Grid items are the direct child elements of the flex container.
In the following diagram, the purple
box represents the grid container with three grid items as orange
boxes.
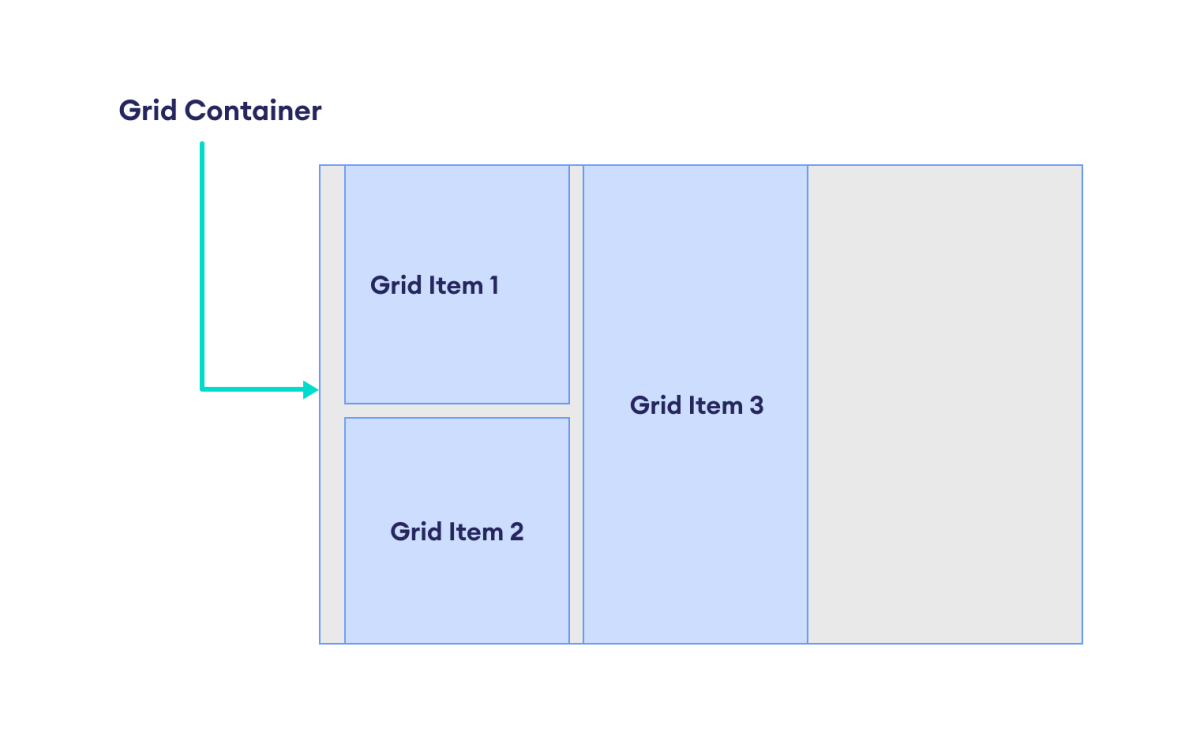
There are different sets of properties for placing and aligning the grid items within the grid container.
Grid Line Numbers
CSS grid consists of a set of horizontal and vertical lines, forming rows and columns. These lines are referred to by their line numbers.
The grid items within the grid container can be placed using these line numbers.
The four adjacent grid lines form a grid cell. The grid item can be placed within one or more grid cells.
Note: The grid lines start from the top left edge of the grid container. The row line numbers increase downwards, and column line numbers increase to the right.
Placing the Grid Items With Line Numbers
The grid items can be placed with the help of grid line numbers. The following properties control the placement of grid items using grid line numbers.
grid-column-start
: defines the starting column line number
grid-column-end
: defines the ending column line number
grid-row-start
: defines the starting row line number
grid-row-end
: defines the ending row line number
These properties take the following values:
line-number
: number to refer to a numbered grid line
span <number>
: grid item will span across the provided number of grid tracks
Example 1: Placing Grid items
Let's place the grid items using the grid line numbers.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Items</title>
</head>
<body>
<div class="container">
<div class="item item-1">1</div>
<div class="item item-2">2</div>
<div class="item item-3">3</div>
<div class="item item-4">4</div>
<div class="item item-5">5</div>
</div>
</body>
</html>
div.container {
/* sets the element as a grid container */
display: grid;
/* defines the grid of 3 rows and three columns */
grid-template: 100px 100px 50px / 100px 150px 100px;
width: 550px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 5px;
font-weight: bold;
}
/* places the first grid item */
div.item-1 {
grid-row-start: 1;
grid-row-end: 3;
background-color: purple;
}
/* places the second grid item */
