Bitwise operators perform operations on integer data at the individual bit-level. These operations include testing, setting, or shifting the actual bits. For example,
a & b
a | b
In the example, &
and |
are bitwise operators.
Here's the list of various bitwise operators included in Swift
Operators | Name | Example |
---|---|---|
& |
Bitwise AND | a & b |
| |
Bitwise OR | a | b |
^ |
Bitwise XOR | a ^ b |
~ |
Bitwise NOT | ~ a |
<< |
Bitwise Shift Left | a << b |
>> |
Bitwise Shift Right | a >> b |
Bitwise AND Operator
The bitwise AND &
operator returns 1 if and only if both the operands are 1. Otherwise, it returns 0.
The bitwise AND operation on a
and b
can be represented in the table below:
a | b | a & b |
---|---|---|
0 | 0 | 0 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
Note: The table above is known as the "Truth Table" for the bitwise AND operator.
Let's take a look at the bitwise AND operation of two integers 12 and 25:
12 = 00001100 (In Binary)
25 = 00011001 (In Binary)
// Bitwise AND Operation of 12 and 25
00001100
& 00011001
_____________
00001000 = 8 (In Decimal)
Example 1: Bitwise AND Operator
var a = 12
var b = 25
var result = a & b
print (result) // 8
In the above example, we have declared two variables a
and b
. Here, notice the line,
var result = a & b
Here, we are performing bitwise AND operation between a
and b
.
Bitwise OR Operator
The bitwise OR |
operator returns 1 if at least one of the operands is 1. Otherwise, it returns 0.
The bitwise OR operation on a
and b
can be represented in the table below:
a | a | a | b |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 1 | 1 |
1 | 0 | 1 |
Let us look at the bitwise OR operation of two integers 12 and 25:
12 = 00001100 (In Binary)
25 = 00011001 (In Binary)
Bitwise OR Operation of 12 and 25
00001100
| 00011001
____________
00011101 = 29 (In decimal)
Example 2: Bitwise OR Operator
var a = 12
var b = 25
var result = a | b
print(result) // 29
Here, we are performing bitwise OR between 12 and 25.
Bitwise XOR Operator
The bitwise XOR ^
operator returns 1 if and only if one of the operands is 1. However, if both the operands are 0, or if both are 1, then the result is 0.
The bitwise XOR operation on a
and b
can be represented in the table below:
a | b | a ^ b |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
Let us look at the bitwise XOR operation of two integers 12 and 25:
12 = 00001100 (In Binary)
25 = 00011001 (In Binary)
Bitwise XOR Operation of 12 and 25
00001100
^ 00011001
____________
00010101 = 21 (In decimal)
Example 3: Bitwise XOR Operator
var a = 12
var b = 25
var result = a ^ b
print(result) // 21
Here, we are performing bitwise XOR between 12 and 25.
Bitwise NOT Operator
The bitwise NOT ~
operator inverts the bit( 0 becomes 1, 1 becomes 0).
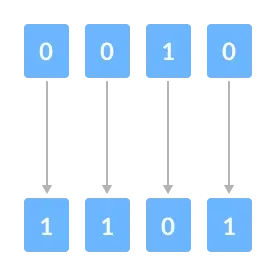
The bitwise NOT operation on a
can be represented in the table below:
It is important to note that the bitwise NOT of any integer N is equal to -(N + 1). For example,
Consider an integer 35. As per the rule, the bitwise NOT of 35 should be -(35 + 1) = -36. Now, let's see if we get the correct answer or not.
35 = 00100011 (In Binary)
// Using bitwise NOT operator
~ 00100011
________
11011100
In the above example, bitwise NOT of 00100011 is 11011100. Here, if we convert the result into decimal we get 220.
However, it is important to note that we cannot directly convert the result into decimal and get the desired output. This is because the binary result 11011100 is also equivalent to -36.
To understand this we first need to calculate the binary output of -36. We use 2's complement to calculate the binary of negative integers.
2's complement
The 2's complement of a number N gives -N. It is computed by inverting the bits(0 to 1 and 1 to 0) and then adding 1. For example,
36 = 00100100 (In Binary)
1's Complement = 11011011
2's Complement :
11011011
+ 1
________
11011100
Here, we can see the 2's complement of 36 (i.e. -36) is 11011100. This value is equivalent to the bitwise complement of 35 that we have calculated in the previous section.
Hence, we can say that the bitwise complement of 35 = -36.
Example 4: Bitwise NOT Operator
var b = 12
var result = ~b
print(result) // -13
In the above example, we have performed the bitwise NOT operation on 12.
The bitwise complement of 12 = - (12 + 1) = -13
i.e. ~12 = -13
This is exactly what we got in the output.
Left Shift Operator
The left shift operator shifts all bits towards the left by a specified number of bits. It is denoted by <<
.
