Bitwise operations are used to manipulate the individual bits of binary values.
NumPy provides several bitwise operations that allow us to perform bitwise operations on arrays of integers.
Bitwise Operators Available in NumPy
In NumPy, there are 6 basic bitwise operators available.
Bitwise Operators | Description |
---|---|
bitwise_and() |
calculates bitwise AND operation |
bitwise_or() |
calculates bitwise OR operation |
invert() |
calculates bitwise NOT operation |
bitwise_xor() |
calculates bitwise XOR operation |
left_shift() |
shifts the bits of an integer to the left |
right_shift() |
shift the bits of an integer to the right |
NumPy Bitwise AND Operation
The bitwise_and()
function returns 1 if and only if both the operands are 1. Otherwise, it returns 0.
The bitwise AND operation on a
and b
can be represented in the table below.
a | b | bitwise_and(a, b) |
---|---|---|
0 | 0 | 0 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
Note: The table above is known as the "Truth Table" for the bitwise AND operator.
Let's take a look at the bitwise AND operation of two integers 12 and 25:
12 = 00001100 (In Binary)
25 = 00011001 (In Binary)
# bitwise AND Operation of 12 and 25
00001100
AND 00011001
_____________
00001000 = 8 (In decimal)
Example: NumPy Bitwise AND Operation
import numpy as np
int1 = 12
int2 = 25
# perform bitwise AND operation
result = np.bitwise_and(int1, int2)
print (result)
# Output: 8
In this example, we have declared two variables int1 and int2. Notice the line,
result = np.bitwise_and(int1, int2)
Here, we are performing bitwise AND operation between int1 and int2 using bitwise_and()
.
Example: NumPy Bitwise AND Operation on two Arrays (Element-wise)
import numpy as np
# create two arrays
array1 = np.array([12, 5, 8])
array2 = np.array([25, 12, 2])
# perform bitwise AND operation
result = np.bitwise_and(array1, array2)
print("array1: ", array1)
print("array2: ", array2)
print("Result of bitwise AND operation: ", result)
Output
array1: [12 5 8] array2: [25 12 2] Result of bitwise AND operation: [8 4 0]
Here, np.bitwise_and(array1, array2)
performs a bitwise AND operation between the corresponding elements of the two arrays: array1 and array2.
NumPy Bitwise OR Operation
The bitwise_or()
function returns 1 if at least one of the operands is 1. Otherwise, it returns 0.
The bitwise OR operation on a
and b
can be represented in the table below.
a | b | bitwise_or(a, b) |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 1 | 1 |
1 | 0 | 1 |
Note: The table above is known as the "Truth Table" for the bitwise OR operator.
Let's take a look at the bitwise OR operation of two integers 12 and 25:
12 = 00001100 (In Binary)
25 = 00011001 (In Binary)
# bitwise OR Operation of 12 and 25
00001100
OR 00011001
____________
00011101 = 29 (In decimal)
Example: NumPy Bitwise OR Operation
import numpy as np
int1 = 12
int2 = 25
# perform bitwise OR operation
result = np.bitwise_or(int1, int2)
print (result)
# Output: 29
In this example, we have declared two variables int1 and int2. Here, notice the line,
result = np.bitwise_or(int1, int2)
Here, we are performing bitwise OR operation between int1 and int2 using bitwise_or()
.
Example: NumPy Bitwise OR Operation on two Arrays (Element-wise)
import numpy as np
# create two arrays
array1 = np.array([12, 5, 8])
array2 = np.array([25, 12, 2])
# perform bitwise OR operation
result = np.bitwise_or(array1, array2)
print("array1: ", array1)
print("array2: ", array2)
print("Result of bitwise OR operation: ", result)
Output
array1: [12 5 8] array2: [25 12 2] Result of bitwise OR operation: [29 13 10]
Here, np.bitwise_or(array1, array2)
performs a bitwise OR operation between the corresponding elements of the two arrays: array1 and array2.
NumPy Bitwise NOT Operation
In NumPy, the invert()
function inverts the bit( 0 becomes 1, 1 becomes 0).
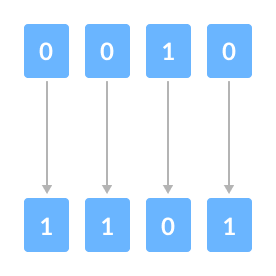
The bitwise NOT operation on a
can be represented in the table below.
a | invert(a) |
---|---|
0 | 1 |
1 | 0 |
Note: The table above is known as the "Truth Table" for the bitwise NOT operator.
