HTML (HyperText Markup Language) is a markup language used to structure and organize the content on a web page. It uses various tags to define the different elements on a page, such as headings, paragraphs, and links.
HTML Hierarchy
HTML elements are hierarchical, which means that they can be nested inside each other to create a tree-like structure of the content on the web page.
This hierarchical structure is called the DOM (Document Object Model), and it is used by the web browser to render the web page. For example,
<!DOCTYPE html>
<html>
<head>
<title>My web page</title>
</head>
<body>
<h1>Hello, world!</h1>
<p>This is my first web page.</p>
<p>It contains a
<strong>main heading</strong> and <em> paragraph </em>.
</p>
</body>
</html>
Browser Output
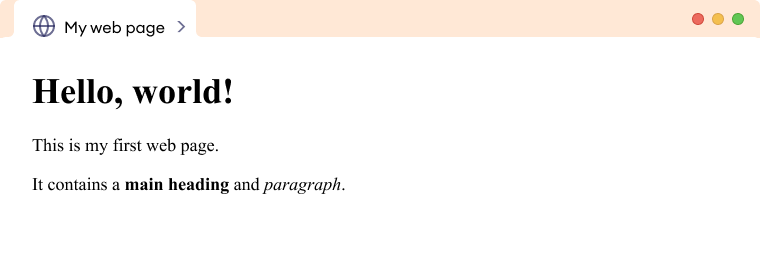
In this example, the html
element is the root element of the hierarchy and contains two child elements: head
and body
. The head
element, in turn, contains a child element called the title
, and the body
element contains child elements: h1
and p
.
Let's see the meaning of the various elements used in the above example.
<html>
: the root element of the DOM, and it contains all of the other elements in the code<head>
: contains metadata about the web page, such as the title and any linked CSS or JavaScript files<title>
: contains the title of the web page, which will be displayed in the web browser's title bar or tab<body>
: contains the main content of the web page, which will be displayed in the web browser's window<p>
: contains the paragraphs of text on the web page<strong>
,<em>
: child elements of the<p>
elements, they are used to mark text as important and emphasized respectively
Note: Only the elements inside the <body>
tag renders in the web browser.
What are HTML elements?
HTML elements consist of several parts, including the opening and closing tags, the content, and the attributes. Here is an explanation of each of these parts:
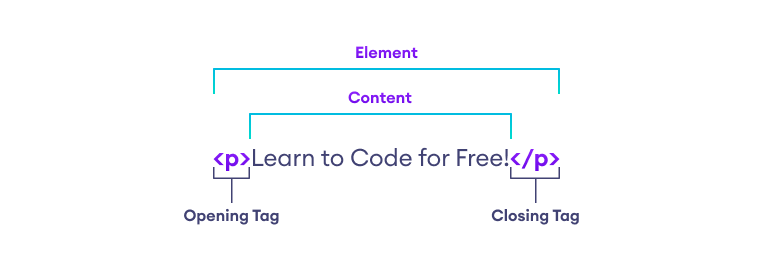
Here,
- The opening tag: This consists of the element name, wrapped in angle brackets. It indicates the start of the element and the point at which the element's effects begin.
- The closing tag: This is the same as the opening tag, but with a forward slash before the element name. It indicates the end of the element and the point at which the element's effects stop.
- The content: This is the content of the element, which can be text, other elements, or a combination of both.
- The element: The opening tag, the closing tag, and the content together make up the element.
What are HTML Attributes?
HTML elements can have attributes, which provide additional information about the element. They are specified in the opening tag of the element and take the form of name-value pairs. Let's see an example:
<a href="http://example.com"> Example </a>
The href
is an attribute. It provides the link information about the <a>
tag. In the above example,
href
- the name of attributehttps://www.programiz.com
- the value of attribute
Note: HTML attributes are mostly optional.
HTML Syntax
We need to follow a strict syntax guidelines to write valid HTML code. This includes the use of tags, elements, and attributes, as well as the correct use of indentation and white space. Here are some key points about HTML syntax:
1. HTML tags consist of the element name, wrapped in angle brackets. For example, <h1>
, <p>
, <img>
are some HTML tags.
2. HTML elements are created by enclosing the content of the element inside the opening and closing tags of the element. For example,
<span>Some text.</span>
is an HTML element.
3. HTML attributes are used to provide additional information about HTML elements and are specified in the opening tag of the element. For example,
<a target="www.google.com">Click Here</a>
Here, target
is an attribute.
4. HTML code should be well-formed and properly indented, with each element on its own line and each level of hierarchy indented by one level. This makes the code easier to read and understand, and can help to avoid errors. For example,
<html>
<head>
<title>My First HTML Page</title>
</head>
<body>
<h1>My First HTML Page</h1>
<p> Hello World!</p>
</body>
</html>