlin on the top of Java project, you do not need to modify the whole code to Koltin. Just use extension functions to add functionalities.
That being said, it is quite easy to abuse power of extension functions. We recommend you to check these resources to learn on when to use extensions and when not to: