A flex container is a parent element having child elements known as flex items.
In the following diagram, the purple
container is a flex container with three flex items in orange
boxes.
These flex items are arranged and aligned using different flex container properties.
The flexbox layout model needs a flex container before using any flex-based properties. The display
property with the flex
value sets an element as a flex container.
<!-- A div element (flex container) having
three paragraphs (flex items) →
<div>
<p>First Item</p>
<p>Second Item</p>
<p>Third Item</p>
</div>
/* CSS */
div {
display: flex;
}
Here, display: flex
sets the div
element as a flex container. All the direct elements within the div
element are referred to as flex items.
The distribution and alignment of space within the flex container are adjusted with the help of the following properties.
- flex-direction
- flex-wrap
- flex-flow
- justify-content
- align-items
- align-content
- gap, row-gap, column-gap
We will look into each of the above flex container properties one by one.
Flex Direction
The flex-direction
property specifies the direction of flex items within the flex container.
The possible value of the flex-direction
property is row
(default value), row-reverse
, column
, and column-reverse
.
Let's look into each of these values.
flex-direction: row
The row
value arranges flex items horizontally from left to right. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS flex-direction</title>
</head>
<body>
<h2>flex-direction: row (default)</h2>
<div class="container">
<div class="box">1</div>
<div class="box">2</div>
<div class="box">3</div>
<div class="box">4</div>
<div class="box">5</div>
</div>
</body>
</html>
div.container {
display: flex;
flex-direction: row;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 40px;
height: 40px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
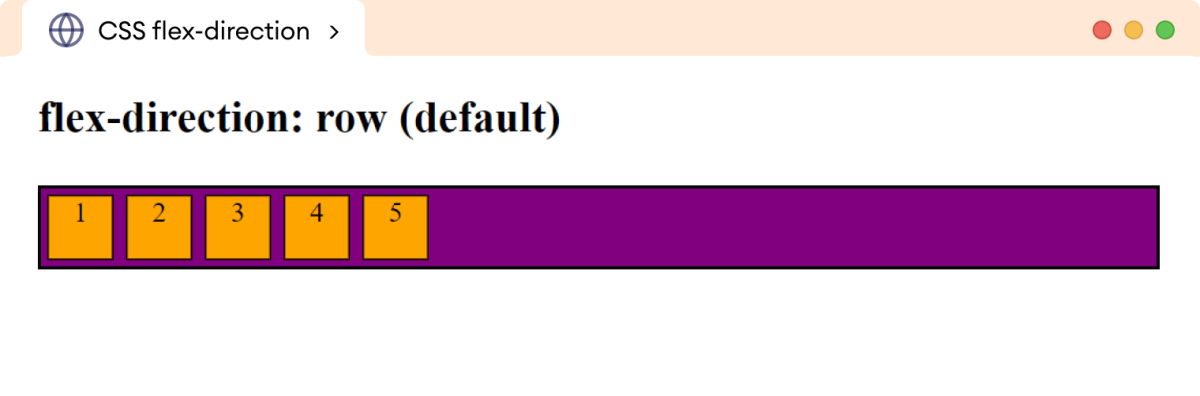
Values of CSS Flex-Direction
The row-reverse value arranges flex items horizontally but in the reverse order. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS flex-direction</title>
</head>
<body>
<h2>flex-direction: row-reverse</h2>
<div class="container">
<div class="box">1</div>
<div class="box">2</div>
<div class="box">3</div>
<div class="box">4</div>
<div class="box">5</div>
</div>
</body>
</html>
div.container {
display: flex;
flex-direction: row-reverse;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 40px;
height: 40px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
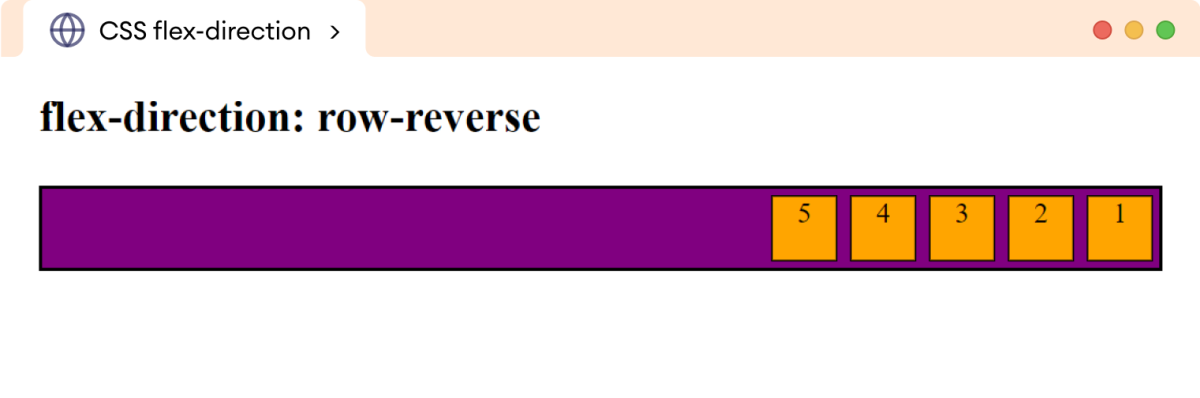
The column value arranges flex items vertically from top to bottom. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS flex-direction</title>
</head>
<body>
<h2>flex-direction: column</h2>
<div class="container">
<div class="box">1</div>
<div class="box">2</div>
<div class="box">3</div>
</div>
</body>
</html>
div.container {
display: flex;
flex-direction: column;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 40px;
height: 40px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
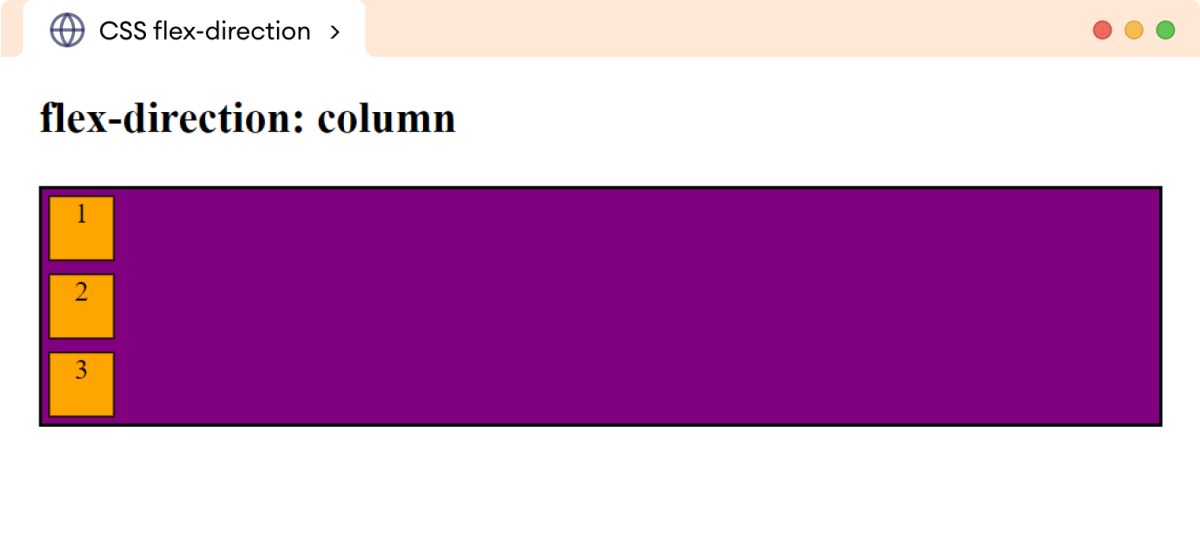
The column-reverse value arranges flex items vertically but in reverse order. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS flex-direction</title>
</head>
<body>
<h2>flex-direction: column-reverse</h2>
<div class="container">
<div class="box">1</div>
<div class="box">2</div>
<div class="box">3</div>
</div>
</body>
</html>
div.container {
display: flex;
/* reverses the items in column */
flex-direction: column-reverse;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 40px;
height: 40px;
text-align: center;
border: 1px solid black;
background-color: orange;
/* sets the margin around each flex item */
margin: 4px;
}
Browser Output
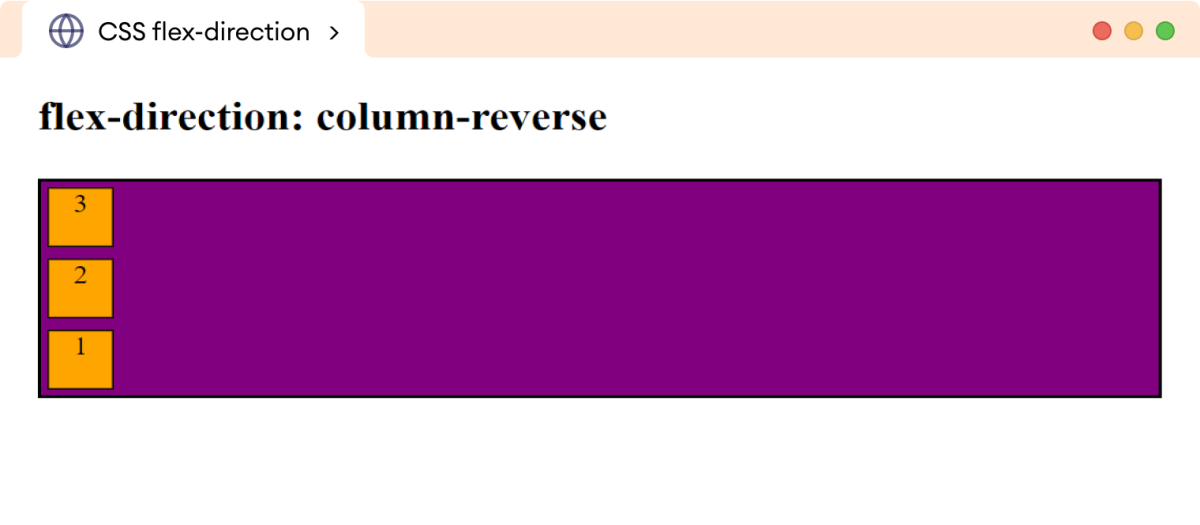
Flex Wrap
The flex-wrap
value allows flex items to wrap in multiple lines. By default, all the flex items are squeezed in a single horizontal direction (row).