It is important to note that the bitwise NOT of any integer N is equal to -(N + 1). For example,
Consider an integer 35. As per the rule, the bitwise NOT of 35 should be -(35 + 1) = -36. Now, let's see if we get the correct answer or not.
35 = 00100011 (In Binary)
# Using bitwise NOT operator
NOT 00100011
________
11011100
In the above example, the bitwise NOT of 00100011 is 11011100. Here, if we convert the result into decimal we get 220.
However, it is important to note that we cannot directly convert the result into decimal and get the desired output. This is because the binary result 11011100 is also equivalent to -36.
To understand this we first need to calculate the binary output of -36. We use 2's complement to calculate the binary of negative integers.
2's complement
The 2's complement of a number N gives -N. It is computed by inverting the bits(0 to 1 and 1 to 0) and then adding 1. For example,
36 = 00100100 (In Binary)
1's Complement = 11011011
2's Complement :
11011011
+ 1
________
11011100
Here, we can see the 2's complement of 36 (i.e. -36) is 11011100. This value is equivalent to the bitwise complement of 35 that we have calculated in the previous section.
Hence, we can say that the bitwise complement of 35 = -36.
Example: NumPy Bitwise NOT Operation
import numpy as np
int1 = 35
# perform bitwise NOT operation
result = np.invert(int1)
print (result)
# Output: -36
In this example, we have performed the bitwise NOT operation on 35.
The bitwise complement of 35 = - (35 + 1) = –36
i.e. invert(35) = -36
This is exactly what we got in the output.
Example: NumPy Bitwise NOT Operation on an Array
import numpy as np
# create two arrays
array1 = np.array([35, 12, 8])
# perform bitwise NOT operation
result = np.invert(array1)
print("array1: ", array1)
print("Result of bitwise NOT operation: ", result)
Output
array1: [35 12 8] Result of bitwise NOT operation: [-36 -13 -9]
As we can see, np.invert(array1)
performs a bitwise NOT operation on elements of array1.
Bitwise XOR Operator
The bitwise_xor()
function returns 1 if and only if one of the operands is 1. However, if both the operands are 0, or if both are 1, then the result is 0.
The bitwise XOR operation on a
and b
can be represented in the table below.
a | b | bitwise_xor(a,b) |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
Let us look at the bitwise XOR operation of two integers 12 and 25:
12 = 00001100 (In Binary)
25 = 00011001 (In Binary)
# bitwise XOR Operation of 12 and 25
00001100
XOR 00011001
____________
00010101 = 21 (In decimal)
Example: Bitwise XOR Operator
import numpy as np
int1 = 12
int2 = 25
# perform bitwise XOR operation
result = np.bitwise_xor(int1, int2)
print (result)
# Output: 21
Here, we are performing bitwise XOR between 12 and 25 using the bitwise_xor()
function.
Example: NumPy Bitwise XOR Operation on two Arrays (Element-wise)
import numpy as np
# create two arrays
array1 = np.array([12, 5, 8])
array2 = np.array([25, 12, 2])
# perform bitwise XOR operation
result = np.bitwise_xor(array1, array2)
print("array1: ", array1)
print("array2: ", array2)
print("Result of bitwise XOR operation: ", result)
Output
array1: [12 5 8] array2: [25 12 2] Result of bitwise XOR operation: [21 9 10]
Here, np.bitwise_xor(array1, array2)
performs a bitwise XOR operation between the corresponding elements of the two arrays: array1 and array2.
Left Shift Operator
We use the left_shift()
function to shift all bits towards the left by a specified number of bits.
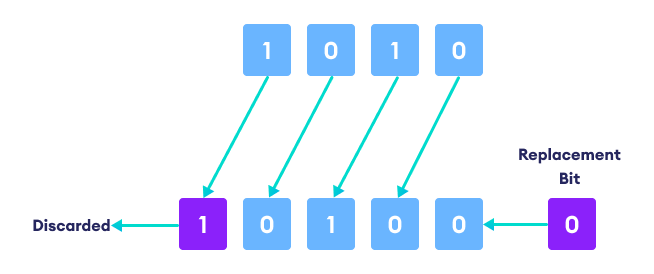
As we can see from the image above,
- We have a 4-digit number. When we perform a 1 bit left shift operation on it, each bit is shifted to the left by 1 bit.
