Java is a high level, class based, object oriented programming language that is widely used across various operating systems.
To build Java programs, you need to have the Java Development Kit (JDK) installed on your device. However, if you're eager to start immediately, you can use our free online Java compiler that allows you to run Java code directly in your browser without any setup.
For those who prefer to use Java on their local machine, this guide will walk you through the installation process on Windows, macOS, and Linux (Ubuntu).
Install Java on Windows
Follow the steps below to install Java on Windows:
- Download JDK(Java Development Kit)
- Run the Installer
- Configure Environment Variables
- Verify Installation
Here's a detailed explanation of each of the steps.
Step 1: Download JDK
Go to the official Oracle website to download the JDK.
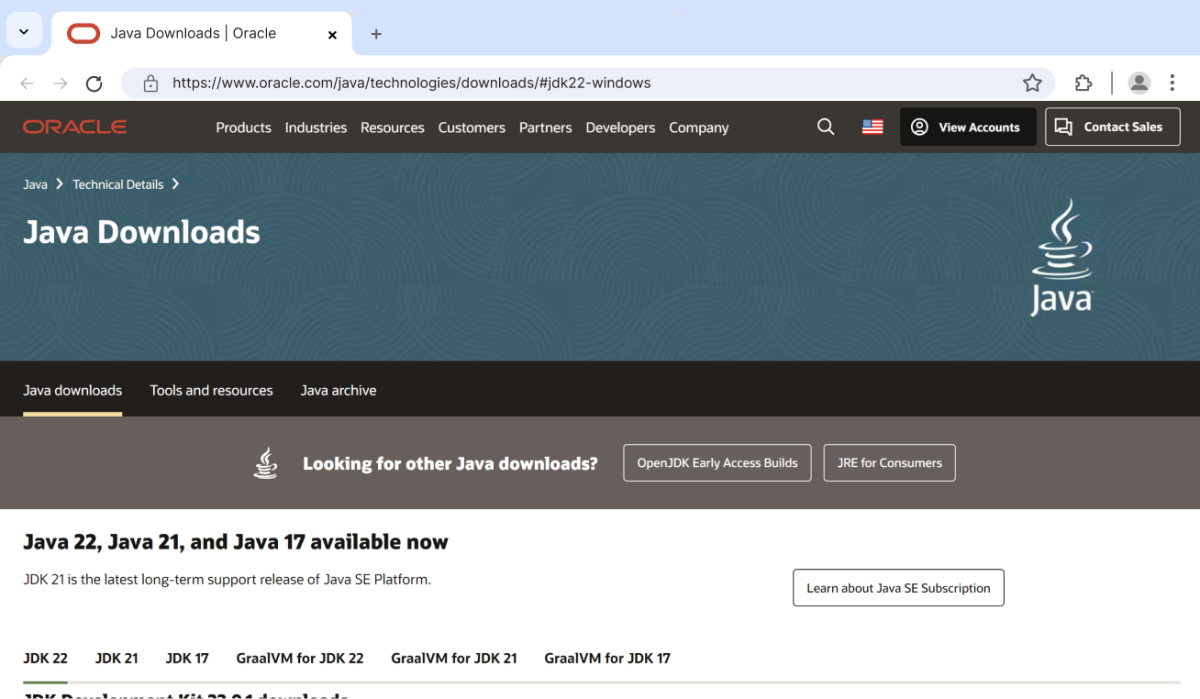
Choose x64 MSI Installer on the windows tab and click on download link.
Step 2: Run the Installer
Now, go to your downloads folder and run the installer you just downloaded. You will be prompted to the screen below.
