A matrix is a two-dimensional data structure where data are arranged into rows and columns. For example,
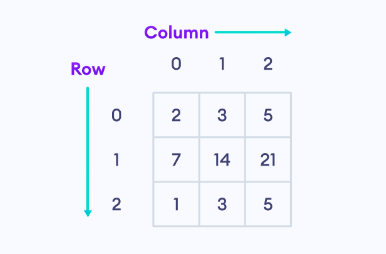
Here, the above matrix is 3 * 3 (pronounced "three by three") matrix because it has 3 rows and 3 columns.
Create a Matrix in R
In R, we use the matrix()
function to create a matrix.
The syntax of the matrix()
function is
matrix(vector, nrow, ncol)
Here,
- vector - the data items of same type
nrow
- number of rowsncol
- number of columns- byrow (optional) - if
TRUE
, the matrix is filled row-wise. By default, the matrix is filled column-wise.
Let's see an example,
# create a 2 by 3 matrix
matrix1 <- matrix(c(1, 2, 3, 4, 5, 6), nrow = 2, ncol = 3, byrow = TRUE)
print(matrix1)
Output
[,1] [,2] [,3] [1,] 1 2 3 [2,] 4 5 6
In the above example, we have used the matrix()
function to create a matrix named matrix1.
matrix(c(1, 2, 3, 4, 5, 6), nrow = 2, ncol = 3, byrow = TRUE)
Here, we have passed data items of integer type and used c()
to combine data items together. And nrow = 2
and ncol = 3
means the matrix has 2 rows and 3 columns.
Since we have passed byrow = TRUE
, the data items in the matrix are filled row-wise. If we didn't pass byrow argument as
matrix(c(1, 2, 3, 4, 5, 6), nrow = 2, ncol = 3)
The output would be
[,1] [,2] [,3] [1,] 1 3 5 [2,] 2 4 6
Access Matrix Elements in R
We use the vector index operator [ ]
to access specific elements of a matrix in R.
The syntax to access a matrix element is
matrix[n1, n2]
Here,
n1
- specifies the row positionn2
- specifies the column position
Let's see an example,
matrix1 <- matrix(c("Sabby", "Cathy", "Larry", "Harry"), nrow = 2, ncol = 2)
print(matrix1)
# access element at 1st row, 2nd column
cat("\nDesired Element:", matrix1[1, 2])
Output
[,1] [,2] [1,] "Sabby" "Larry" [2,] "Cathy" "Harry" Desired Element: Larry
In the above example, we have created a 2 by 2 matrix named matrix1 with 4 string type datas. Notice the use of index operator []
,
matrix1[1, 2]
Here, [1, 2]
specifies we are trying to access element present at 1st row, 2nd column i.e. "Larry"
.
Access Entire Row or Column
In R, we can also access the entire row or column based on the value passed inside []
.
[n, ]
- returns the entire element of the nth row.[ ,n]
- returns the entire element of the nth column.
For example,
matrix1 <- matrix(c("Sabby", "Cathy", "Larry", "Harry"), nrow = 2, ncol = 2)
print(matrix1)
# access entire element at 1st row
cat("\n1st Row:", matrix1[1, ])
# access entire element at 2nd column
cat("\n2nd Column:", matrix1[, 2])
Output
[,1] [,2] [1,] "Sabby" "Larry" [2,] "Cathy" "Harry" 1st Row: Sabby Larry 2nd Column: Larry Harry
Here,
matrix1[1, ]
- access entire elements at 1st row i.e.Sabby
andLarry
matrix1[ ,2]
- access entire elements at 2nd column i.e.Larry
andHarry
Access More Than One Row or Column
We can access more than one row or column in R using the c()
function.
[c(n1,n2), ]
- returns the entire element of n1 and n2 row.[ ,c(n1,n2)]
- returns the entire element of n1 and n2 column.
For example,
# create 2 by 3 matrix
matrix1 <- matrix(c(10, 20, 30, 40, 50, 60), nrow = 2, ncol = 3)
print(matrix1)
# access entire element of 1st and 3rd row
cat("\n1st and 2nd Row:", matrix1[c(1,3), ])
# access entire element of 2nd and 3rd column
cat("\n2nd and 3rd Column:", matrix1[ ,c(2,3)])
Output
[,1] [,2] [,3] [1,] 10 30 50 [2,] 20 40 60 1st and 3rd Row: 10 20 30 40 50 60 2nd and 3rd Column: 30 40 50 60
Here,
[c(1,3), ]
- returns the entire element of 1st and 3rd row.[ ,c(2,3)]
- returns the entire element of 2nd and 3rd column.
Modify Matrix Element in R
We use the vector index operator []
to modify the specified element. For example,
matrix1[1,2] = 140
Here, the element present at 1st row, 2nd column is changed to 140.
Let's see an example,
# create 2 by 2 matrix
matrix1 <- matrix(c(1, 2, 3, 4), nrow = 2, ncol = 2)
# print original matrix
print(matrix1)
# change value at 1st row, 2nd column to 5
matrix1[1,2] = 5
# print updated matrix
print(matrix1)
Output
[,1] [,2] [1,] 1 3 [2,] 2 4 [,1] [,2] [1,] 1 5 [2,] 2 4
Combine Two Matrices in R
In R, we use the cbind()
and the rbind()
function to combine two matrices together.
cbind()
- combines two matrices by columnsrbind()
- combines two matrices by rows
The number of rows and columns of two matrices we want to combine must be equal. For example,
# create two 2 by 2 matrices
even_numbers <- matrix(c(2, 4, 6, 8), nrow = 2, ncol = 2)
odd_numbers <- matrix(c(1, 3, 5, 7), nrow = 2, ncol = 2)
# combine two matrices by column
total1 <- cbind(even_numbers, odd_numbers)
print(total1)
# combine two matrices by row
total2 <- rbind(even_numbers, odd_numbers)
print(total2)
Output
[,1] [,2] [,3] [,4] [1,] 2 6 1 5 [2,] 4 8 3 7 [,1] [,2] [1,] 2 6 [2,] 4 8 [3,] 1 5 [4,] 3 7
Here, first we have used the cbind()
function to combine the two matrices: even_numbers and odd_numbers by column. And rbind()
to combine two matrices by row.
Check if Element Exists in R Matrix
In R, we use the %in%
operator to check if the specified element is present in the matrix or not and returns a boolean value.
TRUE
- if specified element is present in the matrixFALSE
- if specified element is not present in the matrix
For example,
matrix1 <- matrix(c("Sabby", "Cathy", "Larry", "Harry"), nrow = 2, ncol = 2)
"Larry" %in% matrix1 # TRUE
"Kinsley" %in% matrix1 # FALSE
Output
TRUE FALSE
Here,
"Larry"
is present in matrix1, so the method returnsTRUE
"Kinsley"
is not present in matrix1, so the method returnsFALSE