div.item-2 {
grid-column-start: 2;
grid-column-end: 4;
background-color: red;
}
Browser Output
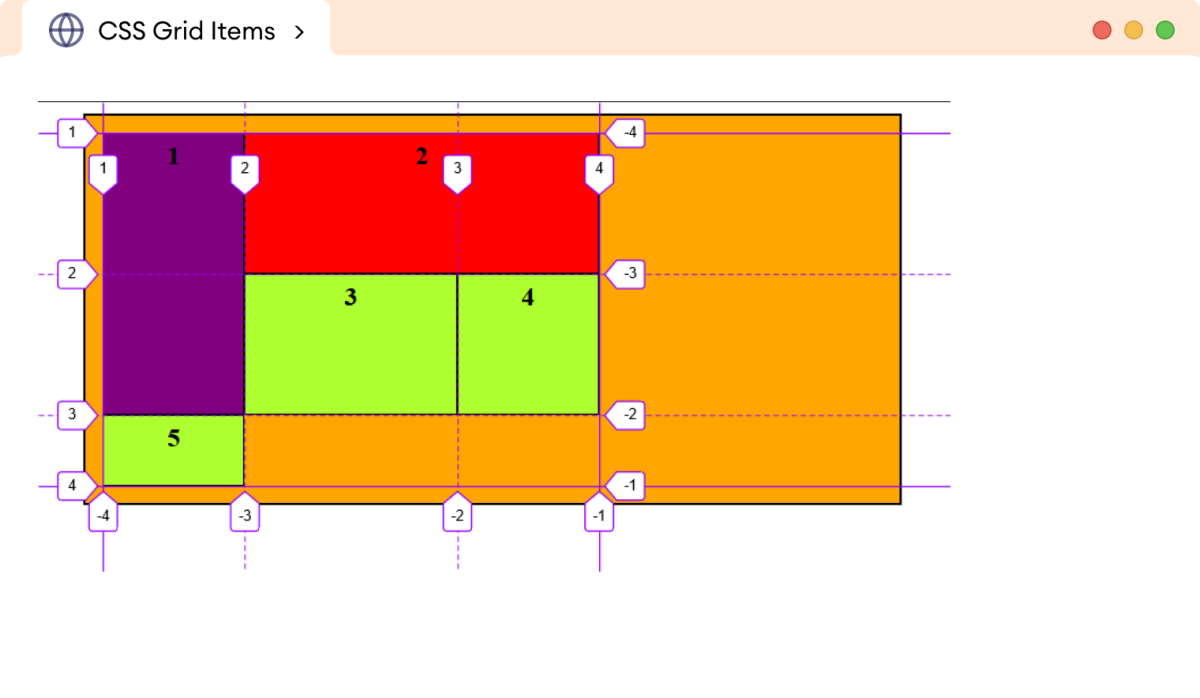
Here,
div.item-1 {
grid-row-start: 1;
grid-row-end: 3;
background-color: purple;
}
sets the first item (purple-colored) from row line 1 to row line 3.
Similarly,
div.item-2 {
grid-column-start: 2;
grid-column-end: 4;
background-color: red;
}
sets the second item (red-colored) from column line 2 to column line 4.
Grid items automatically fill the grid cells in a sequential manner within the grid container by default.
Note: We cannot visualize the grid lines in the browser output, as they are used to position elements on the page and are not meant to be displayed. However, we can use the Grid Inspector provided by the Mozilla Browser in the console to visualize the grid lines.
CSS grid-row and grid-column Properties
The grid-row
and grid-column
are the shorthand properties to specify the grid line numbers.
Here,
grid-row
: defines thegrid-row-start
andgrid-row-end
propertiesgrid-column
: defines thegrid-column-start
andgrid-column-end
properties
The syntax of grid-row
and grid-column
properties are,
grid-row: grid-row-start / grid-row-end;
grid-column: grid-column-start / grid-column-end;
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Items</title>
</head>
<body>
<div class="container">
<div class="item item-1">1</div>
<div class="item item-2">2</div>
<div class="item item-3">3</div>
<div class="item item-4">4</div>
<div class="item item-5">5</div>
</div>
</body>
</html>
div.container {
/* sets the element as a grid container */
display: grid;
/* defines the grid of 3 rows and three columns */
grid-template: 100px 100px 50px / 100px 150px 100px;
width: 550px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 5px;
font-weight: bold;
}
/* places the first grid item */
div.item-1 {
grid-row: 1/4;
background-color: purple;
}
/* places the second grid item */
div.item-2 {
grid-column: 2/4;
background-color: red;
}
Browser Output
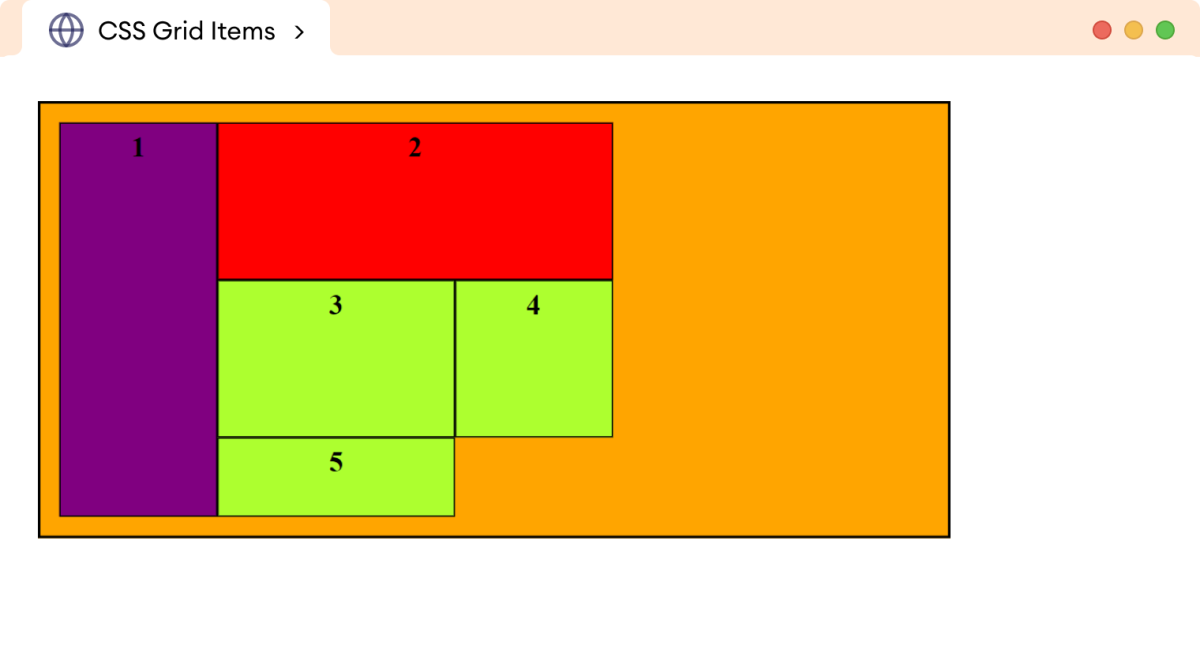
In the above example,
grid-row: 1/4
places the first grid item (purple boxes) from row line 1 to row line 4 within the grid container.