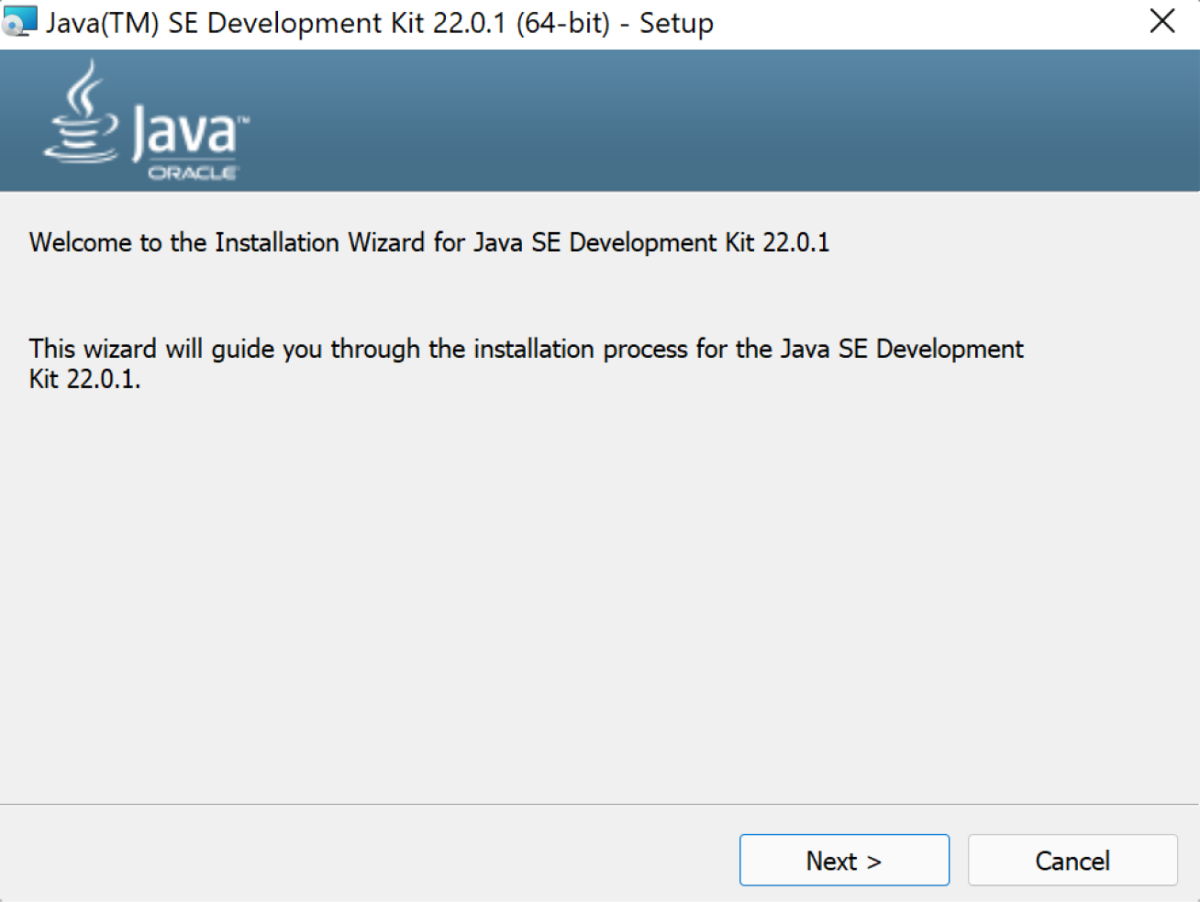
Simply click Next to proceed.
Step 3: Configure Environment Variables
After installation, you will need to tell your system where to find Java. This is done by setting environment variables.
Locate JDK Path: Navigate through your file explorer to reach the JDK installation directory. Normally, it is located at
C:\Program Files\Java\jdk-22\bin
Copy this path.
Access Environment Variables: Search environment variable on the terminal. In system properties, click on environment variables. You will be prompted to the screen below.
