In Swift Inheritance, the subclass inherits the methods and properties of the superclass. This allows subclasses to directly access the superclass members.
Now, if the same method is defined in both the superclass and the subclass, then the method of the subclass class overrides the method of the superclass. This is known as overriding.
We use the override
keyword to declare method overriding. For example,
class Vehicle {
func displayInfo(){
...
}
}
class Car: Vehicle {
// override method
override func displayInfo() {
...
}
}
Here, the displayInfo()
method of the Car subclass overrides the same method of the Vehicle superclass.
Example: Swift Method Overriding
class Vehicle {
// method in the superclass
func displayInfo() {
print("Four Wheeler or Two Wheeler")
}
}
// Car inherits Vehicle
class Car: Vehicle {
// overriding the displayInfo() method
override func displayInfo() {
print("Four Wheeler")
}
}
// create an object of the subclass
var car1 = Car()
// call the displayInfo() method
car1.displayInfo()
Output
Four Wheeler
In the above example, we are overriding the displayInfo()
method of the superclass Vehicle inside the subclass Car.
// inside the Car class
override func displayInfo() {
print("Four Wheeler")
}
Here, we have used the override
keyword to specify the overridden method.
Now, when we call the displayInfo()
method using the object car1 of Car,
car1.displayInfo()
the method inside the subclass is called.
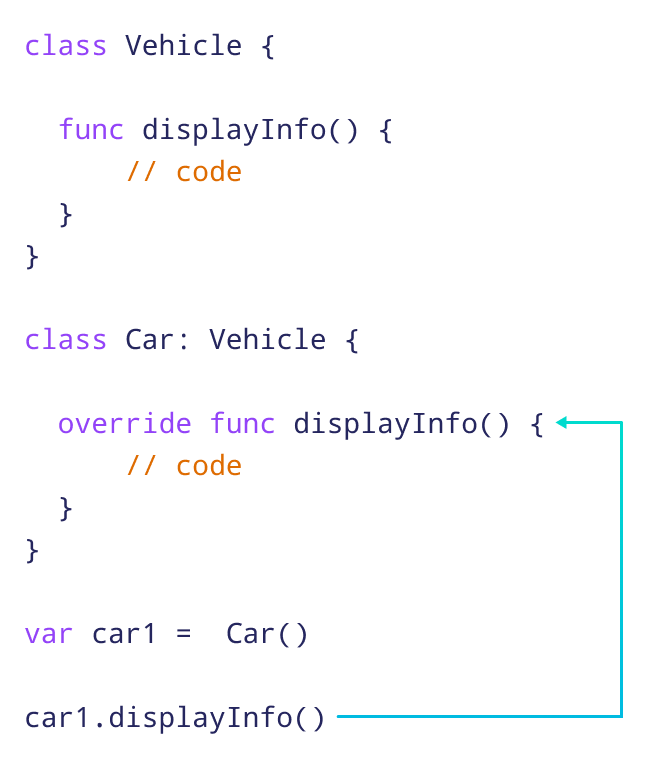
This is because the displayInfo()
method of the Car subclass overrides the same method of the Vehicle superclass.
Access Overridden Method in Swift
To access the method of the superclass from the subclass, we use the super
keyword. For example,
class Vehicle {
// method in the superclass
func displayInfo() {
print("Vehicle: Four Wheeler or Two Wheeler")
}
}
// Car inherits Vehicle
class Car: Vehicle {
// overriding the displayInfo() method
override func displayInfo() {
// access displayInfo() of superclass
super.displayInfo()
print("Car: Four Wheeler")
}
}
// create an object of the subclass
var car1 = Car()
// call the displayInfo() method
car1.displayInfo()
Output
Vehicle: Four Wheeler or Two Wheeler Car: Four Wheeler
In the above example, the displayInfo()
method of the Car subclass overrides the same method of the Vehicle superclass.
Inside the displayInfo()
of Car, we have used
// call method of superclass
super.displayInfo()
to call the displayInfo()
method of Vehicle
So, when we call the displayInfo()
method using the car1 object
// call the displayInfo() method
car1.displayInfo()
both the overridden and the superclass version of the displayInfo()
methods are executed.
Prevent Method Overriding
In Swift, we can prevent the method from overriding.
To make a method non-overridable, we use the final
keyword while declaring a method in the superclass. For example,
class Vehicle {
// prevent overriding
final func displayInfo() {
print("Four Wheeler or Two Wheeler")
}
}
// Car inherits Vehicle
class Car: Vehicle {
// attempt to override
override func displayInfo() {
print("Four Wheeler")
}
}
// create an object of the subclass
var car1 = Car()
// call the displayInfo() method
car1.displayInfo()
In the above example, we have marked the displayInfo()
method in the superclass as final
.
Once the method is declared as final
, we cannot override it. So when we try to override the final method,
override func displayInfo() {
print("Four Wheeler")
we get an error message: error: instance method overrides a 'final' instance method
.
Override Swift Properties
In Swift, we can override the computed properties. For example,
class University {
// computed property
var cost: Int {
return 5000
}
}
class Fee: University {
// override computed property
override var cost: Int {
return 10000
}
}
var amount = Fee()
// access fee property
print("New Fee:", amount.cost)
Output
New Fee: 10000
In the above example, we have created a computed property inside the superclass University. Notice the code inside the subclass Fee,
override var cost: Int {
return 10000
}
We are overriding the computed property cost. Now, when we access the cost property using the object amount of Fee,
// access fee property
amount.cost
the property inside the subclass is called.
Note: We cannot override the stored properties in Swift. For example,
class A {
// stored property
var num = 0
}
class B: A {
// overriding stored property
override var num = 2 // Error Code
}