In computer programming, loops are used to repeat a block of code.
For example, if we want to show a message 100 times, then we can use a loop. It's just a simple example; you can achieve much more with loops.
There are 3 types of loops in Swift:
Swift for-in Loop
In Swift, the for-in
loop is used to run a block of code for a certain number of times. It is used to iterate over any sequences such as an array, range, string, etc.
The syntax of the for-in
loop is:
for val in sequence{
// statements
}
Here, val
accesses each item of sequence
on each iteration. Loop continues until we reach the last item in the sequence
.
Flowchart of for-in Loop
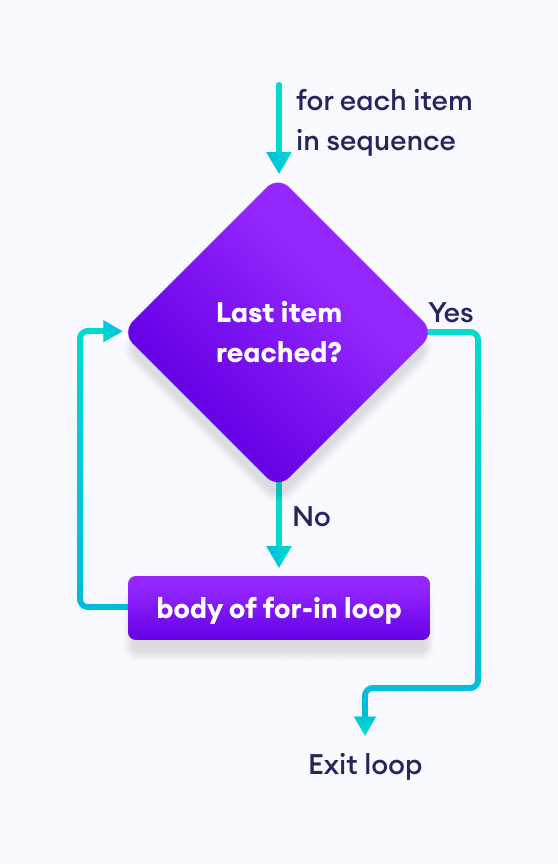
Example: Loop Over Array
// access items of an array
let languages = ["Swift", "Java", "Go", "JavaScript"]
for language in languages {
print(language)
}
Output
Swift Java Go JavaScript
In the above example, we have created an array called languages
.
Initially, the value of language
is set to the first element of the array,i.e. Swift
, so the print statement inside the loop is executed.
language
is updated with the next element of the array and the print statement is executed again. This way the loop runs until the last element of an array is accessed.
for Loop with where Clause
In Swift, we can also add a where
clause with for-in
loop. It is used to implement filters in the loop. That is, if the condition in where
clause returns true
, the loop is executed.
// remove Java from an array
let languages = ["Swift", "Java", "Go", "JavaScript"]
for language in languages where language != "Java"{
print(language)
}
Output
Swift Go JavaScript
In the above example, we have used a for-in
loop to access each elements of languages
. Notice the use of where
clause in the for-in
loop.
for language in languages where language != "Java"
Here, if the condition in where
clause returns true
, the code inside the loop is executed. Otherwise, it is not executed.
Iteration | language | condition: != "Java" | Output |
---|---|---|---|
1st | Swift | true | Swift is printed. |
2nd | Java | false | Java is not printed |
3rd | Go | true | Go is printed |
4th | JavaScript | true | JavaScript is printed |
for Loop With Range
A range is a series of values between two numeric intervals. For example,
var values = 1...3
Here, 1...3
defines a range containing values 1, 2, 3.
In Swift, we can use for
loop to iterate over a range. For example,
// iterate from i = 1 to i = 3
for i in 1...3 {
print(i)
}
Output
1 2 3
In the above example, we have used the for-in
loop to iterate over a range from 1 to 3.
The value of i
is set to 1
and it is updated to the next number of the range on each iteration. This process continues until 3
is reached.
Iteration | Condition | Action |
---|---|---|
1st | true |
1 is printed. i is increased to 2. |
2nd | true |
2 is printed. i is increased to 3. |
3rd | true |
3 is printed. i is increased to 4. |
4th | false |
The loop is terminated |
for Loop with Stride Function
If we want a loop to increment by some fixed value in each iteration, instead of range, we have to use stride(from:to:by) function. For example,
for i in stride(from: 1, to: 10, by: 2) {
print(i)
}
Output
1 3 5 7 9
In the above example, the loop iterates over the range from 1 to 10 with an interval of 2. However, 10 is not included in the sequence.
Here, the value of i
is set to 1
and it is updated by an interval of 2 on each iteration.