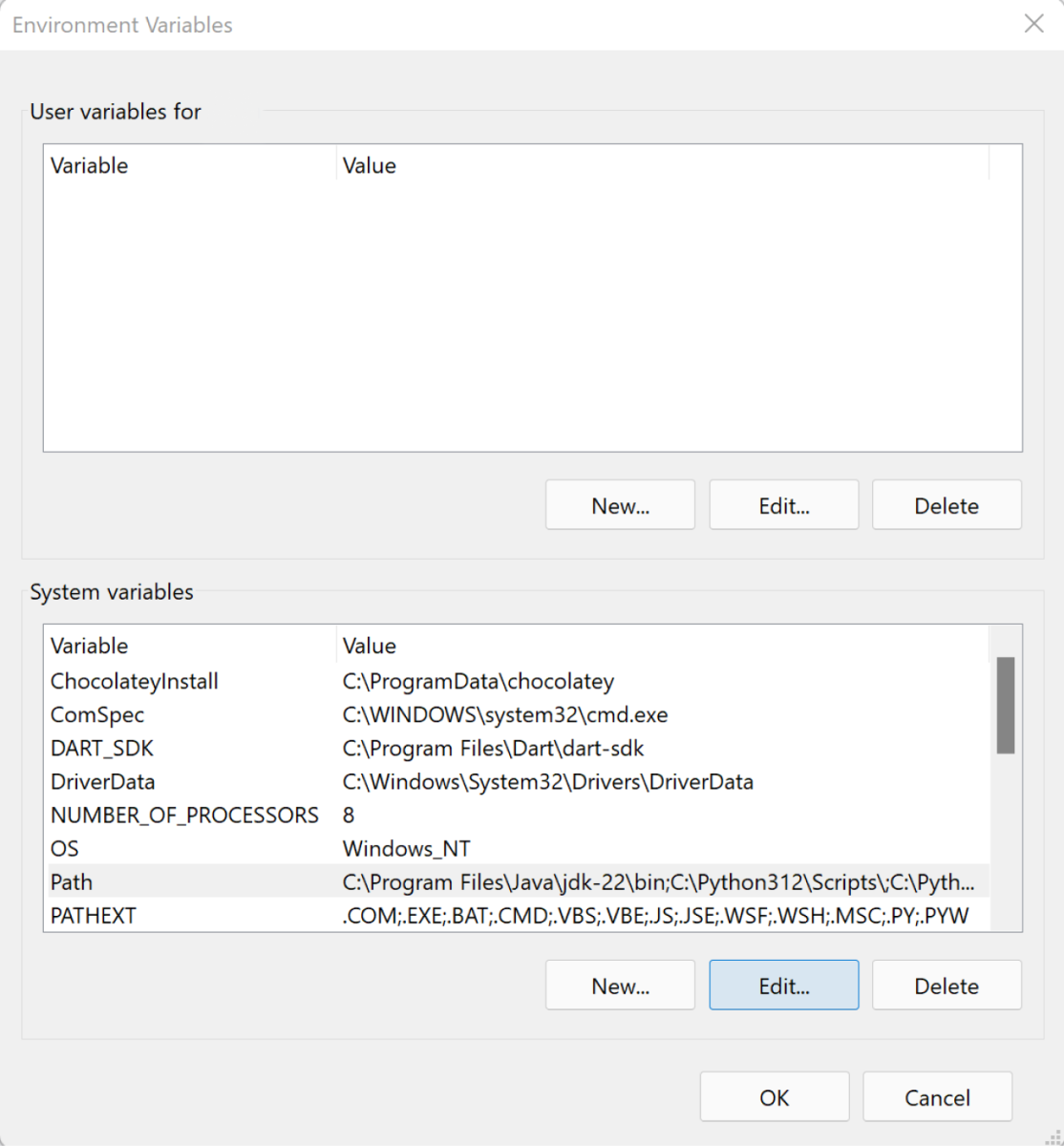
Update the Path Variable:
Find the Path variable in the System variables section and click on Edit. Then, click New and paste your JDK bin path (i.e. C:\Program Files\Java\jdk-22\bin).
Finally, click Ok to close each window.
Set JAVA_HOME Variable:
Back in the environment variables window, under the system variables section, click New to create a new variable.
Now, name the variable JAVA_HOME and set its value to the path of the JDK folder directory (i.e.C:\Program Files\Java\jdk-22).
