A vector is the basic data structure in R that stores data of similar types. For example,
Suppose we need to record the age of 5 employees. Instead of creating 5 separate variables, we can simply create a vector.
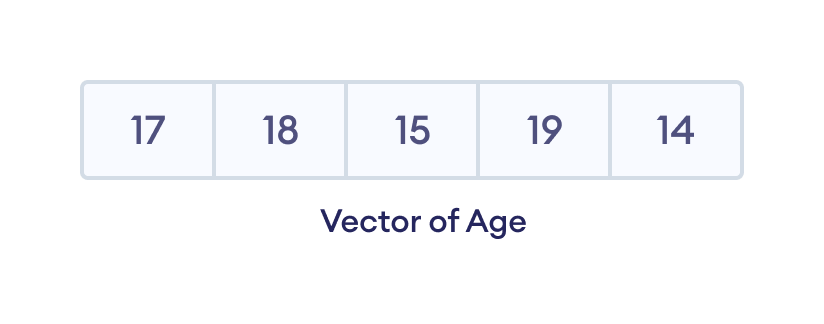
Create a Vector in R
In R, we use the c()
function to create a vector. For example,
# create vector of string types
employees <- c("Sabby", "Cathy", "Lucy")
print(employees)
# Output: [1] "Sabby" "Cathy" "Lucy"
In the above example, we have created a vector named employees with elements: Sabby
, Cathy
, and Lucy
.
Here, the c()
function creates a vector by combining three different elements of employees together.
Access Vector Elements in R
In R, each element in a vector is associated with a number. The number is known as a vector index.
We can access elements of a vector using the index number (1, 2, 3 …). For example,
# a vector of string type
languages <- c("Swift", "Java", "R")
# access first element of languages
print(languages[1]) # "Swift"
# access third element of languages
print(languages[3]). # "R"
In the above example, we have created a vector named languages. Each element of the vector is associated with an integer number.
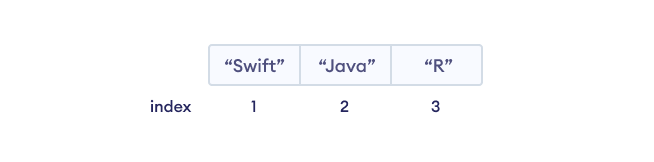
Here, we have used the vector index to access the vector elements
languages[1]
- access the first element"Swift"
languages[3]
- accesses the third element"R"
Note: In R, the vector index always starts with 1. Hence, the first element of a vector is present at index 1, second element at index 2 and so on.
Modify Vector Element
To change a vector element, we can simply reassign a new value to the specific index. For example,
dailyActivities <- c("Eat","Repeat")
cat("Initial Vector:", dailyActivities)
# change element at index 2
dailyActivities[2] <- "Sleep"
cat("\nUpdated Vector:", dailyActivities)
Output
Initial Vector: Eat Repeat Updated Vector: Eat Sleep
Here, we have changed the vector element at index 2 from "Repeat"
to "Sleep"
by simply assigning a new value.
Numeric Vector in R
Similar to strings, we use the c()
function to create a numeric vector. For example,
# a vector with number sequence from 1 to 5
numbers <- c(1, 2, 3, 4, 5)
print(numbers)
# Output: [1] 1 2 3 4 5
Here, we have used the C()
function to create a vector of numeric sequence called numbers.
However, there is an efficient way to create a numeric sequence. We can use the :
operator instead of C()
.
Create a Sequence of Number in R
In R, we use the :
operator to create a vector with numerical values in sequence. For example,
# a vector with number sequence from 1 to 5
numbers <- 1:5
print(numbers)
Output
[1] 1 2 3 4 5
Here, we have used the :
operator to create the vector named numbers with numerical values in sequence i.e. 1 to 5.
Repeat Vectors in R
In R, we use the rep()
function to repeat elements of vectors. For example,
# repeat sequence of vector 2 times
numbers <- rep(c(2,4,6), times = 2)
cat("Using times argument:", numbers)
Output
Using times argument: 2 4 6 2 4 6
In the above example, we have created a numeric vector with elements 2, 4, 6. Notice the code,
rep(numbers, times=2)
Here,
numbers
- vector whose elements to be repeatedtimes = 2
- repeat the vector two times
We can see that we have repeated the whole vector two times. However, we can also repeat each element of the vector. For this we use the each
parameter.
Let's see an example.
# repeat each element of vector 2 times
numbers <- rep(c(2,4,6), each = 2)
cat("\nUsing each argument:", numbers)
Output
Using each argument: 2 2 4 4 6 6
In the above example, we have created a numeric vector with elements 2, 4, 6. Notice the code,
rep(numbers, each = 2)
Here, each = 2
- repeats each element of vector two times
Loop Over a R Vector
We can also access all elements of the vector by using a for loop. For example,
In R, we can also loop through each element of the vector using the for loop. For example,
numbers <- c(1, 2, 3, 4, 5)
# iterate through each elements of numbers
for (number in numbers) {
print(number)
}
Output
[1] 1 [1] 2 [1] 3 [1] 4 [1] 5
Length of Vector in R
We can use the length()
function to find the number of elements present inside the vector. For example,
languages <- c("R", "Swift", "Python", "Java")
# find total elements in languages using length()
cat("Total Elements:", length(languages))
Output
Total Elements: 4
Here, we have used length()
to find the length of the languages vector.