CSS 2D transform property allows the rotation, translation, scale, and skew of elements in a two-dimensional plane. For example,
div {
transform: rotate(20deg);
}
Browser Output
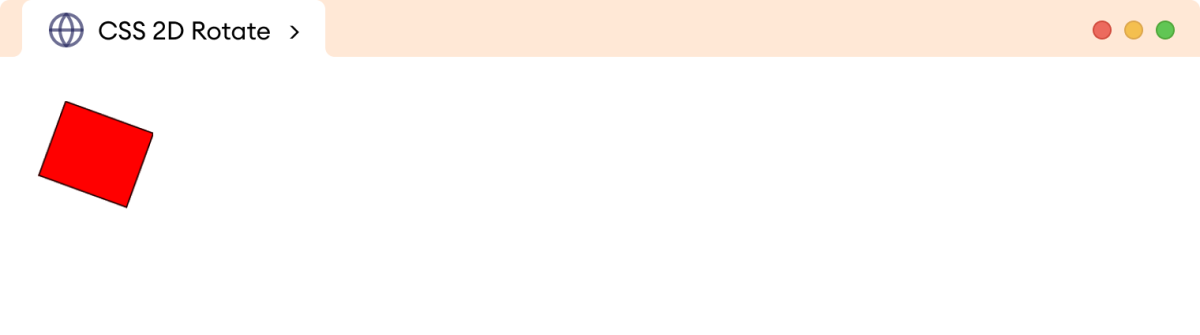
Here, the rotate()
function of the transform
property rotates the div
element with 20
degrees in the clockwise direction.
CSS 2D Transforms Methods
The CSS 2D transform
property allows us to use the following transformation methods:
translate()
: moves an element both horizontally and verticallyrotate()
: rotates an element in either clockwise or anticlockwise directionscale()
: increases or decreases the size of an elementskew()
: distorts an element by tilting along x and/or y axesmatrix()
: combines multiple transform methods into a single method
We will look at each of them briefly.
translate() Method
The translate()
method moves an element along the x-axis and/or y-axis.
The syntax of translate()
method is:
transform: translate(x-value,y-value)
Here, x-value
and y-value
represent the horizontal and vertical distance that the element moves from the original position.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 2D Translate</title>
</head>
<body>
<p>Original</p>
<div></div>
<p>transform: translate(60px, 20px)</p>
<div class="translate"></div>
</body>
</html>
/* styles both divs */
div {
width: 60px;
height: 50px;
background-color: red;
border: 1px solid black;
}
/* translates the div 60px horizontally and 20px vertically */
div.translate {
transform: translate(60px, 20px);
}
Browser Output
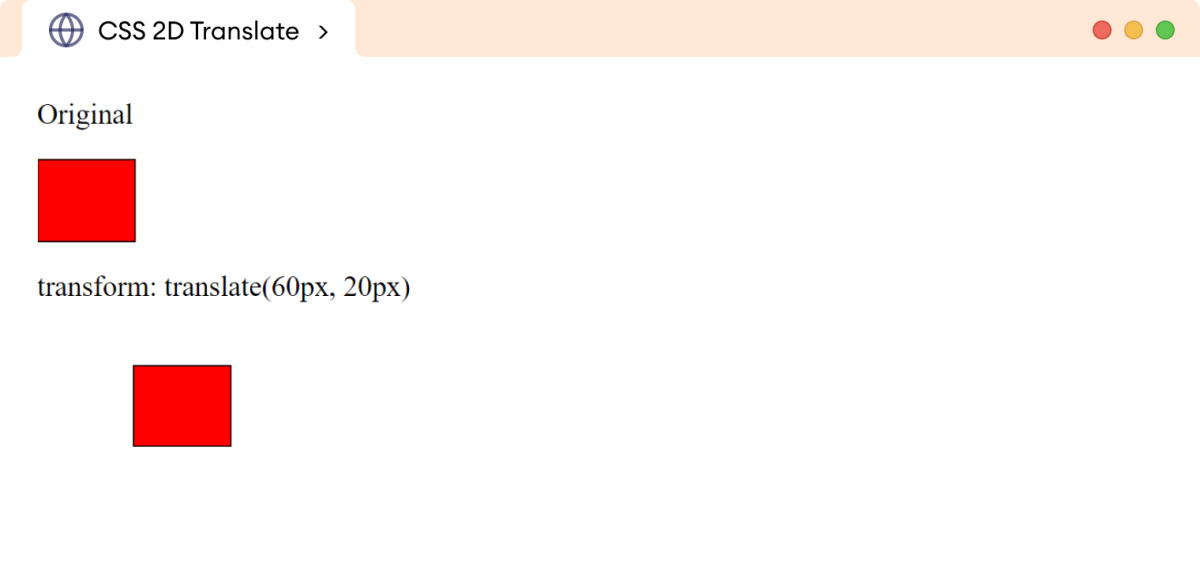
In the above example,
transform: translate(60px, 20px)
moves the second div
element by 60
pixels horizontally and 20
pixels vertically from the initial position.
