An HTML class
is an attribute that can be added to an HTML element to give it a specific class name. For example,
<h2 class="logo">Programiz</h2>
<style>
.logo {
color: blue;
}
</style>
Browser Output
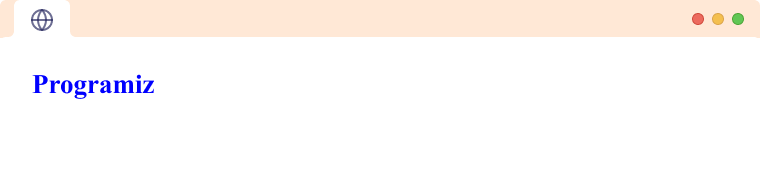
The class
attribute allows CSS and javascript to access the HTML code. In the above example, element <h2>
with class name logo is styled with .logo selector.
Note: We use .
before classname in CSS.
Multiple Class in HTML
An element can have more than one class. For example,
<h2 class="animal dog">Lily</h2>
<style>
.animal {
color: red;
}
.dog {
border: 1px solid black
}
</style>
Browser Output
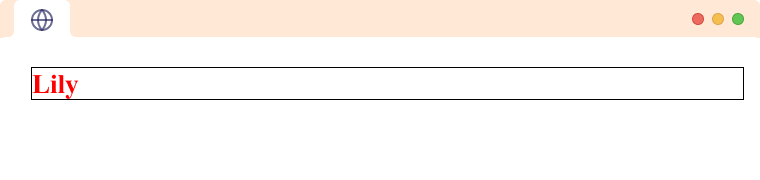
In the above example, <h2>
element has two classname animal and dog. We can add more than one class to an HTML element and separate it with a space.
Same class name on HTML elements
We can also provide the same class name on multiple HTML elements. It makes it easier to apply the same style to multiple elements. Let's us see an example,
<h2 class="animal">Dog</h2>
<h2 class="animal">Monkey</h2>
<style>
.animal {
color: blue;
}
</style>
Browser output
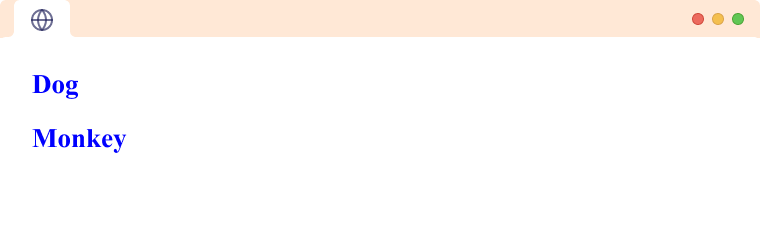
Here, we've used same class name animal on two <h2>
elements.
Class with Javascript in HTML
The class
attribute allows javascript to access the element via class selectors or getElementByClassName
API. For example,
<h2 class="fruit">APPLE</h2>
<script>
let element = document.getElementsByClassName("fruit")[0]
element.innerHTML = "ORANGE"
</script>
Browser output
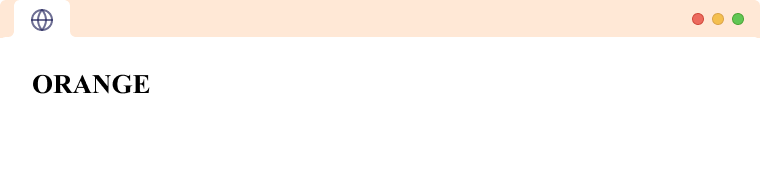
In the above example, we have used document.getElementsByClassName("fruits")
to access the HTML element where the fruit
is the name of the class in <h2>
element. Then, the innerHTML
property changes the content (the text inside the <h2>
tag) from APPLE to ORANGE.
Things to Note
- Class names are case-sensitive. Therefore,
animal
andAnimal
are two different class names. - You cannot use a space in a class name. If you use a space in a class name, it will be treated as two separate class names. For example, if you have the following CSS:
.my class {
font-size: 14px;
color: blue;
}
This will not apply styles to elements with the class my class. Instead, it will apply styles to elements with the class my and the class class (which are treated as two separate class names because of the space between them).
To avoid this issue, you should use a different character (such as a hyphen or underscore) instead of a space in your class names. For example, change the name my class to my-class.
Id vs Class
An HTML id
and a class
are both attributes that can be added to an HTML element to give it an identifier. The main difference between an id
and a class
is that an id
is unique within an HTML document, while a class can be used multiple times on a page.
Moreover, we can only use one id
in an HTML element while we can use multiple classes in an HTML element.