Java Package
A package is simply a container that groups related types (Java classes, interfaces, enumerations, and annotations). For example, in core Java, the ResultSet
interface belongs to the java.sql
package. The package contains all the related types that are needed for the SQL query and database connection.
If you want to use the ResultSet
interface in your code, just import the java.sql package (Importing packages will be discussed later in the article).
As mentioned earlier, packages are just containers for Java classes, interfaces and so on. These packages help you to reserve the class namespace and create a maintainable code.
For example, you can find two Date
classes in Java. However, the rule of thumb in Java programming is that only one unique class name is allowed in a Java project.
How did they manage to include two classes with the same name Date in JDK?
This was possible because these two Date
classes belong to two different packages:
java.util.Date
- this is a normal Date class that can be used anywhere.java.sql.Date
- this is a SQL Date used for the SQL query and such.
Based on whether the package is defined by the user or not, packages are divided into two categories:
Built-in Package
Built-in packages are existing java packages that come along with the JDK. For example, java.lang
, java.util
, java.io
, etc. For example:
import java.util.ArrayList;
class ArrayListUtilization {
public static void main(String[] args) {
ArrayList<Integer> myList = new ArrayList<>(3);
myList.add(3);
myList.add(2);
myList.add(1);
System.out.println(myList);
}
}
Output:
myList = [3, 2, 1]
The ArrayList
class belongs to java.util package
. To use it, we have to import the package first using the import
statement.
import java.util.ArrayList;
User-defined Package
Java also allows you to create packages as per your need. These packages are called user-defined packages.
How to define a Java package?
To define a package in Java, you use the keyword package
.
package packageName;
Java uses file system directories to store packages. Let's create a Java file inside another directory.
For example:
└── com └── test └── Test.java
Now, edit Test.java file, and at the beginning of the file, write the package statement as:
package com.test;
Here, any class that is declared within the test directory belongs to the com.test package.
Here's the code:
package com.test; class Test { public static void main(String[] args){ System.out.println("Hello World!"); } }
Output:
Hello World!
Here, the Test class now belongs to the com.test package.
Package Naming convention
The package name must be unique (like a domain name). Hence, there's a convention to create a package as a domain name, but in reverse order. For example, com.company.name
Here, each level of the package is a directory in your file system. Like this:
└── com └── company └── name
And, there is no limitation on how many subdirectories (package hierarchy) you can create.
How to create a package in Intellij IDEA?
In IntelliJ IDEA, here's how you can create a package:
- Right-click on the source folder.
- Go to new and then package.
- A pop-up box will appear where you can enter the package name.
Once the package is created, a similar folder structure will be created on your file system as well. Now, you can create classes, interfaces, and so on inside the package.
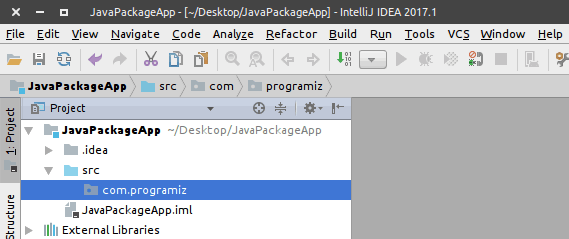
How to import packages in Java?
Java has an import
statement that allows you to import an entire package (as in earlier examples), or use only certain classes and interfaces defined in the package.
The general form of import
statement is:
import package.name.ClassName; // To import a certain class only import package.name.* // To import the whole package
For example,
import java.util.Date; // imports only Date class import java.io.*; // imports everything inside java.io package
The import
statement is optional in Java.
If you want to use class/interface from a certain package, you can also use its fully qualified name, which includes its full package hierarchy.
Here is an example to import a package using the import
statement.
import java.util.Date; class MyClass implements Date { // body }
The same task can be done using the fully qualified name as follows:
class MyClass implements java.util.Date { //body }
Example: Package and importing package
Suppose, you have defined a package com.programiz that contains a class Helper.
package com.programiz; public class Helper { public static String getFormattedDollar (double value){ return String.format("$%.2f", value); } }
Now, you can import Helper class from com.programiz package to your implementation class. Once you import it, the class can be referred directly by its name. Here's how:
import com.programiz.Helper; class UseHelper { public static void main(String[] args) { double value = 99.5; String formattedValue = Helper.getFormattedDollar(value); System.out.println("formattedValue = " + formattedValue); } }
Output:
formattedValue = $99.50
Here,
- the Helper class is defined in com.programiz package.
- the Helper class is imported to a different file. The file contains UseHelper class.
- The
getFormattedDollar()
method of the Helper class is called from inside the UseHelper class.
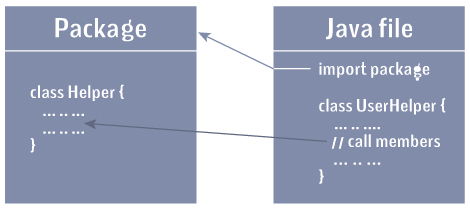
In Java, the import
statement is written directly after the package statement (if it exists) and before the class definition.
For example,
package package.name; import package.ClassName; // only import a Class class MyClass { // body }