The CSS overflow
property is used to adjust the content when its
size is too big relative to the element box. For example,
div {
height: 140px;
background-color: greenyellow;
border: 2px solid black;
}
Browser Output
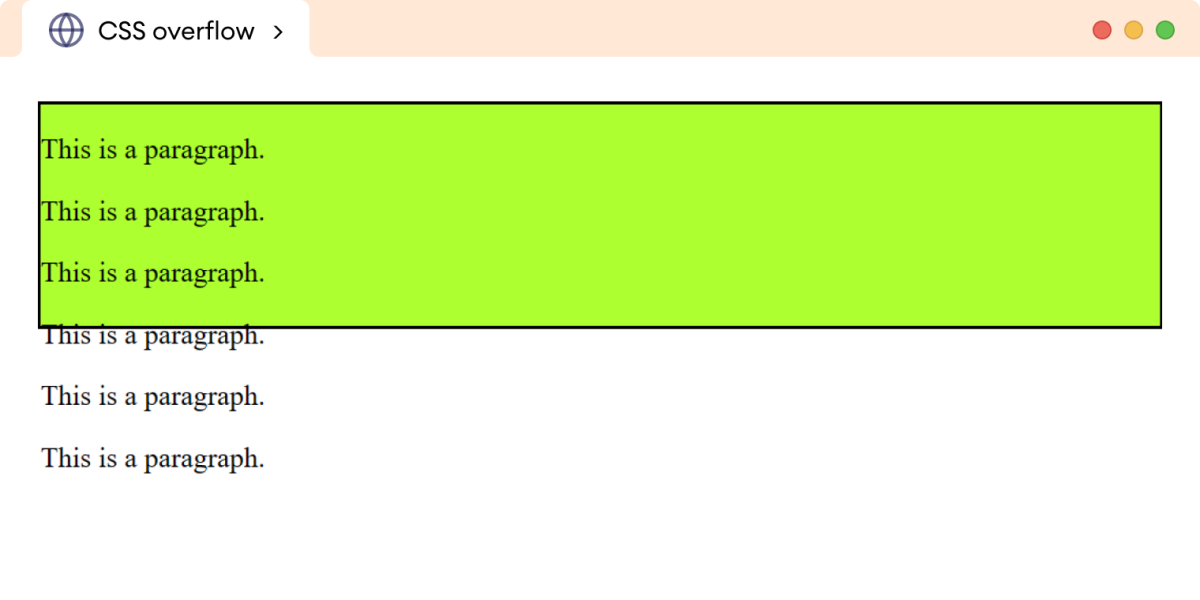
Here, the height of the div
element is smaller than the size of
the content resulting in paragraphs overflowing the div
element.
The overflow
property only works with the block element with a
specified height.
CSS overflow Syntax
The syntax of the overflow
property is as follows:
overflow: visible | hidden | scroll | auto | initial | inherit;
Here,
visible
: allows the content to overflow (default value)hidden
: hides the overflowing content-
scroll
: adds scrollbars to the element box and allows to scroll the content auto
: adds scrollbars only when necessaryinitial
: sets the property value to the default value-
inherit
: inherits the property value from the parent element
CSS overflow: visible
The visible
value of the overflow
property allows
the content to overflow from the container element. This is the default value
of the overflow
property.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Overflow</title>
</head>
<body>
<div>
<p>
"Mostly, when you see programmers, they aren't doing anything.