Similarly,
grid-column: 2/4
places the second grid item (red boxes) from column line 2 to column line 4.
Note: Grid lines can also be assigned custom names, and grid items can be positioned using these names. Line names must be defined while specifying the grid columns and rows.
Spanning the rows and columns
The span
keyword is used to extend rows and columns by a specified number.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Items</title>
</head>
<body>
<div class="container">
<div class="item item-1">1</div>
<div class="item item-2">2</div>
<div class="item item-3">3</div>
<div class="item item-4">4</div>
</div>
</body>
</html>
div.container {
/* sets the element as a grid container */
display: grid;
/* defines the grid of 3 rows and three columns */
grid-template: 100px 100px 50px / 100px 150px 100px;
width: 550px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 5px;
font-weight: bold;
}
/* places the first grid item */
div.item-1 {
grid-column: 1 / span 3;
background-color: purple;
}
Browser Output
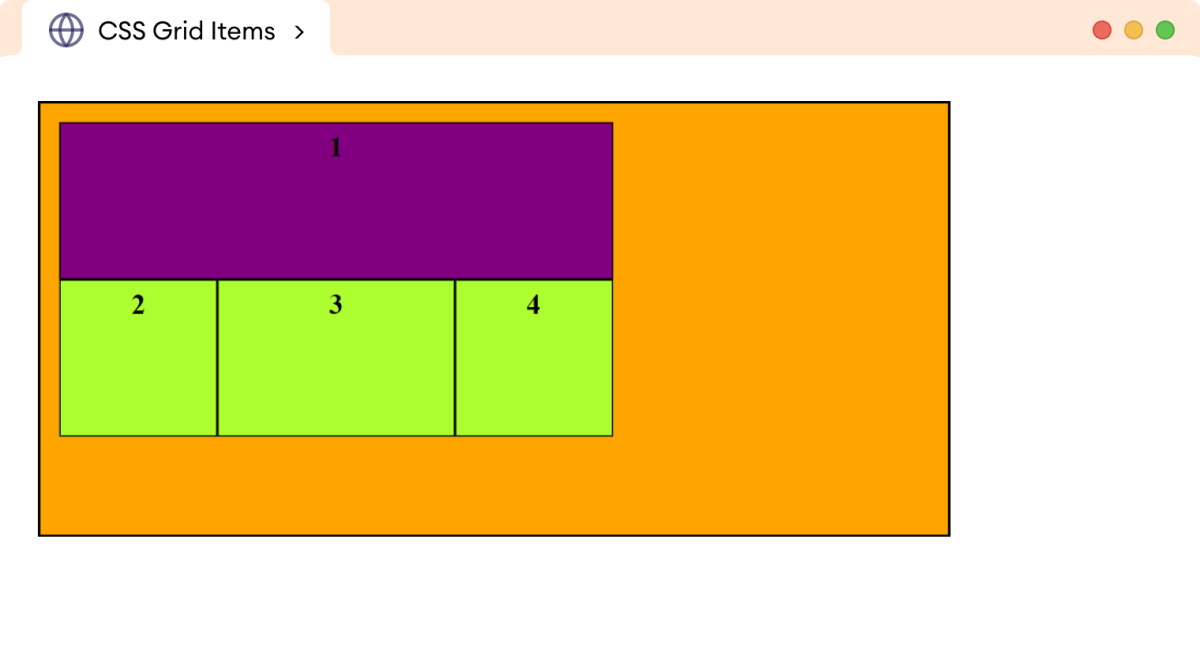
In the above example,
grid-column: 1 / span 3;
extends the first grid item from column line 1 to column line 3 within the grid container.
CSS grid-area Property
The grid-area
property specifies both the row and column positions of a grid item in a single declaration.
The syntax for the grid-area
property is as follows,
grid-area: row-start / column-start / row-end / column-end | item-name;
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Items</title>
</head>
<body>
<div class="container">
<div class="item item-1">1</div>
<div class="item item-2">2</div>
<div class="item item-3">3</div>
<div class="item item-4">4</div>
</div>
</body>
</html>
div.container {
/* sets the element as a grid container */
display: grid;
/* defines the grid of 3 rows and three columns */
grid-template: 100px 100px 50px / 100px 150px 100px;
width: 550px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 5px;
font-weight: bold;
}
/* places the first grid item */
div.item-1 {
grid-area: 1 / 1 / 3/ 3;
background-color: purple;
}
Browser Output
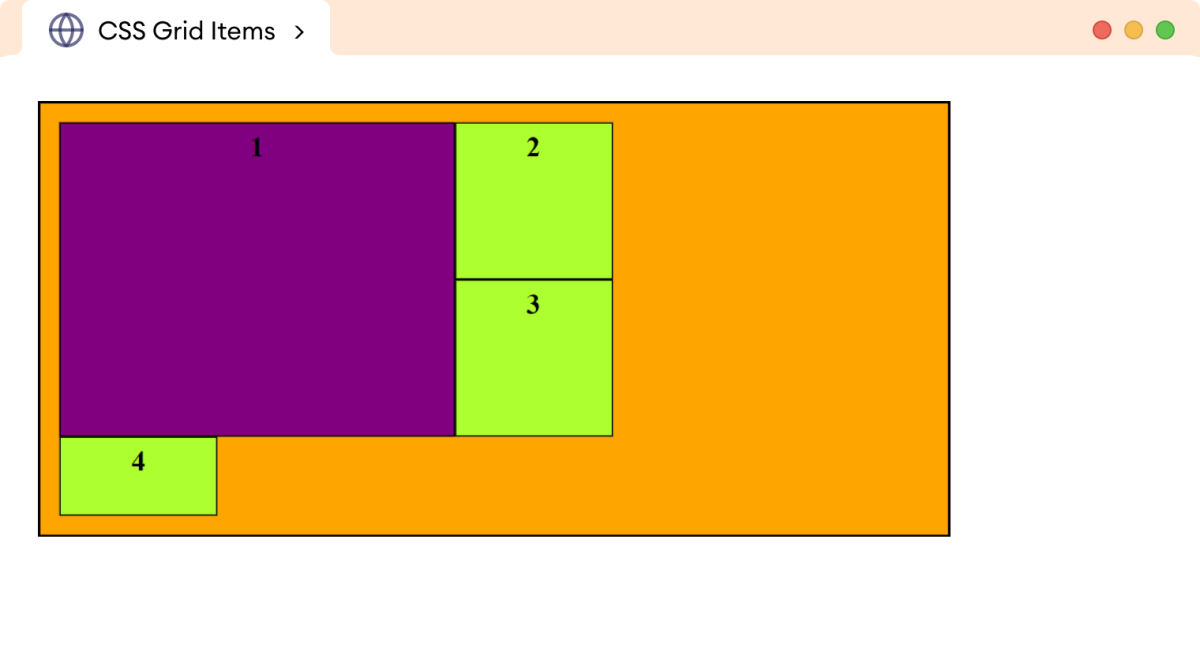
In the above example,
grid-area: 1 / 1 / 3 / 3
is equivalent to
grid-row-start: 1;
grid-column-start: 1;
grid-row-end: 3;
grid-column-end: 3;
Note: The grid-area
property can be specified with an area name, which corresponds to the named grid areas defined by the grid-template-areas
property.
CSS justify-self Property
The justify-self
property is used to control the horizontal alignment of a grid item within its grid cell.
The possible values of the justify-self
property are start
, end
, center
, and stretch
.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Items</title>
</head>
<body>
<div class="container">
<div class="item item-1">1</div>
<div class="item item-2">2</div>
<div class="item item-3">3</div>
<div class="item item-4">4</div>
<div class="item item-5">5</div>
<div class="item item-6">6</div>
</div>
</body>
</html>
div.container {
/* sets the element as a grid container */
display: grid;
/* defines the grid of 2 rows and three columns */
grid-template: 100px 100px / 100px 150px 100px;
width: 550px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 5px;
font-weight: bold;
}
/* aligns the content to the end of grid cell */
div.item-1 {
justify-self: end;
background-color: purple;
}
Browser Output
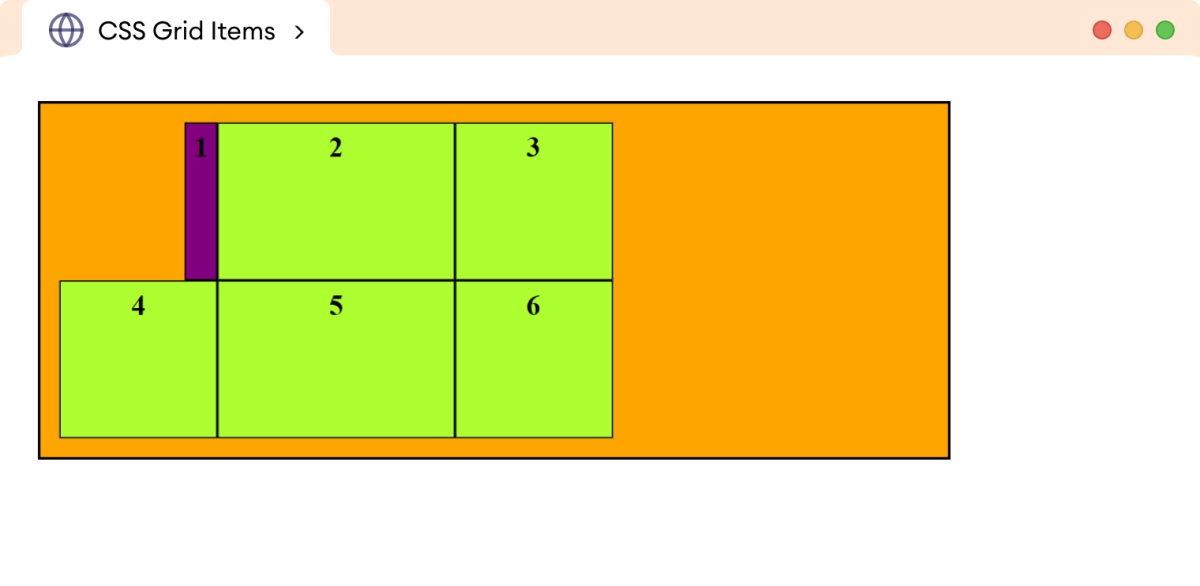
In the above example, justify-self: end
aligns the grid content of the first item to the end
of the grid cell.
Values of justify-self
The start
value of the justify-self
property aligns the grid content to the start of the grid cell. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Items</title>
</head>
<body>
<div class="container">
<div class="item item-1">1</div>
<div class="item item-2">2</div>
<div class="item item-3">3</div>
<div class="item item-4">4</div>
<div class="item item-5">5</div>
<div class="item item-6">6</div>
</div>
</body>
</html>
div.container {
/* sets the element as a grid container */
display: grid;
/* defines the grid of 2 rows and three columns */
grid-template: 100px 100px / 100px 150px 100px;
width: 550px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 5px;
font-weight: bold;
}
/* aligns the content to the start of grid cell */
div.item-1 {
justify-self: start;
background-color: purple;
}
Browser Output
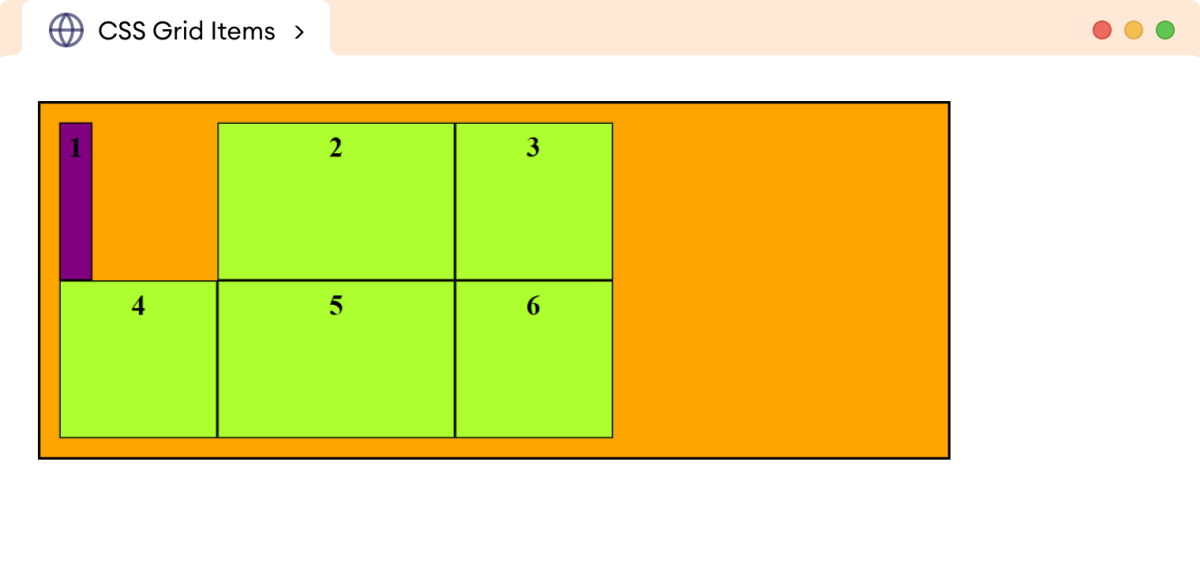
The center
value of the justify-self
property aligns the grid content to the center of the grid cell. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Items</title>
</head>
<body>
<div class="container">
<div class="item item-1">1</div>
<div class="item item-2">2</div>
<div class="item item-3">3</div>
<div class="item item-4">4</div>
<div class="item item-5">5</div>
<div class="item item-6">6</div>
</div>
</body>
</html>
div.container {
/* sets the element as a grid container */
display: grid;
/* defines the grid of 2 rows and three columns */
grid-template: 100px 100px / 100px 150px 100px;
width: 550px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 5px;
font-weight: bold;
}
/* aligns the content to the center of grid cell */
div.item-1 {
justify-self: center;
background-color: purple;
}
Browser Output
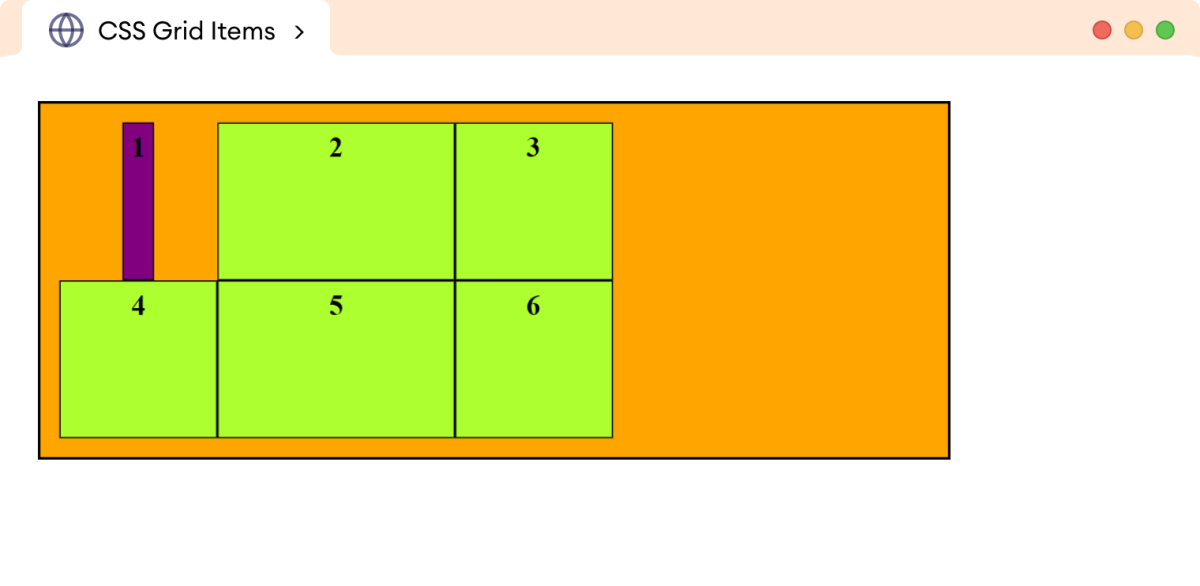
The stretch
value of the justify-self
