A class is a blueprint for creating objects.
The JavaScript class was introduced recently in ES6 (ECMAScript2015). The old way to create objects was using constructor functions.
You can think of a class as a blueprint (prototype) of a house. It contains all the details about the floors, rooms, architecture, etc.
Based on these descriptions, you can build a house. Here, the house is the object.
Just like multiple houses can be built from the same blueprint, we can create many objects from a single class.
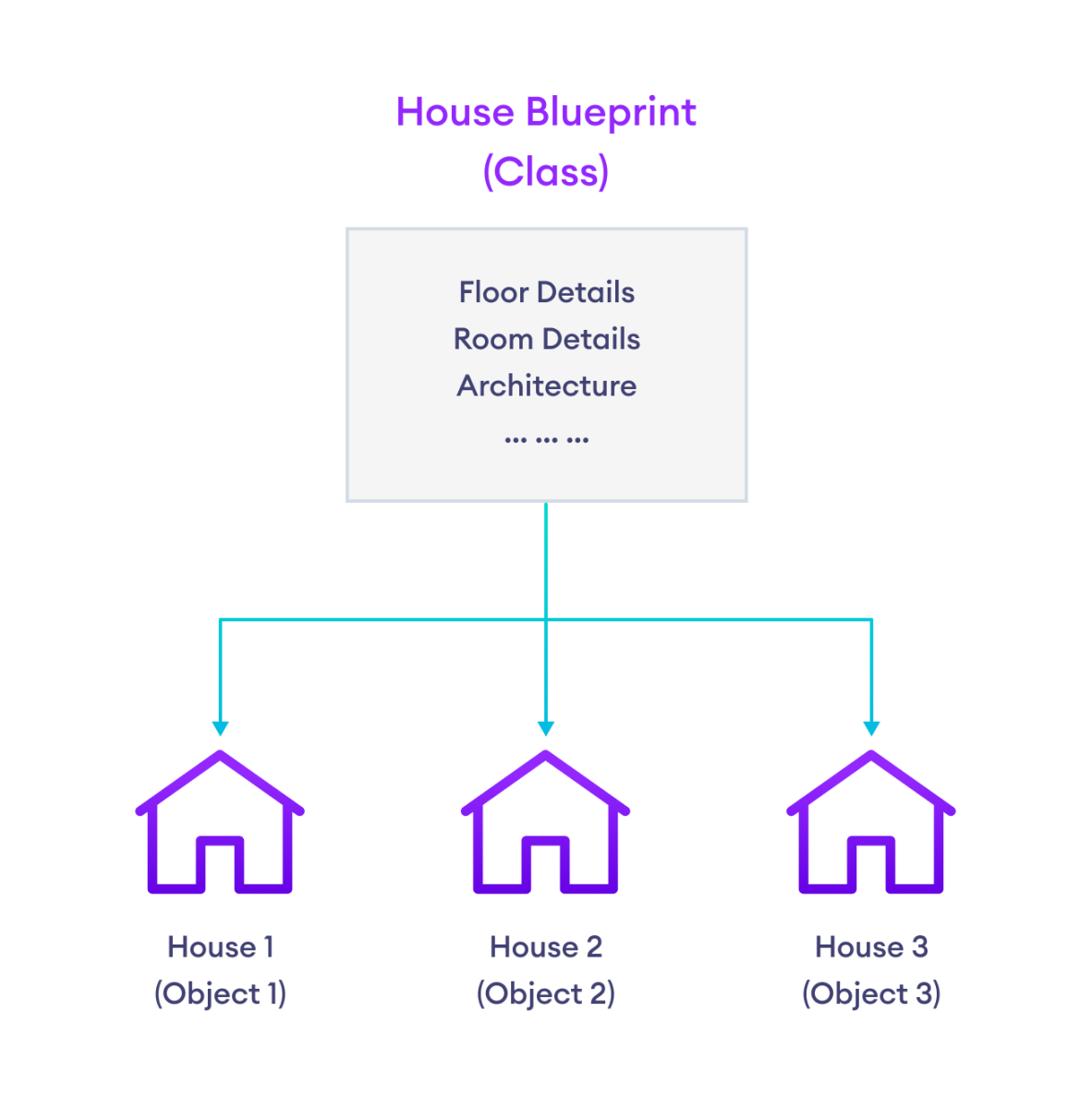
Create JavaScript Class
We use the class
keyword to create a JavaScript class. For example,
// create a class
class Rectangle {
// body of class
};
Class Property
A property is a variable associated with a class. For example,
class Rectangle {
// class properties
width;
height;
};
Note: We don't need to declare class properties using declaration keywords like let
, var
, or const
.