Note: The negative value moves the element in the opposite direction.
The translateX() and translateY() Methods
The translateX()
method moves the element along the x-axis. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 2D TranslateX</title>
</head>
<body>
<p>Original</p>
<div></div>
<p>transform: translateX(60px)</p>
<div class="translate"></div>
</body>
</html>
/* styles both divs */
div {
width: 60px;
height: 50px;
background-color: red;
border: 1px solid black;
}
/* translates the div element 60px horizontally */
div.translate {
transform: translateX(60px);
}
Browser Output
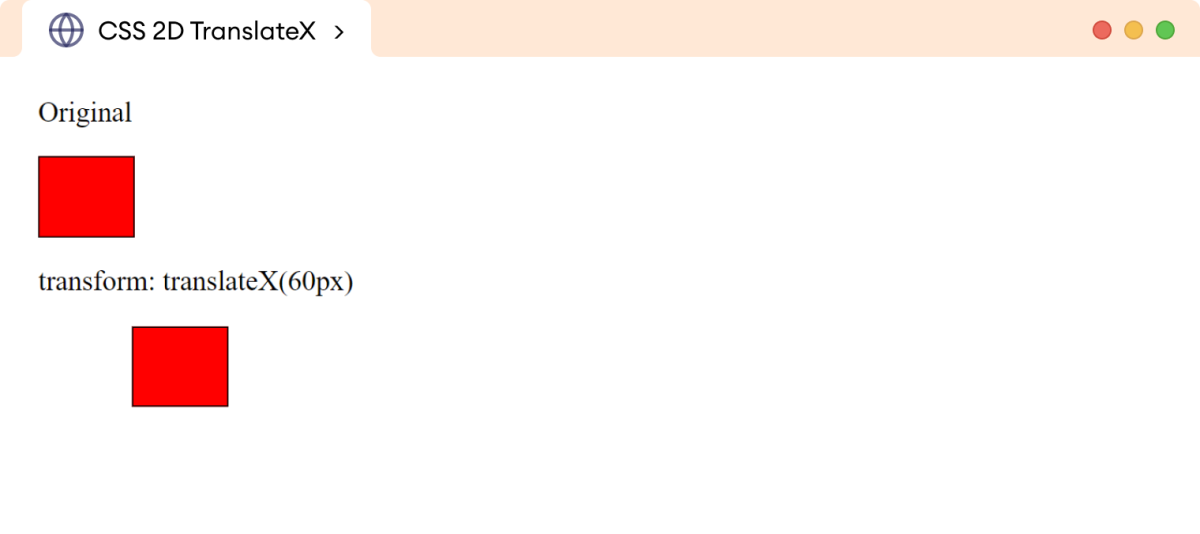
In the above example,
transform: translateX(60px)
moves the second div
element by 60
pixels to the right horizontally from the initial position.
The translateY()
method moves the element along the y-axis. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 2D TranslateY</title>
</head>
<body>
<p>Original</p>
<div></div>
<p>transform: translateY(40px)</p>
<div class="translate"></div>
</body>
</html
/* styles both divs */
div {
width: 60px;
height: 50px;
background-color: red;
border: 1px solid black;
}
/* translates the div element 40px vertically */
div.translate {
transform: translateY(40px);
}
Browser Output
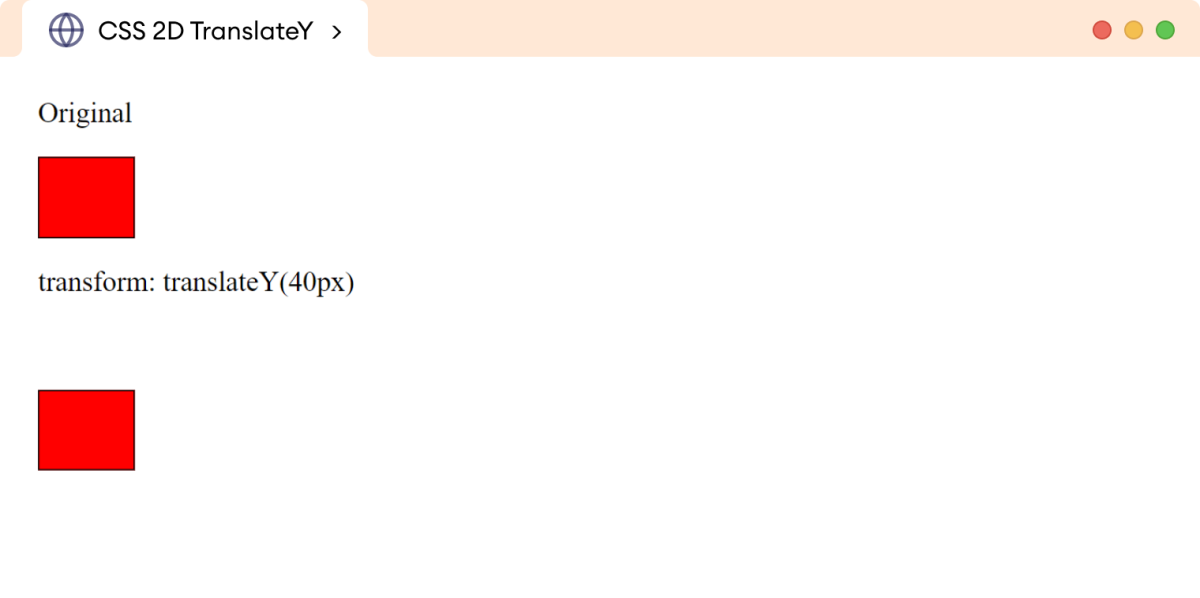
In the above example,
transform: translateY(40px)
moves the second div
element by 40
pixels to the bottom in the vertical direction from the initial position.