The possible values for the flex-wrap
property are nowrap
(default value), wrap
, and wrap-reverse
.
Let's look into each of these values.
flex-wrap: nowrap
The nowrap
value of the flex-wrap
property keeps flex items squeezed in the direction set by flex-direction
. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS flex-wrap</title>
</head>
<body>
<h2>flex-wrap: nowrap</h2>
<div class="container">
<div class="box">1</div>
<div class="box">2</div>
<div class="box">3</div>
<div class="box">4</div>
<div class="box">5</div>
<div class="box">6</div>
<div class="box">7</div>
<div class="box">8</div>
<div class="box">9</div>
<div class="box">10</div>
<div class="box">11</div>
<div class="box">12</div>
</div>
</body>
</html>
div.container {
display: flex;
flex-direction: row;
/* prevents wrapping of flex items; default value */
flex-wrap: nowrap;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 80px;
height: 60px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
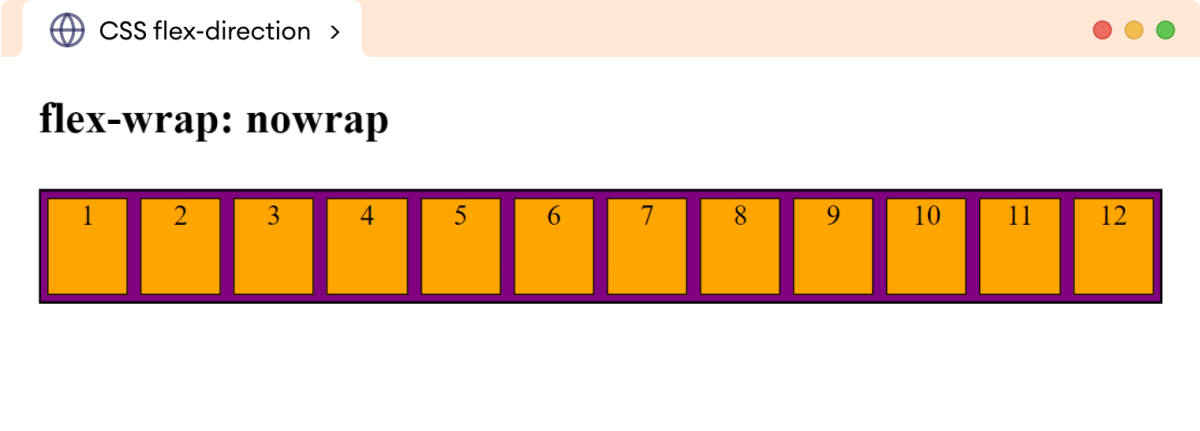
Here, all the flex items are squeezed in the horizontal direction, and their original width is ignored.
Values of CSS Flex-Wrap
The wrap
value allows flex items to be wrapped in multiple lines when their size is too big for the container. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS flex-wrap</title>
</head>
<body>
<h2>flex-wrap: wrap</h2>
<div class="container">
<div class="box">1</div>
<div class="box">2</div>
<div class="box">3</div>
<div class="box">4</div>
<div class="box">5</div>
<div class="box">6</div>
<div class="box">7</div>
<div class="box">8</div>
<div class="box">9</div>
<div class="box">10</div>
<div class="box">11</div>
<div class="box">12</div>
</div>
</body>
</html>
div.container {
display: flex;
flex-direction: row;
/* wraps the flex items in multiple line */
flex-wrap: wrap;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 80px;
height: 60px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
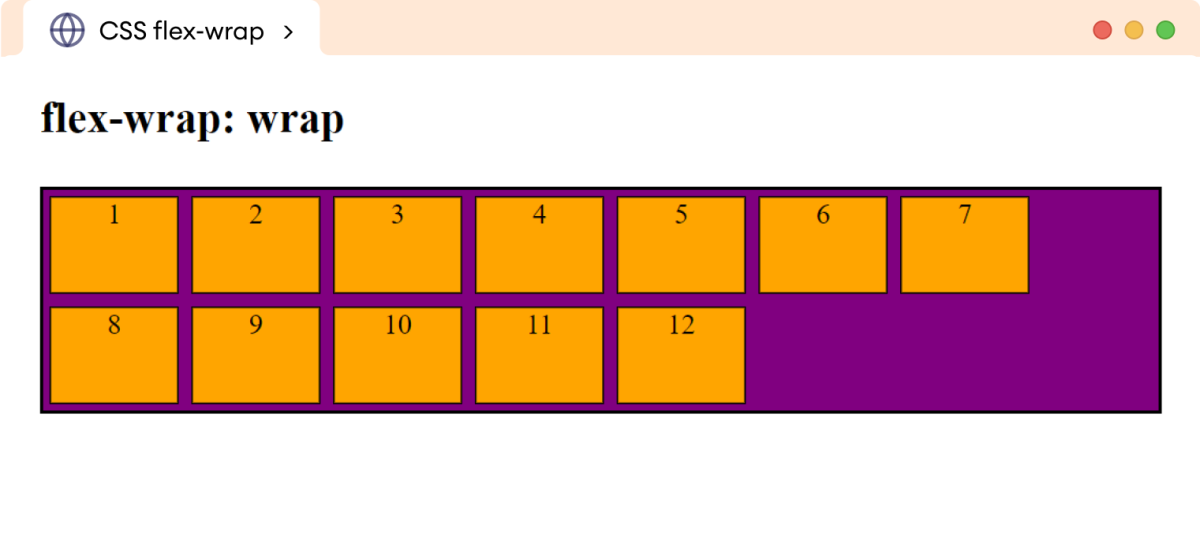
The wrap-reverse
value wraps the flex items but in reverse order. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS flex-wrap</title>
</head>
<body>
<h2>flex-wrap: wrap-reverse</h2>
<div class="container">
<div class="box">1</div>
<div class="box">2</div>
<div class="box">3</div>
<div class="box">4</div>
<div class="box">5</div>
<div class="box">6</div>
<div class="box">7</div>
<div class="box">8</div>
<div class="box">9</div>
<div class="box">10</div>
<div class="box">11</div>
<div class="box">12</div>
</div>
</body>
</html>
div.container {
display: flex;
flex-direction: row;
/* wraps the flex items in multiple lines */
flex-wrap: wrap-reverse;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 80px;
height: 60px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
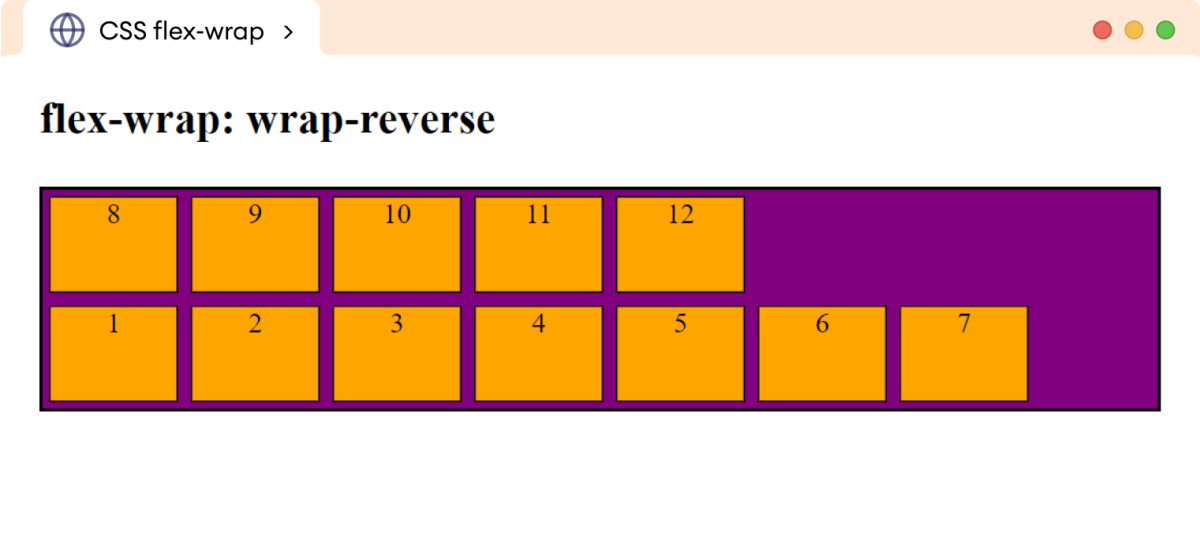
Flex Flow
The flex-flow
is a shorthand property to specify the flex-direction
and flex-wrap
property value. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS flex-flow</title>
</head>
<body>
<h2>flex-flow: row wrap</h2>
<div class="container">
<div class="box">1</div>
<div class="box">2</div>
<div class="box">3</div>
<div class="box">4</div>
<div class="box">5</div>
<div class="box">6</div>
<div class="box">7</div>
<div class="box">8</div>
<div class="box">9</div>
<div class="box">10</div>
<div class="box">11</div>
<div class="box">12</div>
</div>
</body>
</html>
div.container {
display: flex;
/* sets the flex-direction to row and flex-wrap to wrap value */
flex-flow: row wrap;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 80px;
height: 60px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
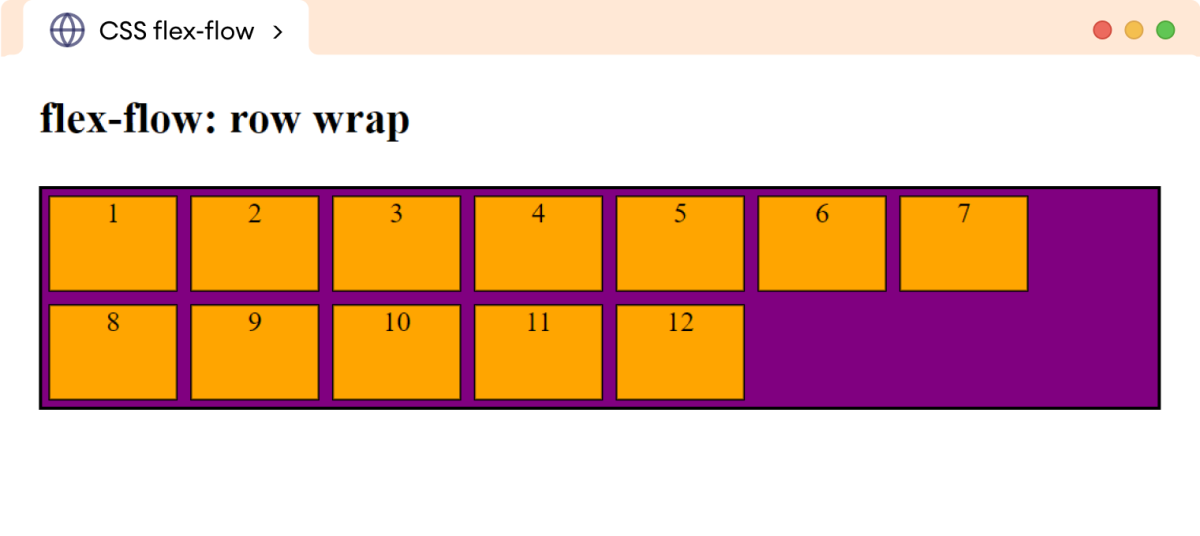
Justify Content
The justify-content
property is used to distribute the available space between the flex items along the main axis.