Class Method
A method is a function associated with a class. For example,
class Rectangle {
width;
height;
// class method
calculateArea() {
let area = this.width * this.height
return area;
};
};
Here, we added the calculateArea()
method to find the area of a rectangle.
The calculateArea()
method uses the class properties — width and height — and returns area.
Note: The JavaScript class is a constructor function itself, and it is essentially a more convenient way to define constructor functions. You can confirm it by using the typeof
operator. For example,
class Rectangle {}
console.log(typeof Rectangle);
// Output: function
Class Constructor
Every class has a special method called a constructor. The constructor()
method allows us to assign values to class properties or perform any setup. For example,
class Rectangle {
width;
height;
// class constructor
constructor(newWidth, newHeight) {
this.width = newWidth;
this.height = newHeight;
};
calculateArea() {
let area = this.width * this.height
return area;
};
};
Here, we created a constructor for the Rectangle
class that accepts two parameters: newWidth and newHeight.
The constructor then assigns newWidth and newHeight to the class properties — width and height, respectively.
Note: The constructor()
method is called automatically whenever we create a new object.
Class Objects
An object is an instance of a class. Each object has its own property and method, as defined in the class.
The syntax to create an object is:
let objectName = new ClassName();
Here, we used the let
keyword to declare objectName. But, you can use any other declaration keyword.
Now, let's continue with the previous example,
class Rectangle {
width;
height;
constructor(newWidth, newHeight) {
this.width = newWidth;
this.height = newHeight;
};
calculateArea() {
let area = this.width * this.height
return area;
};
};
// create an object
let rect = new Rectangle(20, 10);
In the above example, we created an object of the Rectangle
class called rect.
Here, new Rectangle()
automatically calls the constructor of Rectangle
.
So, when we pass 20 and 10 as arguments in Rectangle()
, the constructor takes them as parameters and assigns the values to the class properties width and height, respectively.
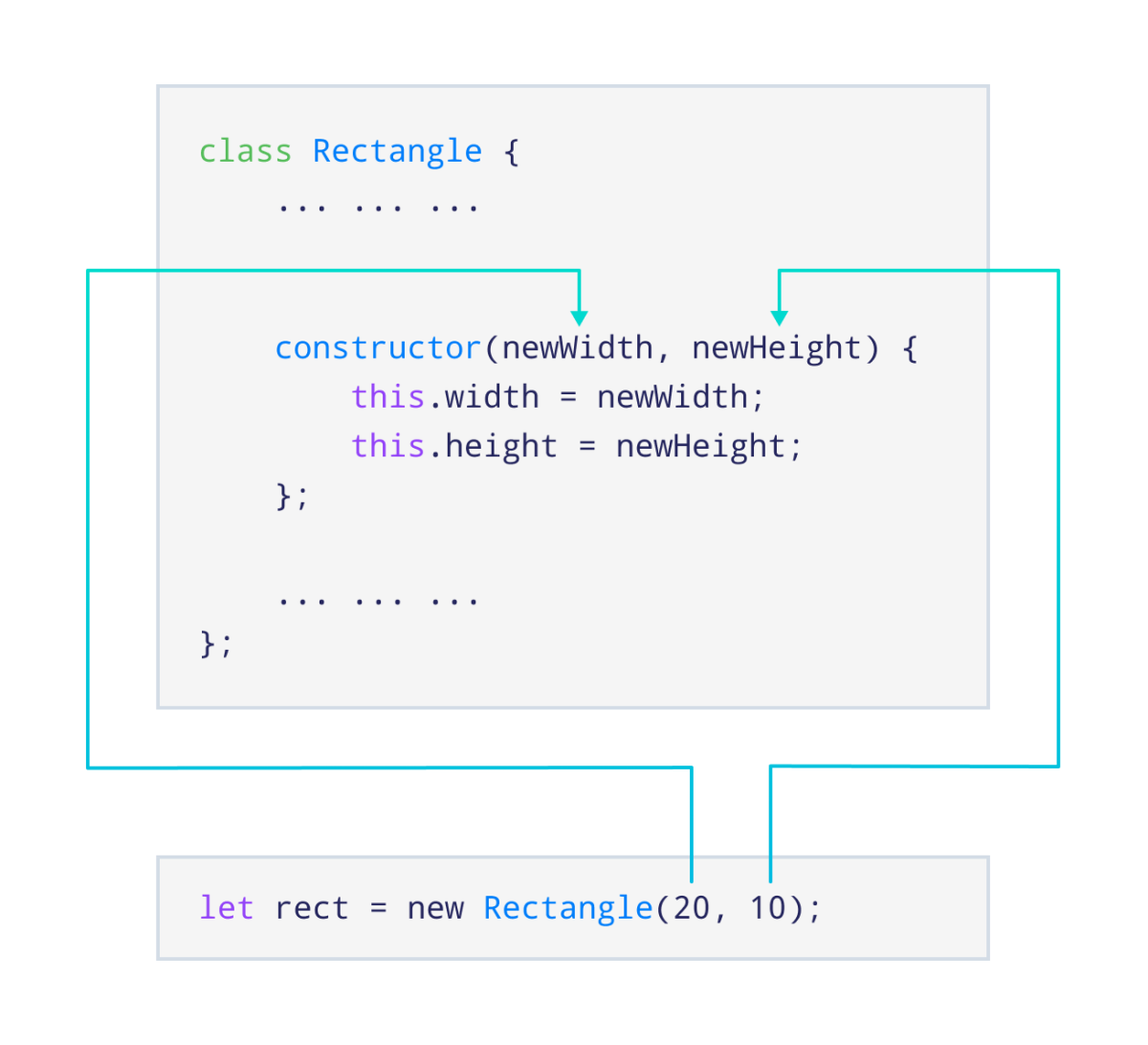
Access Property and Method
We can access the class properties and methods using the dot notation with objects. For example,
class Rectangle {
width;
height;
constructor(newWidth, newHeight) {
this.width = newWidth;
this.height = newHeight;
};
calculateArea() {
let area = this.width * this.height;
return area;
};
};
// object of Rectangle
let rect = new Rectangle(20, 10);
// access properties
console.log("width:", rect.width);
console.log("height:", rect.height);
// access method
console.log("area:", rect.calculateArea());