rotate() Method
The rotate()
method rotates an element in either a clockwise or anti-clockwise direction by a specified degree value.
The syntax of rotate()
method is:
transform: rotate(degree)
The degree value should be suffixed by the deg
keyword.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 2D Rotate</title>
</head>
<body>
<p>Original</p>
<div></div>
<p>transform: rotate(30deg)</p>
<div class="rotate"></div>
</body>
</html>
/* styles both divs */
div {
width: 60px;
height: 50px;
background-color: red;
border: 1px solid black;
}
/* rotates the div element by 30 degrees */
div.rotate {
transform: rotate(30deg);
}
Browser Output
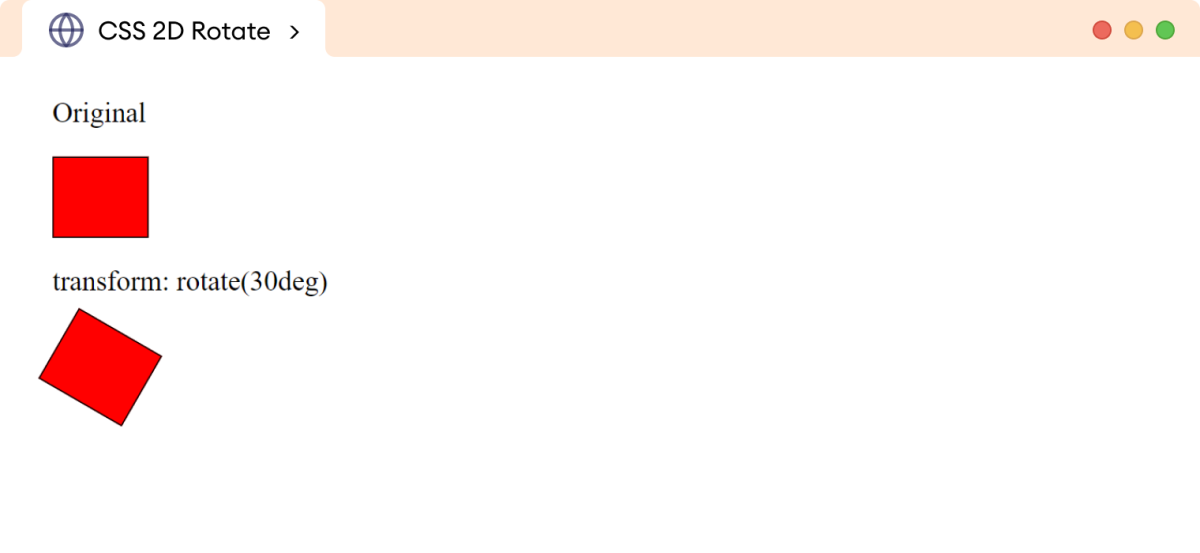
In the above example,
transform: rotate(30deg)
rotates the second div
element by 30
degrees in the clockwise direction.
Note:The negative degree value rotates the element in the anti-clockwise direction.
The rotateX() and rotateY() Methods
The rotateX()
method rotates the element to either a clockwise or an anti-clockwise direction along the x-axis. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 2D RotateX</title>
</head>
<body>
<p>Original</p>
<div>Hello</div>
<p>transform: rotateX(180deg)</p>
<div class="rotate">Hello</div>
</body>
</html>
/* styles both divs */
div {
width: 60px;
height: 50px;
background-color: red;
border: 1px solid black;
}
/* rotates the div element by 180 degrees along x-axis */
div.rotate {
transform: rotateX(180deg);
}
Browser Output
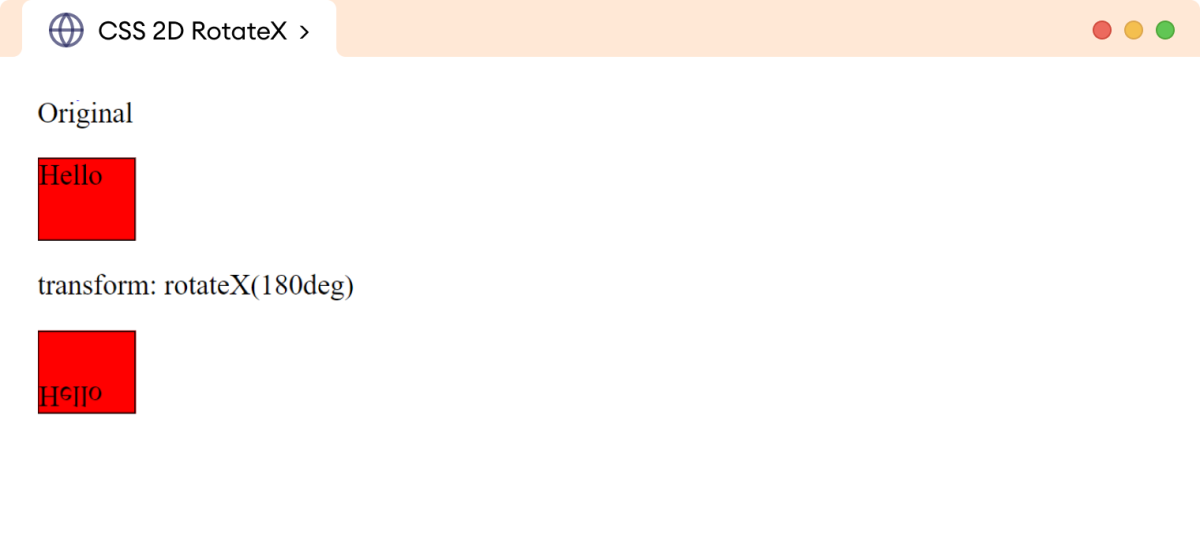
In the above example,
transform: rotateX(180deg)
rotates the second div
element by 180
degrees in a clockwise direction along the x-axis.
The text Hello
inside the first div
appears upside down after rotation.
The rotateY()
method rotates the element to either a clockwise or anti-clockwise direction along the y-axis. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 2D RotateY</title>
</head>
<body>
<p>Original</p>
<div>Hello</div>
<p>transform: rotateY(180deg)</p>
<div class="rotate">Hello</div>
</body>
</html>
/* styles both divs */
div {
width: 60px;
height: 50px;
background-color: red;
border: 1px solid black;
font-weight: bold;
text-align: center;
}
/* rotates the div element by 180 degree along y-axis */
div.rotate {
transform: rotateY(180deg);
}
Browser Output
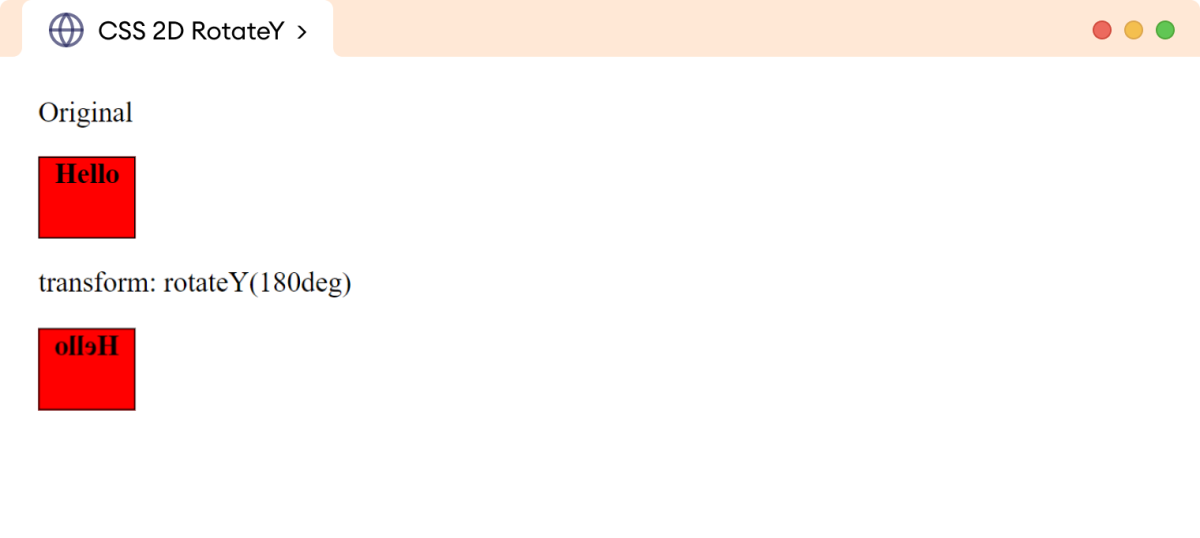
In the above example,
transform: rotateY(180deg)
rotates the second div
element in a clockwise direction by 180
degrees along the y-axis.
The text Hello
inside the first div
appears to be mirrored after the rotation.
scale() Method
The scale()
method scales the element i.e. increases or decreases the size of an element along the x and/or y-axes.
The syntax of scale()
method is:
transform: scale(x-value, y-value)
Here, x-value
and y-value
are the scaling factors to scale the element along the x-axis and y-axis, respectively.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 2D Scale</title>
</head>
<body>
<p>Original</p>
<div></div>
<p>transform: scale(2, 0.5)</p>
<div class="scale"></div>
</body>
</html>
/* styles both divs */
div {
width: 60px;
height: 50px;
margin-left:50px;
background-color: red;
border: 1px solid black;
}
/* scales the div element to twice its size in x-axis and half its size in y-axis*/
div.scale {
transform: scale(2, 0.5);
}
Browser Output
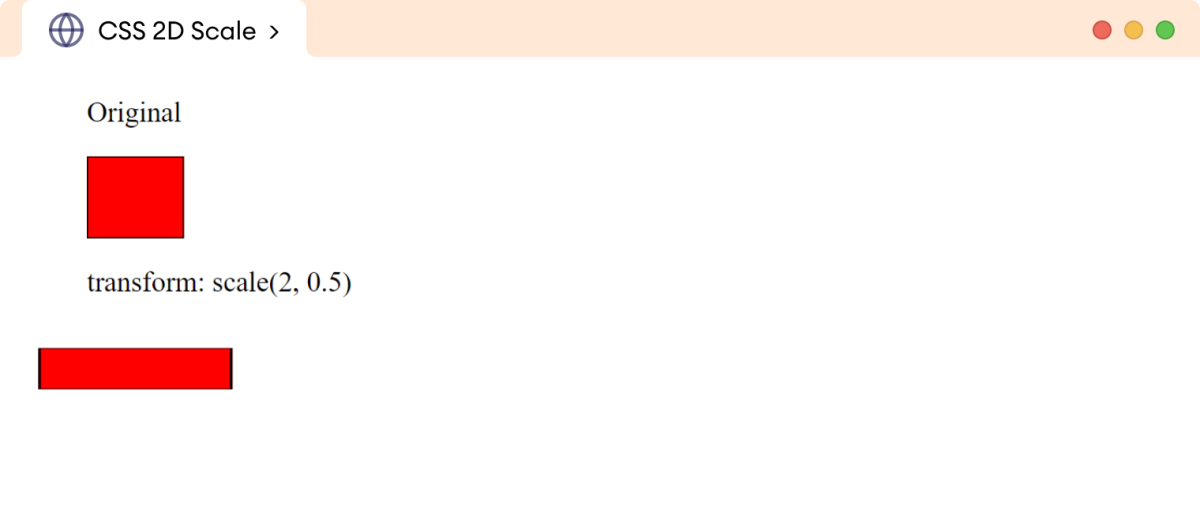
In the above example,
transform: scale(2, 0.5)
scales the second div
element by twice the width in the x-axis and half the height in the y-axis.