The space is distributed horizontally for flex-direction: row
and vertically for flex-direction: column
.
The possible values for the justify-content
property are flex-start
, center
, flex-end
, space-between
, space-around
, and space-evenly
.
Let's look at each of these values.
justify-content: flex-start
The flex-start
value aligns the flex items at the start of the main axis (left for the flex-direction: row/row-reverse
and top for the flex-direction: column/column-reverse
.)
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS justify-content</title>
</head>
<body>
<h2>justify-content: flex-start</h2>
<div class="container">
<div class="box">1</div>
<div class="box">2</div>
<div class="box">3</div>
<div class="box">4</div>
<div class="box">5</div>
</div>
</body>
</html>
div.container {
display: flex;
/* default value */
justify-content: flex-start;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 40px;
height: 40px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
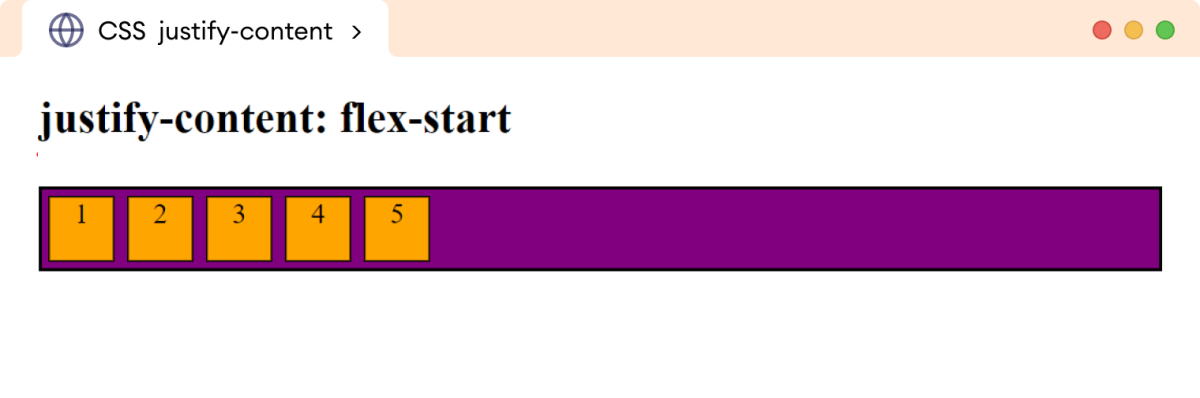
Values of CSS Justify-Content
The center
value aligns the flex items in the center of the main axis. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS justify-content</title>
</head>
<body>
<h2>justify-content: center</h2>
<div class="container">
<div class="box">1</div>
<div class="box">2</div>
<div class="box">3</div>
<div class="box">4</div>
<div class="box">5</div>
</div>
</body>
</html>
div.container {
display: flex;
justify-content: center;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 40px;
height: 40px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
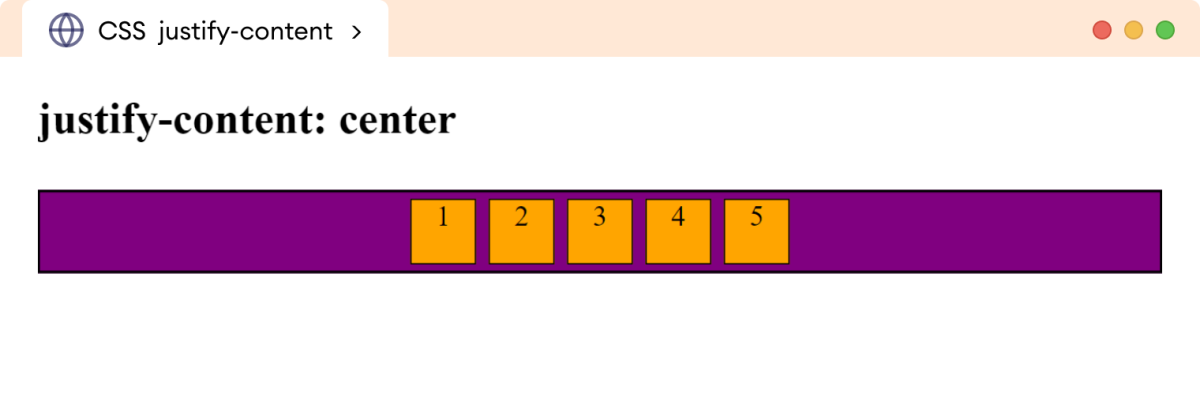
The flex-end
value aligns the flex items at the end of the main axis (right
for the flex-direction: row/row-reverse
and bottom
for the flex-direction: column/column-reverse
.)
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS justify-content</title>
</head>
<body>
<h2>justify-content: flex-end</h2>
<div class="container">
<div class="box">1</div>
<div class="box">2</div>
<div class="box">3</div>
<div class="box">4</div>
<div class="box">5</div>
</div>
</body>
</html>
div.container {
display: flex;
justify-content: flex-end;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 40px;
height: 40px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
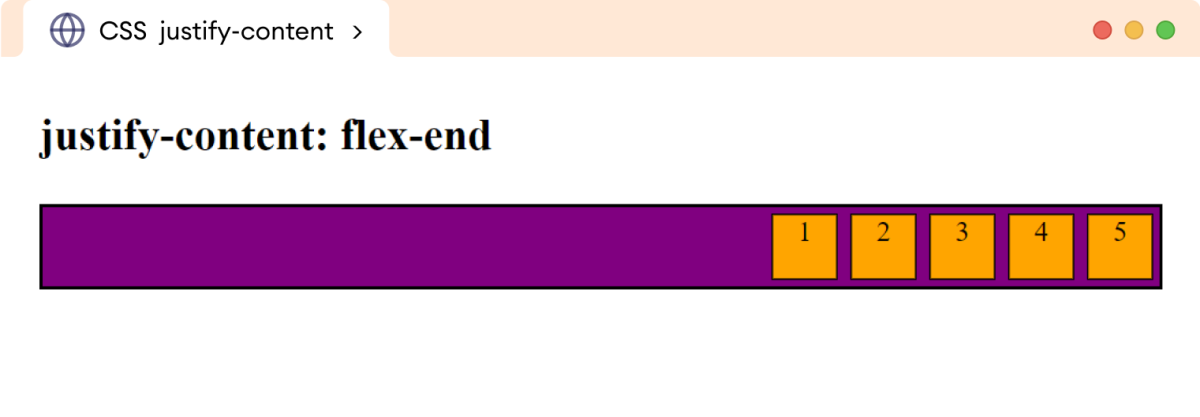
The space-between
value aligns the first item to the starting edge (main-start) and the last item to the ending edge (main-end) within a flex container. The remaining space is evenly divided between the flex items.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS justify-content</title>
</head>
<body>
<h2>justify-content: space-between</h2>
<div class="container">
<div class="box">1</div>
<div class="box">2</div>
<div class="box">3</div>
<div class="box">4</div>
<div class="box">5</div>
</div>
</body>
</html>
div.container {
display: flex;
justify-content: space-between;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 40px;
height: 40px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
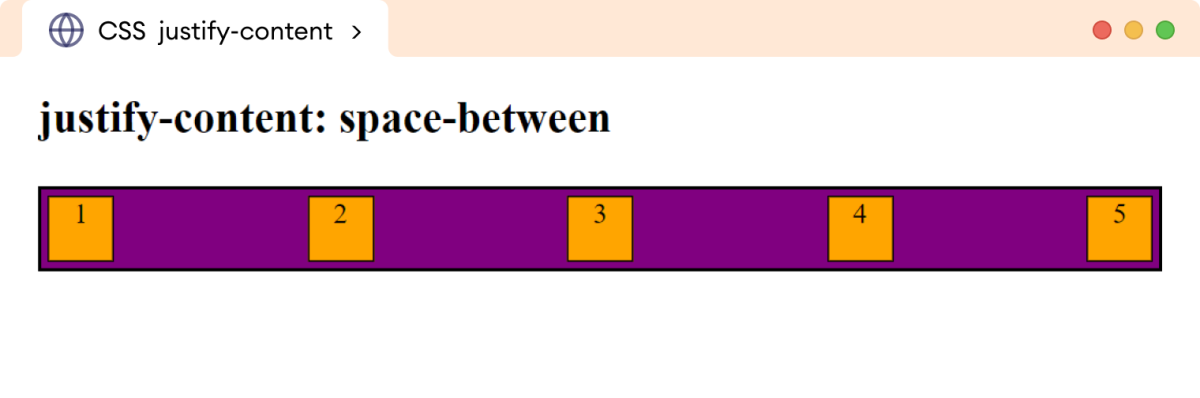
The space-around
value evenly divides the space between items. However, there is half of that space before the first and after the last item. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS justify-content</title>