stretches the content to fill the grid cell. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Items</title>
</head>
<body>
<div class="container">
<div class="item item-1">1</div>
<div class="item item-2">2</div>
<div class="item item-3">3</div>
<div class="item item-4">4</div>
<div class="item item-5">5</div>
<div class="item item-6">6</div>
</div>
</body>
</html>
div.container {
/* sets the element as a grid container */
display: grid;
/* defines the grid of 2 rows and three columns */
grid-template: 100px 100px / 100px 150px 100px;
width: 550px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 5px;
font-weight: bold;
}
/* stretches the content to fill the grid cell */
div.item-1 {
justify-self: stretch;
background-color: purple;
}
Browser Output
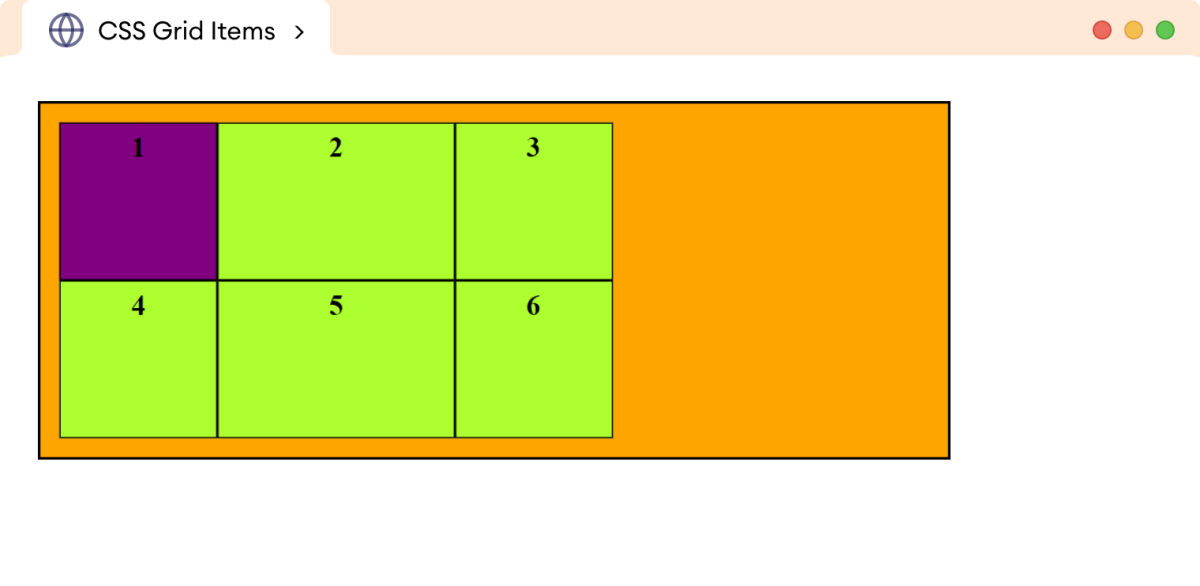
CSS align-self Property
The align-self
property is used to control the vertical alignment of a grid item within its grid cell.
The possible values of the align-self
property are start
, end
, center
, and stretch
.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Items</title>
</head>
<body>
<div class="container">
<div class="item item-1">1</div>
<div class="item item-2">2</div>
<div class="item item-3">3</div>
<div class="item item-4">4</div>
<div class="item item-5">5</div>
<div class="item item-6">6</div>
</div>
</body>
</html>
div.container {
/* sets the element as a grid container */
display: grid;
/* defines the grid of 2 rows and three columns */
grid-template: 100px 100px / 100px 150px 100px;
width: 550px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 5px;
font-weight: bold;
}
/* aligns the content to the end of grid cell */
div.item-1 {
align-self: end;
background-color: purple;
}
Browser Output
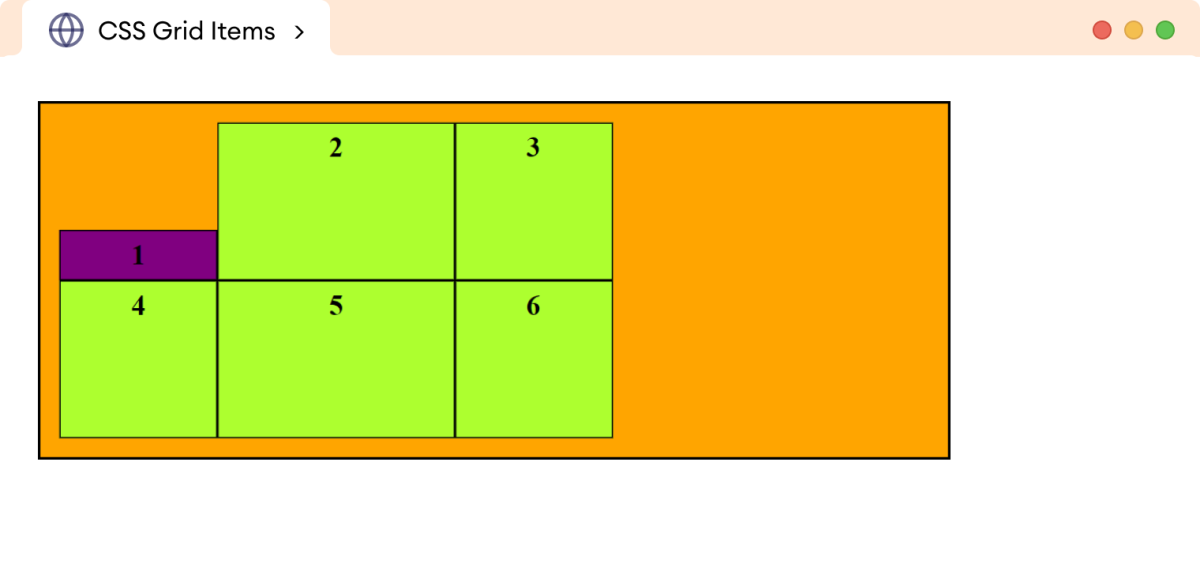
In the above example, align-self: end
aligns the grid content of the first item to the end of the grid cell.