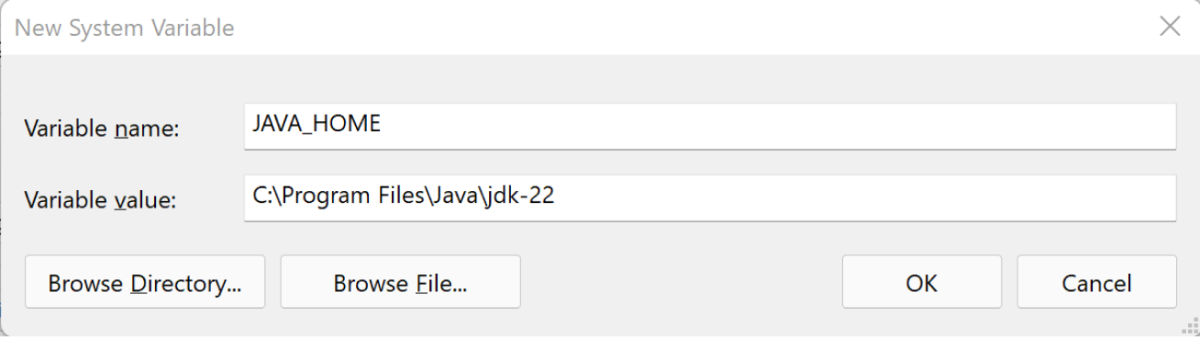
Close all the dialogues with the Ok button.
Step 4: Verify your Installation
After the installation is complete, you can verify whether Java is installed by using the following command in the command prompt.
java --version
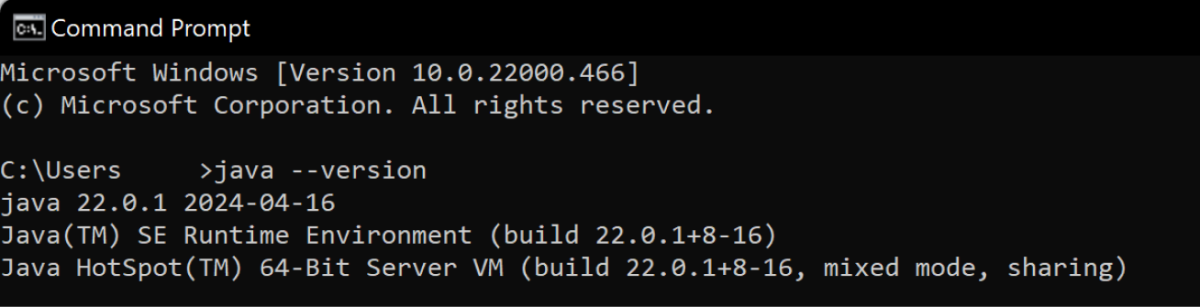
If Java is installed successfully, it will print the version information; otherwise, it will produce an error message indicating that the command is not recognized.
Install Java on macOS
To install Java on macOS, follow the steps below:
- Check Java version
- Download Java Development Kit (JDK)
- Run the Installer
- Configure Environment Variables
- Verify your Installation
Here is a detailed explanation of each of the steps:
Step 1: Check Java Version
Since older versions of macOS may come with Java pre-installed on it. You can check the current version by using the following command in the Terminal app.
java --version
If Java is installed, you'll see the version information and can skip the remaining steps. Otherwise, follow the steps below.
Step 2: Download JDK
Go to the official Oracle website to download the JDK (JDK 22 at the time of writing this tutorial) for macOS.
