In JavaScript, recursion refers to a technique where a function calls itself.
The syntax of a recursive function is:
function recurse() {
// body of function
// call function recursively
recurse();
// body of function
}
recurse();
In the above example, recurse()
calls itself through its body.
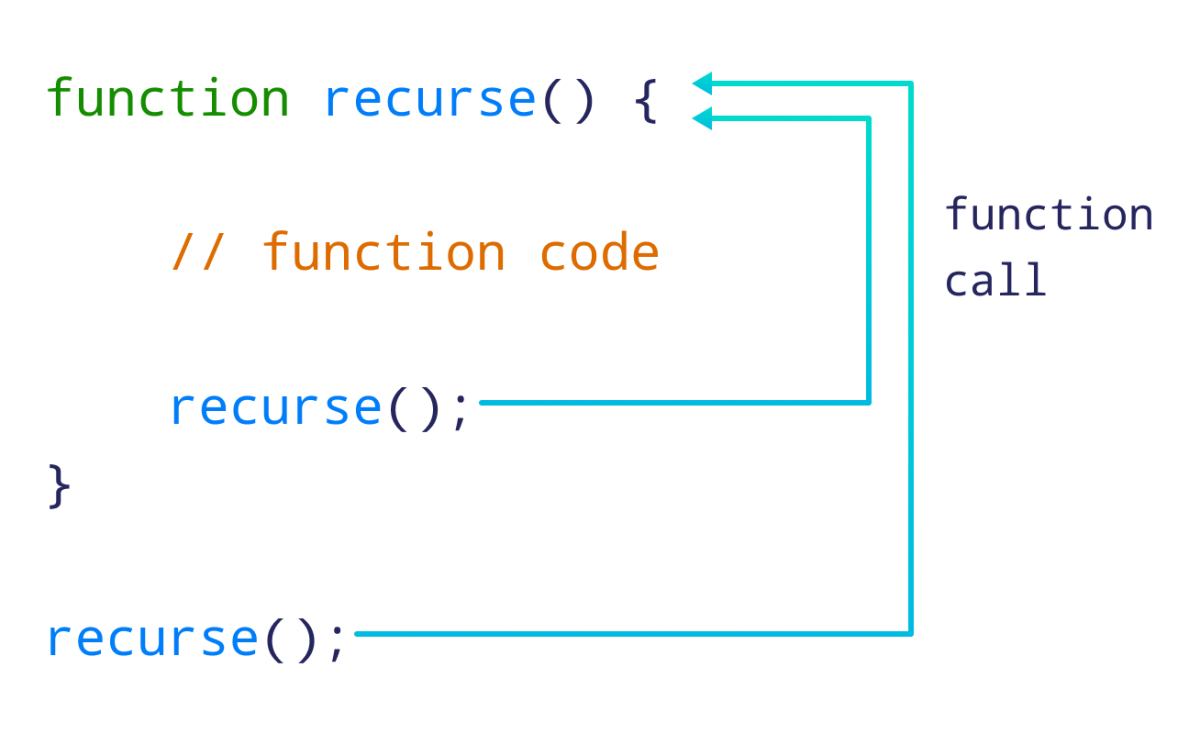
Base Case
A base case is a condition that determines when the recursion should stop.
Every recursive function must have a base case. Otherwise, the function is called infinitely.
We use conditional statements such as if...else
or the ternary operator to create base cases.