Output
width: 20 height: 10 area: 200
In the above program, we created an object of the Rectangle
class named rect. Here,
rect.width
andrect.height
access the class properties width and height, respectively.rect.calculateArea()
accesses the class methodcalculateArea()
.
Accessor Properties
We can also access the properties of a class using two special methods called getter and setter.
We use the get
keyword for getter methods to retrieve the value of a property.
Note: it's good practice to prefix the properties of a class that uses getter and setter methods with an underscore _
.
For example,
class Person {
constructor(name) {
this._name = name;
};
// getter
get getName() {
return `My name is ${this._name}`;
};
};
// create object
let per = new Person("Jack");
// use getter to get name
console.log(per.getName);
// Output: My name is Jack
In the above example, we create the Person
class with a property named _name.
Then, we use the getName()
getter to retrieve the value of _name.
Note: Here, we used the constructor()
method to declare and initialize the _name property. This is a valid method of declaring class properties.
We use the set
keyword for setter methods to set the value of a property. For example,
class Person {
// setter
set setName(name) {
this._name = name;
};
get getName() {
return `My name is ${this._name}`;
};
};
let per = new Person();
// use setter to set name
per.setName = "Tony";
// use get to get name
console.log(per.getName);
// Output: My name is Tony
In the above example, we used the setName()
setter method to initialize the value of _name.
Then we retrieved it using the getName()
getter method.
Note: We didn't use a constructor method in the above example since we used the setter method to initialize the _name property.
Classes Aren't Hoisted
Unlike JavaScript functions and other declarations, the class is not hoisted. That means creating objects of a class before defining the class causes errors. For example,
// create a class object
let per = new Person();
// define the class
class Person {
// body of class
};
// Output: ReferenceError: Cannot access 'Person' before initialization
As you can see, accessing a class before defining it causes an error.
To learn more about hoisting, visit JavaScript Hoisting.
Classes Use Strict Mode
Classes are in "use strict" mode by default. For example,
class Dog {
constructor() {
// declare without let, var, const
tempAge = 8;
this.age = tempAge;
}
}
let dog = new Dog();
// Output: ReferenceError: tempAge is not defined
In the above example, we directly assigned the value 8 to the tempAge variable without declaring it.
In strict mode, using variables without declaring them first causes errors.
Thus, we get the output ReferenceError: tempAge is not defined
.
Also Read: