An array is a collection of similar types of data. For example,
Suppose we need to record the age of 5 students. Instead of creating 5 separate variables, we can simply create an array:
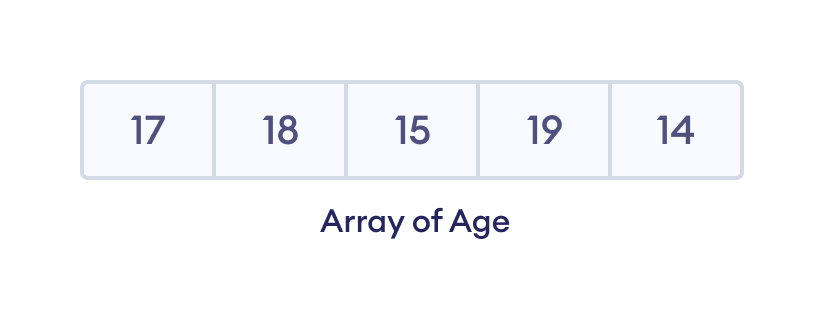
Create a Swift Array
Here's how we create an array in Swift.
// an array of integer type
var numbers : [Int] = [2, 4, 6, 8]
print("Array: \(numbers)")
Output
Array: [2, 4, 6, 8]
In the above example, we have created an array named numbers
. Here, [Int]
specifies that the array can only store integer data.
Swift is a type inference language that is, it can automatically identify the data type of an array based on its values. Hence, we can create arrays without specifying the data type. For example,
var numbers = [2, 4, 6, 8]
print("Array: \(numbers)") // [2, 4, 6, 8]
Create an Empty Array
In Swift, we can also create an empty array. For example,
var value = [Int]()
print(value)
Output
[ ]
In the above example, value
is an empty array that doesn't contain any element.
It is important to note that, while creating an empty array, we must specify the data type inside the square bracket []
followed by an initializer syntax ()
. Here, [Int]()
specifies that the empty array can only store integer data elements.
Note: In Swift, we can create arrays of any data type like Int
, String
, etc.
Access Array Elements
In Swift, each element in an array is associated with a number. The number is known as an array index.
We can access elements of an array using the index number (0, 1, 2 …). For example,
var languages = ["Swift", "Java", "C++"]
// access element at index 0
print(languages[0]) // Swift
// access element at index 2
print(languages[2]) // C++
In the above example, we have created an array named languages
.
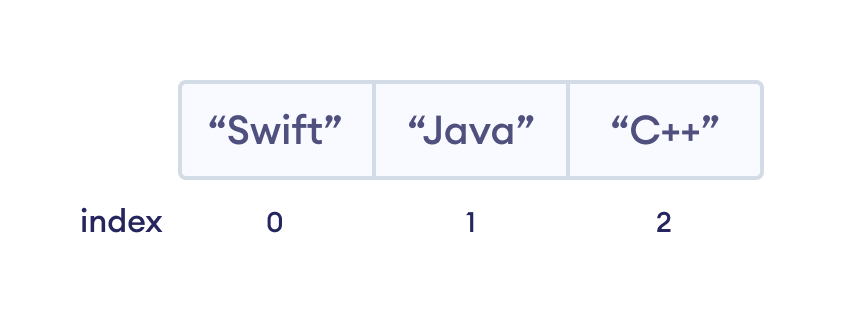
Here, we can see each array element is associated with the index number. And, we have used the index number to access the elements.
Note: The array index always starts with 0. Hence, the first element of an array is present at index 0, not 1.
Add Elements to an Array
Swift Array provides different methods to add elements to an array.
1. Using append()
The append()
method adds an element at the end of the array. For example,
var numbers = [21, 34, 54, 12]
print("Before Append: \(numbers)")
// using append method
numbers.append(32)
print("After Append: \(numbers)")
Output
Before Append: [21, 34, 54, 12] After Append: [21, 34, 54, 12, 32]
In the above example, we have created an array named numbers. Notice the line,
numbers.append(32)
Here, append()
adds 32 at the end of the array.