One of the attractive things about programmers is that you
cannot tell whether or not they are working simply by looking at
them. Very often they're sitting there seemingly drinking coffee
and gossiping, or just staring into space. What the programmer
is trying to do is get a handle on all the individual and
unrelated ideas that are scampering around in his head." —
Charles M. Strauss
</p>
</div>
</body>
</html>
div {
height: 100px;
/* default value */
overflow: visible;
background-color: greenyellow;
border: 2px solid black;
}
p {
font-size: 24px;
margin: 0;
}
Brower Output
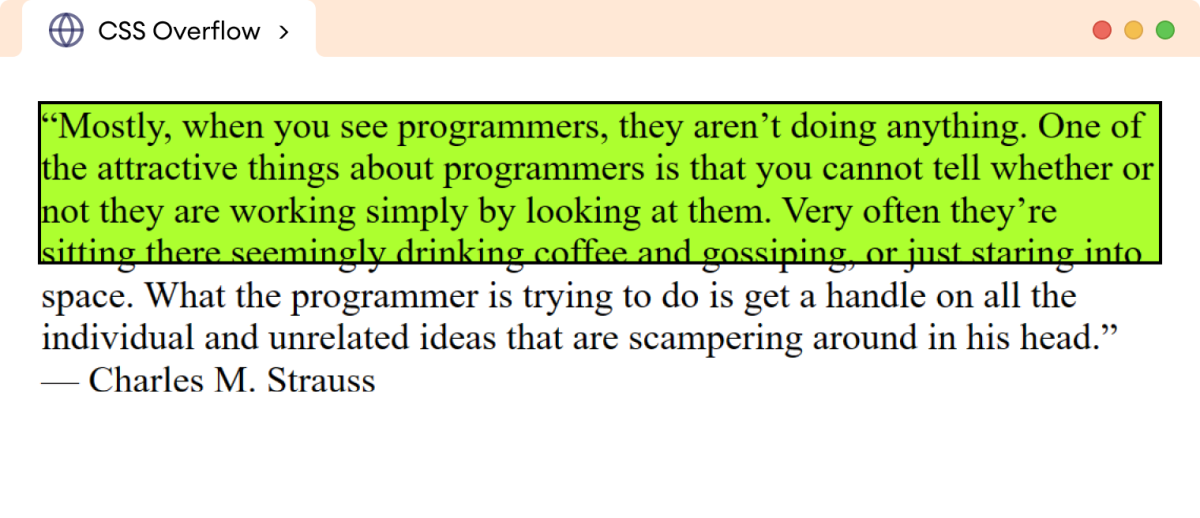
In this example, the content of the p
element is not clipped off
and overflows from the container div
element.
CSS overflow: hidden
The hidden
value of the overflow
property clips off
the overflowing content from the container element. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Overflow</title>
</head>
<body>
<div>
<p>
"Mostly, when you see programmers, they aren't doing anything.
One of the attractive things about programmers is that you
cannot tell whether or not they are working simply by looking at
them. Very often they're sitting there seemingly drinking coffee
and gossiping, or just staring into space. What the programmer
is trying to do is get a handle on all the individual and
unrelated ideas that are scampering around in his head." —
Charles M. Strauss
</p>
</div>
</body>
</html>
div {
height: 100px;
overflow: hidden;
background-color: greenyellow;
border: 2px solid black;
}
p {
font-size: 24px;
margin: 0;
}
Browser Output
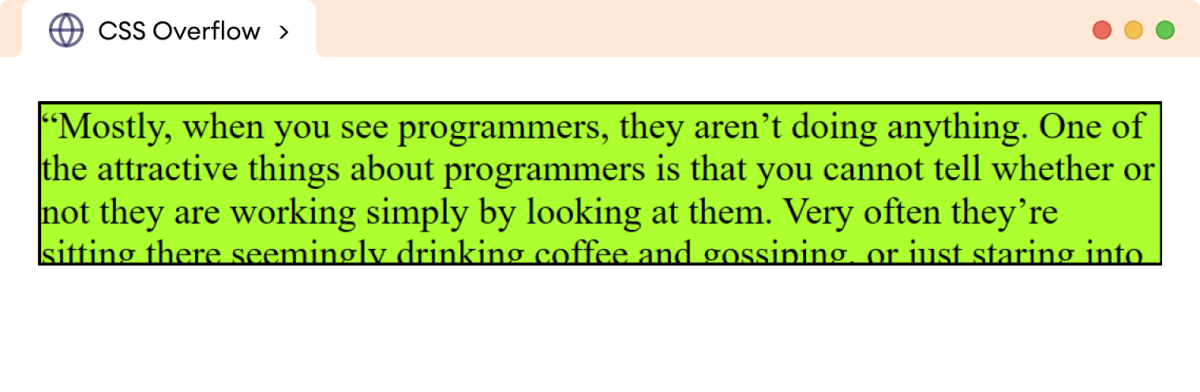
In the above example, the overflowing content of the p
element is
clipped off and hidden from the container div
element.
Note: The overflow: hidden
removes the space
occupied by the overflowed content completely from the document.
CSS overflow: scroll
The scroll
value of the overflow
property creates a
scroll bar within the container for the overflowing content. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Overflow</title>
</head>
<body>
<div>
<p>
"Mostly, when you see programmers, they aren't doing anything.