The scaleX() and scaleY() Methods
The scaleX()
method scales the element only horizontally while keeping its height unchanged. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 2D ScaleX</title>
</head>
<body>
<p>Original</p>
<div></div>
<p>transform: scaleX(2)</p>
<div class="scale"></div>
</body>
</html>
/* styles both divs */
div {
width: 60px;
height: 50px;
background-color: red;
border: 1px solid black;
margin-left: 50px;
}
/* scales the div element to twice its size in x-axis */
div.scale {
transform: scaleX(2);
}
Browser Output
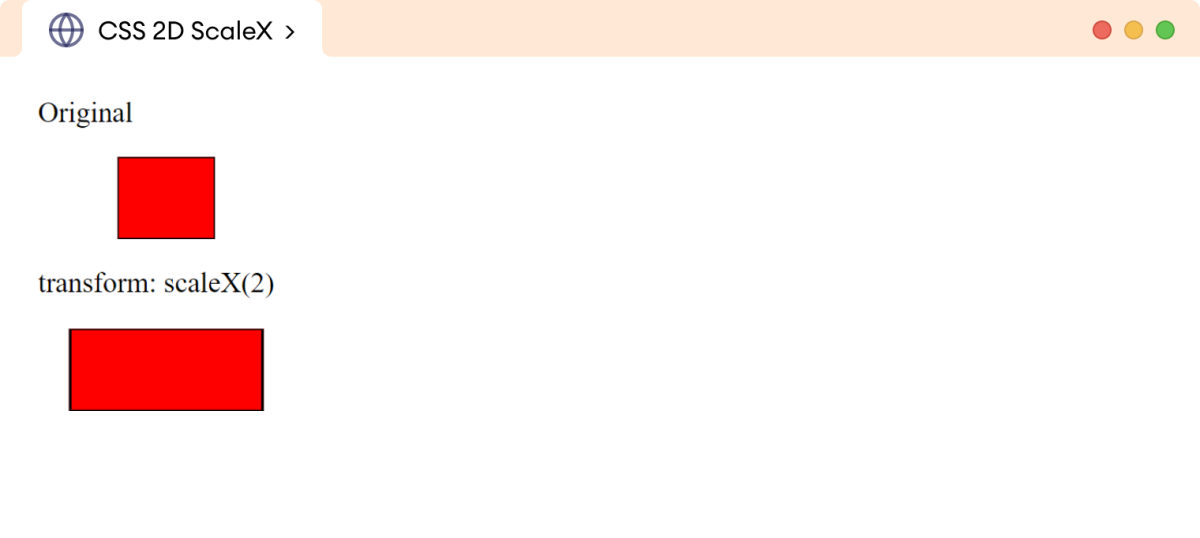
In the above example,
transform: scaleX(2)
scales the width of the second div
element by two times the original width in the x-axis.
The scaleY()
method scales the element only vertically while keeping its width constant. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 2D ScaleY</title>
</head>
<body>
<p>Original</p>
<div></div>
<p>transform: scaleY(1.5)</p>
<div class="scale"></div>
</body>
</html>
/* styles both divs */
div {
width: 60px;
height: 50px;
background-color: red;
border: 1px solid black;
}
/* scales the div element to 1.5 times of its size along y-axis */
div.scale {
transform: scaleY(1.5);
}
Browser Output
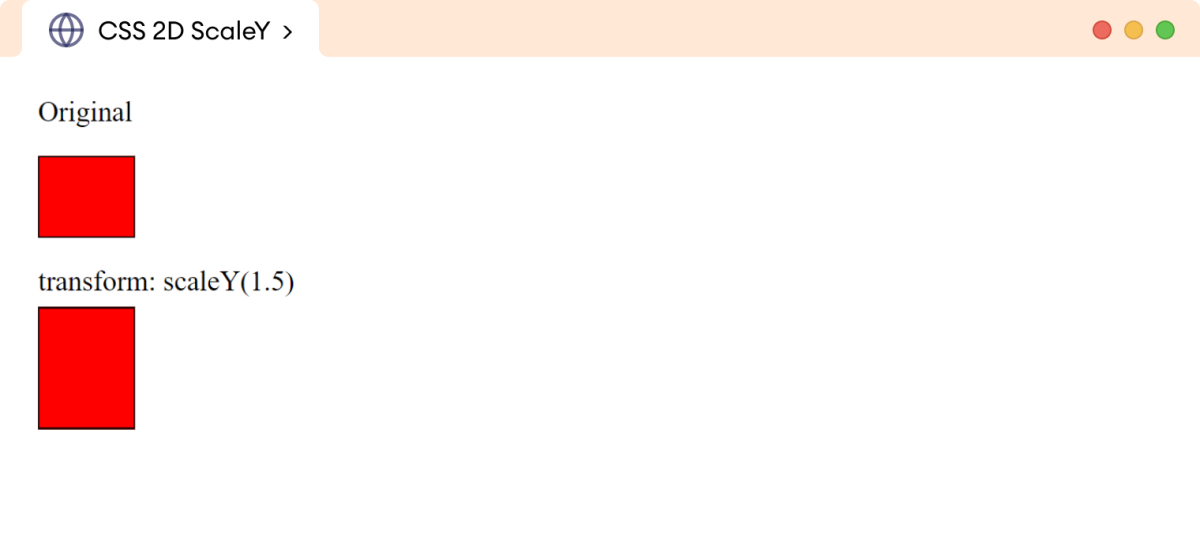
In the above example,
transform:scaleY(1.5)
scales the height of the element by 1.5
times of its original height in the y-axis.
skew() Method
The skew()
method tilts an element along the x-axis and/or y-axis as per a given angle.
The syntax of skew()
method is:
transform: skew(x-angle,y-angle)
Here, x-angle
and y-angle
represent the degree by which the elements will be skewed along the x-axis and y-axis, respectively.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 2D Skew</title>
</head>
<body>
<p>Original</p>
<div></div>
<p>transform: skew(10deg, 20deg)</p>
<div class="skew"></div>
</body>
</html>
/* styles both divs */
div {
width: 60px;
height: 50px;
background-color: red;
border: 1px solid black;
}
/* skew the div element by 10 degrees along the x-axis and 20 degrees along y-axis */
div.skew {
transform: skew(10deg, 20deg);
}
Browser Output
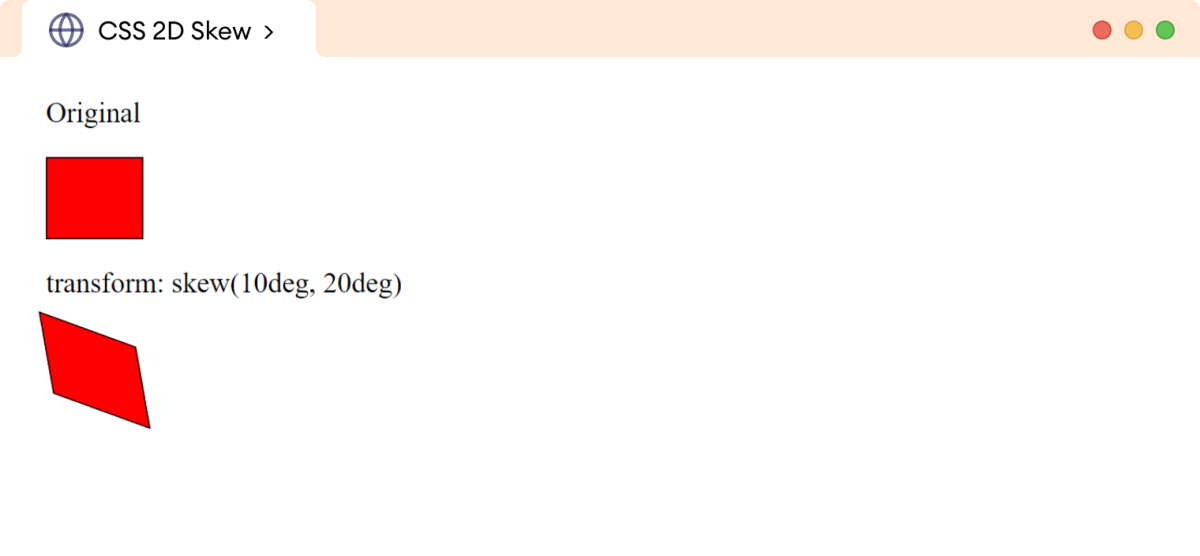
In the above example,
transform: skew(10deg, 20deg)
skews the second div
element by 10
degrees along the x-axis and 20
degrees along the y-axis.
This leads the element to be slanted or skewed in both horizontal and vertical directions.
Note: The skew angles can be positive or negative, depending on the direction we want to skew the element. Positive values tilt the element in one direction, while negative values tilt it in the opposite direction.
The skewX() and skewY() Methods
The skewX()
method skews an element along the x-axis as per given angle. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 2D SkewX</title>
</head>
<body>
<p>Original</p>
<div></div>
<p>transform: skewX(30deg)</p>
<div class="skew"></div>
</body>
</html>
/* styles both divs */
div {
width: 60px;
height: 50px;
background-color: red;
border: 1px solid black;
}
/* skews the div element by 30 degree along x-axis */
div.skew {
transform: skewX(30deg);
}
Browser Output
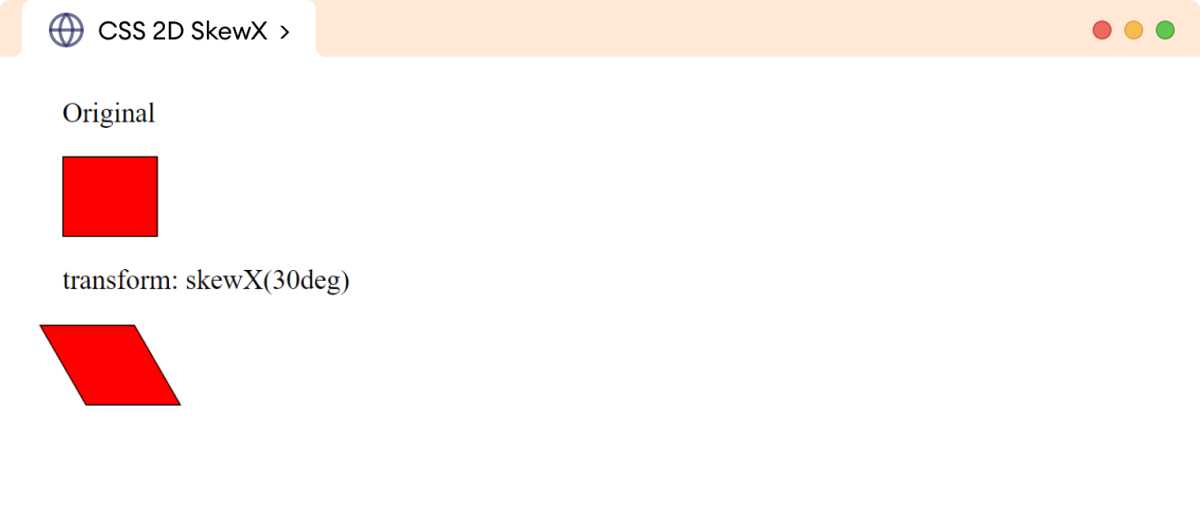
In the above example,
transform: skewX(30deg))
skews the second div
element by 30
degrees along the x-axis.