</head>
<body>
<h2>justify-content: space-around</h2>
<div class="container">
<div class="box">1</div>
<div class="box">2</div>
<div class="box">3</div>
<div class="box">4</div>
<div class="box">5</div>
</div>
</body>
</html>
div.container {
display: flex;
justify-content: space-around;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 40px;
height: 40px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
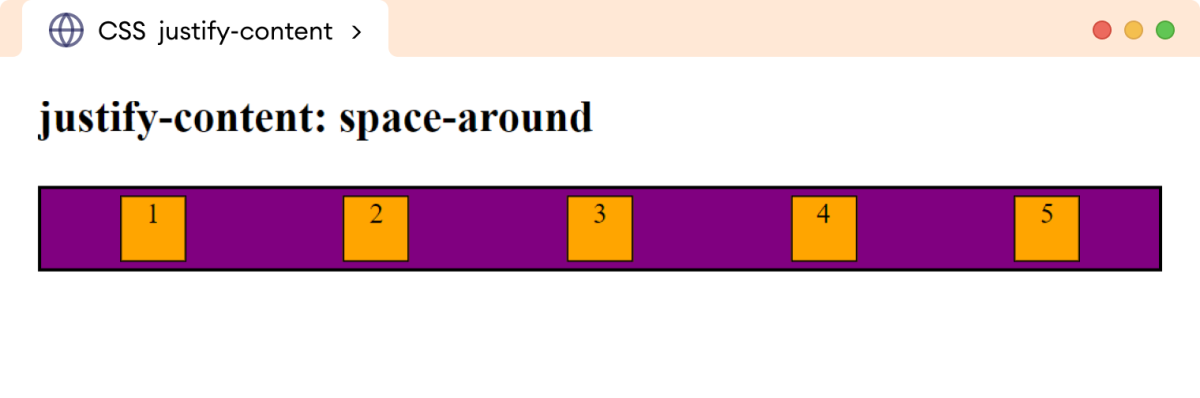
The space-evenly
value evenly divides the space between the flex items. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS justify-content</title>
</head>
<body>
<h2>justify-content: space-evenly</h2>
<div class="container">
<div class="box">1</div>
<div class="box">2</div>
<div class="box">3</div>
<div class="box">4</div>
<div class="box">5</div>
</div>
</body>
</html>
div.container {
display: flex;
justify-content: space-evenly;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 40px;
height: 40px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
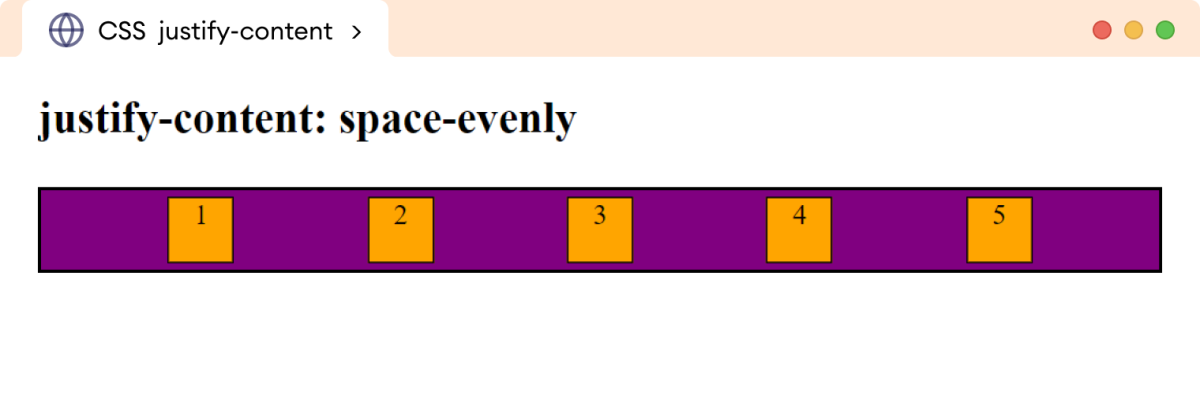
Align Items
The align-items
property distributes the available space between the flex items along the cross axis.
The space is distributed vertically for flex-direction: row
and horizontally for flex-direction: column
.
The possible values for the align-items
property are flex-start
(default value), center
, flex-end
, baseline
, and stretch
.
Let's look at each of these values.
align-items: flex-start
The flex-start
value aligns the flex items at the start of the cross-axis (top for the flex-direction: row/row-reverse
and left for the flex-direction: column/column-reverse
).
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS align-items</title>
</head>
<body>
<h2>align-items: flex-start</h2>
<div class="container">
<div class="box">1</div>
<div class="box">2</div>
<div class="box">3</div>
<div class="box">4</div>
<div class="box">5</div>
</div>
</body>
</html>
div.container {
height: 160px;
display: flex;
align-items: flex-start;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 40px;
height: 40px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
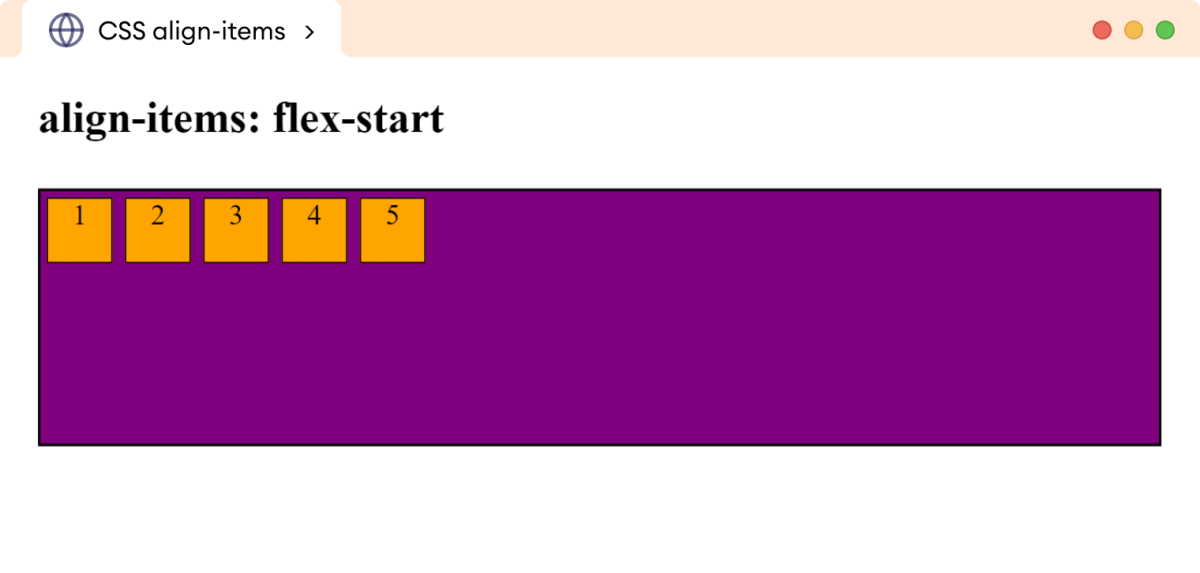
Note: It is important to set the height of the flex container while the flex-direction
is set to row
. Without the height, the flex items will not have any space to be aligned, and no effect will be visible.
Values of CSS Align-Items
The center
value aligns the flex items in the center of the cross-axis. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS align-items</title>
</head>
<body>
<h2>align-items: center</h2>
<div class="container">
<div class="box">1</div>
<div class="box">2</div>
<div class="box">3</div>
<div class="box">4</div>
<div class="box">5</div>
</div>
</body>
</html>
div.container {
height: 160px;
display: flex;
align-items: center;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 40px;
height: 40px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
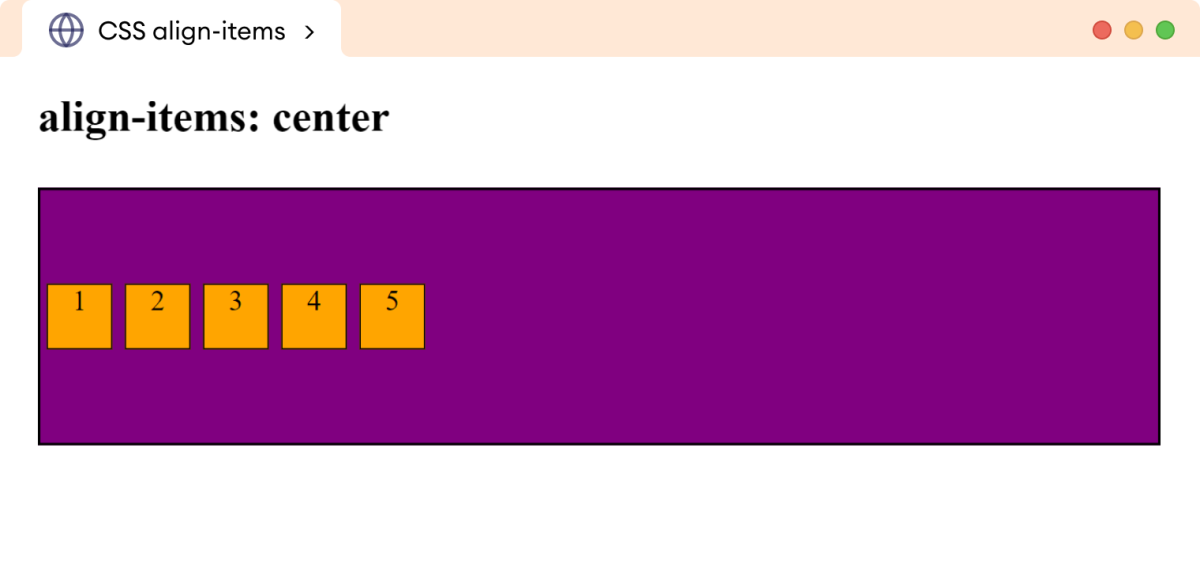
The flex-end
value aligns the flex items at the end of the cross axis. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS align-items</title>
</head>
<body>
<h2>align-items: flex-end</h2>
<div class="container">
<div class="box">1</div>
<div class="box">2</div>
<div class="box">3</div>
<div class="box">4</div>
<div class="box">5</div>
</div>
</body>
</html>
div.container {
height: 160px;
display: flex;
align-items: flex-end;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 40px;
height: 40px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
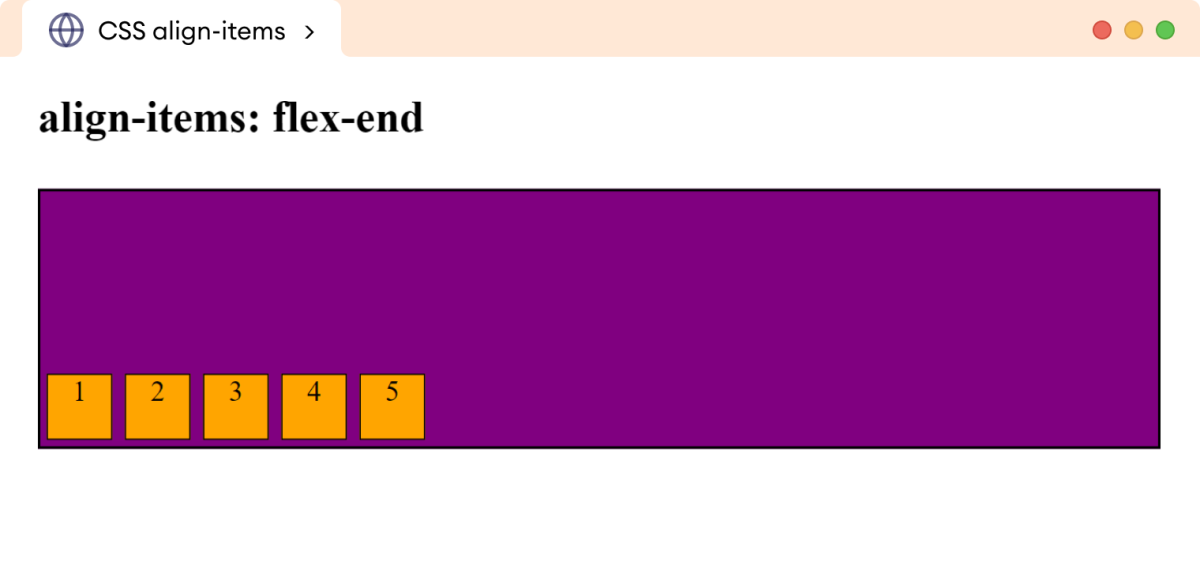
The baseline
value aligns the flex items along the baseline of items. This means that the text in all of the flex items will be aligned to the same line.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS align-items</title>
</head>
<body>
<h2>align-items: baseline</h2>
<div class="container">
<div class="box box1">1</div>
<div class="box box2">2</div>
<div class="box box3">3</div>
<div class="box box4">4</div>
<div class="box box5">5</div>
</div>
</body>
</html>
div.container {
height: 160px;
display: flex;
/* arranges the flex items with respective to baseline of flex items */
align-items: baseline;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 40px;
height: 40px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
div.box3 {
width: 90px;
height: 90px;
/* sets the line-height */
line-height: 70px;
background-color: skyblue;
}
div.box4 {
width: 100px;
height: 100px;
/* sets the line-height */
line-height: 140px;
background-color: greenyellow;
}
Browser Output
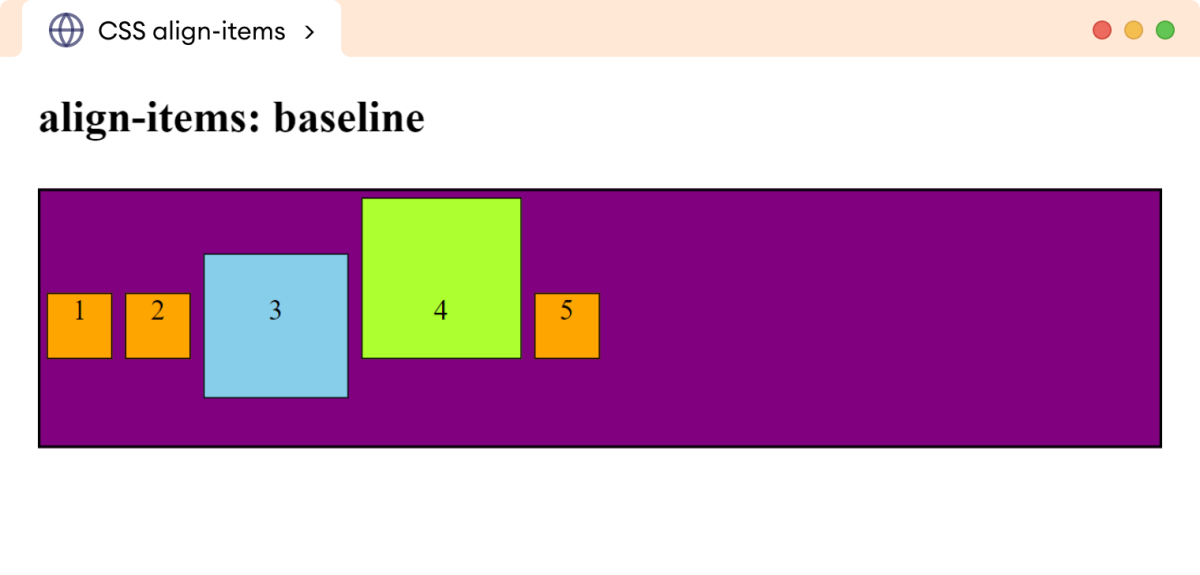
In the above example, the baseline of flex items is first aligned, and then the flex items are aligned on the same baseline.
Note: A baseline is an imaginary horizontal line upon which the base of most characters sits.
The stretch
value stretches the flex items to fill the container along the cross-axis. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS align-items</title>
</head>
<body>
<h2>align-items: stretch</h2>
<div class="container">
<div class="box box1">1</div>
<div class="box box2">2</div>
<div class="box box3">3</div>
<div class="box box4">4</div>
<div class="box box5">5</div>
</div>
</body>
</html>
div.container {
height: 160px;
display: flex;
/* stretches the flex items */
align-items: stretch;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 40px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
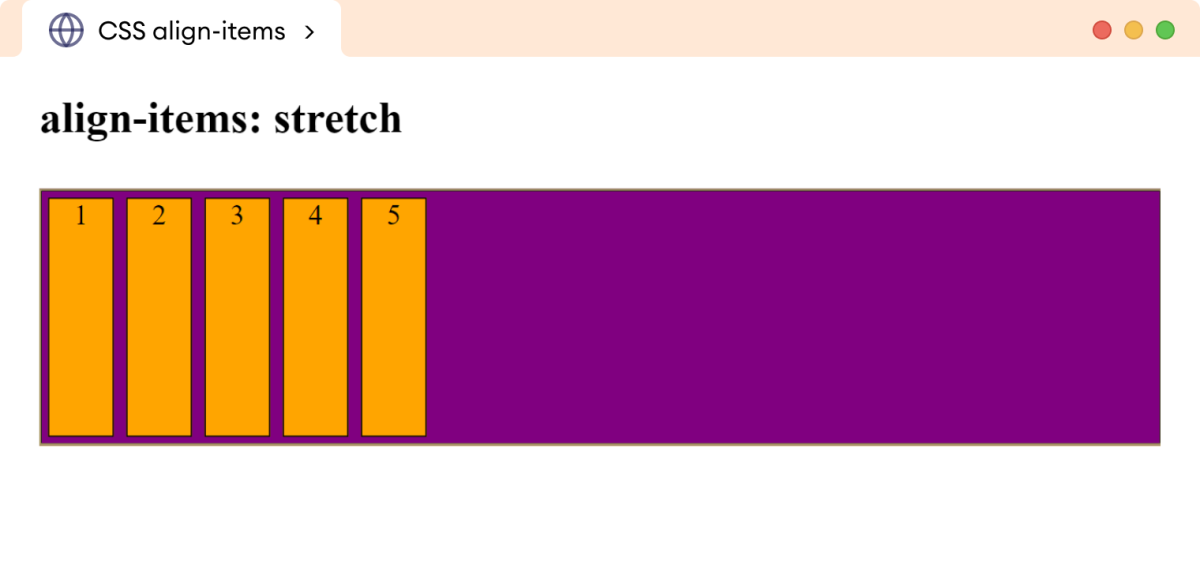
In the above example, the flex items are stretched to fill the container vertically.
Note that we haven't set the height
of the flex items. If we set the height to flex items, the align-items: stretch
doesn't work, and the flex items will maintain the specified height.
If a specific height is set on the flex items, the align-items: stretch
property won't have any effect on those items. The items will maintain their specified height, and stretching won't occur.
Align Content
The align-content
property controls the alignment of flex lines when there is extra space in the cross-axis.
The possible values of the align-content
property are normal
(default), flex-start
, flex-end
, center
, space-between
, space-around
, space-evenly
, and stretch
.
Let's look into each of these values individually.
align-content: flex-start
The flex-start
value aligns the flex lines at the start of the cross-axis (top for the flex-direction: row/row-reverse
and left for the flex-direction: column/column-reverse
.)