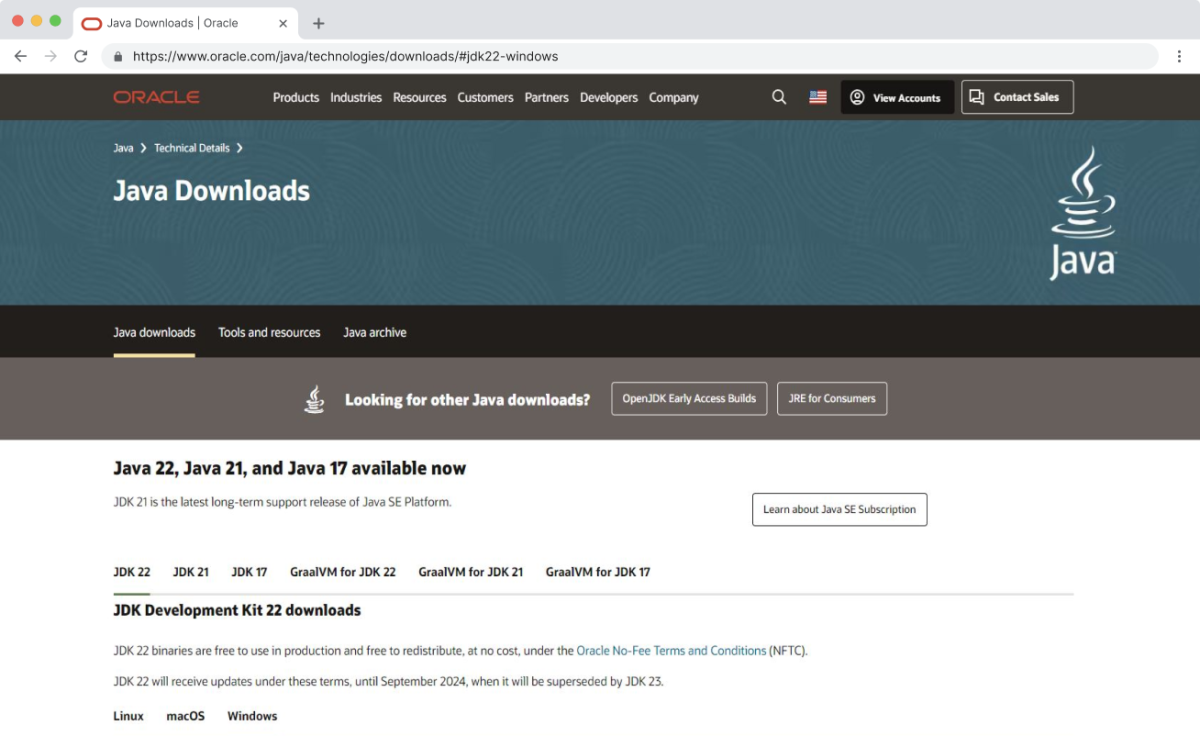
The appropriate installer for Java depends on your Mac's chip architecture:
For Intel-based Macs:
Download the macOS x64 DMG Installer
For Apple Silicon (M1) Macs:
Download the macOS ARM64 DMG Installer.
Note: In case you downloaded the wrong installer, you will be prompted with the correct installer on opening the installer file.
Step 3: Run the Installer
Once the download is complete, run the installer you just downloaded. You will be prompted to the screen below.
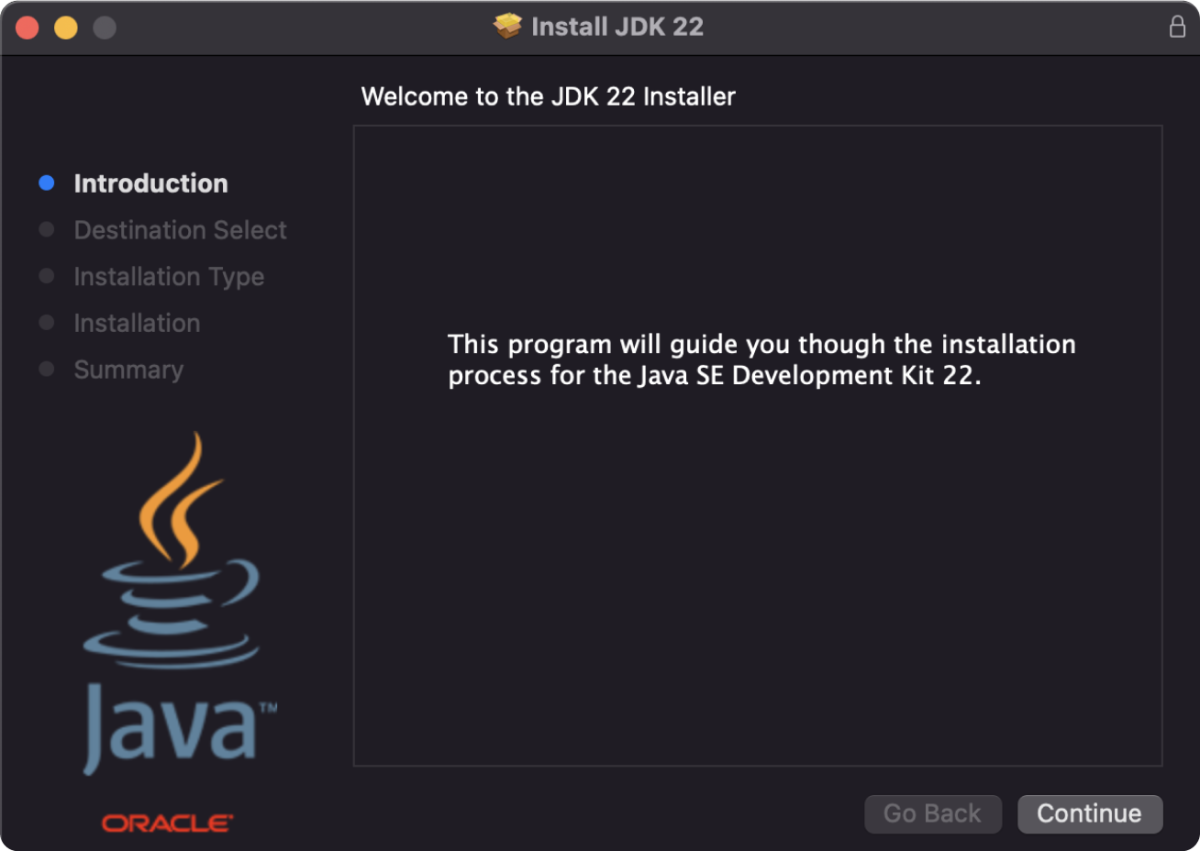
Follow the on-screen instructions to initiate the installation process. You may need to enter your administrator password as well.
Step 4: Configure Environment Variables
After the installation, you need to set up environment variables to tell your system where to find Java.
At first, open Terminal app and type the following command:
nano ~/.bash_profile