The syntax of a recursive function with a base condition looks like this:
function recurse() {
// base case
if(condition) {
recurse();
}
else {
// stop calling recurse()
}
}
recurse();
Flowchart of Recursive Function
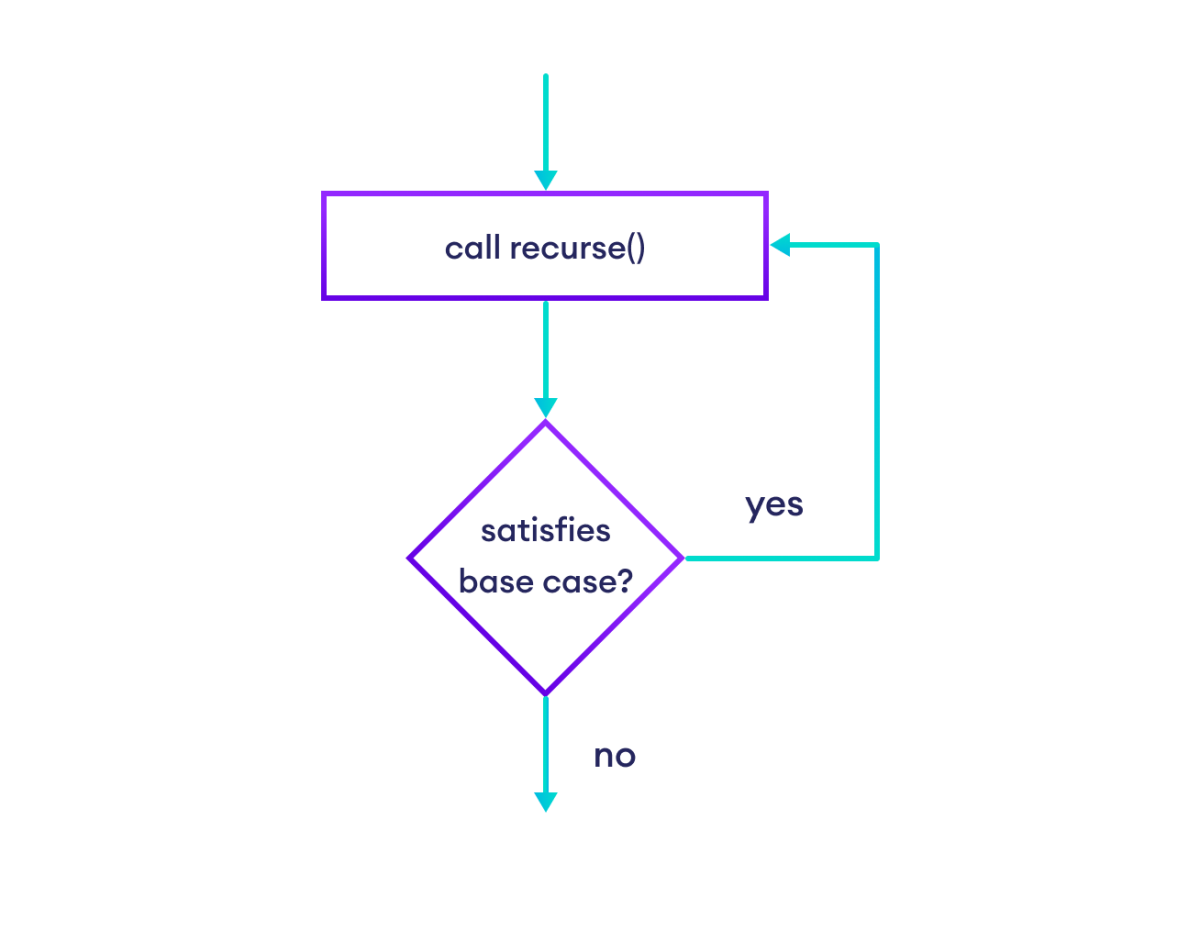
Example 1: Countdown Till 1
// Program to count down numbers to 1
// recursive function
function countDown(num) {
// display num
console.log(num);
// decrease num
// then, assign new value to newNum
const newNum = num - 1;
// base case
if (newNum > 0) {
// call countDown with newNum
countDown(newNum);
}
}
countDown(3);
Output
3 2 1
In the above program, we have called the countDown()
function recursively.
The base case is newNum > 0
, which means countDown()
must keep calling itself as long as the value of newNum is greater than 0.
Here is how the above program works:
Iteration | Variable | Base case: newNum > 0 | Action |
---|---|---|---|
1st | num = 3 newNum = 2 |
true |
3 is printed. newNum becomes 2. countDown() is called. |
2nd | num = 2 newNum = 1 |
true |
2 is printed. newNum becomes 1. countDown() is called. |
3rd | num = 1 newNum = 0 |
false |
1 is printed. newNum becomes 0. countDown() isn't called. |
Example 2: Find Factorial
// Program to find the factorial of a number
// recursive function
function factorial(num) {
// base case
// recurse only if num is greater than 0
if (num > 1) {
return num * factorial(num - 1);
}
else {
return 1;
}
}
let x = 3;
// store result of factorial() in variable
let y = factorial(x);
console.log(`The factorial of ${x} is ${y}`);
Output
The factorial of 3 is 6
Here, the factorial()
function calls itself recursively as long as the num variable is greater than 1.
We can divide the overall recursion process into two halves.
The first half includes:
Iteration | Variable | Base case: num > 1 | Action |
---|---|---|---|
1st | num = 3 |
true |
factorial(3) returns 3 * factorial(2) |
2nd | num = 2 |
true |
factorial(2) returns 2 * factorial(1) |
3rd | num = 1 |
false |
factorial(1) returns 1 |
This is how the first half of the recursive process works:
- First,
factorial(3)
returns3 * factorial(2)
and waits forfactorial(2)
to compute. - Then,
factorial(2)
returns2 * factorial(1)
and waits forfactorial(1)
to compute. - Finally,
factorial(1)
doesn't pass the base case, so it directly returns 1.