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS align-content</title>
</head>
<body>
<h2>align-content: flex-start</h2>
<div class="container">
<div class="box box1">1</div>
<div class="box box2">2</div>
<div class="box box3">3</div>
<div class="box box4">4</div>
<div class="box box5">5</div>
<div class="box box6">6</div>
<div class="box box7">7</div>
<div class="box box8">8</div>
<div class="box box9">9</div>
<div class="box box10">10</div>
<div class="box box11">11</div>
</div>
</body>
</html>
div.container {
height: 180px;
display: flex;
/* setting a flex-wrap value is important */
flex-wrap: wrap;
/* aligns the flex lines to the flex-start */
align-content: flex-start;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 80px;
height: 40px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
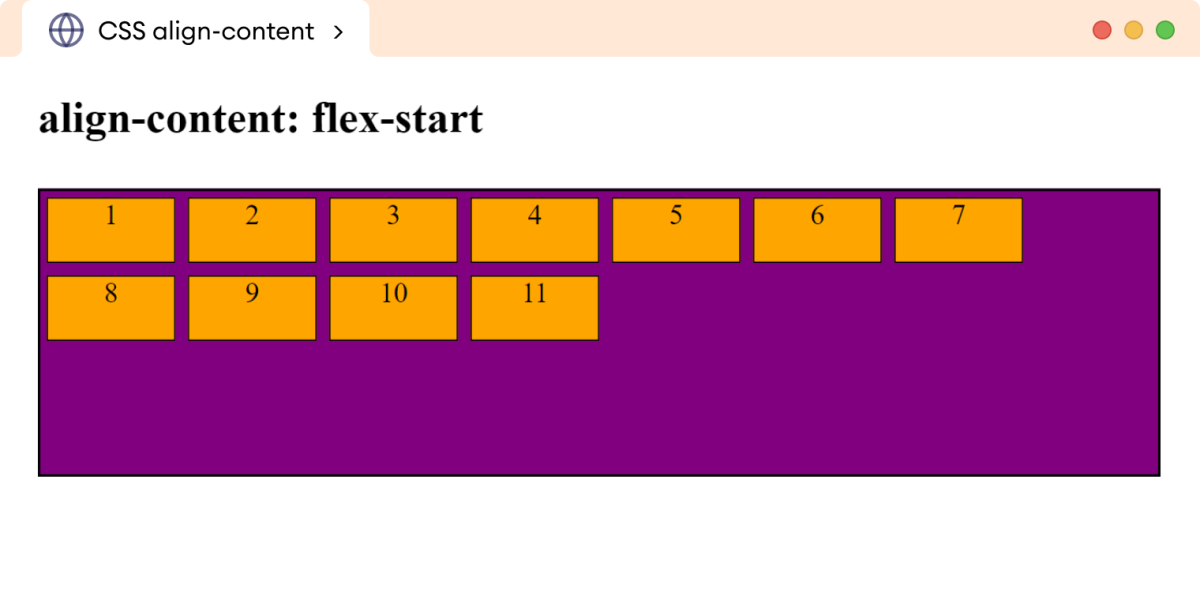
Note: The align-content
property only affects the alignment of flex items in a multi-line flex container. If the flex container is single-line (i.e., if the flex-wrap property is set to no-wrap), then the align-content
property will have no effect.
Values of CSS Align-Content
The center
value aligns the flex lines in the center of the flex container. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS align-content</title>
</head>
<body>
<h2>align-content: center</h2>
<div class="container">
<div class="box box1">1</div>
<div class="box box2">2</div>
<div class="box box3">3</div>
<div class="box box4">4</div>
<div class="box box5">5</div>
<div class="box box6">6</div>
<div class="box box7">7</div>
<div class="box box8">8</div>
<div class="box box9">9</div>
<div class="box box10">10</div>
<div class="box box11">11</div>
</div>
</body>
</html>
div.container {
height: 180px;
display: flex;
/* setting a flex-wrap value is important */
flex-wrap: wrap;
/* aligns the flex lines in the center*/
align-content: center;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 80px;
height: 40px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
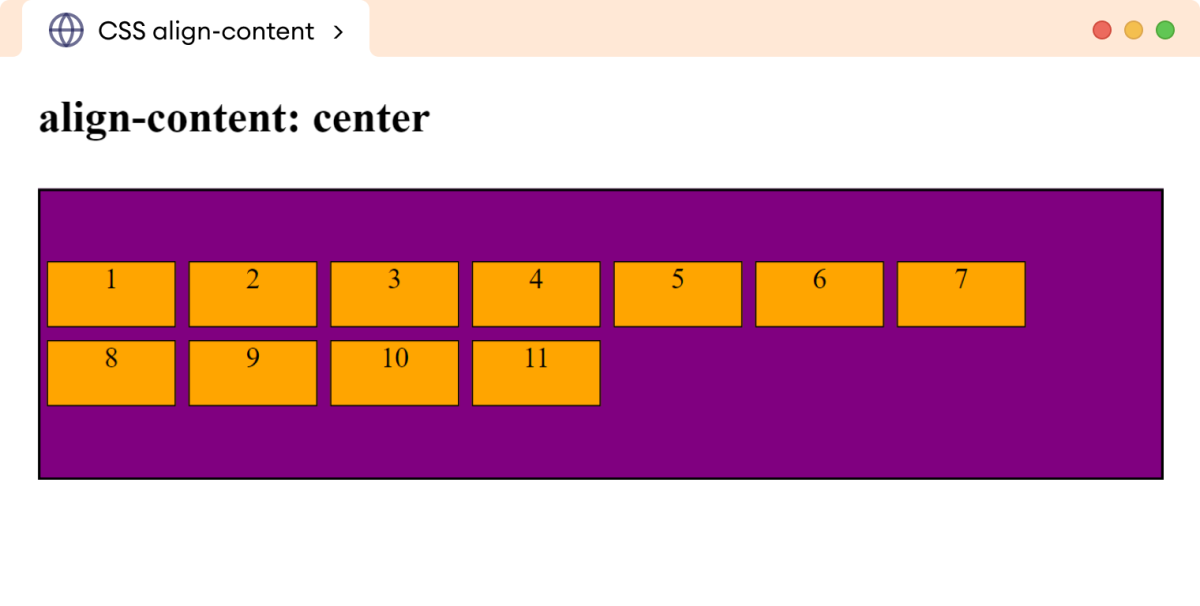
The flex-end
value aligns the flex items at the end of the cross-axis. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS align-content</title>
</head>
<body>
<h2>align-content: flex-end</h2>
<div class="container">
<div class="box box1">1</div>
<div class="box box2">2</div>
<div class="box box3">3</div>
<div class="box box4">4</div>
<div class="box box5">5</div>
<div class="box box6">6</div>
<div class="box box7">7</div>
<div class="box box8">8</div>
<div class="box box9">9</div>
<div class="box box10">10</div>
<div class="box box11">11</div>
</div>
</body>
</html>
div.container {
height: 180px;
display: flex;
/* setting a flex-wrap value is important */
flex-wrap: wrap;
/* aligns the flex lines in the end*/
align-content: flex-end;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 80px;
height: 40px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
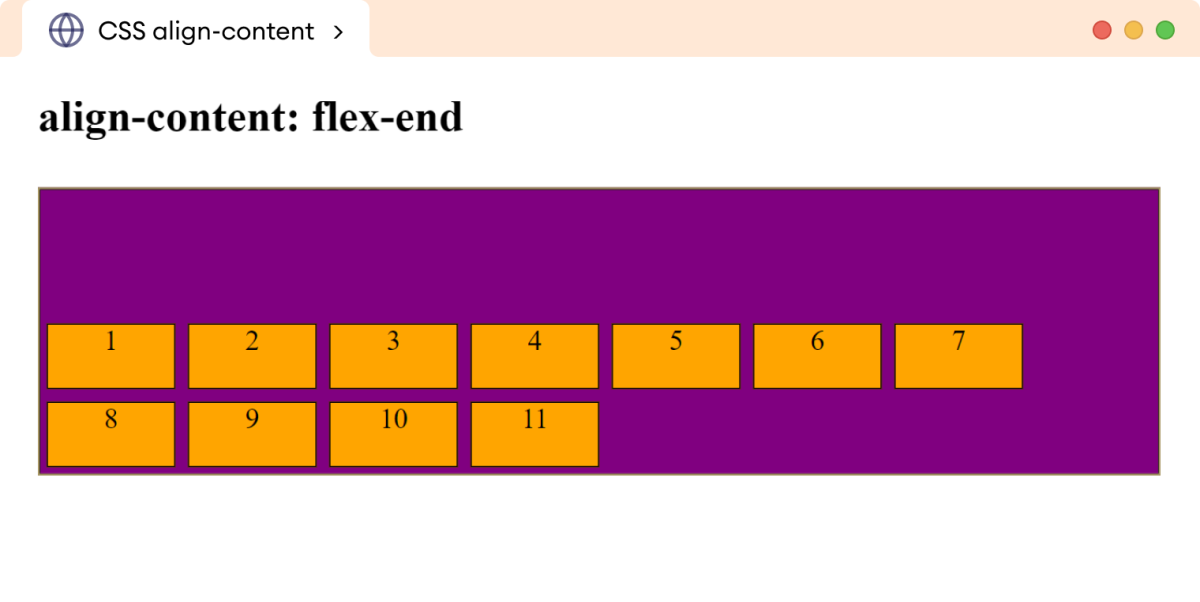
The space-between
value distributes space evenly between the lines of the flex container. The first line starts at the starting edge, and the last line ends at the ending edge.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS align-content</title>
</head>
<body>
<h2>align-content: space-between</h2>
<div class="container">
<div class="box box1">1</div>
<div class="box box2">2</div>
<div class="box box3">3</div>
<div class="box box4">4</div>
<div class="box box5">5</div>
