The CSS margin
property adds space around an element. It creates an empty area outside the border of an element. For example,
h1 {
border: 4px solid black;
margin: 50px;
}
Browser Output
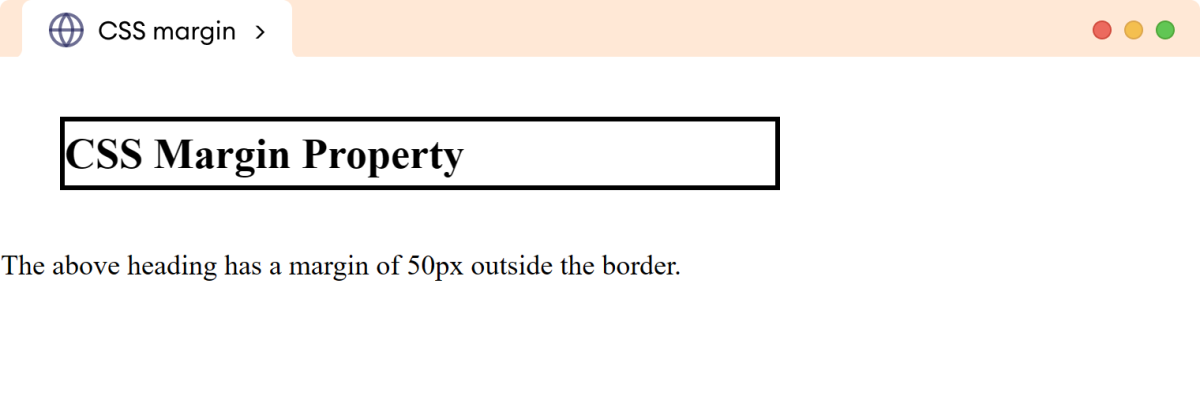
Here, the margin
property adds a 50px
space around the border of the h1
element.
CSS margin Syntax
The syntax of the margin
property is as follows,
margin: auto | length | percentage | inherit;
Here,
auto
: the browser calculates the margin automaticallylength
: defines the margin in length units such aspx
,pt
,em
, etcpercentage
: defines the margin in percentage (%)inherit
: inherits the value from the parent element
The default value of the margin
property is 0.
Note: The margin
property accepts the negative value, reducing the margin space in the specified direction.
Example 1: CSS margin Property
Let's see an example of the margin
property,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS margin</title>
</head>
<body>
<p class="margin">This paragraph has a margin of 60px.</p>
<p>This is a normal paragraph.</p>
</body>
</html>
p {
border: 4px solid black;
}
p.margin {
margin: 60px;
}
Browser Output
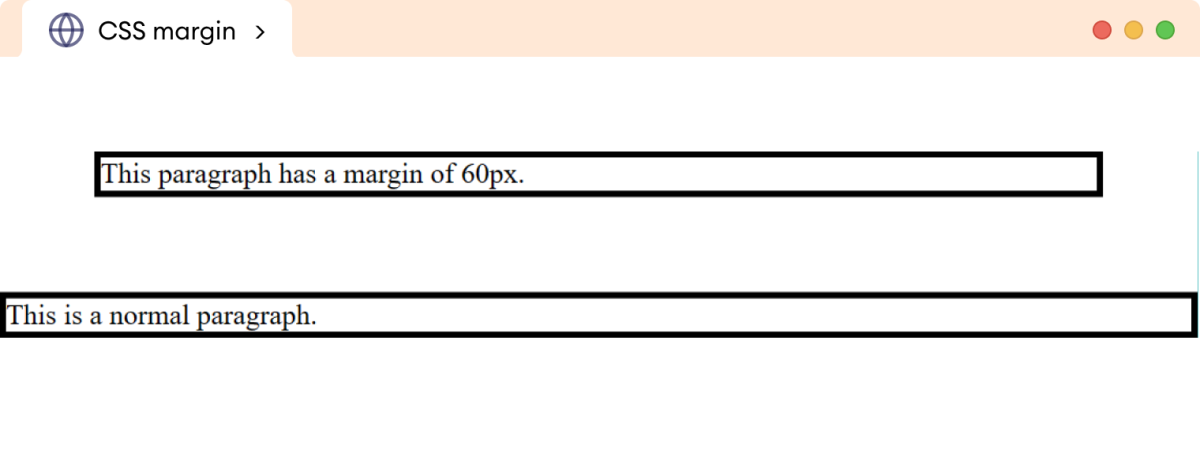
Example 2: CSS margin Property
The percentage value is relative to the size of the parent element. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS margin</title>
</head>
<body>
<p>This paragraph has a margin of 5%.</p>
<div>
<p>This paragraph has a margin of 5%.</p>
</div>
</body>
</html>
p {
margin: 5%;
border: 4px solid black;
}
div {
width: 400px;
height: 100px;
border: 2px solid black;
}
Browser Output
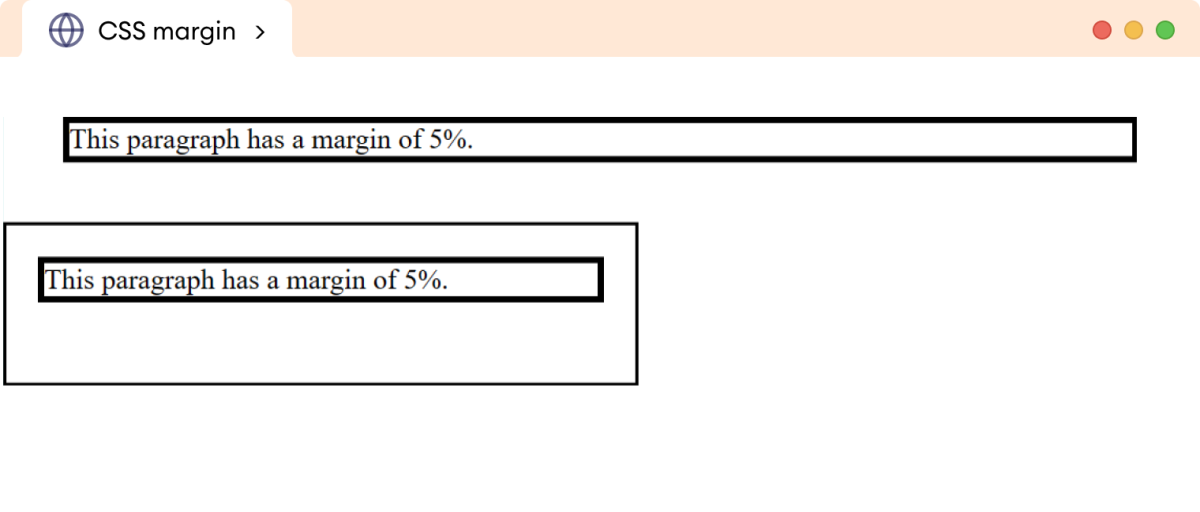
In the above example, the margin
space is different for both paragraphs, even having the same margin
of 5%
. This is because the percentage
value is relative to the size of the parent element.
The margin
of the first paragraph is relative to the size of the body
element, while the margin
of the second paragraph is relative to the size of the div
element.
Hence, the same margin
produces a different space for both paragraphs.
CSS margin Constituent Properties
The margin
property constitutes the following CSS properties to specify the margin for individual sides of the element.
margin-top
: adds a margin to the top sidemargin-right
: adds a margin to the right sidemargin-bottom
: adds a margin to the bottom sidemargin-left
: adds a margin to the left side
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS margin</title>
</head>
<body>
<p>
This paragraph has a margin of 30px at the top, 80px at the right,
25px at the bottom, and 60px at the left.
</p>
</body>
</html>
p {
border: 4px solid black;
margin-top: 30px;
margin-right: 80px;
margin-bottom: 25px;
margin-left: 40px;
}
Browser Output
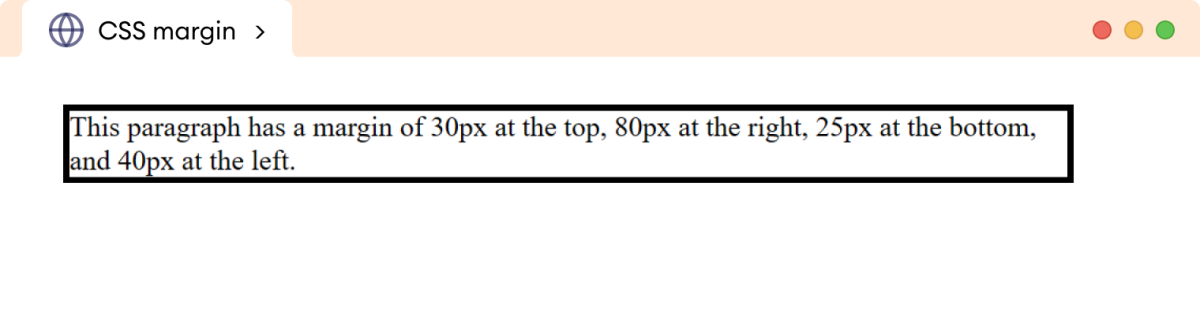
CSS margin as shorthand Property
The margin
property can be used as shorthand for specifying the margin of one to four sides of an element. For example,
margin: 10px; /* applies to all sides */
margin: 20px 40px; /* applies 20px to top & bottom, and 40px to left & right */
margin: 20px 40px 60px; /* applies 20px to top, 40px to left & right, and 60px to bottom */
margin: 10px 20px 30px 40px; /* applies to top, right, bottom, and left respectively */
Let's look a complete example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS margin</title>
</head>
<body>
<h2>margin: 30px 60px 80px 20px</h2>
<p>
The margin in the above heading follows order as top (30px), right
(60px), bottom (80px), and left (20px).