We can also use the append()
method to add all elements of one array to another. For example,
var primeNumbers = [2, 3, 5]
print("Array1: \(primeNumbers)")
var evenNumbers = [4, 6, 8]
print("Array2: \(evenNumbers)")
// join two arrays
primeNumbers.append(contentsOf: evenNumbers)
print("Array after append: \(primeNumbers)")
Output
Array1: [2, 3, 5] Array2: [4, 6, 8] Array after append: [2, 3, 5, 4, 6, 8]
In the above example, we have two arrays named primeNumbers and evenNumbers. Notice the statement,
primeNumbers.append(contentsOf: evenNumbers)
Here, we are adding all elements of evenNumbers to primeNumbers.
Note: We must use contentOf
with append()
if we want to add all elements from one array to another.
2. Using insert()
The insert()
method is used to add elements at the specified position of an array. For example,
var numbers = [21, 34, 54, 12]
print("Before Insert: \(numbers)")
numbers.insert(32, at: 1)
print("After insert: \(numbers)")
Output
Before insert: [21, 34, 54, 12] After insert: [21, 32, 34, 54, 12]
Here, numbers.insert(32, at:1)
adds 32 at the index 1.
Modify the Elements of an Array
We can use the array index to modify the array element. For example,
var dailyActivities = ["Eat","Repeat"]
print("Initial Array: \(dailyActivities)")
// change element at index 1
dailyActivities[1] = "Sleep"
print("Updated Array: \(dailyActivities)")
Output
Initial Array: ["Eat", "Repeat"] Updated Array: ["Eat", "Sleep"]
Here, initially the value at index 1 is Repeat
. We then changed the value to Sleep
using
dailyActivities[1] = "Sleep"
Remove an Element from an Array
We can use the remove()
method to remove the last element from an array. For example,
var languages = ["Swift","Java","Python"]
print("Initial Array: \(languages)")
// remove element at index 1
let removedValue = languages.remove(at: 1)
print("Updated Array: \(languages)")
print("Removed value: \(removedValue)")
Output
Initial Array: ["Swift", "Java", "Python"] Updated Array: ["Swift", "Python"] Removed value: Java
Similarly, we can also use
removeFirst()
- to remove the first elementremoveLast()
- to remove the last elementremoveAll()
- to remove all elements of an array
Other Array Methods
Method | Description |
---|---|
sort() |
sorts array elements |
shuffle() |
changes the order of array elements |
forEach() |
calls a function for each element |
contains() |
searches for the element in an array |
swapAt() |
exchanges the position of array elements |
reverse() |
reverses the order of array elements |
Looping Through Array
We can use the for loop to iterate over the elements of an array. For example,
// an array of fruits
let fruits = ["Apple", "Peach", "Mango"]
// for loop to iterate over array
for fruit in fruits {
print(fruit)
}
Output
Apple Peach Mango
Find Number of Array Elements
We can use the count
property to find the number of elements present in an array. For example,
let evenNumbers = [2,4,6,8]
print("Array: \(evenNumbers)")
// find number of elements
print("Total Elements: \(evenNumbers.count)")
Output
Array: [2, 4, 6, 8] Total Elements: 4
Check if an Array is Empty
The isEmpty
property is used to check if an array is empty or not. For example,
// array with elements
let numbers = [21, 33, 59, 17]
print("Numbers: \(numbers)")
// check if numbers is empty
var result = numbers.isEmpty
print("Is numbers empty? : \(result)")
// array without elements
let evenNumbers = [Int]()
print("Even Numbers: \(evenNumbers)")
// check if evenNumbers is empty
result = evenNumbers.isEmpty
print("Is evenNumbers empty? : \(result)")
Output
Numbers: [21, 33, 59, 17] Is numbers empty? : false Even Numbers: [] Is evenNumbers empty? : true
In the above example, we have used isEmpty
property to check if arrays numbers and evenNumbers are empty. Here, isEmpty
returns
true
- if the array is emptyfalse
- if the array is not empty
Array With Mixed Data Types
Till now, we have been using arrays that hold elements of a single data type.
However, in Swift, we can also create arrays that can hold elements of multiple data types. For example,
// array with String and integer data
var address: [Any] = ["Scranton", 570]
print(address)
Output
["Scranton", 570]
In the above example, we have created an array named address.
var address: [Any] = ["Scranton", 570]
Here, [Any]
specifies that address can hold elements of any data type. In this case, it stores both String
and Integer
data.