Values of align-self
The start
value of the align-self
property aligns the grid content to the start of the grid cell. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Items</title>
</head>
<body>
<div class="container">
<div class="item item-1">1</div>
<div class="item item-2">2</div>
<div class="item item-3">3</div>
<div class="item item-4">4</div>
<div class="item item-5">5</div>
<div class="item item-6">6</div>
</div>
</body>
</html>
div.container {
/* sets the element as a grid container */
display: grid;
/* defines the grid of 2 rows and three columns */
grid-template: 100px 100px / 100px 150px 100px;
width: 550px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 5px;
font-weight: bold;
}
/* aligns the content to the start of grid cell */
div.item-1 {
align-self: start;
background-color: purple;
}
Browser Output
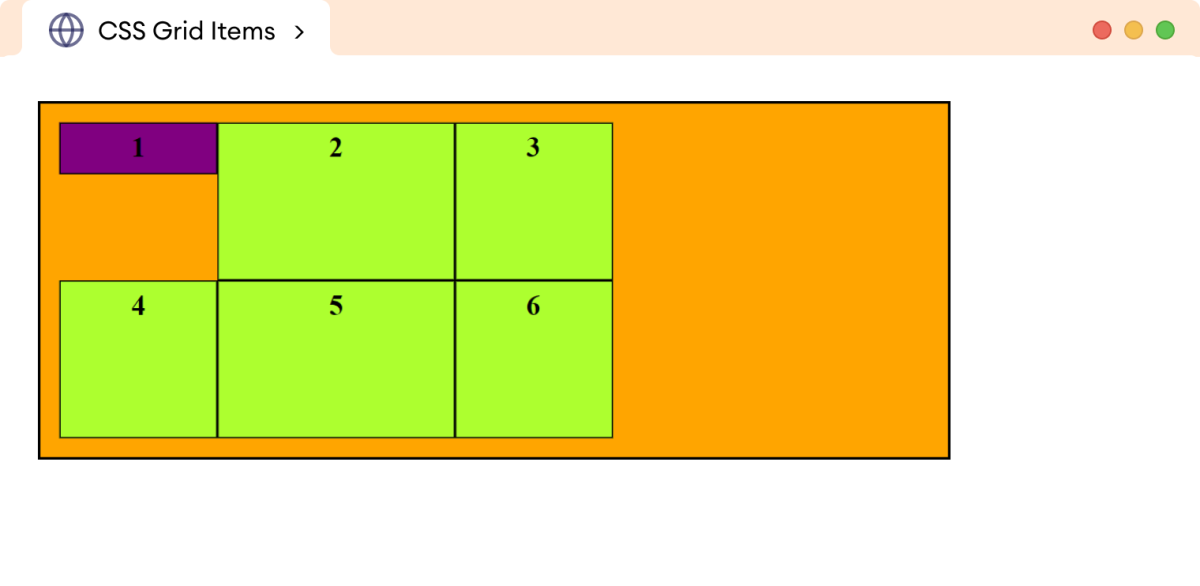
The center
value of the align-self
property aligns the grid content to the center of the grid cell. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Items</title>
</head>
<body>
<div class="container">
<div class="item item-1">1</div>
<div class="item item-2">2</div>
<div class="item item-3">3</div>
<div class="item item-4">4</div>
<div class="item item-5">5</div>
<div class="item item-6">6</div>
</div>
</body>
</html>
div.container {
/* sets the element as a grid container */
display: grid;
/* defines the grid of 2 rows and three columns */
grid-template: 100px 100px / 100px 150px 100px;
width: 550px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 5px;
font-weight: bold;
}
/* aligns the content to the center of grid cell */
div.item-1 {
align-self: center;
background-color: purple;
}
Browser Output
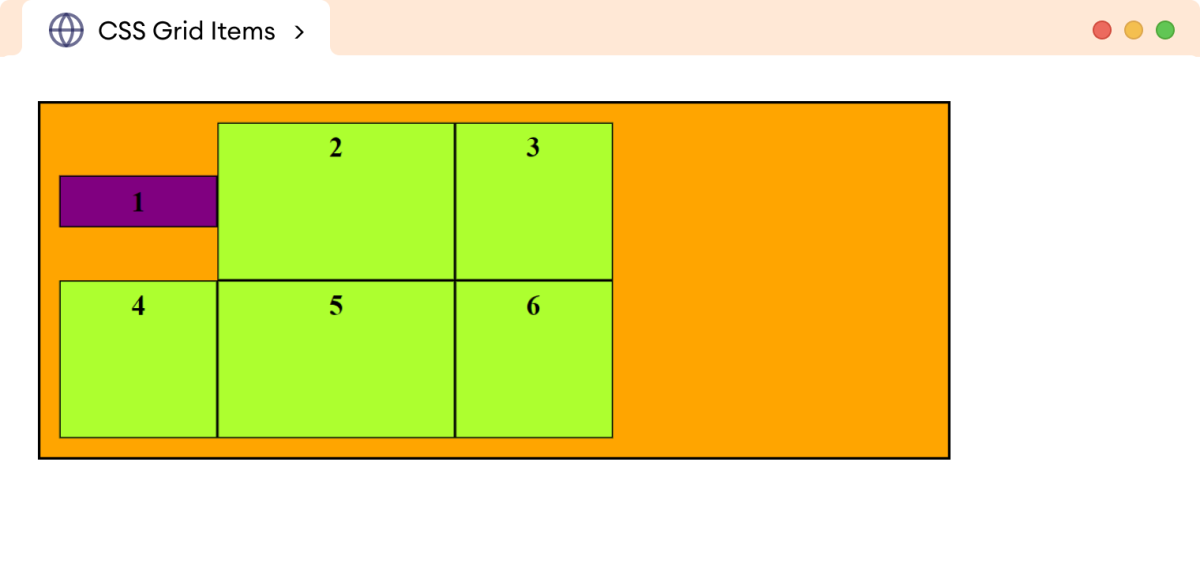
The stretch
value of the align-self
stretches the content to fill the grid cell. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Items</title>
</head>
<body>
<div class="container">
<div class="item item-1">1</div>
<div class="item item-2">2</div>
<div class="item item-3">3</div>