</p>
</body>
</html>
h2 {
/* applies top, right, bottom, and left respectively */
margin: 40px 60px 80px 30px;
background-color: orange;
}
Browser Output
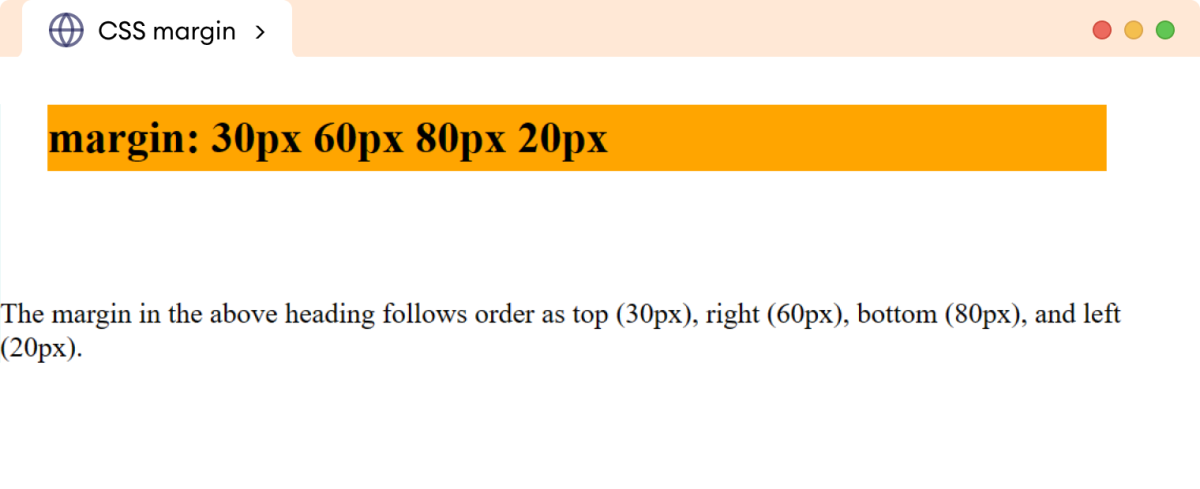
Learn more about CSS Margin
The adjacent vertical margins of block-level elements collapse to form a single margin.
Here, adjacent vertical margins refer to the margins between two adjacent block-level elements (such as <div>
or <p>
tags) that are positioned vertically, one above the other.
Suppose we have two block-level elements, A
, followed by B
beneath it, where A
has a margin of 40px
and B
has a margin of 20px
. Then the vertical margin between A
and B
is collapsed to form a single margin of 40px
.
The size of the collapsed margin is equal to the largest of the margins between them.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS margin</title>
</head>
<body>
<p class="first">This paragraph has a margin of 40px for all sides.</p>
<p class="second">This paragraph has a margin of 20px for all sides.</p>
</body>
</html>
p.first {
margin: 40px;
}
p.second {
margin: 20px;
}
p {
border: 4px solid black;
}
Browser Output
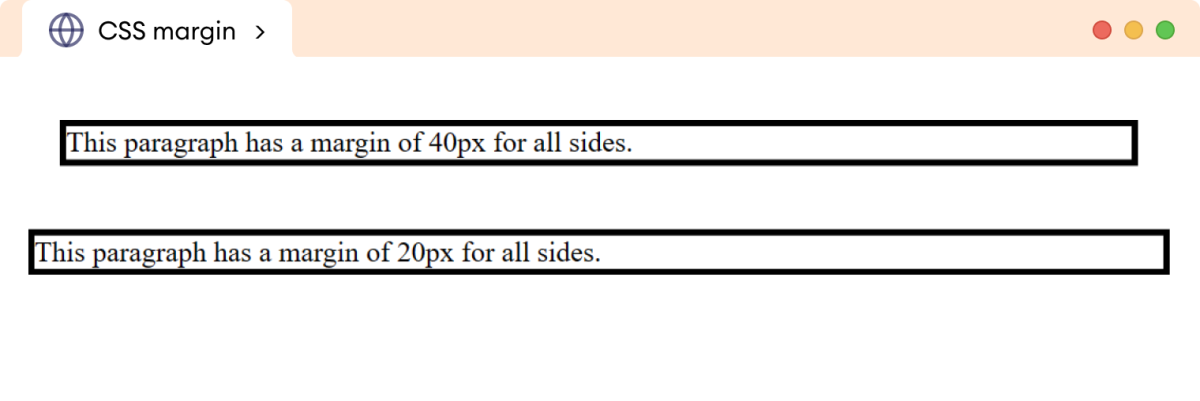
In the above example, two margins of 40px
and 20px
are applied between the two paragraphs. Both vertical adjacent margins collapse, resulting 40px
margin between them.
