CSS Grid provides an efficient method for creating responsive and dynamic layouts that adapt to different screen sizes and devices.
This layout system is built upon flexible rows and columns.
Responsive Image Gallery Using CSS Grid
CSS grid properties can be used to create a responsive image gallery that adapts to different screen sizes.
We can use four-column layouts for desktop and laptop displays, a two-column layout for tablets, and finally, a single-column layout for mobile devices.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Grid Gallery</title>
</head>
<body>
<div class="gallery">
<img class="four-grid-cells"
src="https://images.pexels.com/photos/3408744/pexels-photo-3408744.jpeg"
alt="Northern Winter Sky Image" />
<img
src="https://images.pexels.com/photos/1142950/pexels-photo-1142950.jpeg"
alt="Shining Stars Image" />
<img class="wide-image"
src="https://images.pexels.com/photos/3933881/pexels-photo-3933881.jpeg"
alt="A River Flowing Image" />
<img
src="https://images.pexels.com/photos/5409751/pexels-photo-5409751.jpeg"
alt="A cloudy Mountain Image" />
<img
src="https://images.pexels.com/photos/4101555/pexels-photo-4101555.jpeg"
alt="A Winter Rainbow Image" />
<img class="wide-image"
src="https://images.pexels.com/photos/443446/pexels-photo-443446.jpeg"
alt="A Clean Mountain Lake" />