<div class="item item-4">4</div>
<div class="item item-5">5</div>
<div class="item item-6">6</div>
</div>
</body>
</html>
div.container {
/* sets the element as a grid container */
display: grid;
/* defines the grid of 2 rows and three columns */
grid-template: 100px 100px / 100px 150px 100px;
width: 550px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 5px;
font-weight: bold;
}
/* stretches the content to fill the grid cell */
div.item-1 {
align-self: stretch;
background-color: purple;
}
Browser Output
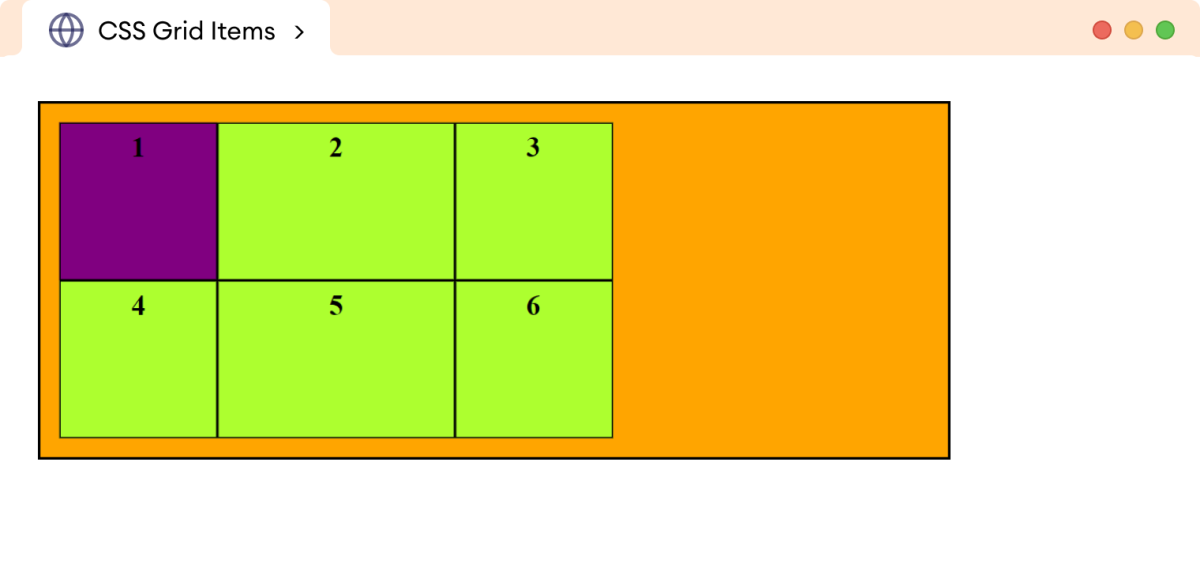
CSS place-self Property
The place-self
property is a shorthand property to specify the justify-self
and align-self
property in a single declaration.
The syntax of the place-self property is as follows:
place-self: align-self place-self;
Here, the first value sets align-self
, and the second value sets the justify-self
property. If the second value is omitted, the first value is assigned to both properties.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Items</title>
</head>
<body>
<div class="container">
<div class="item item-1">1</div>
<div class="item item-2">2</div>
<div class="item item-3">3</div>
<div class="item item-4">4</div>
<div class="item item-5">5</div>
<div class="item item-6">6</div>
</div>
</body>
</html>
div.container {
/* sets the element as a grid container */
display: grid;
/* defines the grid of 2 rows and three columns */
grid-template: 100px 100px / 100px 150px 100px;
width: 550px;
border: 2px solid black;
background-color: orange;
padding: 12px;
}
/* styles all grid items */
div.item {
border: 1px solid black;
background-color: greenyellow;
text-align: center;
padding: 5px;
font-weight: bold;
}
/* aligns the content to the end of grid cell */
div.item-1 {
place-self: center center;
background-color: purple;
}
Browser Output
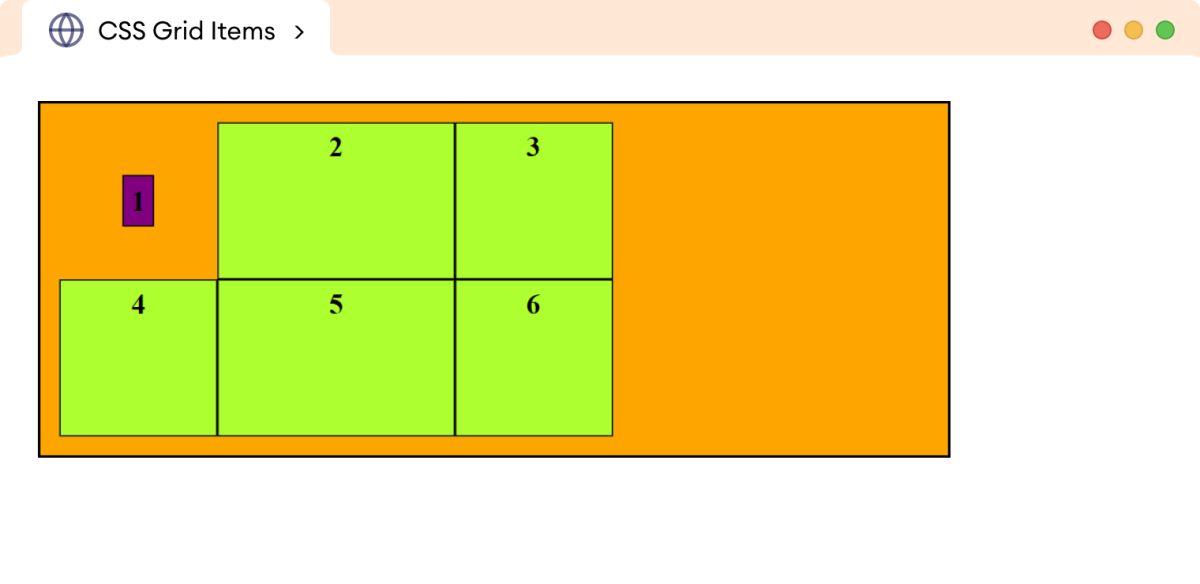
In the above example,
place-self: center center;
aligns the grid content horizontally and vertically center within a grid cell.