This will open the .bash_profile file in a text editor.
Now, add the following lines at the end of the file to set the JAVA_HOME variable.
export JAVA_HOME=$(/usr/libexec/java_home)
export PATH=$JAVA_HOME/bin:$PATH
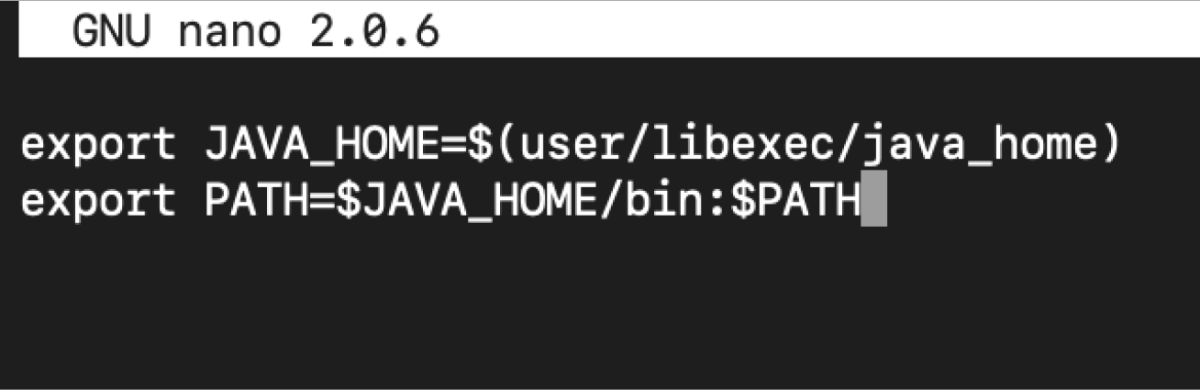
Press Ctrl + X to exit, then Y to save the changes, and Enter to confirm the filename.
Close and reopen the terminal for the changes to take effect.
Step 5: Verify your Installation
Once the installation is complete, you can verify whether Java is installed by using the following command in the Terminal app.
java --version
If Java is installed successfully, it will print the version information; otherwise, it will produce an error message indicating that the command is not recognized.
Install Java on Linux
Linux has various distributions and the installation process differs slightly from each other. For now, we will focus on Ubuntu distribution.