<div class="box box6">6</div>
<div class="box box7">7</div>
<div class="box box8">8</div>
<div class="box box9">9</div>
<div class="box box10">10</div>
<div class="box box11">11</div>
</div>
</body>
</html>
div.container {
height: 180px;
display: flex;
/* setting a flex-wrap value is important */
flex-wrap: wrap;
/* aligns the flex lines in the end*/
align-content: space-between;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 80px;
height: 40px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
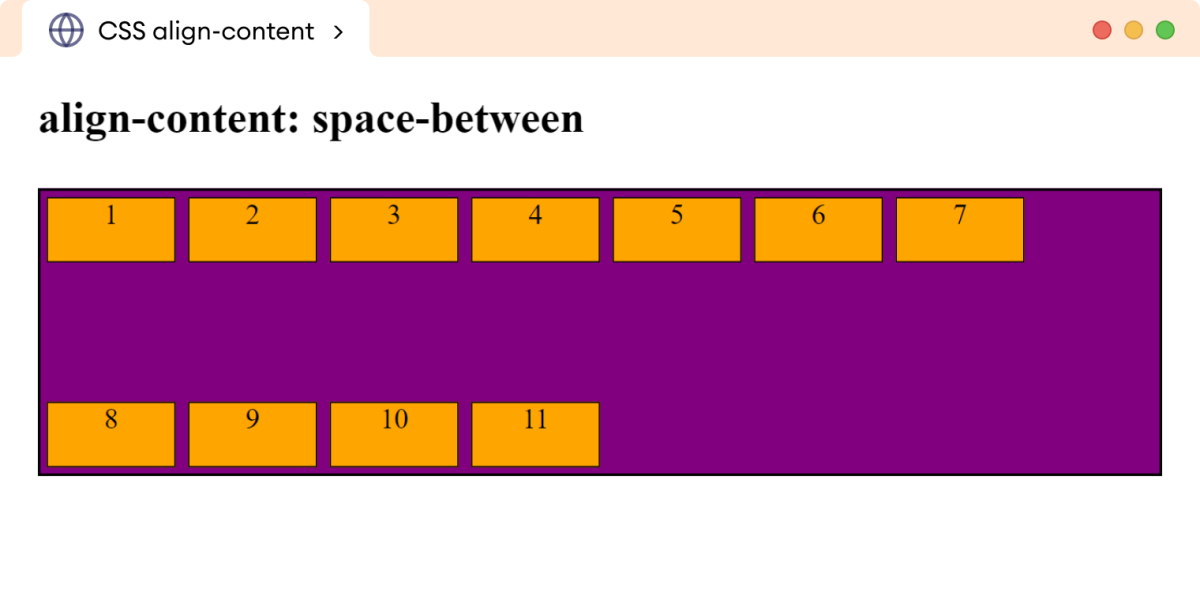
The space-around
value distributes the space evenly between the lines of flex items. However, there is half of that space before the first and after the last flex line.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS align-content</title>
</head>
<body>
<h2>align-content: space-around</h2>
<div class="container">
<div class="box box1">1</div>
<div class="box box2">2</div>
<div class="box box3">3</div>
<div class="box box4">4</div>
<div class="box box5">5</div>
<div class="box box6">6</div>
<div class="box box7">7</div>
<div class="box box8">8</div>
<div class="box box9">9</div>
<div class="box box10">10</div>
<div class="box box11">11</div>
</div>
</body>
</html>
div.container {
height: 180px;
display: flex;
/* setting a flex-wrap value is important */
flex-wrap: wrap;
align-content: space-around;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 80px;
height: 40px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
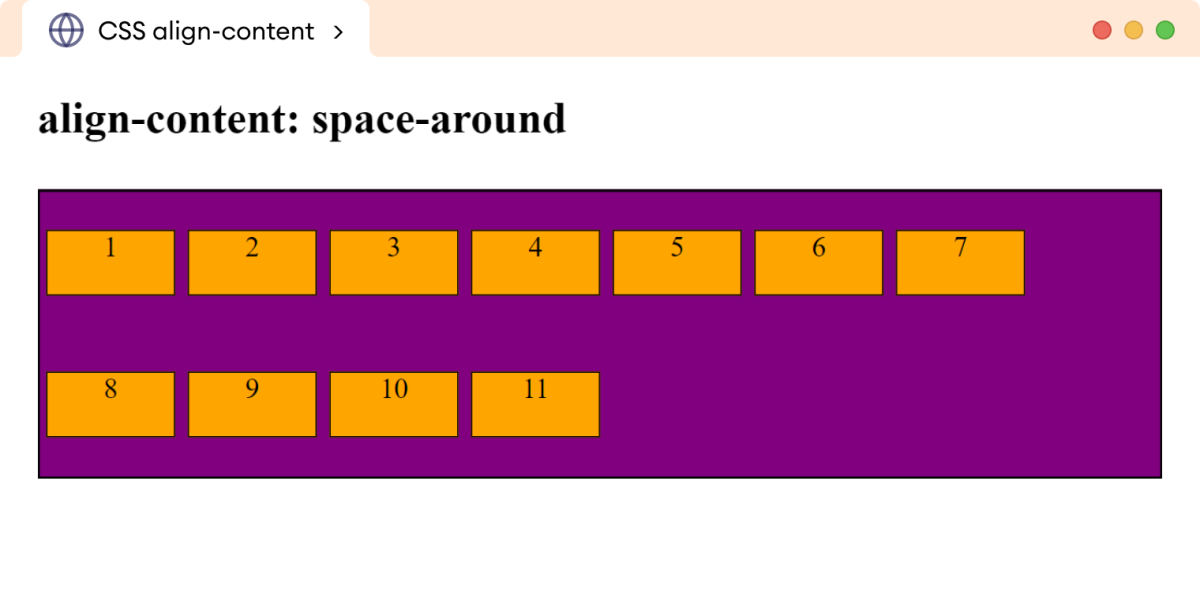
The space-evenly
value distributes space evenly around and between the flex lines. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS align-content</title>
</head>
<body>
<h2>align-content: space-evenly</h2>
<div class="container">
<div class="box box1">1</div>
<div class="box box2">2</div>
<div class="box box3">3</div>
<div class="box box4">4</div>
<div class="box box5">5</div>
<div class="box box6">6</div>
<div class="box box7">7</div>
<div class="box box8">8</div>
<div class="box box9">9</div>
<div class="box box10">10</div>
<div class="box box11">11</div>
</div>
</body>
</html>
div.container {
height: 180px;
display: flex;
/* setting a flex-wrap value is important */
flex-wrap: wrap;
align-content: space-evenly;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 80px;
height: 40px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
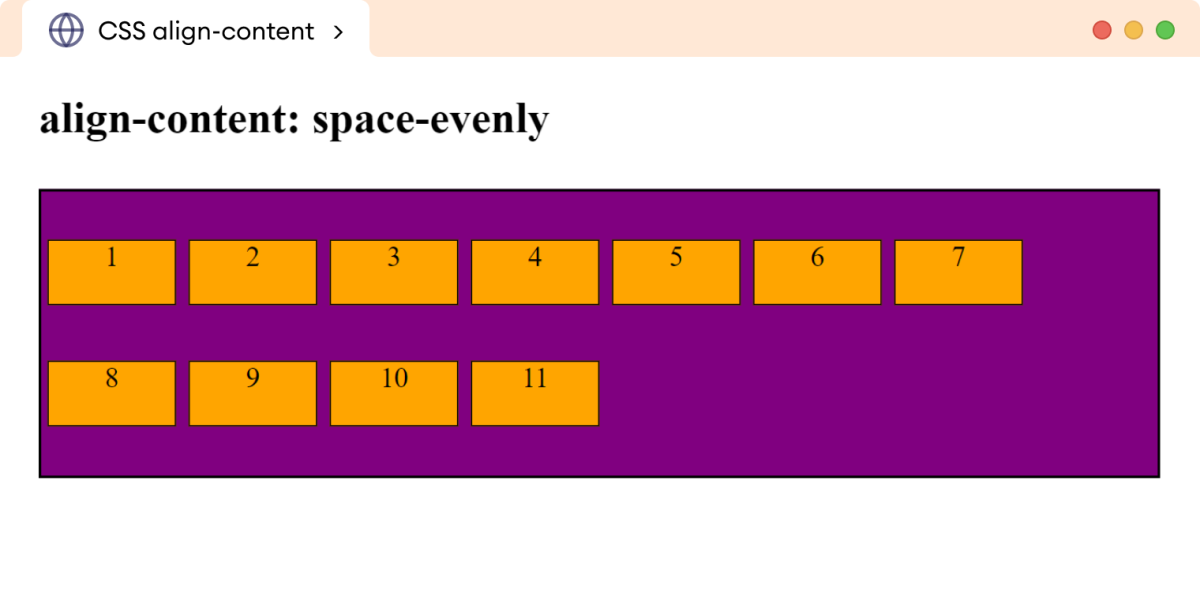
The stretch
value stretches the flex lines to fill the container along the cross-axis. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS align-content</title>
</head>
<body>
<h2>align-content: stretch</h2>
<div class="container">
<div class="box box1">1</div>
<div class="box box2">2</div>
<div class="box box3">3</div>
<div class="box box4">4</div>
<div class="box box5">5</div>
<div class="box box6">6</div>
<div class="box box7">7</div>
<div class="box box8">8</div>
<div class="box box9">9</div>
<div class="box box10">10</div>
<div class="box box11">11</div>
</div>
</body>
</html>
div.container {
height: 180px;
display: flex;
/* setting a flex-wrap value is important */
flex-wrap: wrap;
align-content: stretch;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 80px;
text-align: center;
border: 1px solid black;
background-color: orange;
margin: 4px;
}
Browser Output
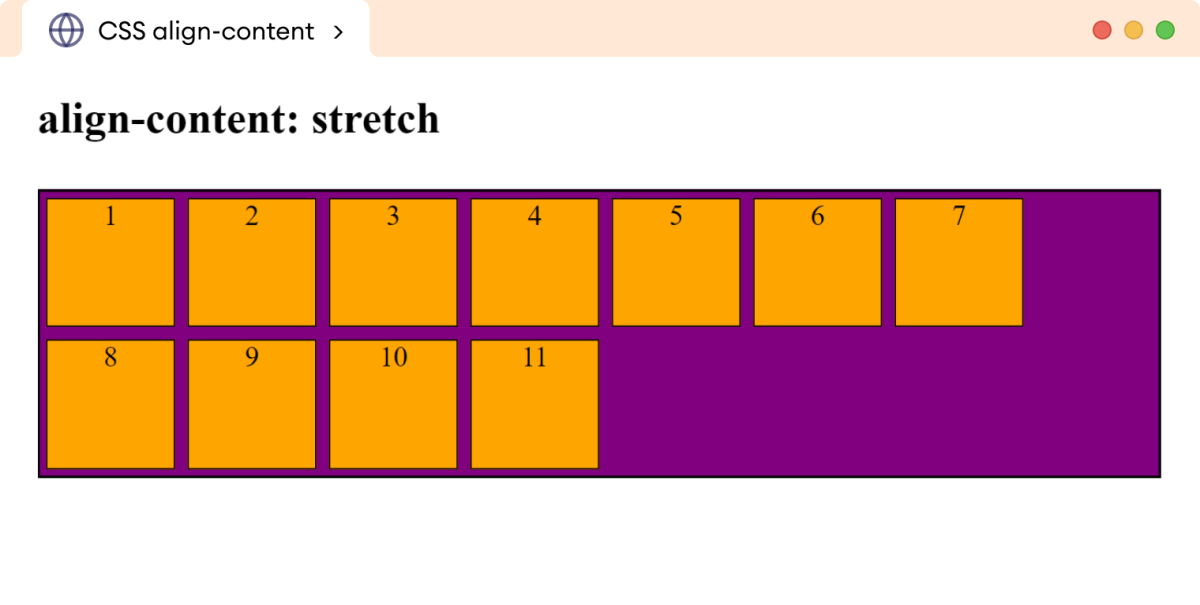
In the above example, the flex lines are stretched to fill the container vertically.
