The CSS clear
property controls the behavior of elements that are adjacent to floated elements. It allows to turn off the wrapping of the text and moves the element below the floating element.
Let's look at an example.
p {
float: right;
}
p.second {
clear: right;
}
Browser Output
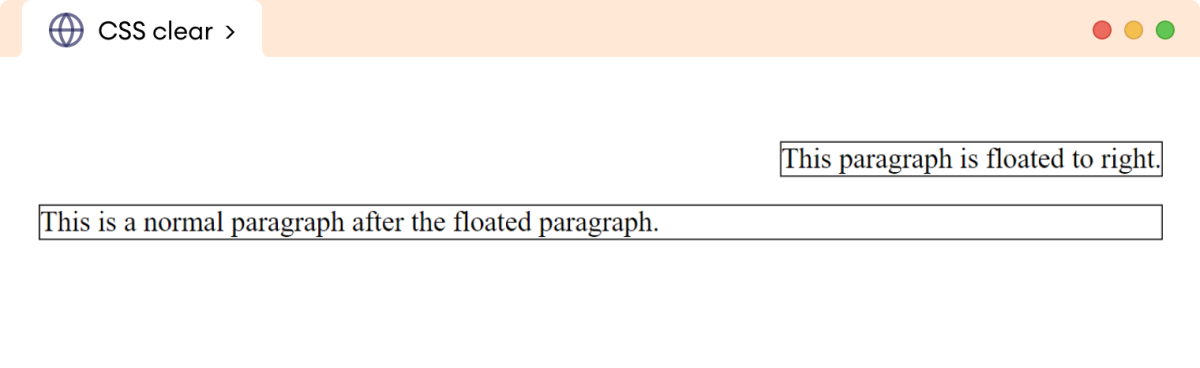
Here, the clear
property moves the adjacent paragraph below the floated paragraph and prevents wrapping the text around the floated paragraph.
CSS clear Syntax
The syntax of the clear
property is as follows,
clear: none | left | right | both | initial | inherit;
Here,
none
: allows the element to float (default value)left
: moves element below any left floating elementsright
: moves element below any right floating elementsboth
: moves element below any floating elements (both left and right)initial
: sets the property value to the default valueinherit
: inherits the property value from its parent element
Note: The clear
property applies to both floating and non-floating elements.
CSS clear none Example
The none
value of the clear
property is the default value and allows elements to float. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS clear</title>
</head>
<body>
<div>
<img
src="https://freepngimg.com/thumb/doraemon/35051-9-doraemon-transparent-thumb.png"
alt="Doraemon Photo"
/>
<p>
Doraemon is a beloved cartoon character adored by kids all over
the world. Doraemon is a robotic cat from the future who travels
back in time to help a young boy named Nobita. With his magical
gadgets and clever solutions, Doraemon always manages to turn
Nobita's troubles into exciting adventures. Whether it's
rescuing friends, solving problems, or teaching important life
lessons, Doraemon's kind heart and unwavering friendship make
him a true hero.
</p>
</div>
</body>
</html>
img {
/* moves the image to the right of its parent */
float: right;
width: 100px;
height: 100px;
}
p {
/* default value; makes no change */
clear: none;
}
/* styles the div element */
div {
border: 2px solid black;
}
Browser Output
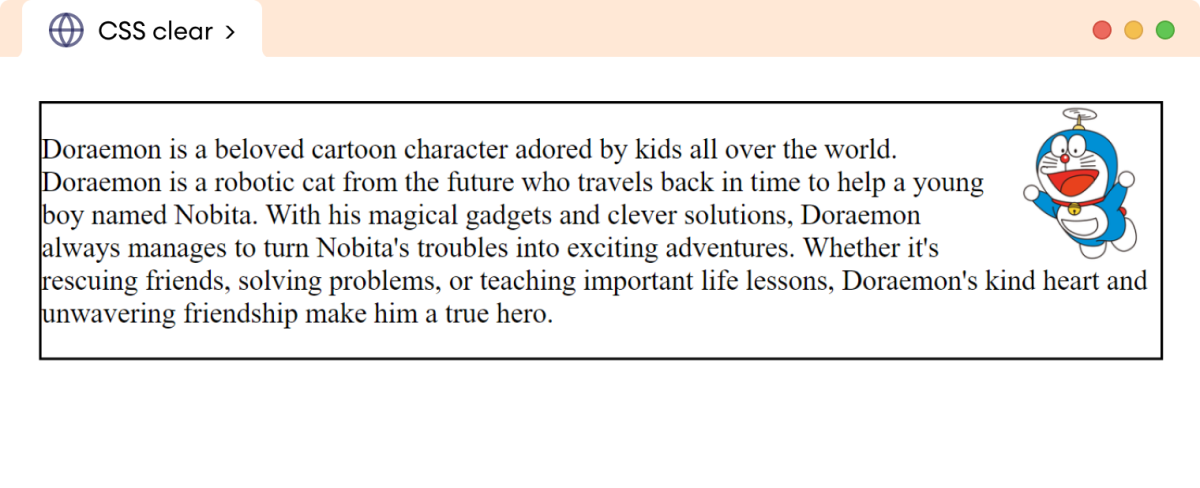
In the above example, the none
(default value) of the clear
property still allows the paragraph to wrap around the floated image element.
