The FileWriter
class of the java.io
package can be used to write data (in characters) to files.
It extends the OutputStreamWriter class.
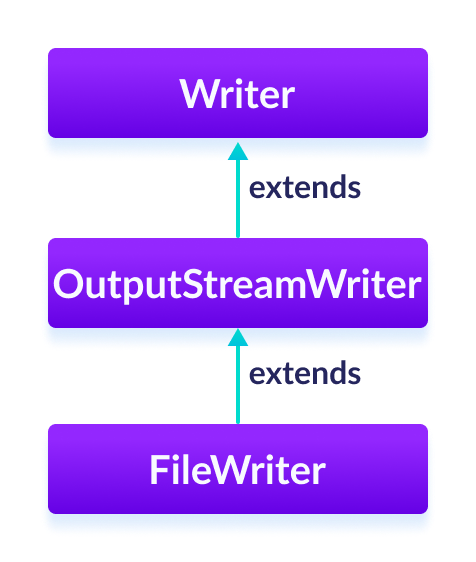
Before you learn more about FileWriter
, make sure to know about Java File.
Create a FileWriter
In order to create a file writer, we must import the Java.io.FileWriter
package first. Once we import the package, here is how we can create the file writer.
1. Using the name of the file
FileWriter output = new FileWriter(String name);
Here, we have created a file writer that will be linked to the file specified by the name.
2. Using an object of the file
FileWriter input = new FileWriter(File fileObj);
Here, we have created a file writer that will be linked to the file specified by the object of the file.
In the above example, the data are stored using some default character encoding.
However, since Java 11 we can specify the type of character encoding (UTF8 or UTF16) as well.
FileWriter input = new FileWriter(String file, Charset cs);
Here, we have used the Charset
class to specify the character encoding of the file writer.
Methods of FileWriter
The FileWriter
class provides implementations for different methods present in the Writer
class.
write() Method
write()
- writes a single character to the writerwrite(char[] array)
- writes the characters from the specified array to the writerwrite(String data)
- writes the specified string to the writer
Example: FileWriter to write data to a File
import java.io.FileWriter;
public class Main {
public static void main(String args[]) {
String data = "This is the data in the output file";
try {
// Creates a FileWriter
FileWriter output = new FileWriter("output.txt");
// Writes the string to the file
output.write(data);
// Closes the writer
output.close();
}
catch (Exception e) {
e.getStackTrace();
}
}
}
In the above example, we have created a file writer named output. The output reader is linked with the output.txt file.
FileWriter output = new FileWriter("output.txt");
To write data to the file, we have used the write()
method.
Here when we run the program, the output.txt file is filled with the following content.
This is a line of text inside the file.
getEncoding() Method
The getEncoding()
method can be used to get the type of encoding that is used to write data. For example,
import java.io.FileWriter;
import java.nio.charset.Charset;
class Main {
public static void main(String[] args) {
String file = "output.txt";
try {
// Creates a FileReader with default encoding
FileWriter output1 = new FileWriter(file);
// Creates a FileReader specifying the encoding
FileWriter output2 = new FileWriter(file, Charset.forName("UTF8"));
// Returns the character encoding of the reader
System.out.println("Character encoding of output1: " + output1.getEncoding());
System.out.println("Character encoding of output2: " + output2.getEncoding());
// Closes the reader
output1.close();
output2.close();
}
catch(Exception e) {
e.getStackTrace();
}
}
}
Output
The character encoding of output1: Cp1252 The character encoding of output2: UTF8
In the above example, we have created 2 file writer named output1 and output2.
- output1 does not specify the character encoding. Hence the
getEncoding()
method returns the default character encoding. - output2 specifies the character encoding, UTF8. Hence the
getEncoding()
method returns the specified character encoding.
Note: We have used the Charset.forName()
method to specify the type of character encoding. To learn more, visit Java Charset (official Java documentation).
close() Method
To close the file writer, we can use the close()
method. Once the close()
method is called, we cannot use the writer to write the data.
Other methods of FileWriter
Method | Description |
---|---|
flush() |
forces to write all the data present in the writer to the corresponding destination |
append() |
inserts the specified character to the current writer |
To learn more, visit Java FileWriter (official Java documentation).