HTML elements can be broadly categorized into one of two categories:
- Inline Elements:
<span>
,<a>
,<strong>
,<img>
etc. - Block Elements:
<p>
,<div>
,<h1>
,<figure>
etc.
HTML Inline Elements
Inline elements are displayed on the same line. They do not start on a new line and take up only as much width as their contents require. An example of an inline element is the <span>
tag.
<p>This is how <span style="border: 1px solid black">span</span> works. </p>
Browser Output
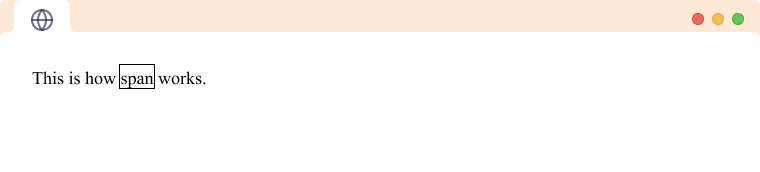
If we have multiple span tags, they get placed on the same line. For example,
<p> The following spans will be inline; <span style="border: 1px solid black">Span 1</span> and <span style="border: 1px solid black">Span 2</span>.</p>
Browser Output
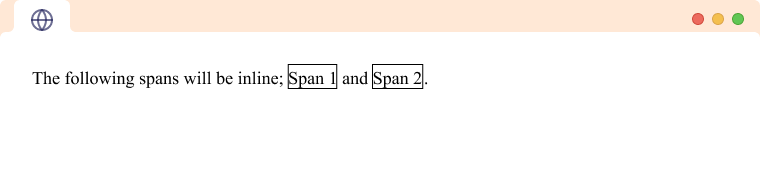
Some examples of inline elements are:
- HTML
<a>
tag - HTML
<input>
tag - HTML
<span>
tag
Most HTML formatting tags are also inline. For example:
- HTML
<b>
tag - HTML
<em>
tag - HTML
<strong>
tag, etc
HTML Block Elements
Block elements take up the whole horizontal space available in its container. They start on a new line and take up as much height as their contents require.
An example of a block element is the HTML Paragraph Tag.
<p style="border: 1px solid black">This is how block elements works. </p>
Browser Output
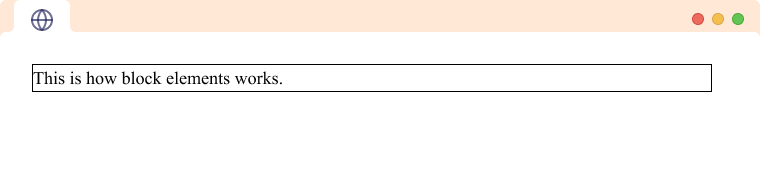
If we have multiple block elements, they will each take a separate line. For example,
<p style="border: 1px solid black">Paragraphs are</p> <p style="border: 1px solid black">Block Elements.</p>
Browser Output
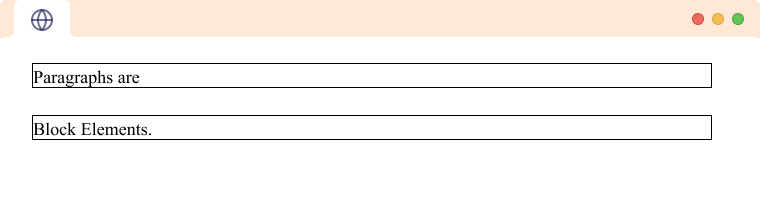
Some frequently used Block elements are:
- HTML
<div>
tag - HTML Headings
<h1>
-<h6>
- HTML
<p>
tag, etc
Css With Html Inline And Block Elements
CSS properties height
and width
have no effect on inline elements.
<p> The following span is an <span style="border: 1px solid black">Inline Element.</span></p>
<style>
span {
height: 200px; /* No Effect on Element */
width: 200px; /* No Effect on Element */
}
</style>
Browser Output
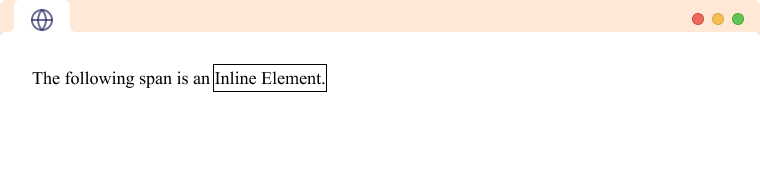
Inline elements also do not have top and bottom margins, they only respect left and right margin.
