CSS is used for styling the look and formatting of a document written in HTML. There are three ways to add CSS in HTML
- Inline CSS: Styles added directly to the HTML element.
- Internal CSS: Styles defined at the
head
section of the document. - External CSS: Styles defined in a separate file.
Inline Style
Inline style is the approach of adding CSS rules directly to the HTML element using the style
attribute. For example,
<pstyle="color: red;">Hello, Good Morning</p>
Here,
style
- defines the CSS for the element<p>
color: red
- changes the text of the<p>
element to the color red
The style
attribute can be added to any element but the styles will only be applied to that particular element. For example,
<html lang="en">
<head>
<title>Browser</title>
</head>
<body>
<h1>This is h1</h1>
<p>This paragraph doesn't have CSS.</p>
<p style="color:red">This paragraph is styled with inline CSS.</p>
</body>
</html>
Browser Output
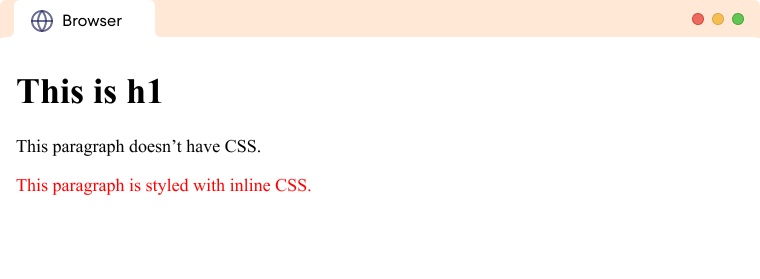
Internal CSS
Internal CSS applies CSS styles to a specific HTML document. Internal CSS is defined inside an HTML document using <style>
attribute within the head
tag of an HTML. For example,
<head>
<style>
p {
color: red;
}
</style>
</head>
Here,
<style>
- defines CSSp
- selectsp
tag and applies CSS to itcolor: red;
- changes the text color of the content of<p>
elements to red
Now, let's look at a complete example of how we use internal CSS in an HTML.
<html lang="en">
<head>
<style>
p {
color: red;
}
</style>
<title>Browser</title>
</head>
<body>
<h1>Internal CSS Example</h1>
<p>This is Paragraph 1</P>
<p>This is paragraph 2</p>
<div>This is a content inside a div</div>
</body>
</html>
Browser Output
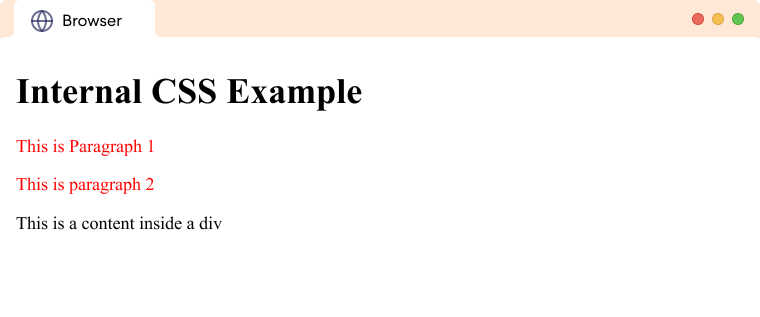
Note: The styles defined in an internal stylesheet will only affect the elements on the current HTML page and will not be available on other HTML pages.
external-css External CSS
External CSS is an approach to applying CSS styles to HTML pages by defining the CSS in a separate file.
Let's see an example:
p {
color: blue;
}
Here, we have CSS in a separate file named style.css. The external CSS file should have a .css extension.
The external CSS file can be linked to the HTML document by using the link
element in the HTML. For example,
<head>
<link href="style.css" rel="stylesheet">
</head>
We use the <link>
tag to link the style.css file to an HTML document. In the above code,
href="style.css"
- URL or file path to the external CSS file.rel="stylesheet"
- indicates the linked document is a CSS file
Example: External CSS
Let's see a complete example of using external CSS in an HTML document.
style.css
p {
color: blue;
}
Now, let's connect it with html file
<html lang="en">
<head>
<link href="style.css" rel="stylesheet">
<title>Browser</title>
</head>
<body>
<p>This is a sample text.</p>
</body>
</html>
Browser Output:
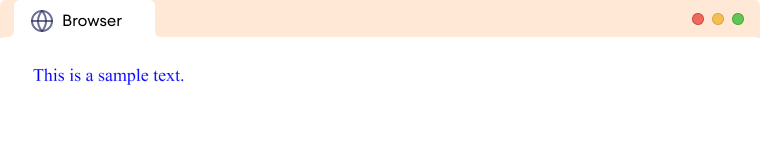
Using Multiple Stylesheet
We can link multiple CSS file to an HTML file. Suppose we have the following two CSS files.
style.css
p {
color: red;
}
div {
color: yellow;
}
main.css
body {
background: lightgreen;
}
Now, let's link these two CSS files to our HTML document.
<html lang="en">
<head>
<link href="main.css" rel="stylesheet">
<link href="style.css" rel="stylesheet">
<title>Browser</title>
</head>
<body>
<h1>Multiple Stylesheet Example</h1>
<p>This is Paragraph 1</P>
<p>This is paragraph 2</p>
<div>This is a content inside a div</div>
</body>
</html>
Browser Output
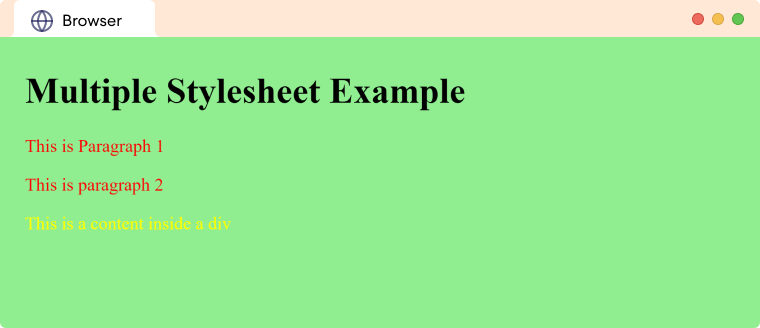
Here, we have linked main.css and style.css to our HTML file. Adding multiple CSS files helps us organize our CSS files into manageable parts.
Inline Style Override Internal Style
If an internal CSS and inline CSS are both applied to a tag, the styling from the inline tag is applied. Let's see an example.
<html lang="en">
<head>
<style>
h1 {
color: blue;
}
p {
background: red;
}
</style>
<title>Browser</title>
</head>
<body>
<h1 style="color: green">Priority Example</h1>
<p>This is Paragraph 1</P>
<p style="background: orange">This is paragraph 2</p>
</body>
</html>
Browser Output
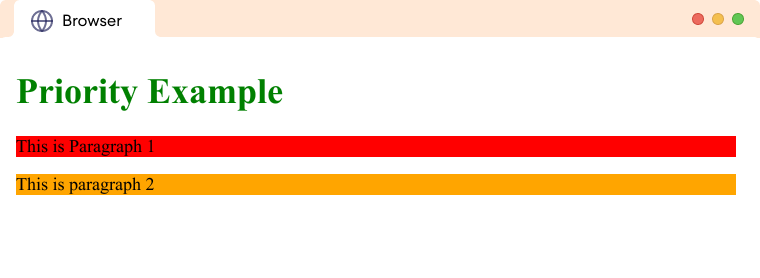
As you can see the styling from inline CSS is applied to elements.