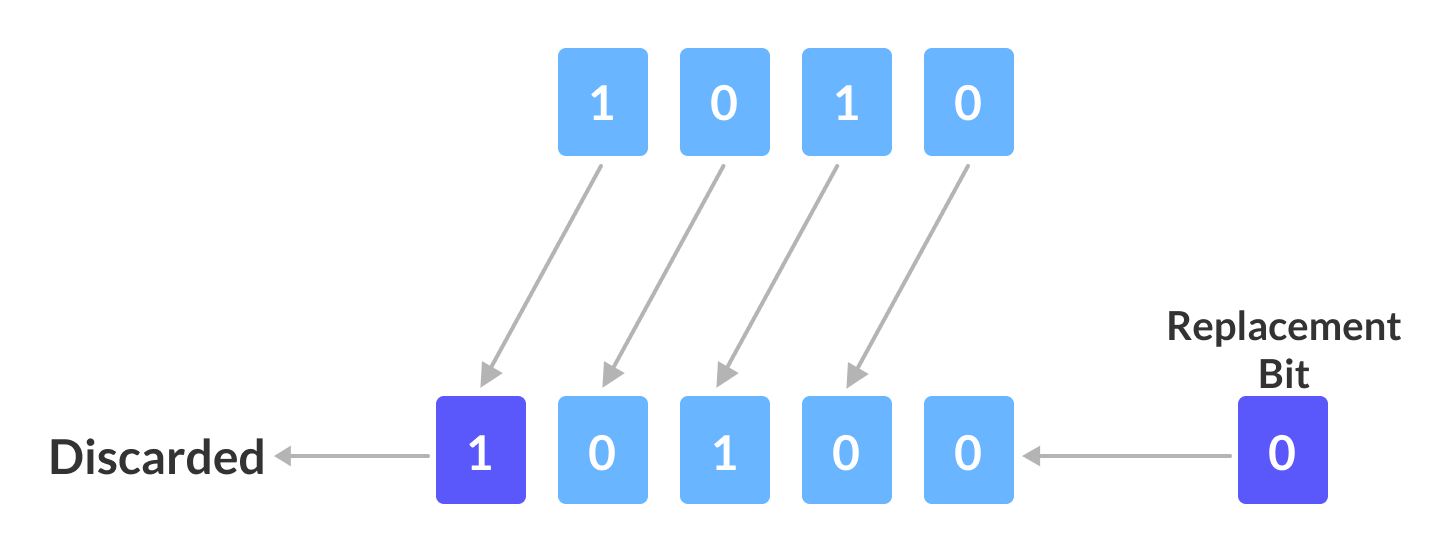
As we can see from the image above,
- We have a 4-digit number. When we perform a 1 bit left shift operation on it, each bit is shifted to the left by 1 bit.
- As a result, the left-most bit is discarded, while the right-most bit remains vacant. This vacancy is replaced by 0.
Example 5: Left Shift Operator
var a = 3
var result = a << 2
print(result) // 12
In the above example, we have created a variable a
with the value 3
. Notice the statement
var result = a << 2
Here, we are performing 2 bits left shift operation on a
.
Right Shift Operator
The right shift operator shifts all bits towards the right by a certain number of specified bits. It is denoted by >>
.
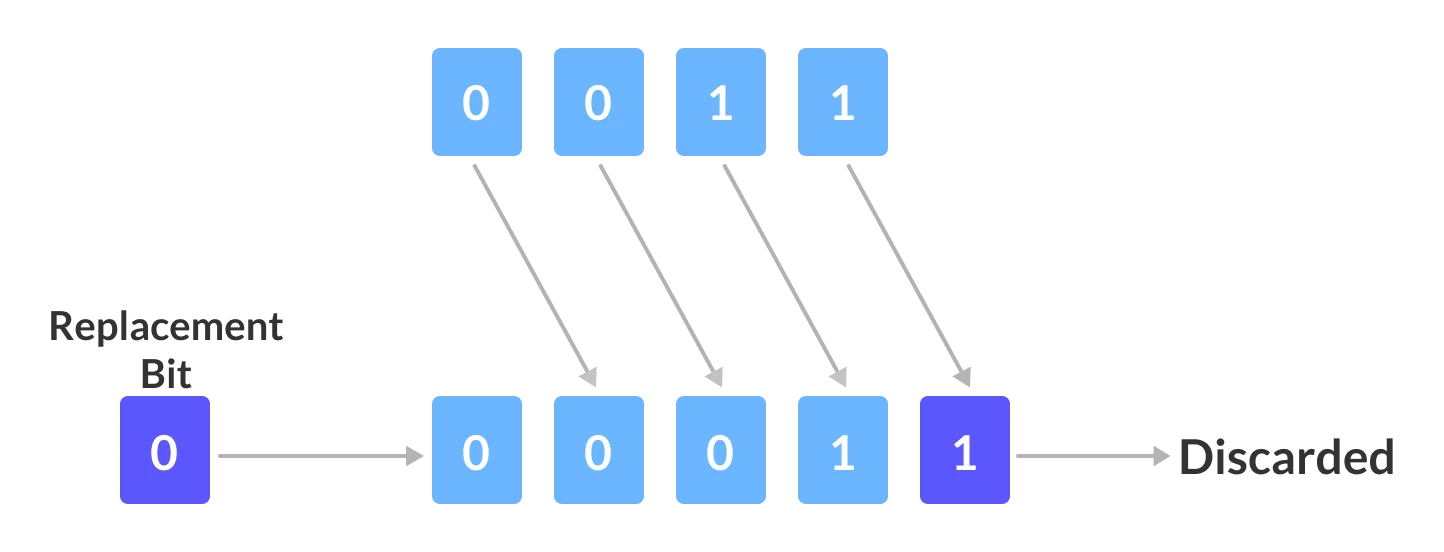
As we can see from the image above,
- We have a 4-digit number. When we perform a 1-bit right shift operation on it, each bit is shifted to the right by 1 bit.
- As a result, the right-most bit is discarded, while the left-most bit remains vacant. This vacancy is replaced by 0 for unsigned numbers.
- For signed numbers, the sign bit (0 for positive number, 1 for negative number) is used to fill the vacated bit positions.
Note: Signed integer represents both positive and negative integers while an unsigned integer only represents positive integers.
Example 5: Right Shift Operator
var a = 4
var result = a >> 2
print(result) // 1
a = -4
result = a >> 2
print(result) // -1
In the above example, we are performing 2 bits right shift operations on values 4 and -4.
As you can see the result is different for 4 and -4. This is because 4 is an unsigned integer so the vacancy is filled with 0 and -4 is a negative signed number so vacancy is filled with 1.