In Swift, two or more functions may have the same name if they differ in parameters (different number of parameters, different types of parameters, or both).
These functions are called overloaded functions and this feature is called function overloading. For example:
// same function name different arguments
func test() { ... }
func test(value: Int) -> Int{ ... }
func test(value: String) -> String{ ... }
func test(value1: Int, value2: Double) -> Double{ ... }
Here, the test()
function is overloaded. These functions have the same name but accept different arguments.
Note: The return types of the above functions are not the same. It is because function overloading is not associated with return types. Overloaded functions may have the same or different return types, but they must differ in parameters.
Example1: Overloading with Different Parameter Types
// function with Int type parameter
func displayValue(value: Int) {
print("Integer value:", value)
}
// function with String type parameter
func displayValue(value: String) {
print("String value:", value)
}
// function call with String type parameter
displayValue(value: "Swift")
// function call with Int type parameter
displayValue(value: 2)
Output
String value: Swift Integer value: 2
In the above example, we have overloaded the displayValue()
function:
- one function has
Int
type parameter - another has
String
type parameter
Based on the type of the argument passed during the function call, the corresponding function is called.
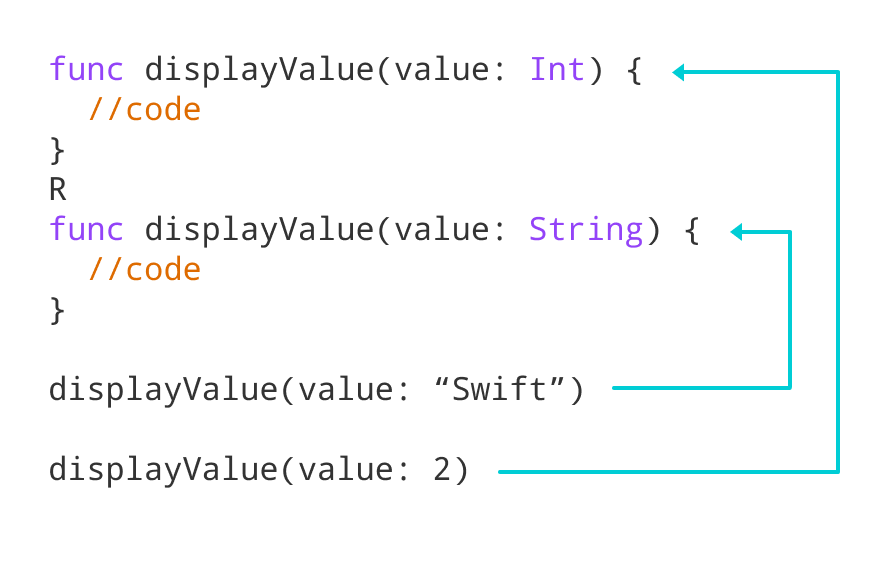
Example2: Overloading with Different Number of Parameters
// function with two parameters
func display(number1: Int, number2: Int) {
print("1st Integer: \(number1) and 2nd Integer: \(number2)")
}
// function with a single parameter
func display(number: Int) {
print("Integer number: \(number)")
}
// function call with single parameter
display(number: 5)
// function call with two parameters
display(number1: 6, number2: 8)
Output
Integer number: 5 1st Integer: 6 and 2nd Integer: 8
Here, we have overloaded the display()
function with different numbers of parameters.
Based on the number of arguments passed during the function call, the corresponding function is called.
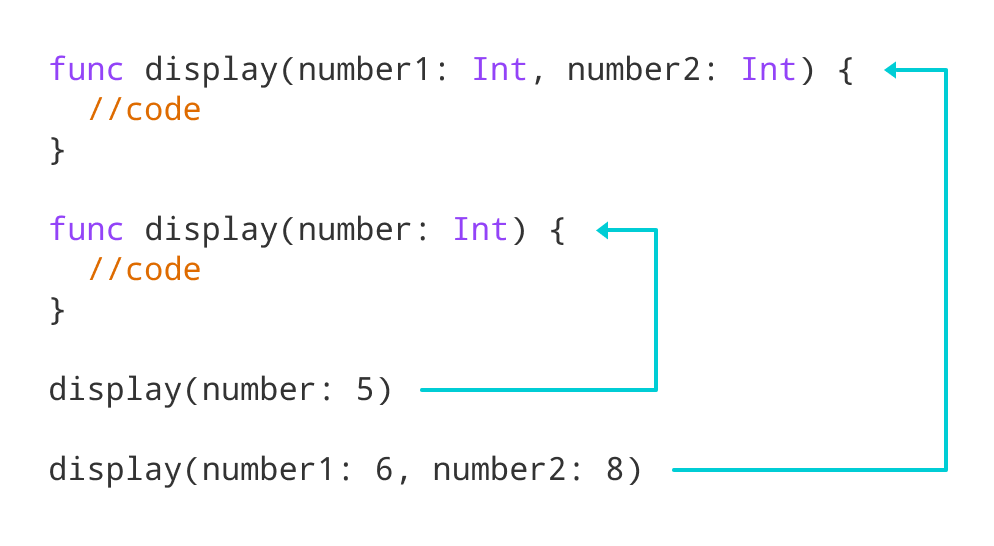
Example 3: Function overloading with Argument Label
func display(person1 age:Int) {
print("Person1 Age:", age)
}
func display(person2 age:Int) {
print("Person2 Age:", age)
}
display(person1: 25)
display(person2: 38)
Output
Person1 Age: 25 Person2 Age: 38
In the above example, two functions with the same name display()
have the same number and the same type of parameters. However, we are still able to overload the function.
It is because, in Swift, we can also overload the function by using argument labels.
Here, two functions have different argument labels. And, based on the argument label used during the function call, the corresponding function is called.