CSS clear left Example
The clear
property with a value of left
clears the left side of the preceding floated element, causing it to be positioned below in a new line.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS clear</title>
</head>
<body>
<div>
<img
src="https://freepngimg.com/thumb/doraemon/35051-9-doraemon-transparent-thumb.png"
alt="Doraemon Photo"
/>
<p>
Doraemon is a beloved cartoon character adored by kids all over
the world. Doraemon is a robotic cat from the future who travels
back in time to help a young boy named Nobita.
</p>
</div>
</body>
</html>
img {
/* moves the image to the left of its parent */
float: left;
width: 100px;
height: 100px;
}
p {
/* clears the left side and moves into a new line */
clear: left;
}
/* styles the div element */
div {
border: 2px solid black;
}
Browser Output
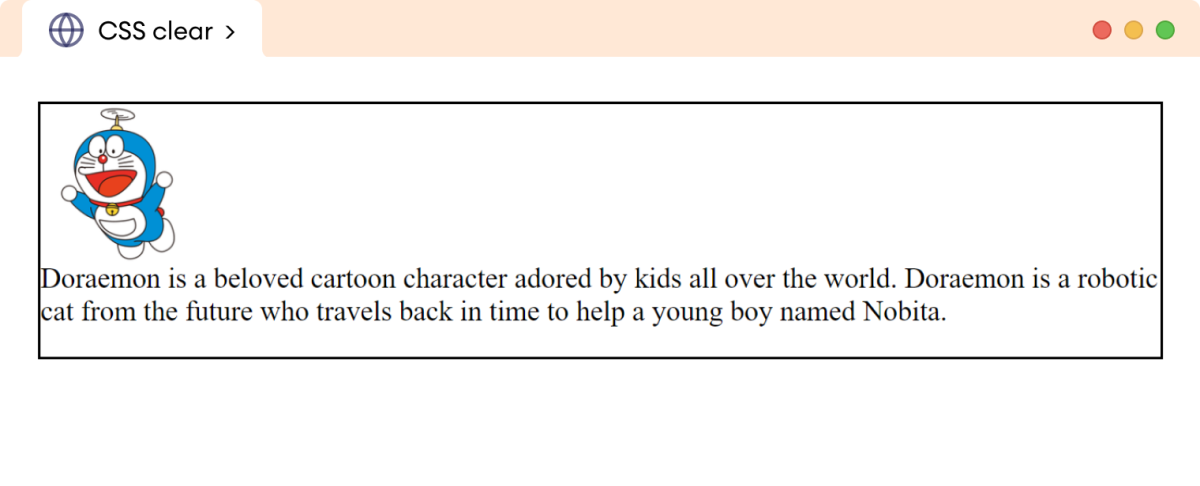
In the above example, the clear: left
on the p
element clears the left space and moves it to a new line.
CSS clear right Example
The clear
property with a value of right
clears the right side of the preceding floated element, causing it to be positioned below in a new line.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS clear</title>
</head>
<body>
<div>
<img
src="https://freepngimg.com/thumb/doraemon/35051-9-doraemon-transparent-thumb.png"
alt="Doraemon Photo"
/>
<p>
Doraemon is a beloved cartoon character adored by kids all over
the world. Doraemon is a robotic cat from the future who travels
back in time to help a young boy named Nobita.
</p>
</div>
</body>
</html>
img {
/* moves the image to the right of its parent */
float: right;
width: 100px;
height: 100px;
}
p {
/* clears the left side and moves into a new line */
clear: right;
}
/* styles the div element */
div {
border: 2px solid black;
}
Browser Output
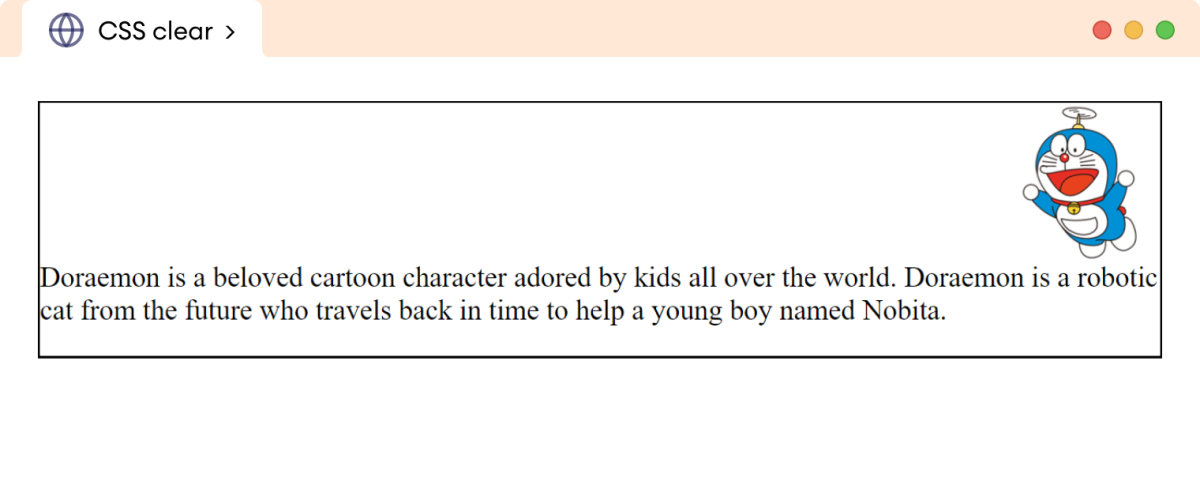
In the above example, the clear: right
on the p
element clears the right space and moves it to a new line.