Note: The margin collapse occurs only for the top and bottom margins, not for the left and right margins.
The inline elements don't have any effect on the top and bottom margins. This is because inline elements follow the natural flow of the text, and applying top and bottom margins disrupts this flow and causes layout inconsistencies.
For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS margin</title>
</head>
<body>
<p>
This <span>span</span> element does not have any effect on the top
and bottom margins.
</p>
</body>
</html>
span {
/* adds 30px margin */
margin: 30px;
border: 2px solid black;
background-color: greenyellow;
}
p {
border: 4px solid black;
}
Browser Output
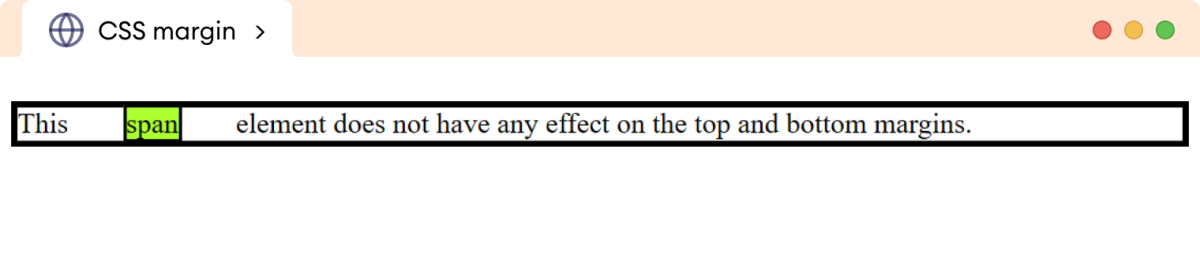
In the above example, only the left and right margins are applied, ignoring the top and bottom margins.
We can add margin to inline elements by changing the display
property to inline-block
or block
. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS margin</title>
</head>
<body>
<p>
This <span>span</span> element have top and bottom margins.
</p>
</body>
</html>
span {
margin: 30px;
display: inline-block;
border: 2px solid black;
background-color: greenyellow;
}
p {
border: 4px solid black;
}
Browser Output
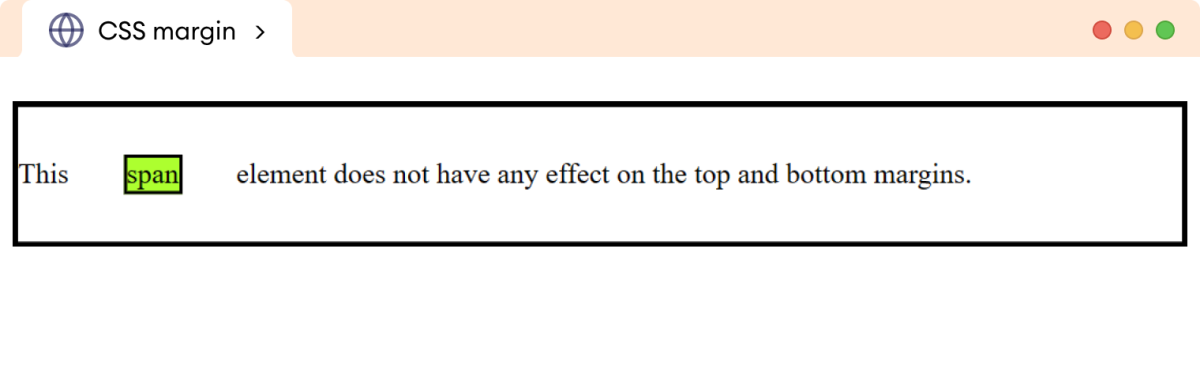
Here, the margin
property is applied to all four sides of the inline element.