- As a result, the left-most bit is discarded, while the right-most bit remains vacant. This vacancy is replaced by 0.
Example: NumPy Left Shift Operation
import numpy as np
int1 = 3
# perform left shift operation
result = np.left_shift(int1,2)
print (result)
# Output: 12
In this example, we have created a variable int1 with the value 3. Notice the statement
result = np.left_shift(int1, 2)
Here, we are performing 2 bits left shift operation on int1.
Example: Bitwise Left Shift Operation on an Array
import numpy as np
# create two arrays
array1 = np.array([3, 5, 8])
bit_shift = np.array([2, 4, 2])
# perform bitwise left shift operation
result = np.left_shift(array1, bit_shift)
print("array1: ", array1)
print("Result of bitwise left shift operation: ", result)
Output
array1: [3 5 8] Result of bitwise left shift operation: [12 80 32]
Here, np.left_shift(array1, bit_shift)
performs a bitwise left shift operation on the elements of the array1 array.
Right Shift Operator
We use the right_shift()
function to shift all bits towards the right by a specified number of bits.
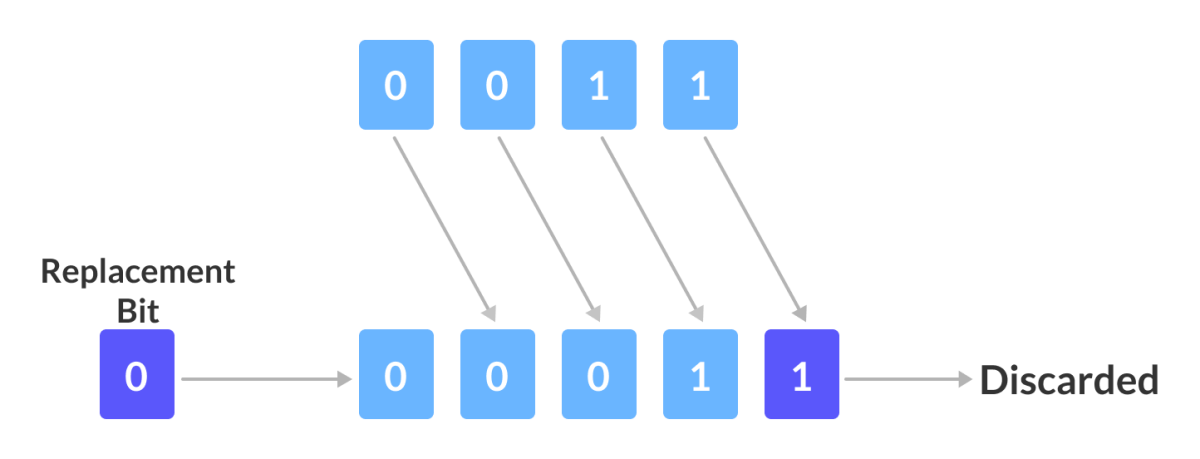
As we can see from the image above,
- We have a 4-digit number. When we perform a 1-bit right shift operation on it, each bit is shifted to the right by 1 bit.
- As a result, the right-most bit is discarded, while the left-most bit remains vacant. This vacancy is replaced by 0 for unsigned numbers.
- For signed numbers, the sign bit (0 for positive number, 1 for negative number) is used to fill the vacated bit positions.
Note: Signed integers represent both positive and negative integers while an unsigned integer only represents positive integers.
Example: NumPy Right Shift Operation
import numpy as np
int1 = 4
# perform right shift operation
result = np.right_shift(int1,2)
print (result) # Output: 1
int2 = -4
# perform right shift operation
result = np.right_shift(int2,2)
print (result) # Output: -1
In this example, we are performing 2 bits right shift operations on values 4 and -4.
As we can see the result is different for 4 and -4. This is because 4 is an unsigned integer so the vacancy is filled with 0 and -4 is a negative signed number so vacancy is filled with 1.
Example: Bitwise Left Shift Operation on an Array
import numpy as np
# create two arrays
array1 = np.array([4, -4, 10, -11])
bit_shift = np.array([2, 2, 3, 3])
# perform bitwise right shift operation
result = np.right_shift(array1, bit_shift)
print("array1: ", array1)
print("Result of bitwise right shift operation: ", result)
Output
array1: [ 4 -4 10 -11] Result of bitwise right shift operation: [ 1 -1 1 -2]
Here, np.right_shoft(array1, bit_shift)
performs a bitwise right shift operation on the elements of the array1 array.