Templates are powerful features of C++ which allows us to write generic programs.
We can create a single function to work with different data types by using a template.
Defining a Function Template
A function template starts with the keyword template
followed by template parameter(s) inside <>
which is followed by the function definition.
template <typename T>
T functionName(T parameter1, T parameter2, ...) {
// code
}
In the above code, T
is a template argument that accepts different data types (int
, float
, etc.), and typename
is a keyword.
When an argument of a data type is passed to functionName()
, the compiler generates a new version of functionName()
for the given data type.
Calling a Function Template
Once we've declared and defined a function template, we can call it in other functions or templates (such as the main()
function) with the following syntax
functionName<dataType>(parameter1, parameter2,...);
For example, let us consider a template that adds two numbers:
template <typename T>
T add(T num1, T num2) {
return (num1 + num2);
}
We can then call it in the main()
function to add int
and double
numbers.
int main() {
int result1;
double result2;
// calling with int parameters
result1 = add<int>(2, 3);
cout << result1 << endl;
// calling with double parameters
result2 = add<double>(2.2, 3.3);
cout << result2 << endl;
return 0;
}
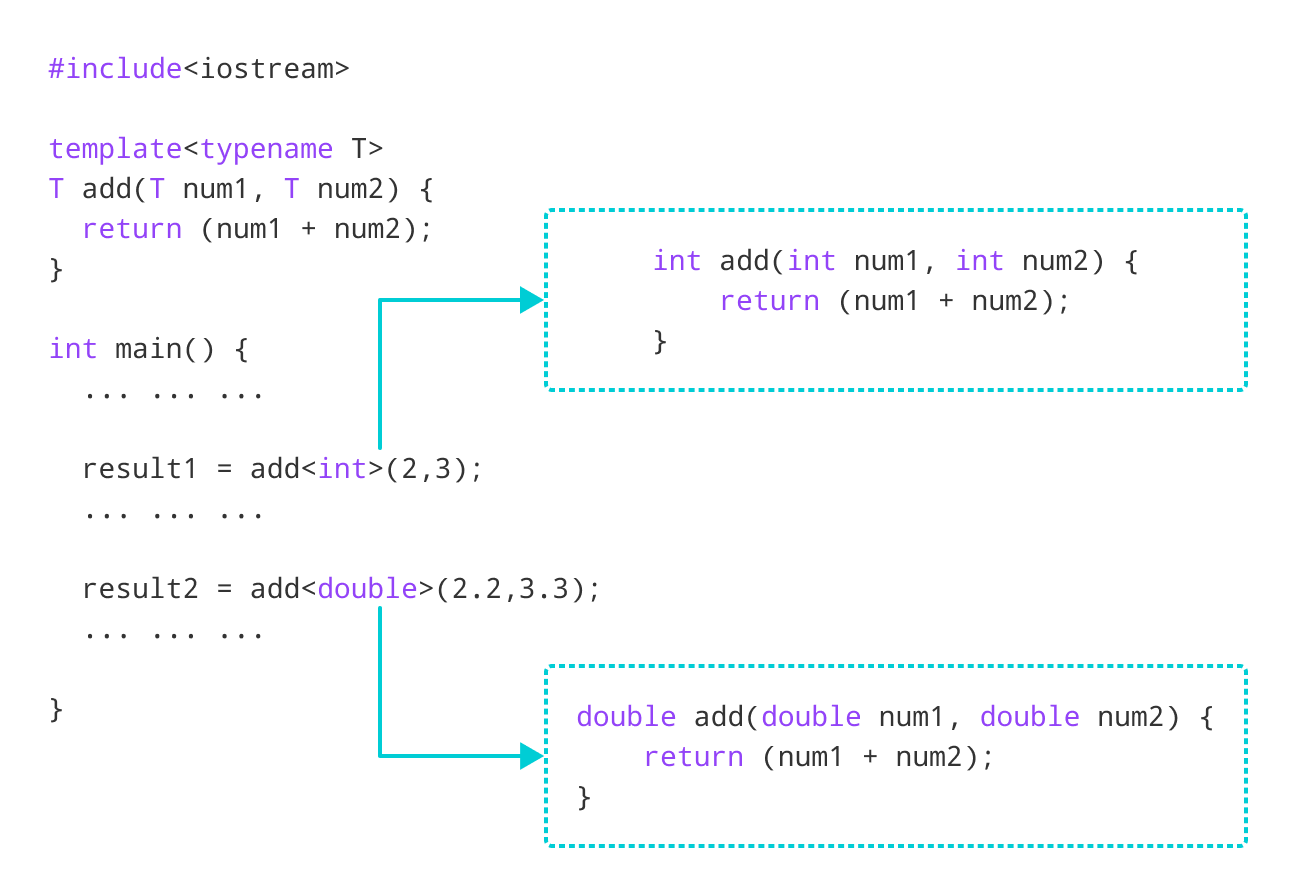
Example: Adding Two Numbers Using Function Templates
#include <iostream>
using namespace std;
template <typename T>
T add(T num1, T num2) {
return (num1 + num2);
}
int main() {
int result1;
double result2;
// calling with int parameters
result1 = add<int>(2, 3);
cout << "2 + 3 = " << result1 << endl;
// calling with double parameters
result2 = add<double>(2.2, 3.3);
cout << "2.2 + 3.3 = " << result2 << endl;
return 0;
}
Output
2 + 3 = 5 2.2 + 3.3 = 5.5