<img
src="https://images.pexels.com/photos/1142950/pexels-photo-1142950.jpeg"
alt="Shining Stars Image" />
</div>
</body>
</html>
.gallery {
display: grid;
grid-template-columns: repeat(4, 1fr);
grid-auto-rows: auto;
grid-auto-flow: dense;
gap: 6px;
}
img {
width: 100%;
height: 100%;
object-fit: cover;
}
img.four-grid-cells {
grid-row: span 2 / auto;
grid-column: span 2 / auto;
}
img.wide-image {
grid-column: span 2 / auto;
}
Browser Output
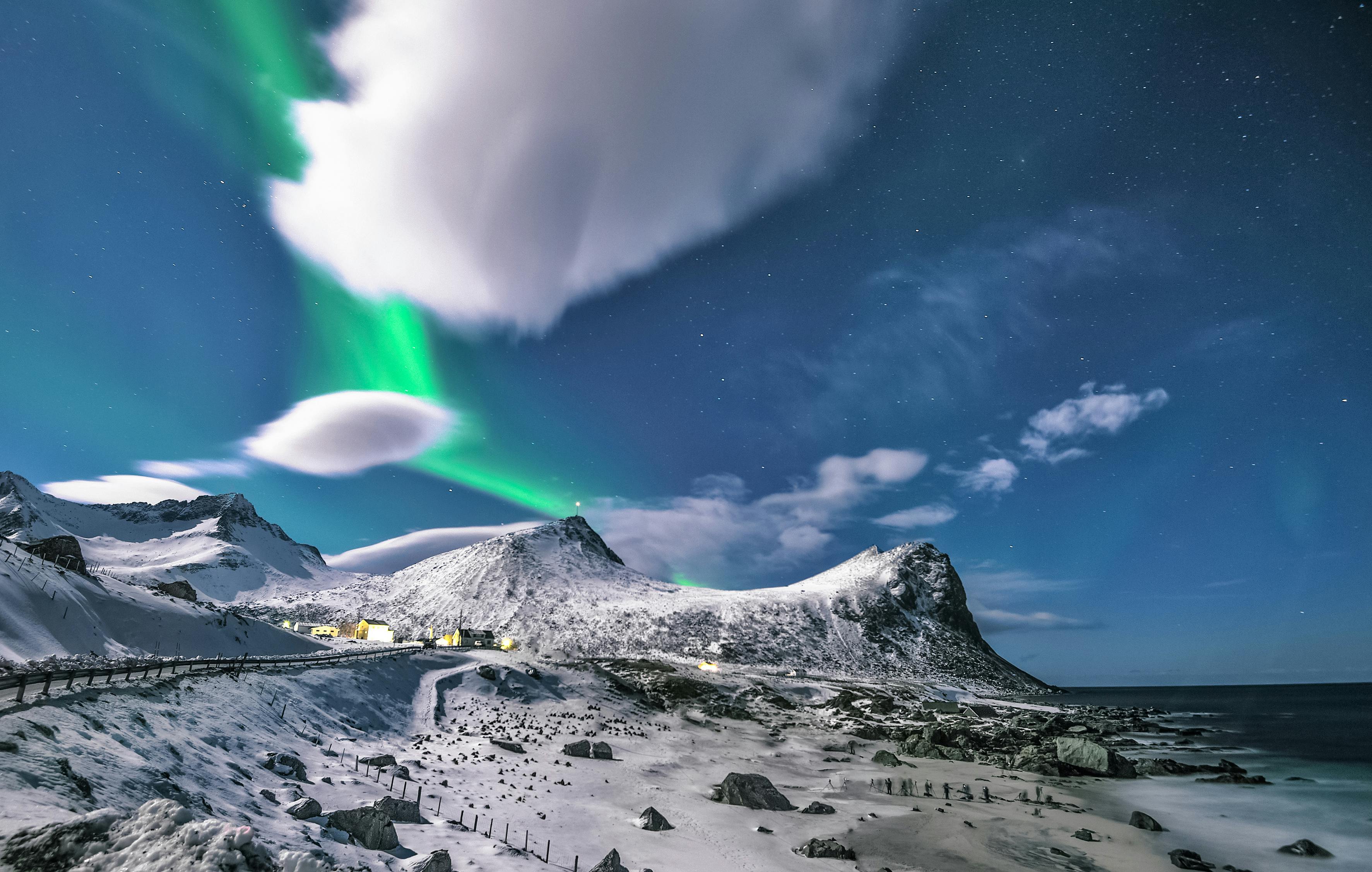
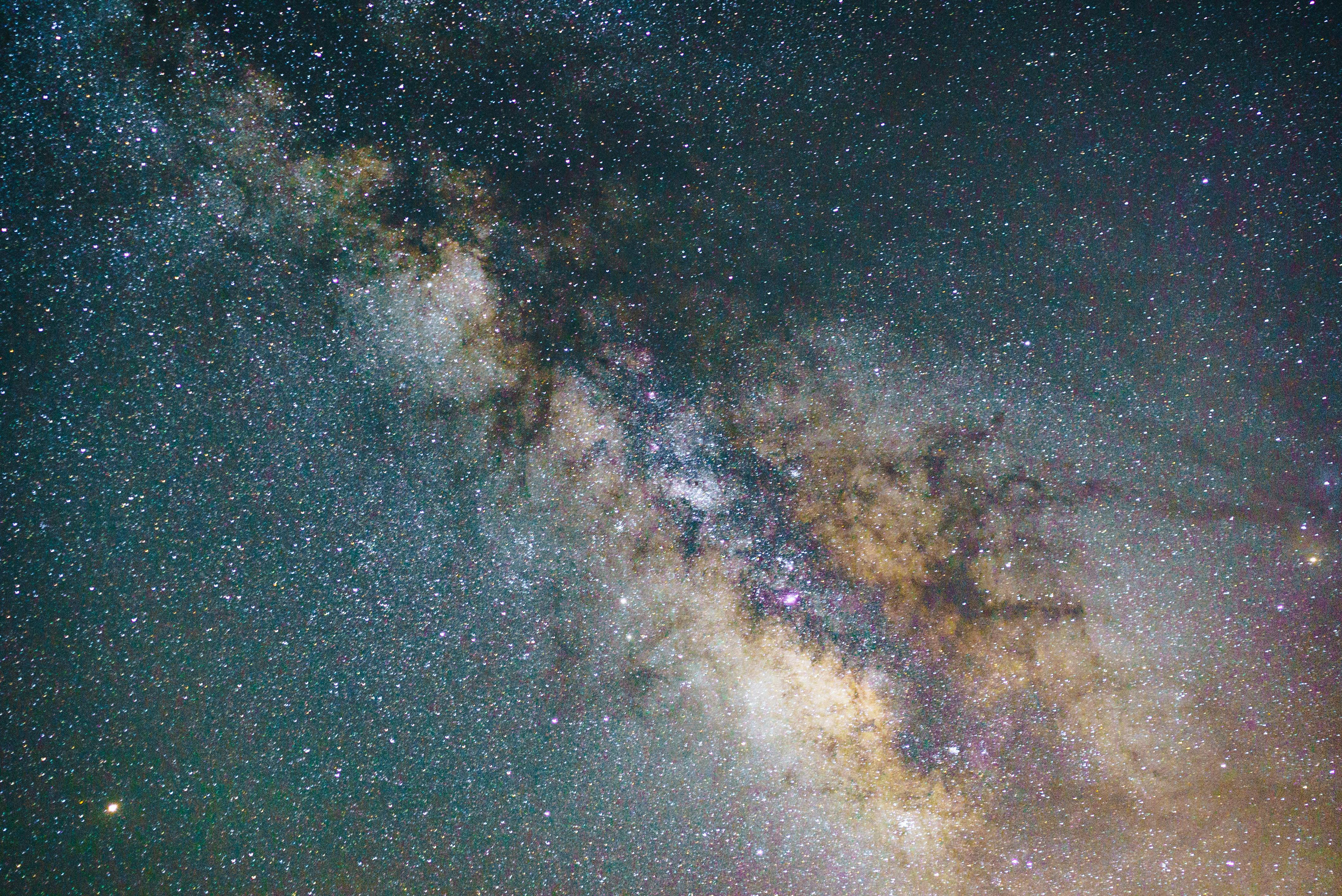
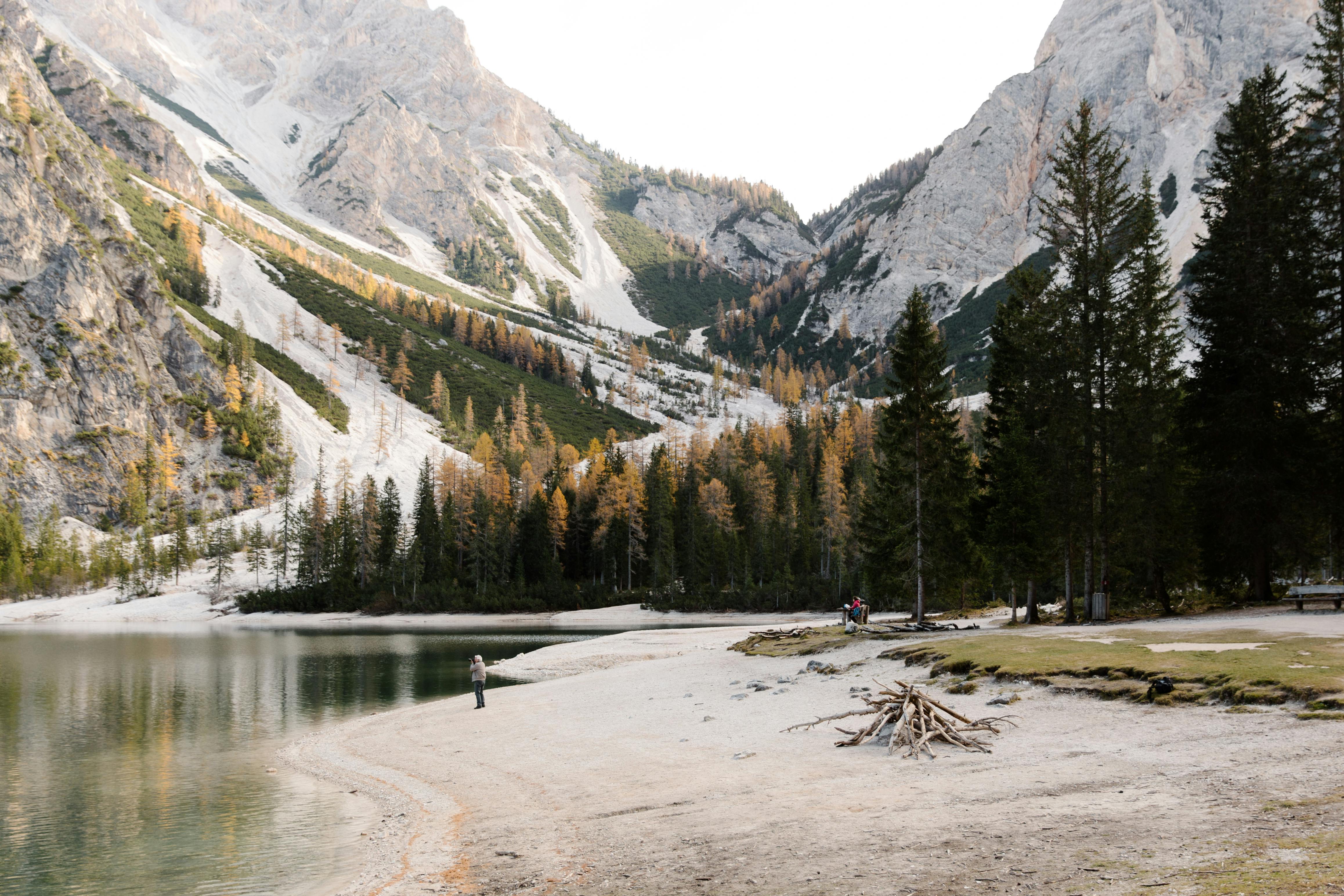
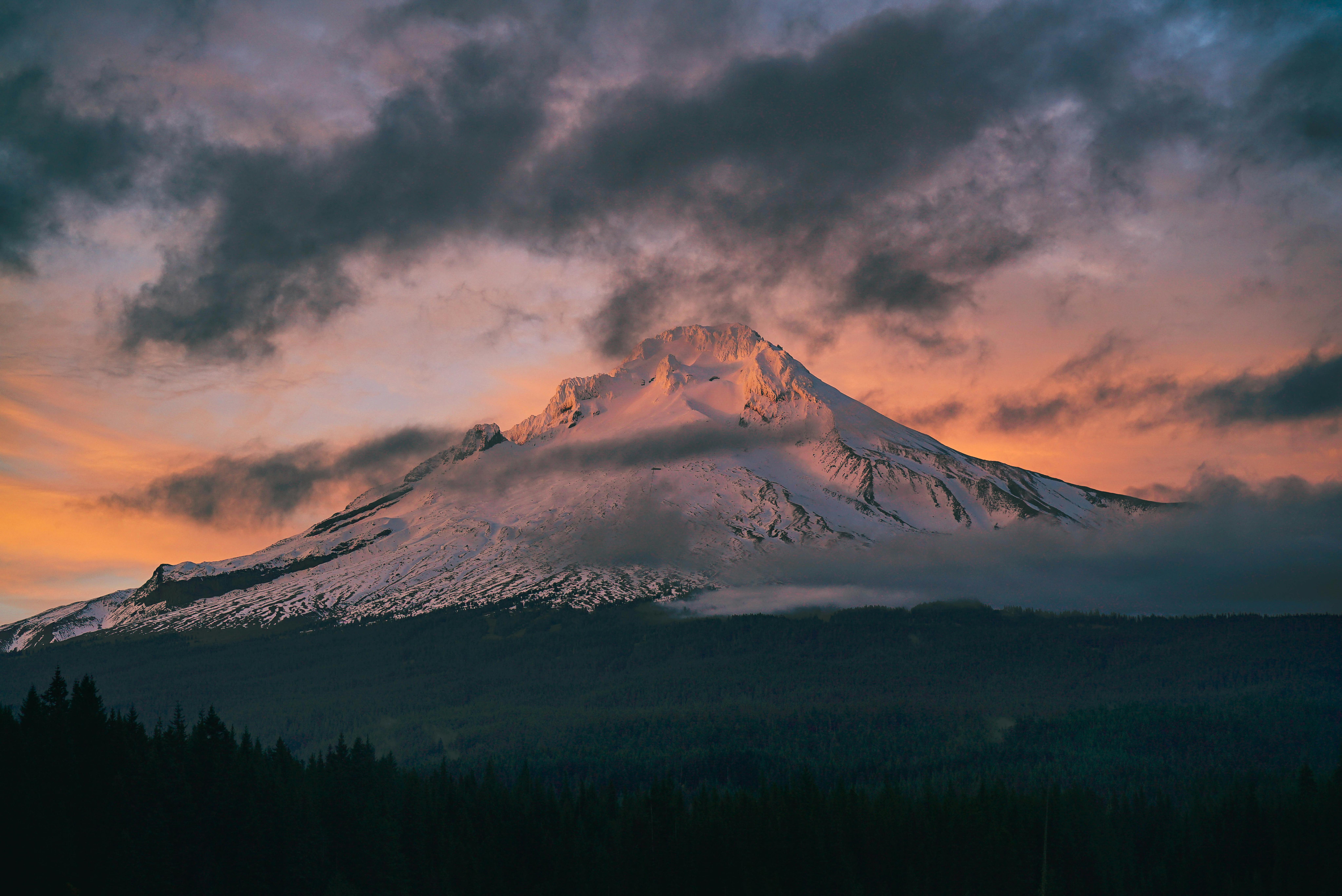
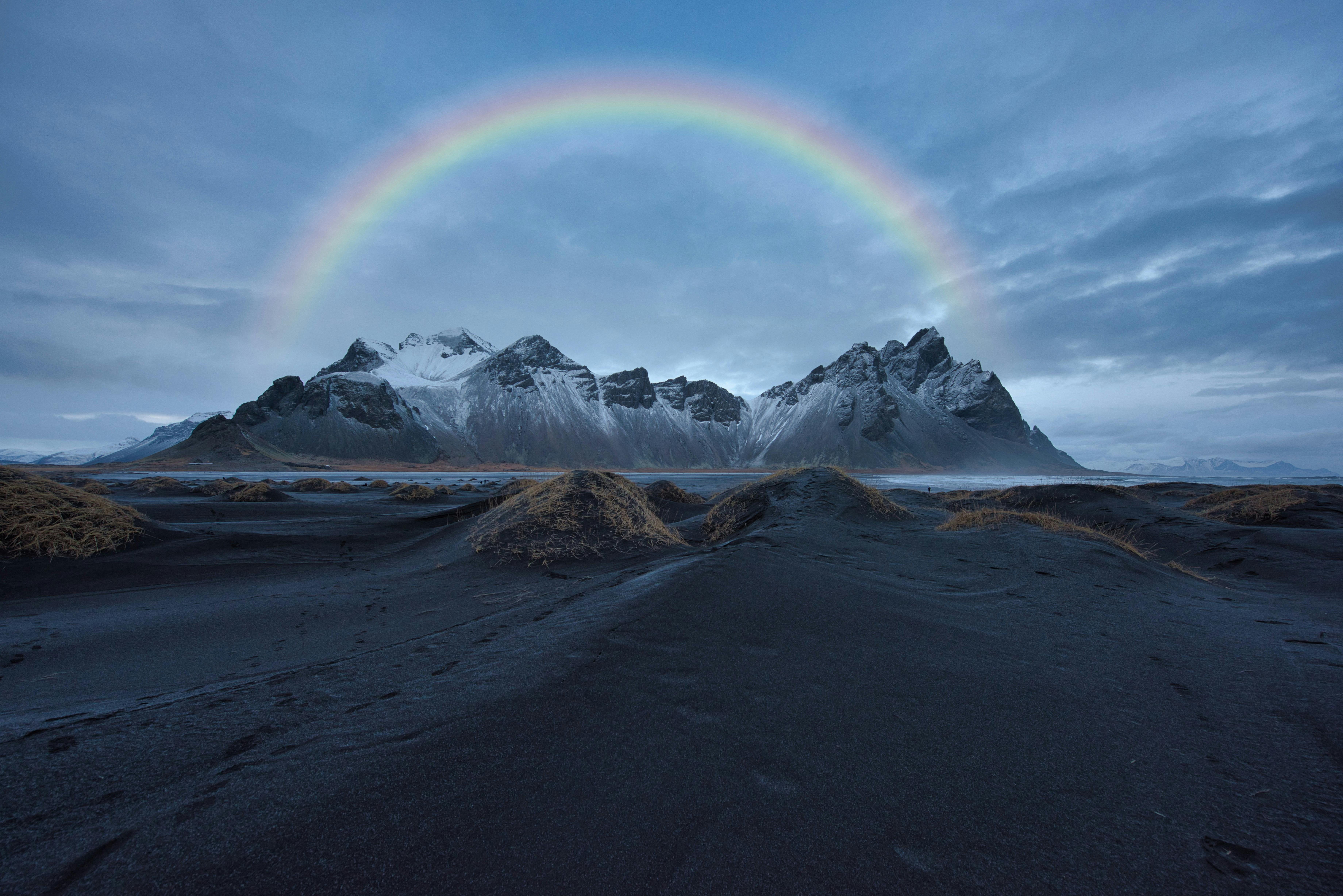
