In SQL, the UPDATE
statement is used to modify existing records in a database table.
Example
--update a single value in the given row
UPDATE Customers
SET age = 21
WHERE customer_id = 1;
Here, the SQL command updates the age column to 21 where the customer_id equals 1.
SQL UPDATE TABLE Syntax
UPDATE table_name
SET column1 = value1, column2 = value2, ...
[WHERE condition];
Here,
- table_name is the name of the table to be modified
- column1, column2, ... are the names of the columns to be modified
- value1, value2, ... are the values to be set to the respective columns
- [WHERE condition] is an optional clause specifying which rows should be updated
Update a Single Value in a Row
In SQL, we can update a single value by using the UPDATE
command with a WHERE
clause. For example,
-- update a single value in the given row
UPDATE Customers
SET first_name = 'Johnny'
WHERE customer_id = 1;
Here, the SQL command changes the value of the first_name column to Johnny if customer_id is equal to 1.
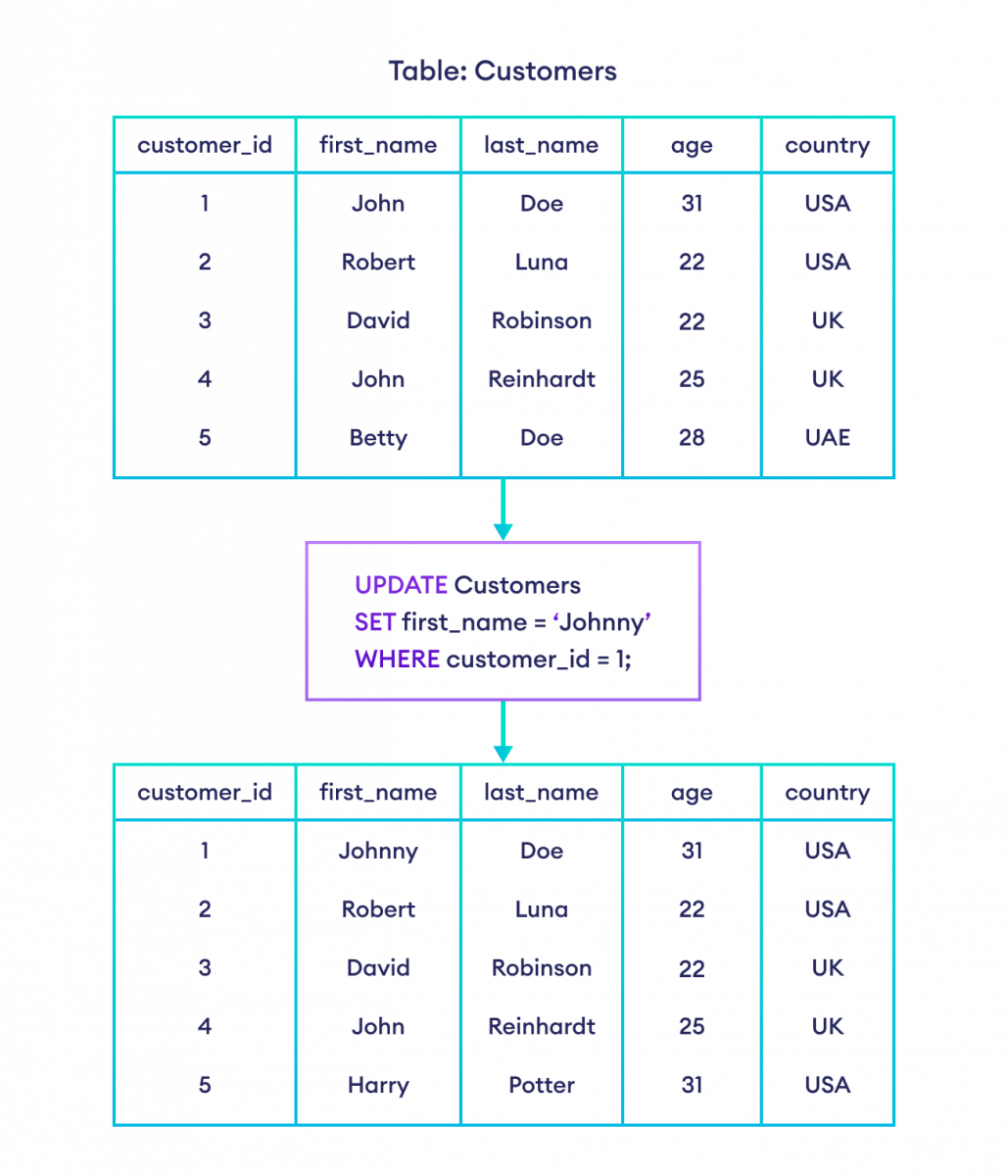
Update Multiple Values in a Row
We can also update multiple values in a single row at once. For example,
-- update multiple values in the given row
UPDATE Customers
SET first_name = 'Johnny', last_name = 'Depp'
WHERE customer_id = 1;
Here, the SQL command changes the value of the first_name column to Johnny and last_name to Depp if customer_id is equal to 1.
Update Multiple Rows
We use the UPDATE
statement to update multiple rows at once. For example,
-- update multiple rows satisfying the condition
UPDATE Customers
SET country = 'NP'
WHERE age = 22;
Here, the SQL command changes the value of the country
column to NP if age is 22.
If there is more than one row where age equals to 22, all the matching rows will be modified.
Update all Rows
We can update all the rows in a table at once by omitting the WHERE
clause. For example,
-- update all rows
UPDATE Customers
SET country = 'NP';
Here, the SQL command changes the value of the country column to NP for all rows.
Note: We should be cautious while using the UPDATE
statement. If we omit the WHERE
clause, all the rows will be changed, and this change is irreversible.
Also Read: