HTML <canvas>
is used to create graphics in HTML. We create the graphics inside the <canvas>
using JavaScript. For example,
<canvas id="circle-canvas" height="200" width="200" style="border: 1px solid;"></canvas>
<script>
let canvas = document.getElementById("circle-canvas");
let ctx = canvas.getContext("2d");
ctx.beginPath();
ctx.arc(100, 100, 80, 0, 2 * Math.PI, false);
ctx.fillStyle = 'blue';
ctx.fill();
</script>
Browser Output
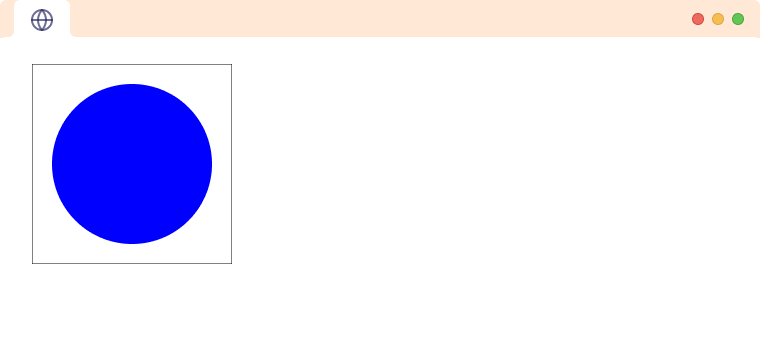
Here, we are using JavaScript along with the Canvas API to create a circle inside the canvas. This code creates a simple drawing using the canvas element in an HTML page.
The canvas has an id circle-canvas and dimensions of 200x200 pixels. This creates a surface on which we can draw using JavaScript.
We have used the getContext()
method of the canvas element and stored it in the ctx variable. It allows us to access the drawing context and draw on the canvas.
In the above example,
beginPath()
- creates a new path, any subsequent path commands will start from this pointarc()
- draws a circle where,
the circle is centered at (100, 100)
the circle has a radius of 80 pixels
the circle is drawn to cover the entire circumference in a counter-clockwise direction, by starting at 0 radians and ending at 2*Math.PI radiansfillStyle
- sets the fill color of the circle to bluefill()
- fills the circle with the blue color