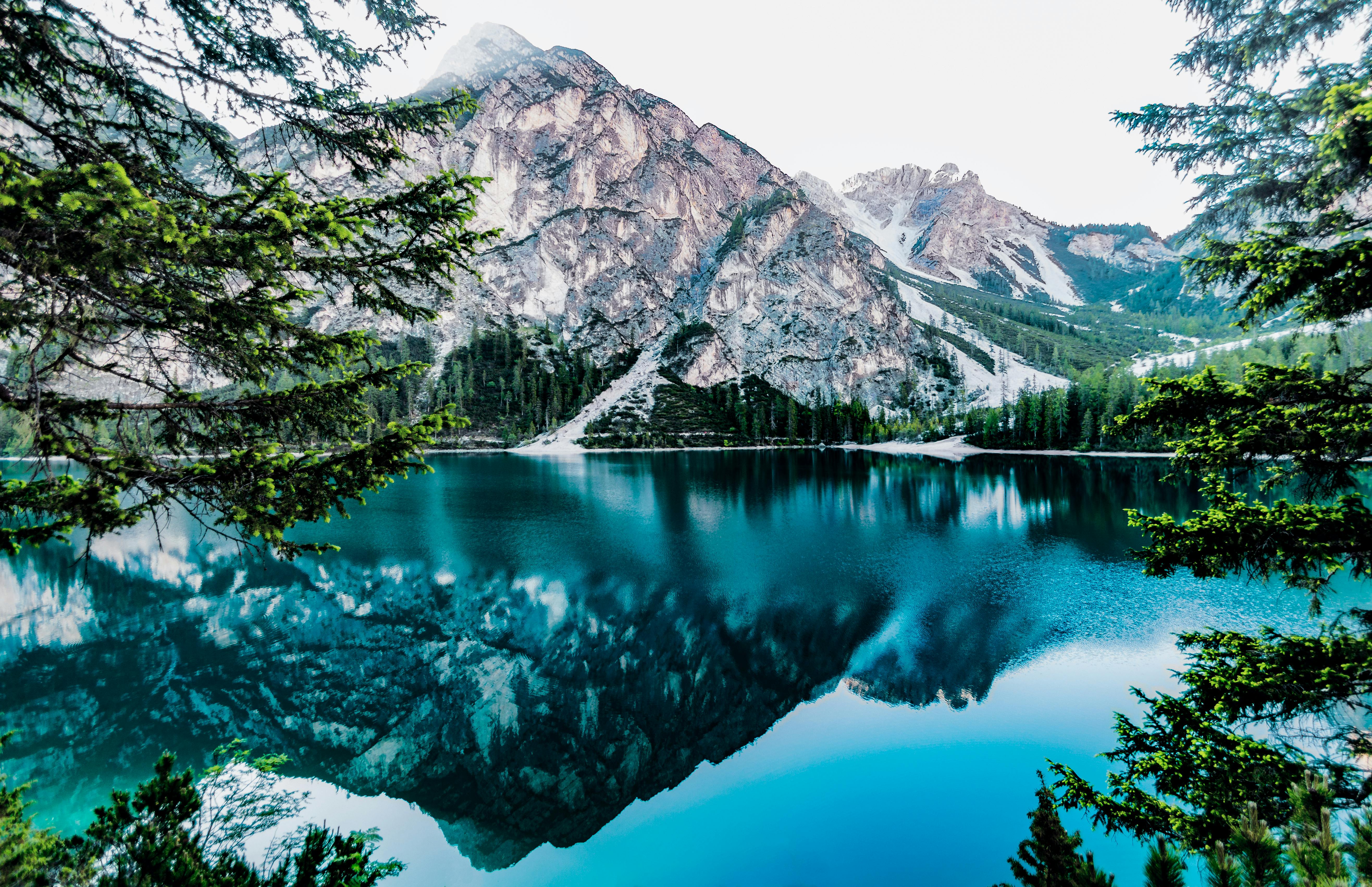
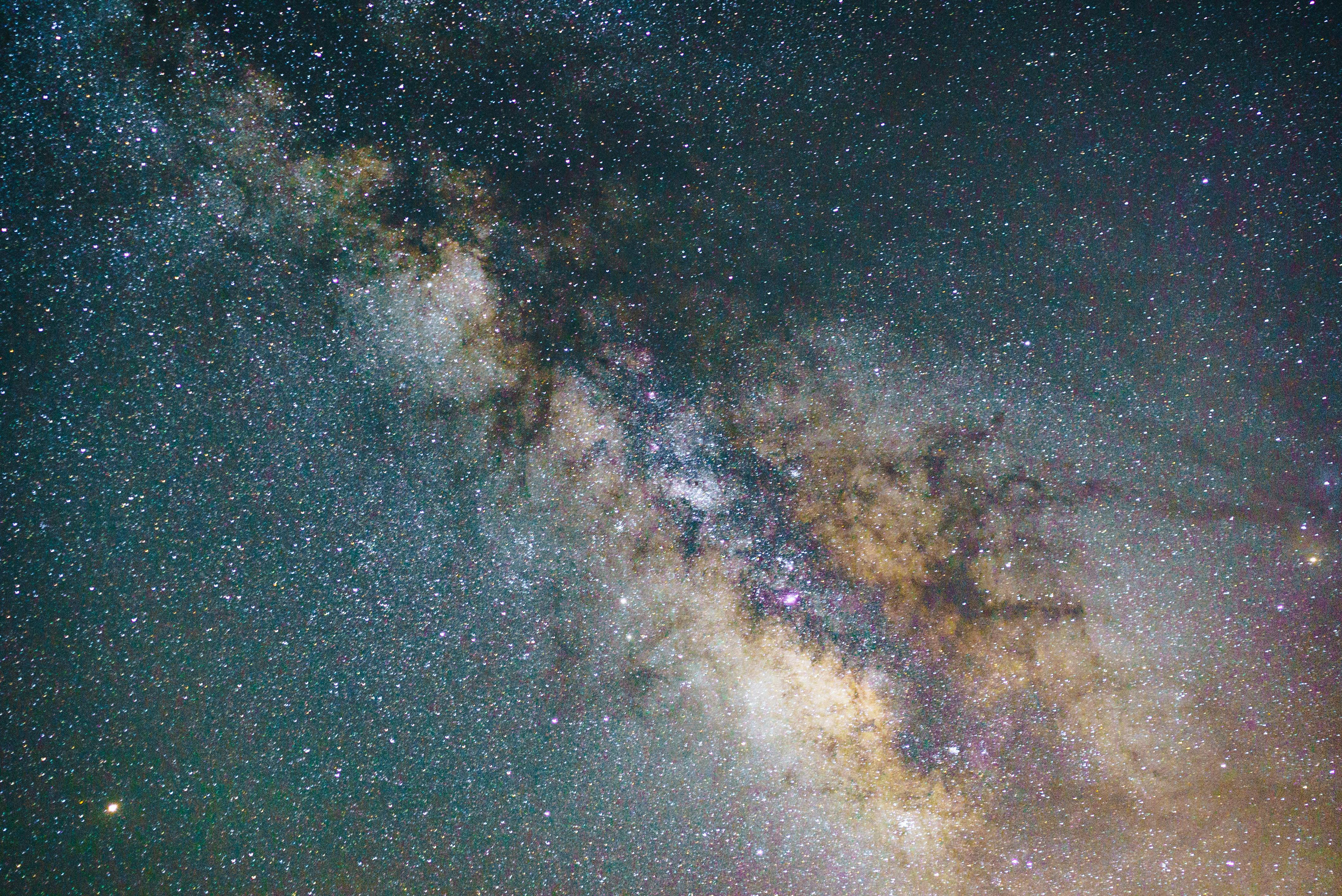
In the above example,
-
grid-template-columns: repeat(4, 1fr)
creates a four-column grid layout with equal-width columns
-
grid-auto-rows: auto
implicitly adds the rows of required size, until all grid items are placed
-
grid-auto-flow: dense
fits the grid content in all possible grid cells, without leaving any gaps between the grid items
-
grid-row: span 2 / auto
spans the specified element across two rows, adapting the row size as needed
-
grid-column: span 2 / auto
spans the specified element across two columns, adapting the column size as needed.