<div><span>1</span><span>2</span></div>
<div><span>3</span><span>4</span></div>
<style>
span {
margin-top: 10px;
margin-bottom: 10px;
margin-left: 10px;
margin-right: 10px;
border: 1px solid black;
}
</style>
Browser Output
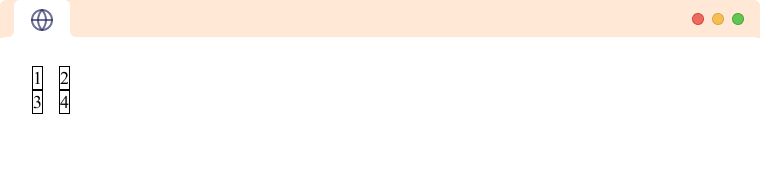
Inline elements respect padding in all directions.
<p><span>This is a span</span></p>
<style>
span {
padding: 10px;
border: 1px solid black;
}
</style>
Browser Output
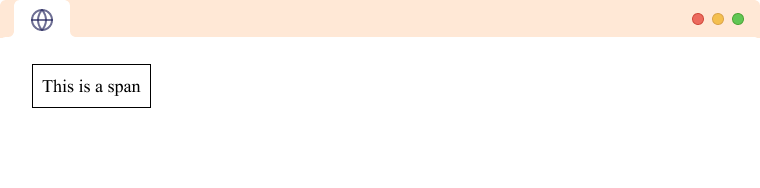
Block elements respect height
and width
properties. We can change both height and width of block elements using CSS.
<p style="border: 1px solid black;">This paragraph is a block element.</p>
<style>
p {
height: 200px; /* This works */
width: 200px; /* This works */
}
</style>
Browser Output
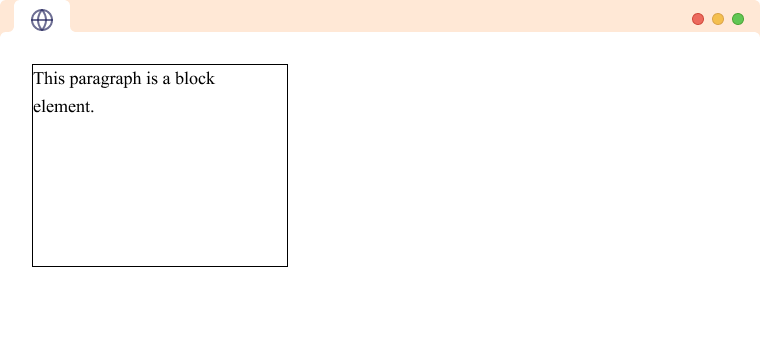
Block elements take up the whole width of the parent container even if we set their width to a smaller size. Hence we cannot have a block element with another element in the same line without CSS. For example,
<p style="width: 20px; border: 1px solid black;background-color: lightblue">1</p><p style="width: 20px; border: 1px solid black; background-color: lightblue">2</p>
Browser Output
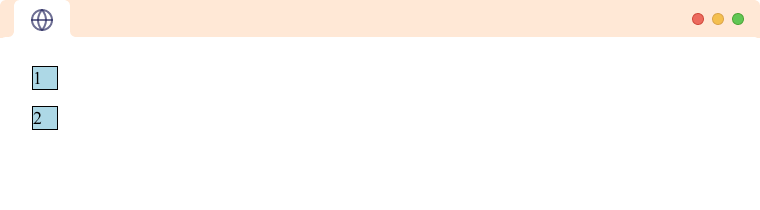
Block elements also respect top and bottom margins and padding directions. For example,
<div>Block</div>
<div>Block</div>
<style>
div {
border: 1px solid black;
padding: 10px;
margin: 10px;
}
</style>
Browser Output
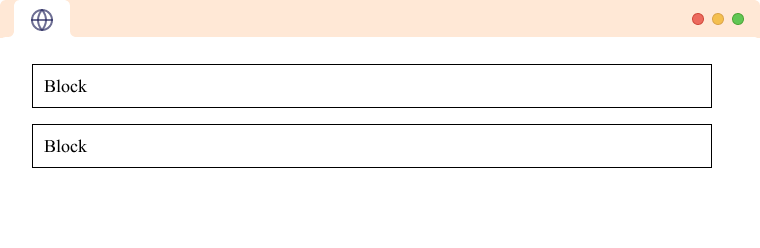