The BufferedReader
class of the java.io
package can be used with other readers to read data (in characters) more efficiently.
It extends the abstract class Reader
.
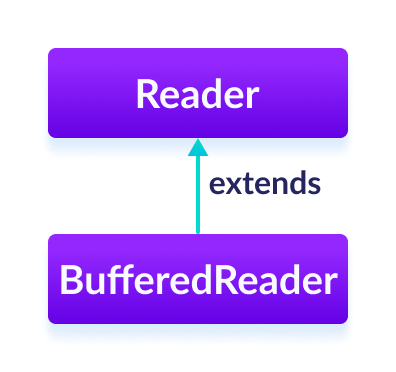
Working of BufferedReader
The BufferedReader
maintains an internal buffer of 8192 characters.
During the read operation in BufferedReader
, a chunk of characters is read from the disk and stored in the internal buffer. And from the internal buffer characters are read individually.
Hence, the number of communication to the disk is reduced. This is why reading characters is faster using BufferedReader
.
Create a BufferedReader
In order to create a BufferedReader
, we must import the java.io.BuferedReader
package first. Once we import the package, here is how we can create the reader.
// Creates a FileReader
FileReader file = new FileReader(String file);
// Creates a BufferedReader
BufferedReader buffer = new BufferedReader(file);
In the above example, we have created a BufferedReader
named buffer with the FileReader
named file.
Here, the internal buffer of the BufferedReader
has the default size of 8192 characters. However, we can specify the size of the internal buffer as well.
// Creates a BufferdReader with specified size internal buffer
BufferedReader buffer = new BufferedReader(file, int size);
The buffer will help to read characters from the files more quickly.
Methods of BufferedReader
The BufferedReader
class provides implementations for different methods present in Reader
.
read() Method
read()
- reads a single character from the internal buffer of the readerread(char[] array)
- reads the characters from the reader and stores in the specified arrayread(char[] array, int start, int length)
- reads the number of characters equal to length from the reader and stores in the specified array starting from the position start
For example, suppose we have a file named input.txt with the following content.
This is a line of text inside the file.
Let's try to read the file using BufferedReader
.
import java.io.FileReader;
import java.io.BufferedReader;
class Main {
public static void main(String[] args) {
// Creates an array of character
char[] array = new char[100];
try {
// Creates a FileReader
FileReader file = new FileReader("input.txt");
// Creates a BufferedReader
BufferedReader input = new BufferedReader(file);
// Reads characters
input.read(array);
System.out.println("Data in the file: ");
System.out.println(array);
// Closes the reader
input.close();
}
catch(Exception e) {
e.getStackTrace();
}
}
}
Output
Data in the file: This is a line of text inside the file.
In the above example, we have created a buffered reader named input. The buffered reader is linked with the input.txt file.
FileReader file = new FileReader("input.txt");
BufferedReader input = new BufferedReader(file);
Here, we have used the read()
method to read an array of characters from the internal buffer of the buffered reader.
skip() Method
To discard and skip the specified number of characters, we can use the skip()
method. For example,
import java.io.FileReader;
import java.io.BufferedReader;
public class Main {
public static void main(String args[]) {
// Creates an array of characters
char[] array = new char[100];
try {
// Suppose, the input.txt file contains the following text
// This is a line of text inside the file.
FileReader file = new FileReader("input.txt");
// Creates a BufferedReader
BufferedReader input = new BufferedReader(file);
// Skips the 5 characters
input.skip(5);
// Reads the characters
input.read(array);
System.out.println("Data after skipping 5 characters:");
System.out.println(array);
// closes the reader
input.close();
}
catch (Exception e) {
e.getStackTrace();
}
}
}
Output
Data after skipping 5 characters: is a line of text inside the file.
In the above example, we have used the skip()
method to skip 5 characters from the file reader. Hence, the characters 'T'
, 'h'
, 'i'
, 's'
and ' '
are skipped from the original file.
close() Method
To close the buffered reader, we can use the close()
method. Once the close()
method is called, we cannot use the reader to read the data.
Other Methods of BufferedReader
Method | Description |
---|---|
ready() |
checks if the file reader is ready to be read |
mark() |
mark the position in reader up to which data has been read |
reset() |
returns the control to the point in the reader where the mark was set |
To learn more about BufferedReader
, visit Java BufferedReader (official Java documentation).