Now, let's add the following styles for tablet devices.
@media screen and (max-width: 768px) {
.gallery {
grid-template-columns: 1fr 1fr;
}
}
Here, the grid-template-columns: 1fr 1fr
property specifies
that the grid should have two columns of equal width.
Browser Output
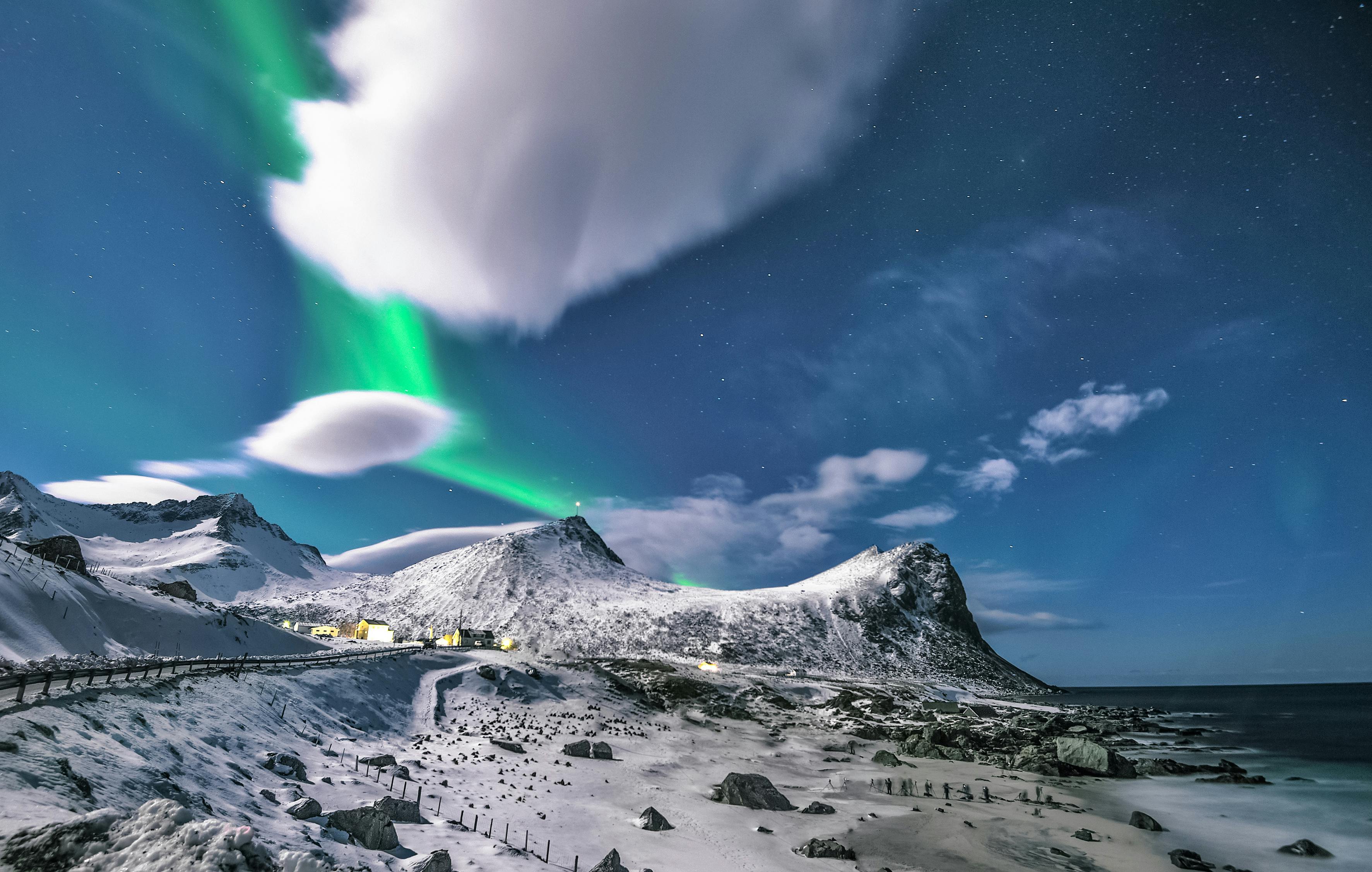
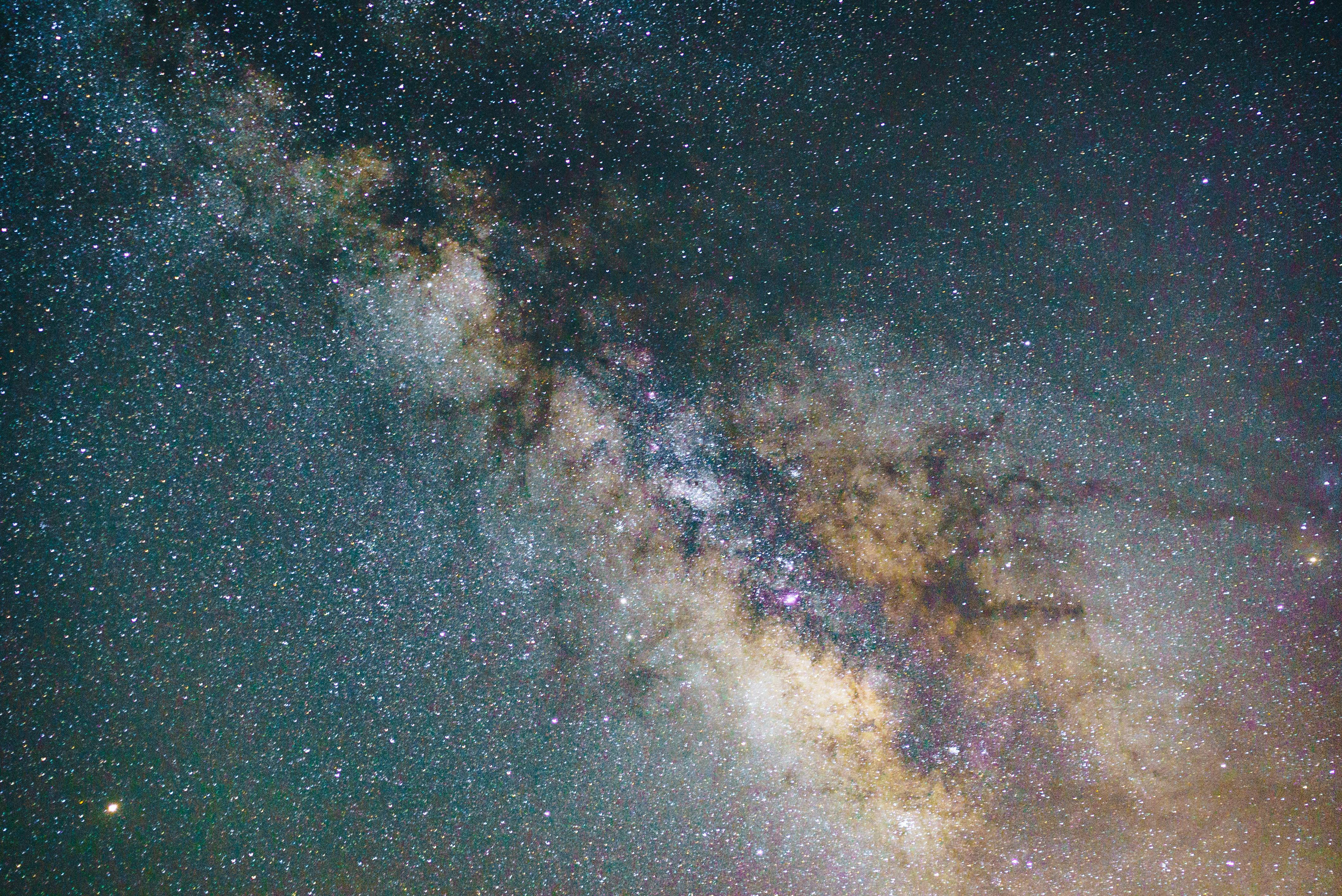
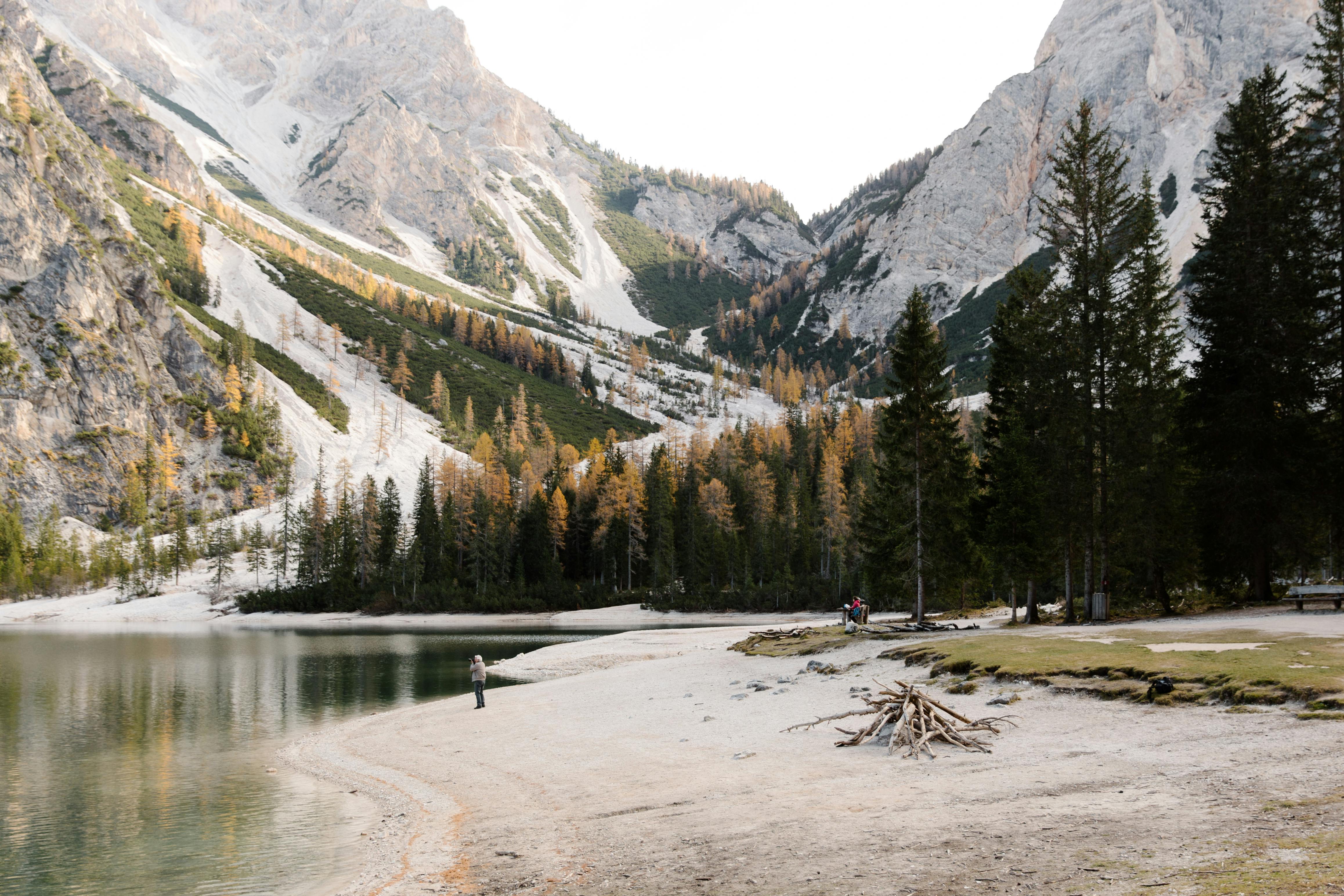
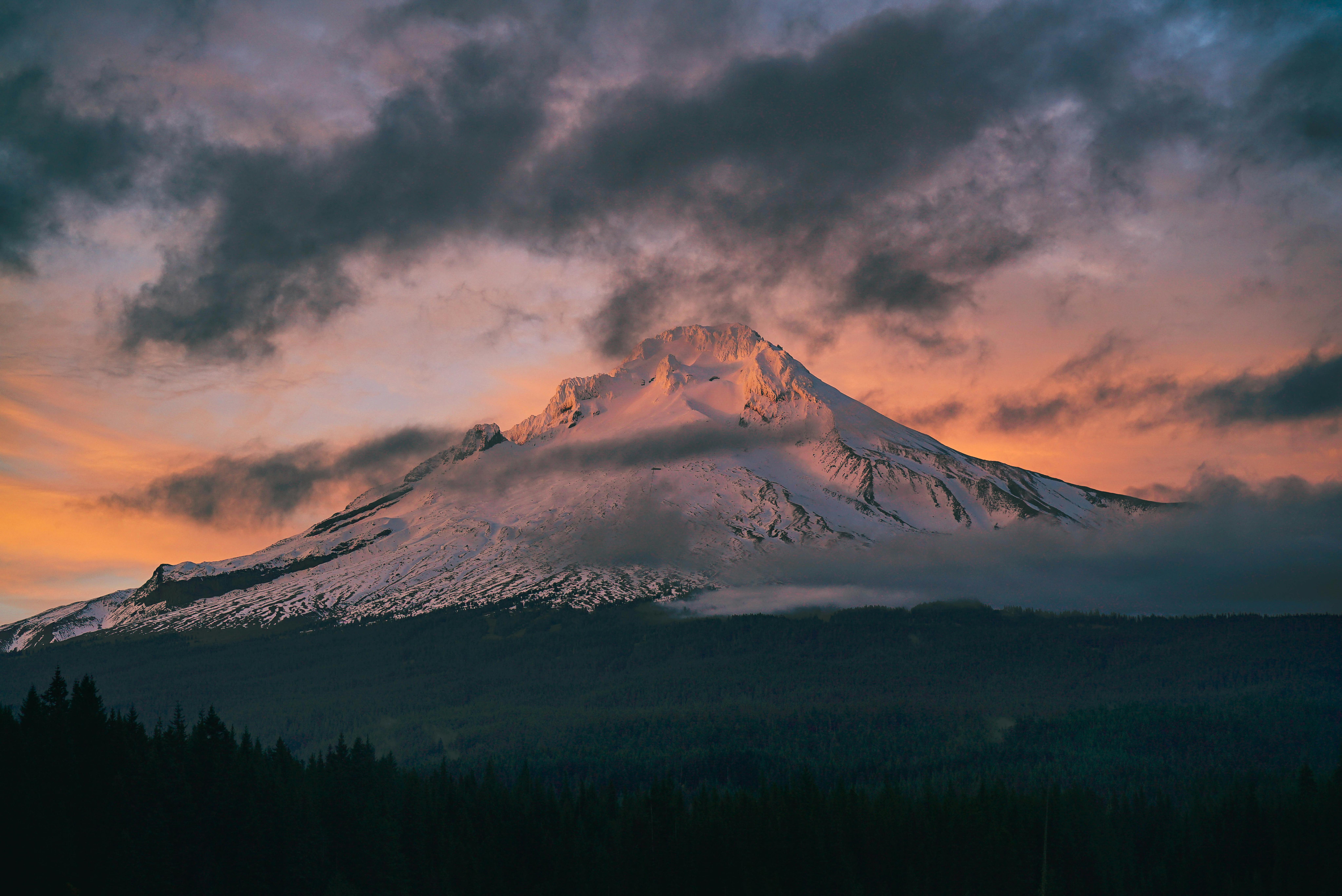
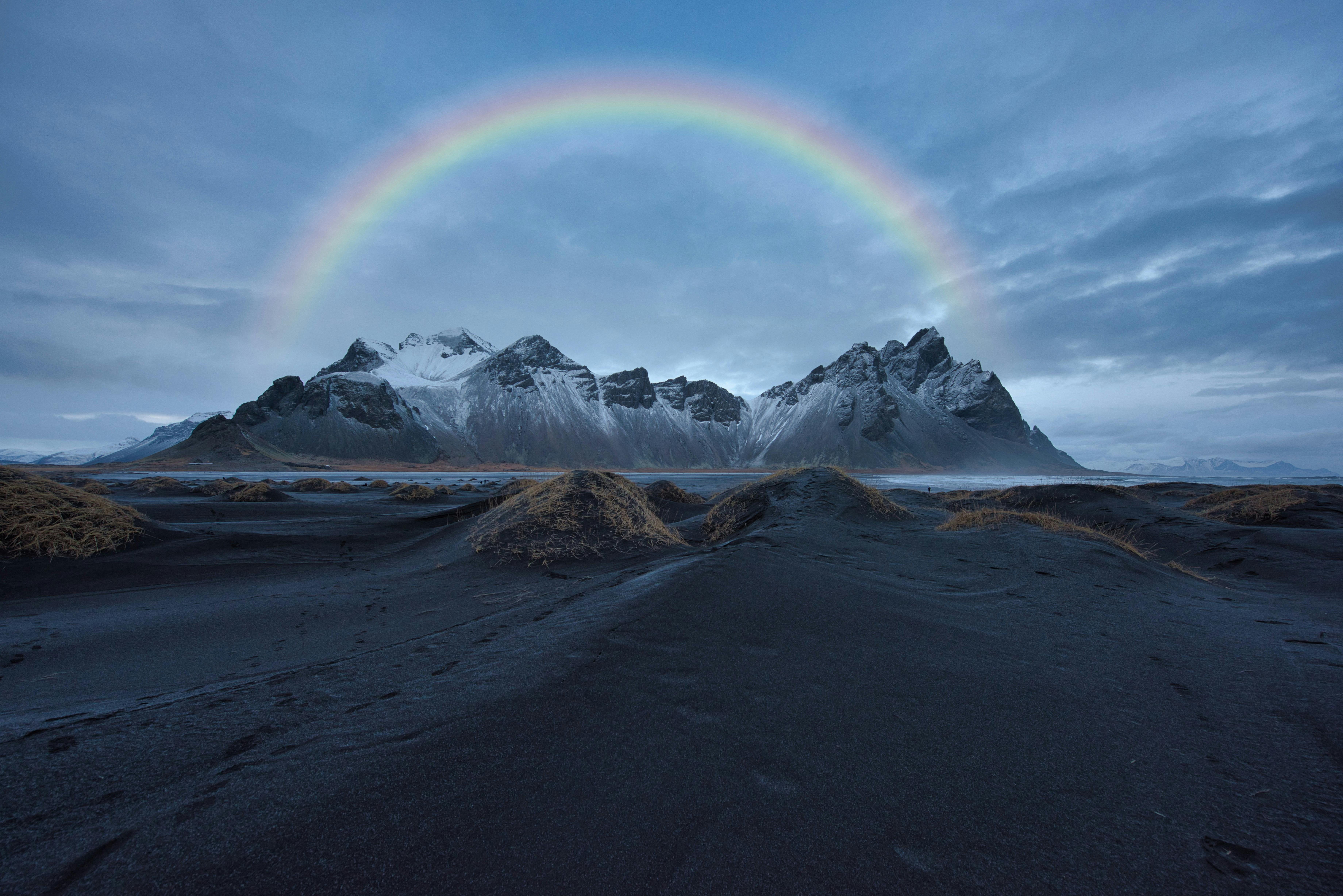
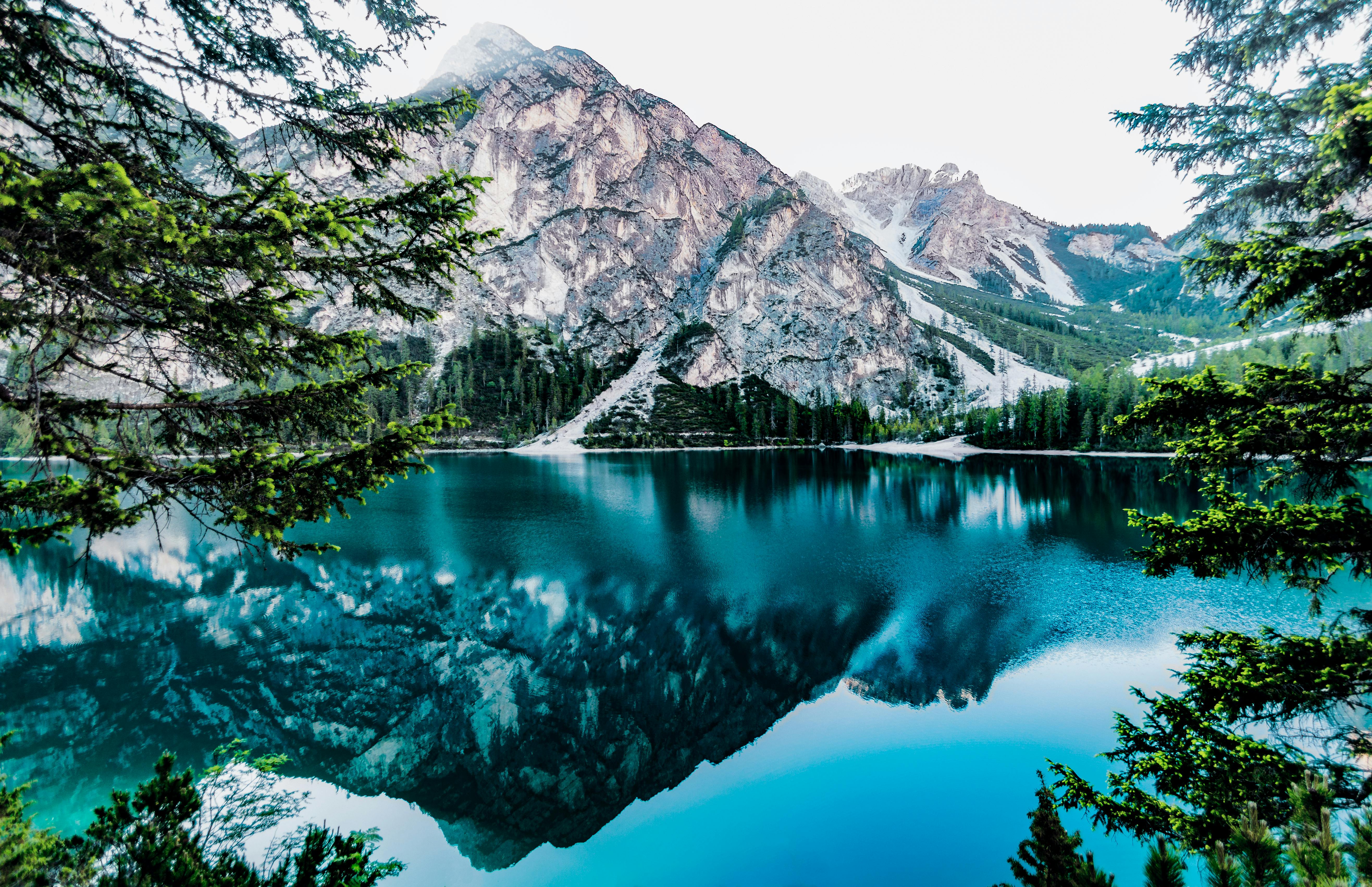
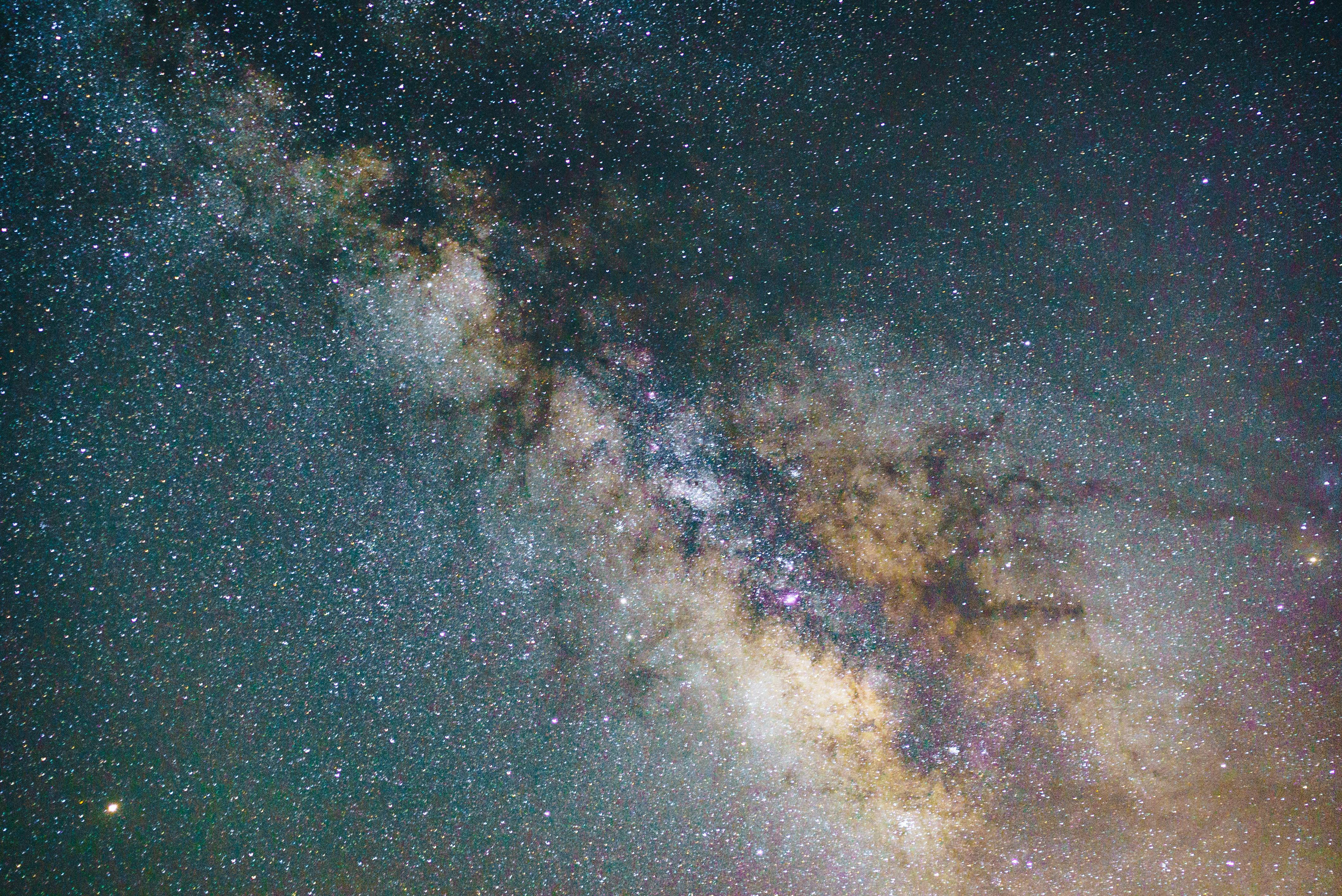
Finally, the following style changes the 2-column grid layout to a single-column layout for mobile devices.