The skewY()
method skews an element along the y-axis as per a given angle. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 2D SkewY</title>
</head>
<body>
<p>Original</p>
<div></div>
<p>transform: skewY(30deg)</p>
<div class="skew"></div>
</body>
</html>
/* styles both divs */
div {
width: 60px;
height: 50px;
background-color: red;
border: 1px solid black;
}
/* skews the div element by 30 degrees along y-axis */
div.skew {
transform: skewY(30deg);
}
Browser Output
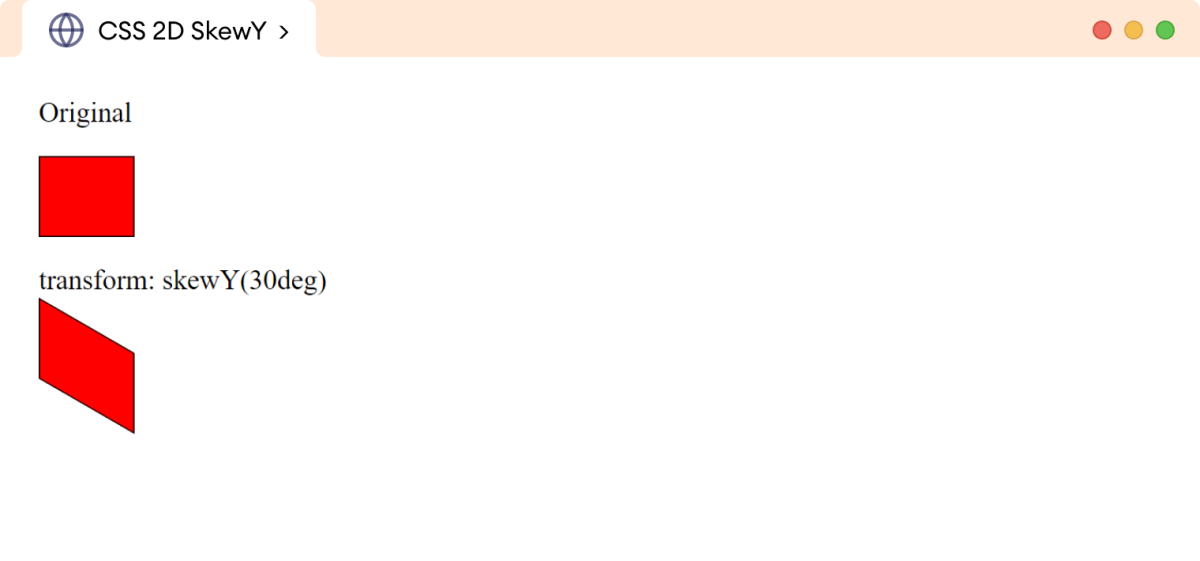
In the above example,
transform: skewY(30deg)
skews the second div
element by 30
degrees along the y-axis.
matrix() Method
The matrix()
method combines all the 2D transform methods into a single method.
The syntax of matrix()
method is:
matrix(scaleX(), skewY(), skewX(), scaleY(), translateX(), translateY())
The matrix()
method takes six parameters that allow it to rotate, scale, translate, and skew the elements.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 2D Matrix</title>
</head>
<body>
<p>Original</p>
<div></div>
<p>transform: matrix(1.5, 0.5,- .0.5, 1, 50, 10)</p>
<div class="matrix"></div>
</body>
</html>
/* styles both divs */
div {
width: 60px;
height: 50px;
background-color: red;
border: 1px solid black;
}
/* applies matrix transformation to the div element*/
div.matrix {
transform: matrix(1.5, 0.5, -0.5, 1, 50, 10);
}
Browser Output
In the above example,
transform: matrix(1.5, 0.5, -0.5, 1, 50, 10)
- scales the
div
horizontally by1.5
times of its width - skews the
div
vertically by a radian of0.5
, causing slanting effect - skews the
div
vertically by a radian of-0.5
,causing slanting effect in opposite direction - doesn't scale the
div
vertically - translates the
div
horizontally by50
pixels to the right in horizontal direction - translates the
div
by10
pixels downward in the vertical direction
Note: The skew values in the matrix()
function are specified in radians, not degrees.