Note that we haven't set the height
to flex items.
If a specific height is set on the flex items, the align-content: stretch
property won't have any effect on those items. The flex lines will maintain their specified flex item height, and stretching won't occur.
Gap Between Flex Items
The row-gap
and column-gap
properties control the spacing between the flex items within the flex container.
Let's look at each of these individually.
CSS row-gap Property
The row-gap
property sets the gap between rows of flex items across the cross-axis. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS row-gap</title>
</head>
<body>
<h2>row-gap: 20px</h2>
<div class="container">
<div class="box box1">1</div>
<div class="box box2">2</div>
<div class="box box3">3</div>
<div class="box box4">4</div>
<div class="box box5">5</div>
<div class="box box6">6</div>
<div class="box box7">7</div>
<div class="box box8">8</div>
<div class="box box9">9</div>
<div class="box box10">10</div>
<div class="box box11">11</div>
</div>
</body>
</html>
div.container {
height: 180px;
display: flex;
flex-wrap: wrap;
/* creates a gap of 20px */
row-gap: 20px;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 70px;
height: 70px;
text-align: center;
border: 1px solid black;
background-color: orange;
}
Browser Output
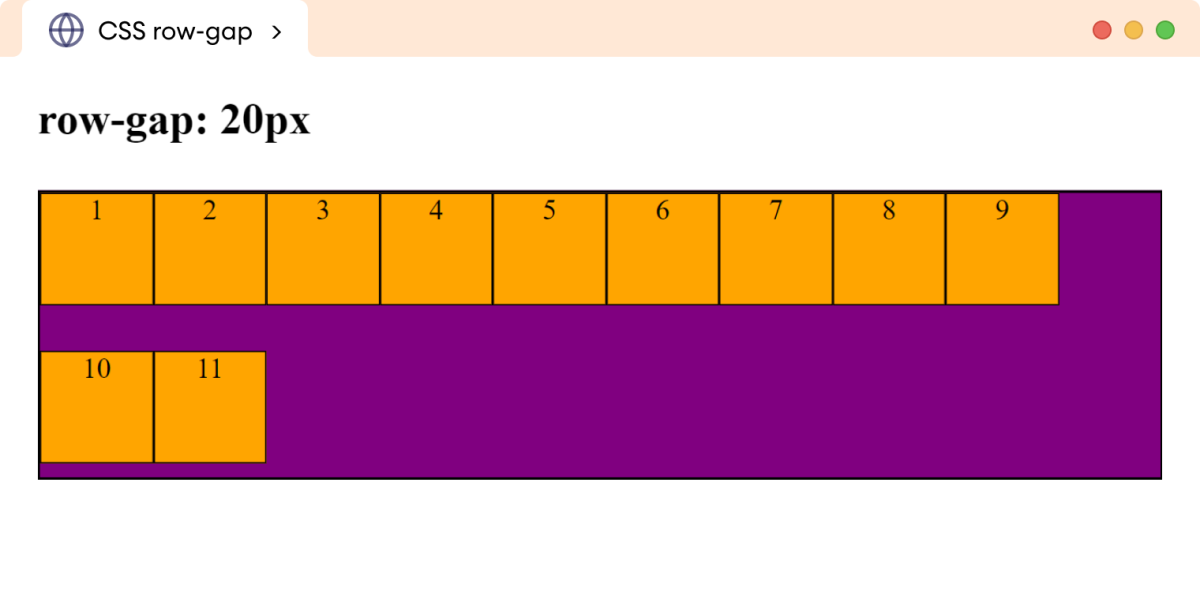
CSS column-gap Property
The column-gap
property sets the gap between columns of flex items across the main axis. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS column-gap</title>
</head>
<body>
<h2>column-gap: 20px</h2>
<div class="container">
<div class="box box1">1</div>
<div class="box box2">2</div>
<div class="box box3">3</div>
<div class="box box4">4</div>
<div class="box box5">5</div>
<div class="box box6">6</div>
<div class="box box7">7</div>
<div class="box box8">8</div>
<div class="box box9">9</div>
<div class="box box10">10</div>
<div class="box box11">11</div>
</div>
</body>
</html>
div.container {
height: 180px;
display: flex;
flex-wrap: wrap;
/* creates a gap of 20px */
column-gap: 20px;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 70px;
height: 70px;
text-align: center;
border: 1px solid black;
background-color: orange;
}
Browser Output
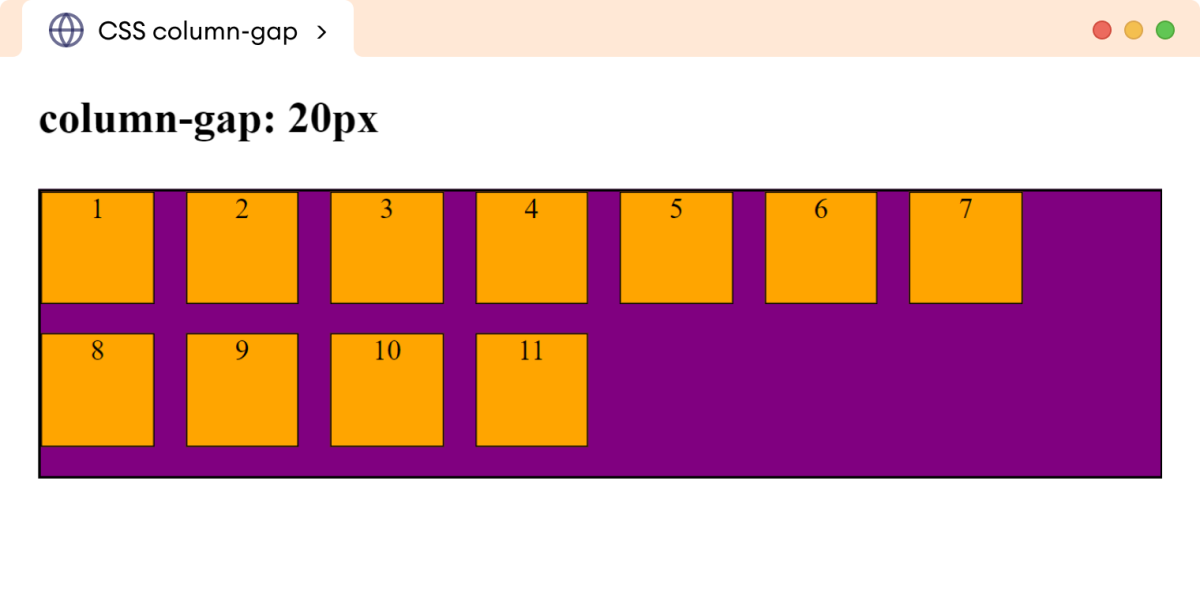
CSS gap Property
The gap
is the shorthand property that allows setting both row and column gaps between the flex items. For example,
gap: 20px; //creates a gap of 20px between rows and columns
gap: 10px 20px; //creates a row gap of 10px and a column gap of 20px
Let's look at a complete example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS gap</title>
</head>
<body>
<h2>gap: 40px</h2>
<div class="container">
<div class="box box1">1</div>
<div class="box box2">2</div>
<div class="box box3">3</div>
<div class="box box4">4</div>
<div class="box box5">5</div>
<div class="box box6">6</div>
<div class="box box7">7</div>
<div class="box box8">8</div>
<div class="box box9">9</div>
<div class="box box10">10</div>
<div class="box box11">11</div>
</div>
</body>
</html>
div.container {
height: 180px;
display: flex;
flex-wrap: wrap;
/* creates 40px gap between rows and columns */
gap: 40px;
border: 2px solid black;
background-color: purple;
}
div.box {
width: 60px;
height: 60px;
text-align: center;
border: 1px solid black;
background-color: orange;
}
Browser Output
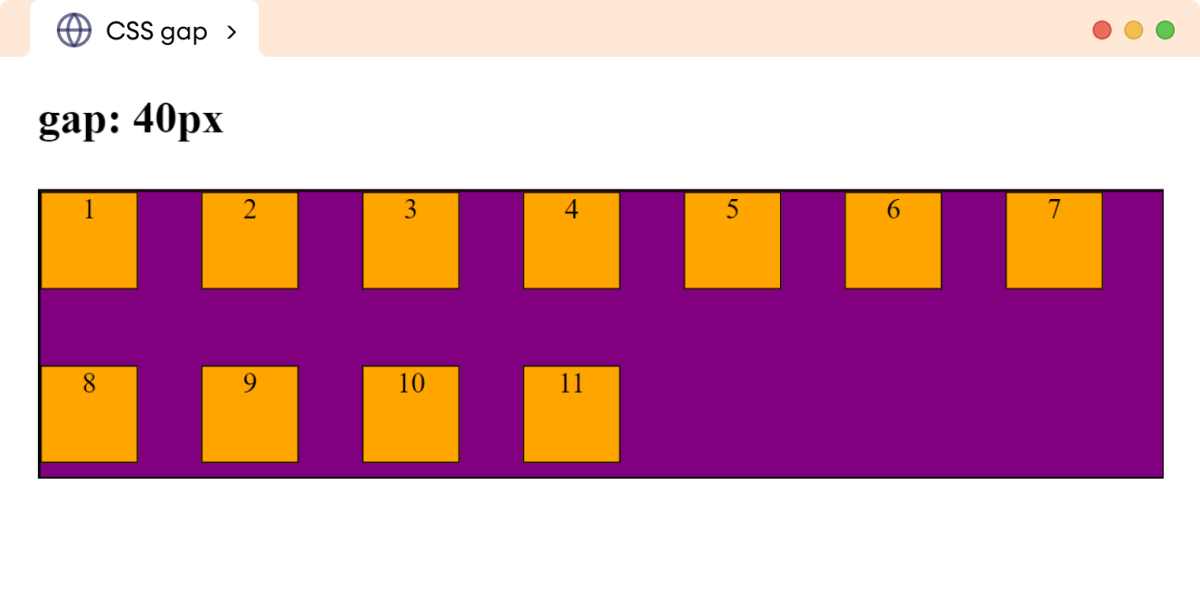