Follow the steps below to install Java on your Ubuntu system:
- Install Java
- Verify Installation
Step 1: Install Java
Open the Terminal and update your package list to ensure you get the latest version of the software:
sudo apt update
Install the default JDK with:
sudo apt install default-jdk
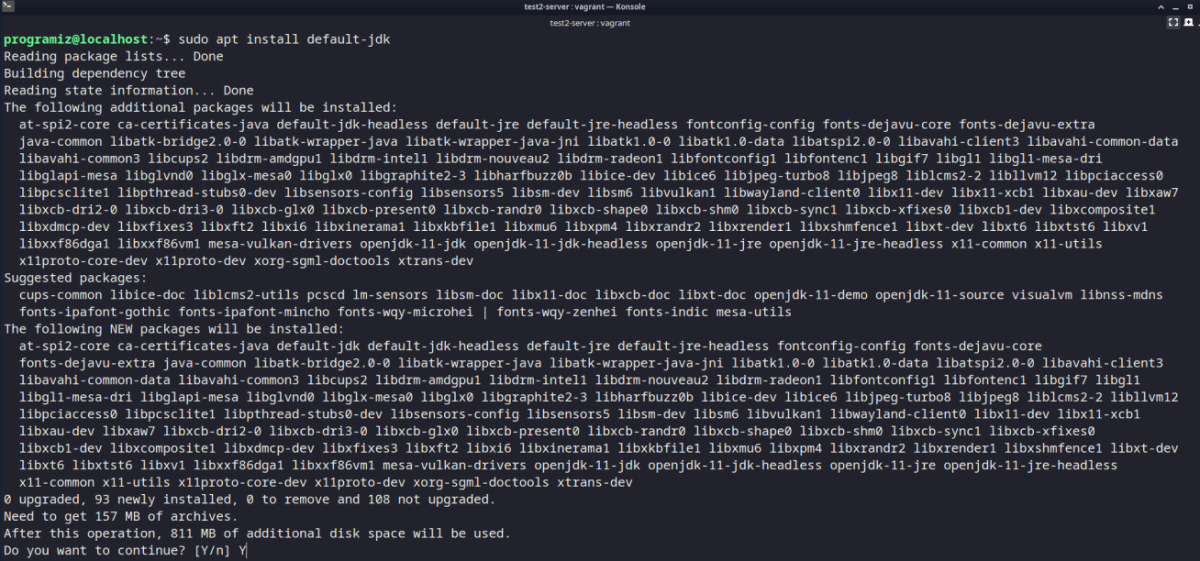
Step 2: Verify Installation
Check if Java was installed successfully:
java --version
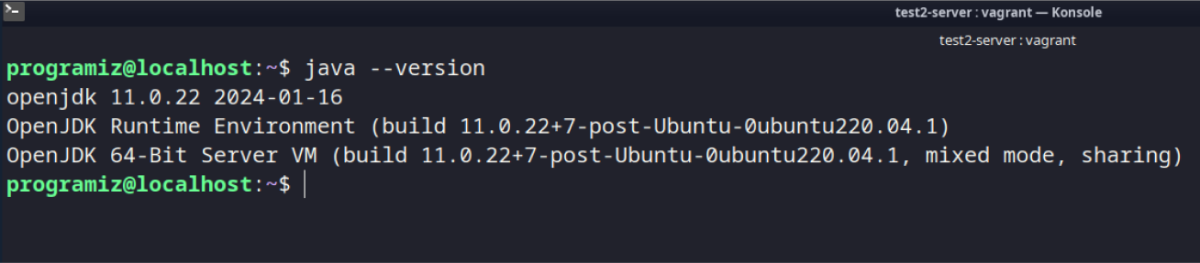
If Java is installed successfully, it will print the version information; otherwise, it will produce an error message indicating that the command is not recognized.
Run Your First Java Program
Open Notepad (or any editor you prefer) and enter the following code in the new file.
class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Then save this file as HelloWorld.java (select "All Files" in "Save as type").
Now, open your command prompt, navigate to the location of that program, and compile with
javac HellowWorld.java
Then, execute your program with
java HelloWorld
You should see Hello World printed to the command prompt.
Now that you have set everything up to run Java programs on your computer, you'll be learning how the basic program works in Java in the next tutorial.