After that, the second half takes place in reverse:
Iteration | Variable | Base case: num > 1 | Action |
---|---|---|---|
3rd | num = 1 |
false |
factorial(1) returns 1 |
2nd | num = 2 |
true |
factorial(2) returns 2 * 1 |
1st | num = 3 |
true |
factorial(3) returns 3 * 2 |
Here, after factorial(1)
returns 1, factorial(2)
returns 2 * 1
.
Finally, factorial(3)
returns 3 * 2
, which is stored in the result variable.
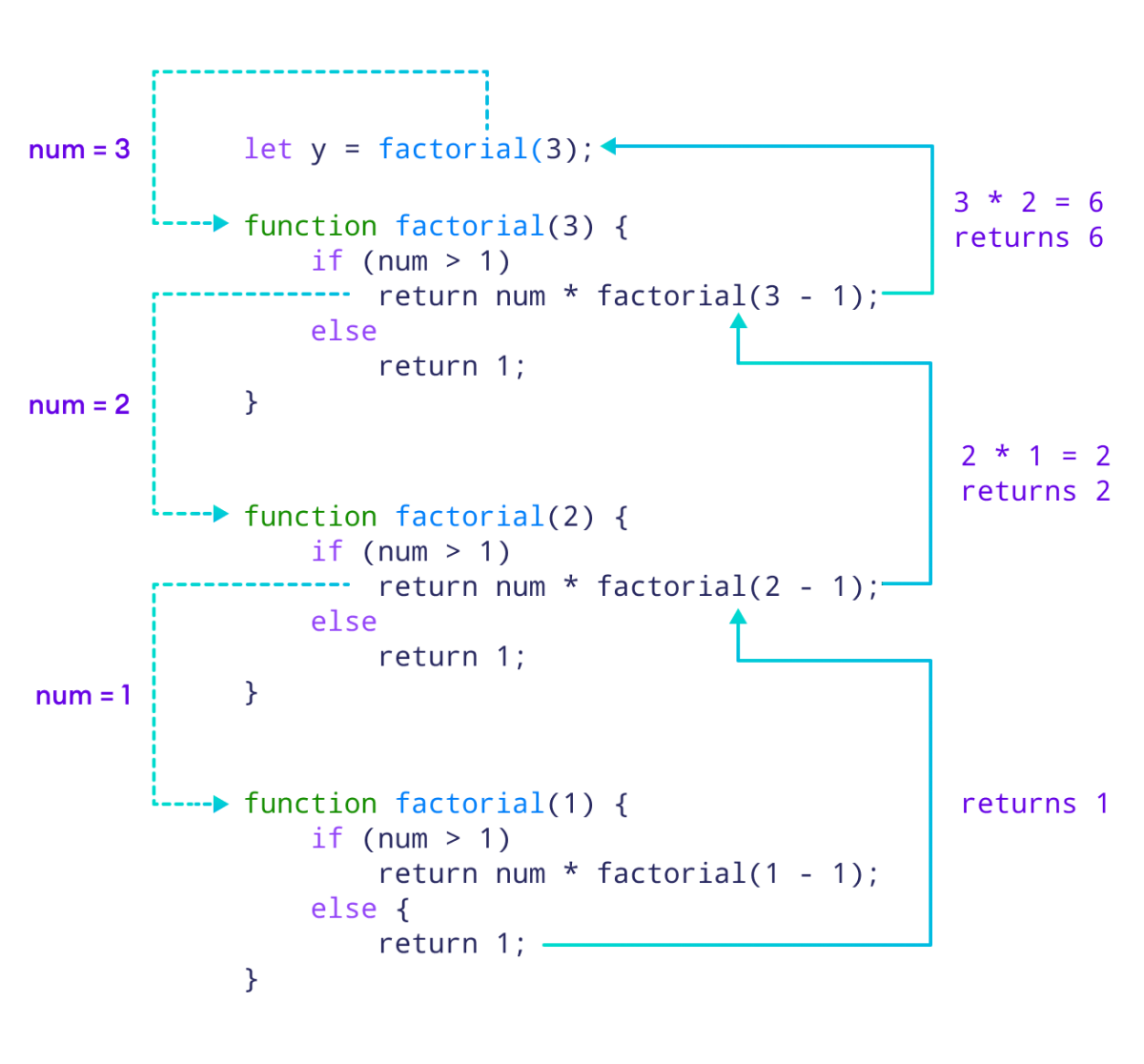
Frequently Asked Questions
Yes, JavaScript does have a recursion limit.
The recursion limit prevents errors caused by too many nested function calls.
However, the limit varies depending on the JavaScript engine and the environment in which the code is running.
For instance, the maximum limit can differ between Firefox and Chrome. Whereas, devices with higher memory might have higher recursion limits than devices with less memory.