@media screen and (max-width: 480px) {
/* changes the grid layout to a single column */
div.gallery {
grid-template-columns: 1fr;
}
/* resets the grid placement properties for
the images spanning four grid cells */
img.four-grid-cells {
grid-row: auto;
grid-column: auto;
}
/* resets the grid placement properties for
the images spanning two grid columns */
img.wide-image {
grid-column: auto;
}
}
Browser Output
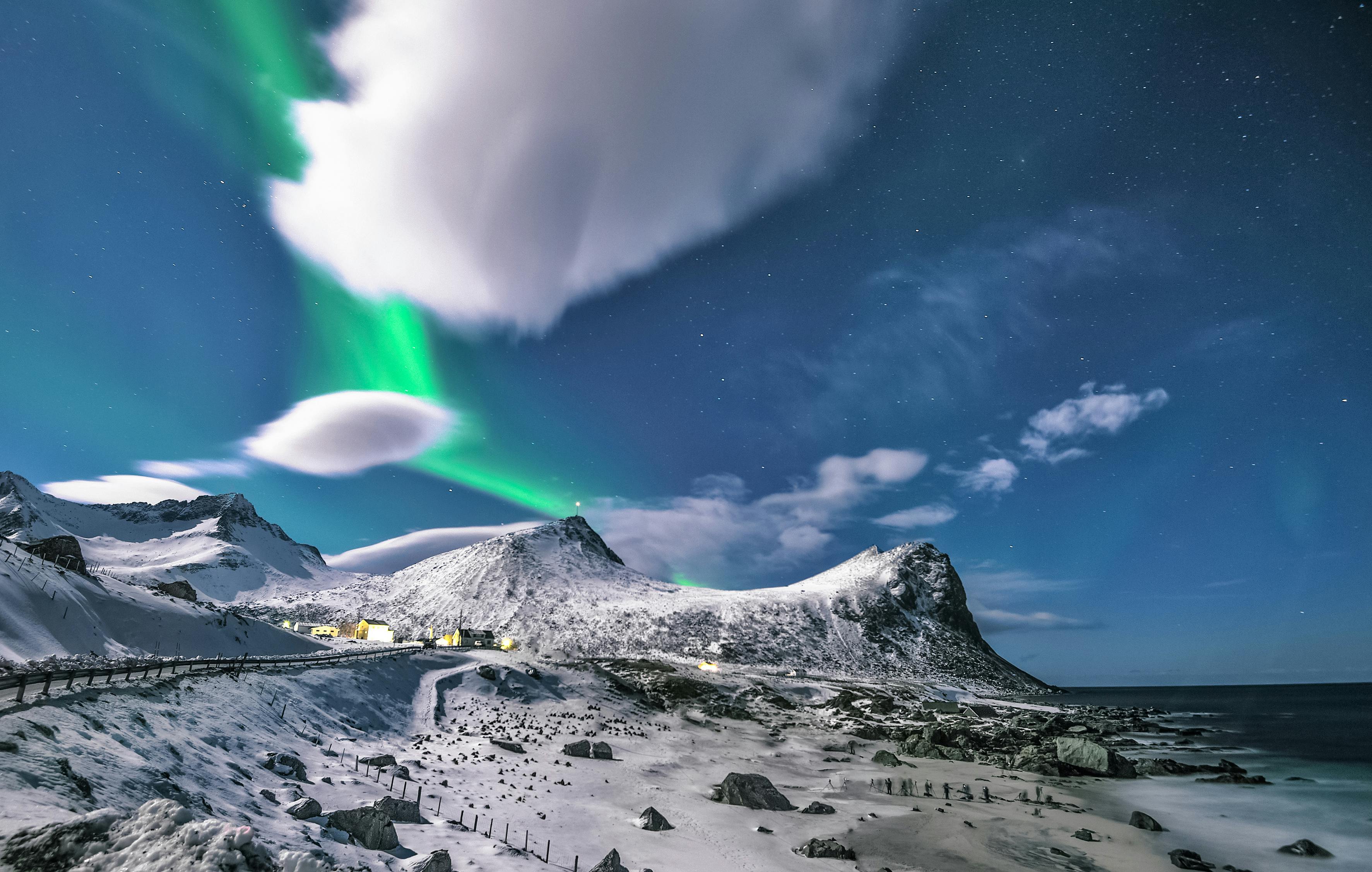
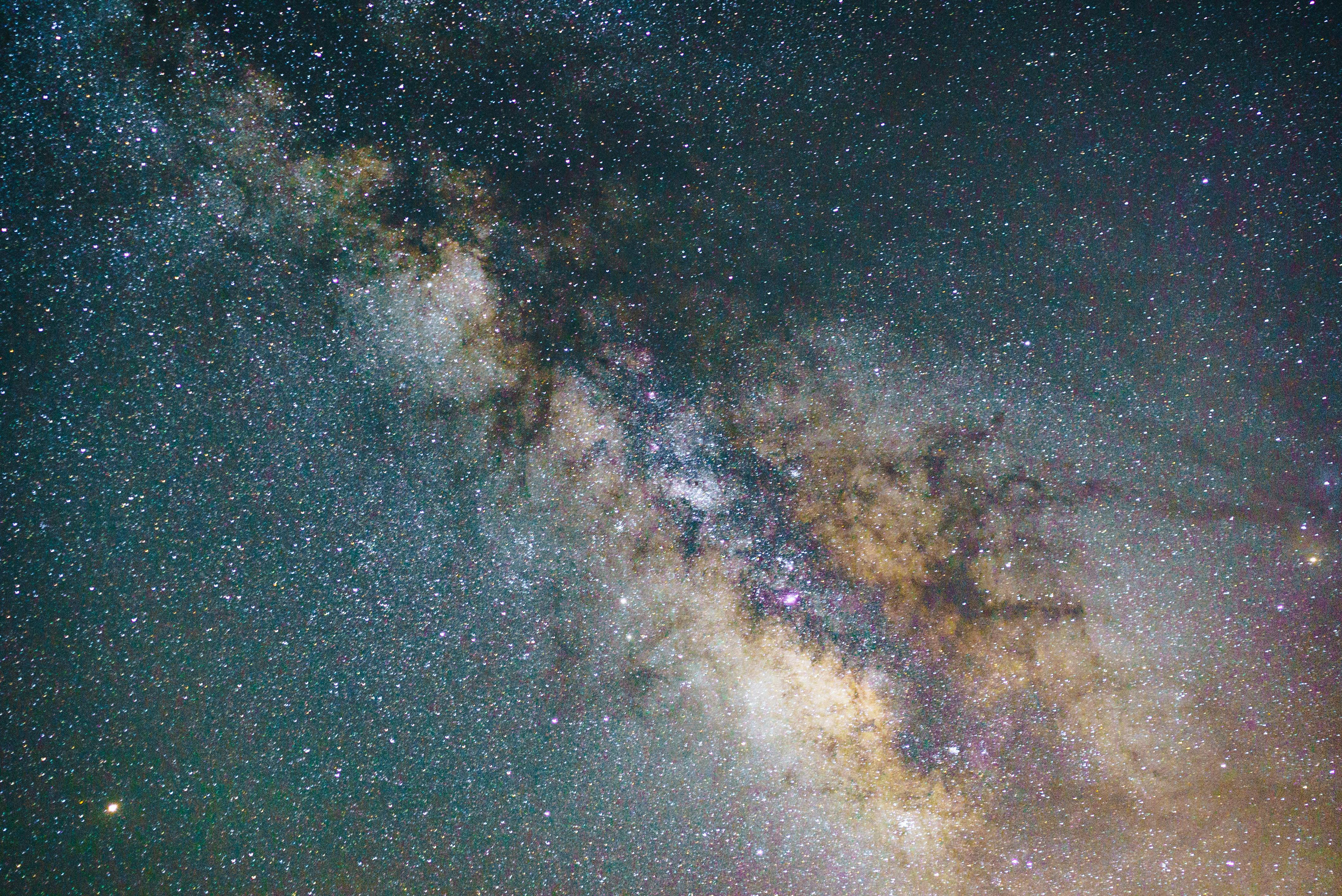