One of the attractive things about programmers is that you
cannot tell whether or not they are working simply by looking at
them. Very often they're sitting there seemingly drinking coffee
and gossiping, or just staring into space. What the programmer
is trying to do is get a handle on all the individual and
unrelated ideas that are scampering around in his head." —
Charles M. Strauss
</p>
</div>
</body>
</html>
div {
height: 120px;
/* creates both the horizontal and vertical scrollbar */
overflow: scroll;
background-color: greenyellow;
border: 2px solid black;
}
p {
font-size: 24px;
margin: 0;
}
Browser Output
“Mostly, when you see programmers, they aren’t doing anything. One of the attractive things about programmers is that you cannot tell whether or not they are working simply by looking at them. Very often they’re sitting there seemingly drinking coffee and gossiping, or just staring into space. What the programmer is trying to do is get a handle on all the individual and unrelated ideas that are scampering around in his head.” — Charles M. Strauss
Note: The overflow: scroll
adds a scroll bar to
both the horizontal and vertical sides of the container even when we might not
need it.
CSS overflow: auto
The auto
value of the overflow
property adds the
scroll bar automatically only when necessary.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Overflow</title>
</head>
<body>
<div>
<p>
"Mostly, when you see programmers, they aren't doing anything.
One of the attractive things about programmers is that you
cannot tell whether or not they are working simply by looking at
them. Very often they're sitting there seemingly drinking coffee
and gossiping, or just staring into space. What the programmer
is trying to do is get a handle on all the individual and
unrelated ideas that are scampering around in his head." —
Charles M. Strauss
</p>
</div>
</body>
</html>
div {
height: 120px;
/* adds scrollbar only where necessary */
overflow: auto;
background-color: greenyellow;
border: 2px solid black;
}
p {
font-size: 24px;
margin: 0;
}
Browser Output
“Mostly, when you see programmers, they aren’t doing anything. One of the attractive things about programmers is that you cannot tell whether or not they are working simply by looking at them. Very often they’re sitting there seemingly drinking coffee and gossiping, or just staring into space. What the programmer is trying to do is get a handle on all the individual and unrelated ideas that are scampering around in his head.” — Charles M. Strauss
Note: The auto
value is different from the
scroll
value of the overflow
property. The
scroll
value adds a scrollbar to the element regardless of
whether it is needed or not while the auto
value adds a scrollbar
only when necessary.
CSS overflow-x and overflow-y property
The overflow-x
property is used to adjust the
content overflow in only the horizontal direction. For
example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Overflow</title>
</head>
<body>
<div>
<p>
"Mostly, when you see programmers, they aren't doing anything.
One of the attractive things about programmers is that you
cannot tell whether or not they are working simply by looking at
them. Very often they're sitting there seemingly drinking coffee
and gossiping, or just staring into space. What the programmer
is trying to do is get a handle on all the individual and
unrelated ideas that are scampering around in his head." —
Charles M. Strauss
</p>
</div>
</body>
</html>
div {
width: 500px;
/* adds scrollbar in horizontal direction */
overflow-x: scroll;
background-color: greenyellow;
border: 2px solid black;
}
p {
/* specifying width greater than div */
width: 700px;
font-size: 24px;
margin: 0;
}
Browser Output
“Mostly, when you see programmers, they aren’t doing anything. One of the attractive things about programmers is that you cannot tell whether or not they are working simply by looking at them. Very often they’re sitting there seemingly drinking coffee and gossiping, or just staring into space. What the programmer is trying to do is get a handle on all the individual and unrelated ideas that are scampering around in his head.” — Charles M. Strauss
The overflow-y
property is used to adjust the
content overflow in only the vertical direction. For
example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Overflow</title>
</head>
<body>
<div>
<p>
"Mostly, when you see programmers, they aren't doing anything.
One of the attractive things about programmers is that you
cannot tell whether or not they are working simply by looking at
them. Very often they're sitting there seemingly drinking coffee
and gossiping, or just staring into space. What the programmer
is trying to do is get a handle on all the individual and
unrelated ideas that are scampering around in his head." —
Charles M. Strauss
</p>
</div>
</body>
</html>
div {
height: 120px;
/* adds scrollbar in the vertical direction */
overflow-y: scroll;
background-color: greenyellow;
border: 2px solid black;
}
p {
font-size: 24px;
margin: 0;
}
Browser Output
“Mostly, when you see programmers, they aren’t doing anything. One of the attractive things about programmers is that you cannot tell whether or not they are working simply by looking at them. Very often they’re sitting there seemingly drinking coffee and gossiping, or just staring into space. What the programmer is trying to do is get a handle on all the individual and unrelated ideas that are scampering around in his head.” — Charles M. Strauss