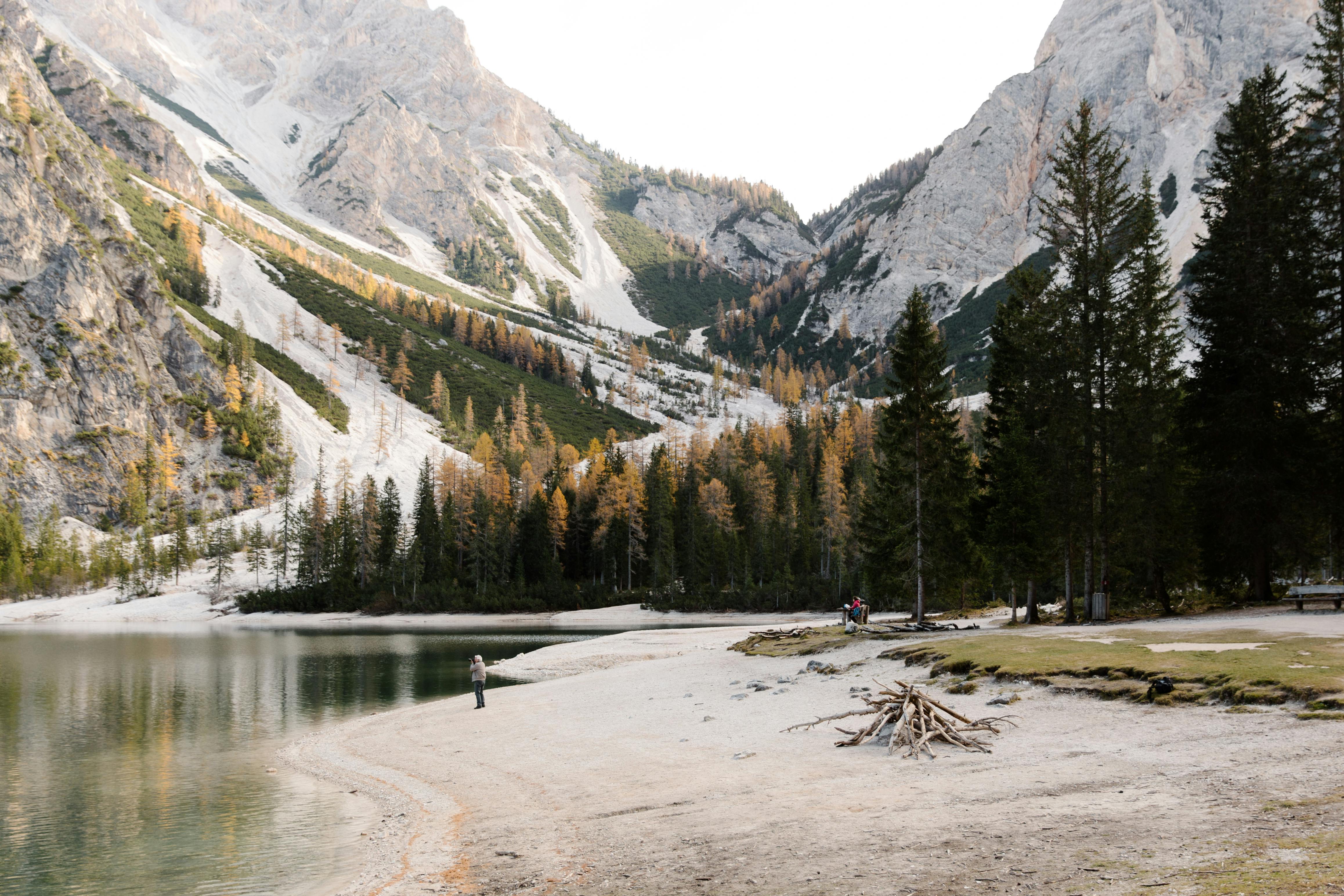
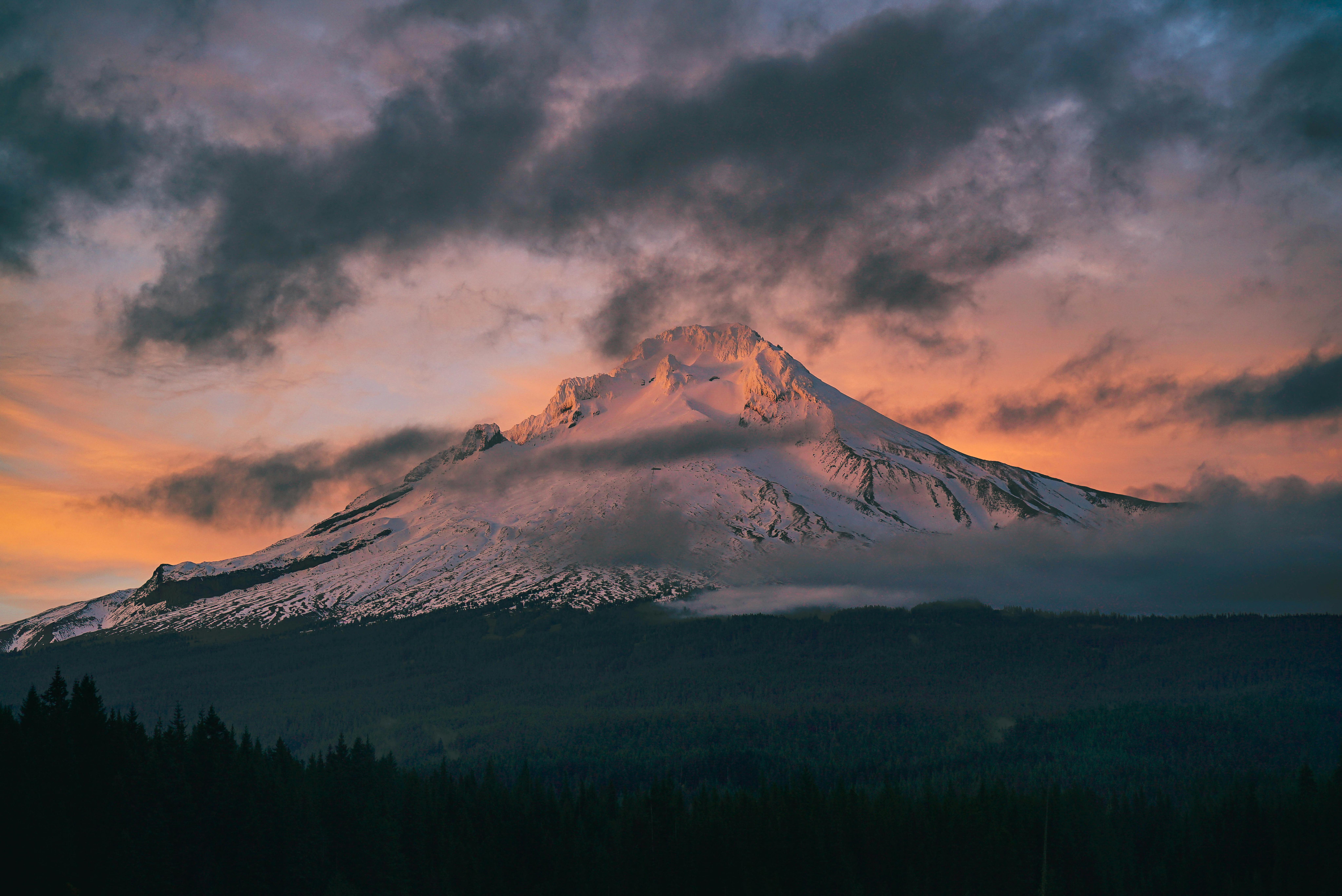
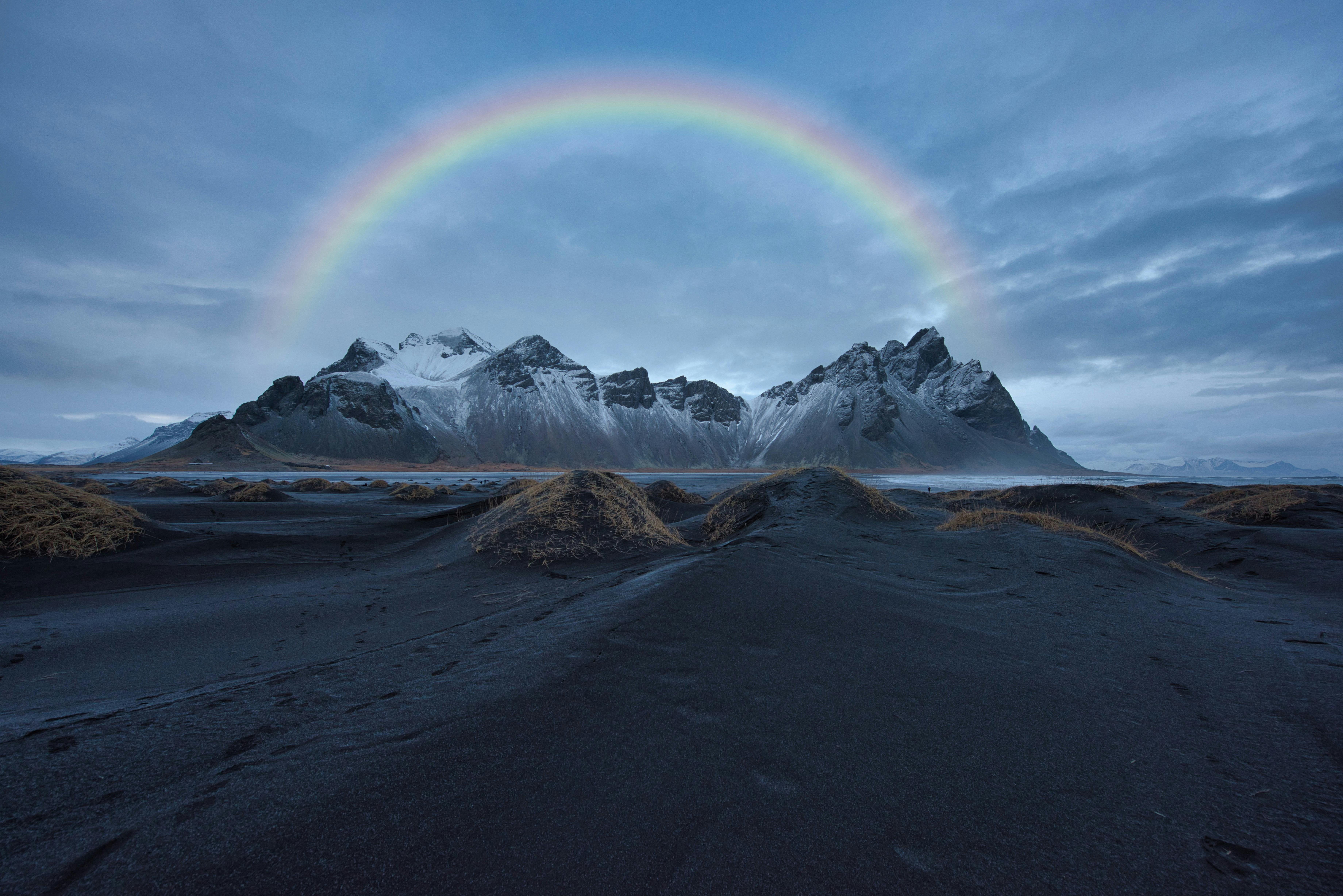
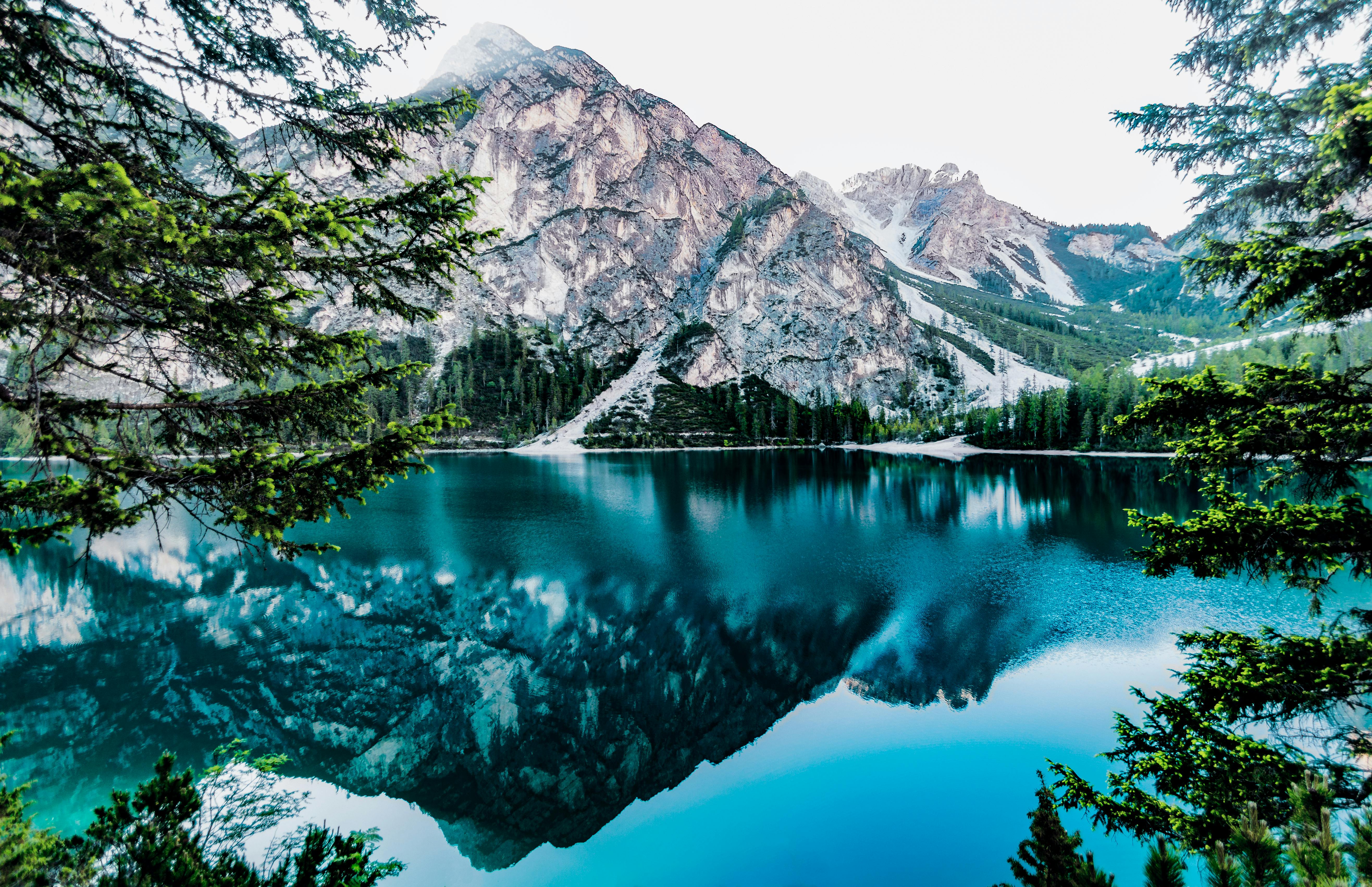
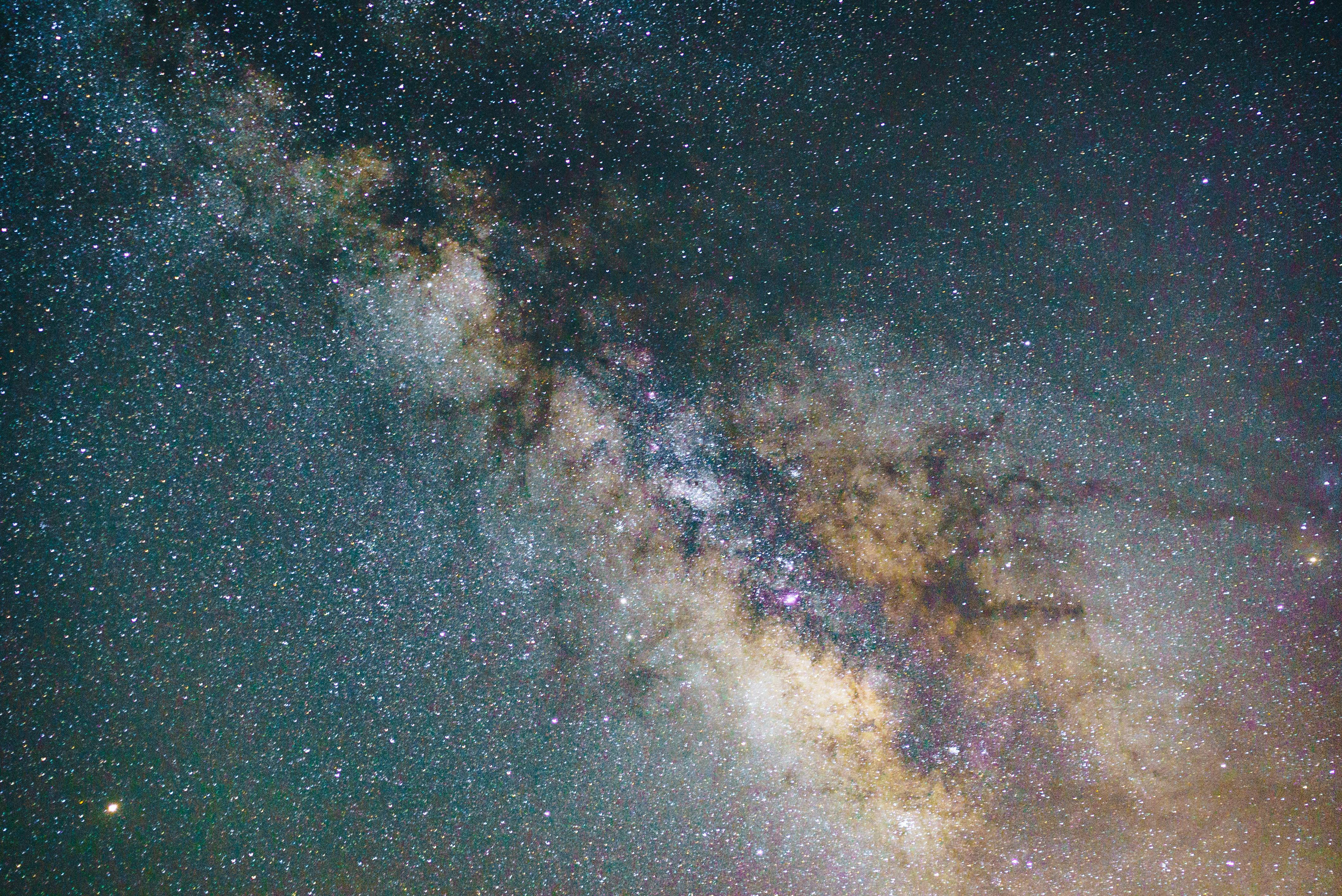
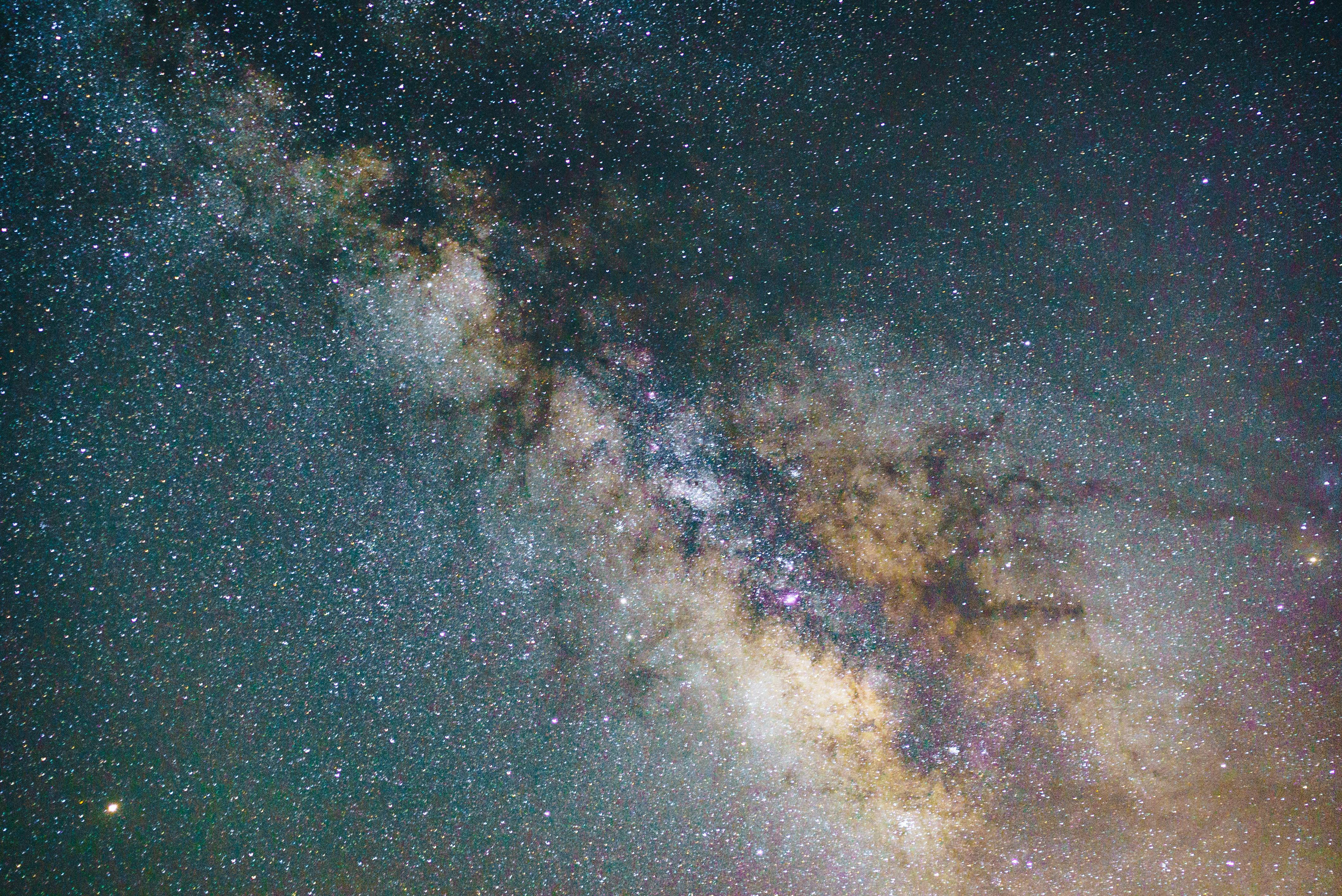