Issues with CSS float
There are the following two main issues with the CSS float
property,
- The parent or container box collapses completely when all its contents are floated.
- The floated element overflows the parent element if its height is greater than the parent element.
Let's have a look at each of them.
CSS Collapsing Issue
The parent element or container box collapses when all the contents are floated. The floated elements move from the normal flow of the document causing and as a result parent box loses its dimension.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS clear</title>
</head>
<body>
<div>
<img
src="https://freepngimg.com/thumb/doraemon/35051-9-doraemon-transparent-thumb.png"
alt="Doraemon Photo" />
<p>
Doraemon is a beloved cartoon character adored by kids all over
the world. Doraemon is a robotic cat from the future who travels
back in time to help a young boy named Nobita.
</p>
</div>
</body>
</html>
img,
p {
/* moves both image and p to the right of its parent */
float: right;
border: 2px solid orange;
}
img {
width: 100px;
height: 100px;
}
/* styles the div element */
div {
padding: 12px;
border: 2px solid black;
background-color: greenyellow;
}
Browser Output
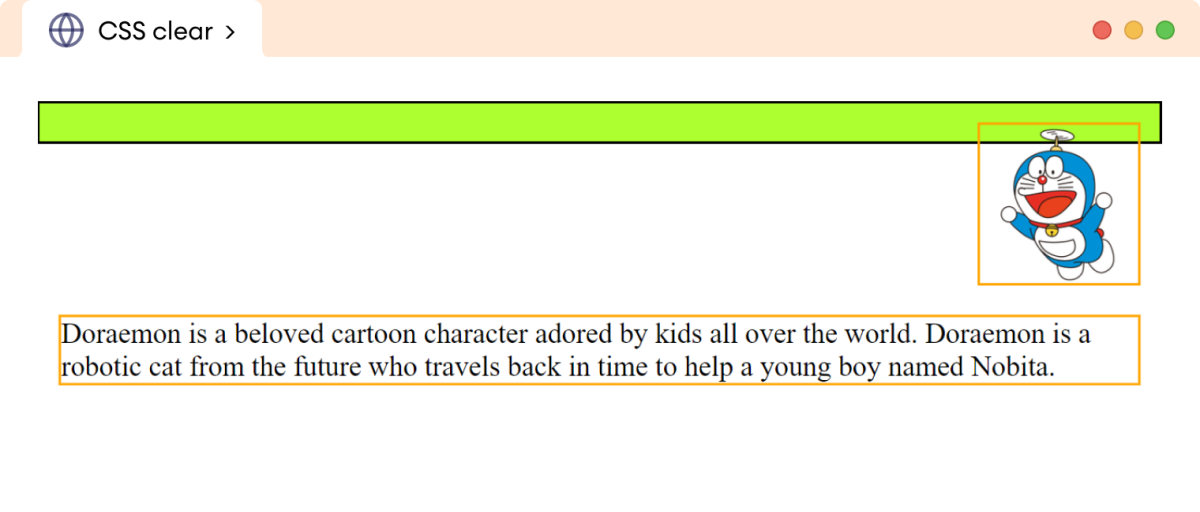
In the above example, both p
and img
elements are set to float: right
causing the parent div
to collapse.
The space visible within the div
is due to the height of the padding
and the border
.
CSS Overflow Issue
The floated element overflows the parent or container element if its height exceeds the height of the parent element.
The floated element's content is still visible outside the parent element causing the overlapping with other elements on the page.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS clear</title>
</head>
<body>
<div>
<img
src="https://freepngimg.com/thumb/doraemon/35051-9-doraemon-transparent-thumb.png"
alt="Doraemon Photo"
/>
<p>
Doraemon is a beloved cartoon character adored by kids all over
the world. Doraemon is a robotic cat from the future who travels
back in time to help a young boy named Nobita.
</p>
</div>
</body>
</html>
img {
/* moves the image to the right of its parent */
float: right;
width: 170px;
height: 170px;
border: 2px solid orange;
}
/* styles the div element */
div {
border: 2px solid black;
}
Browser Output
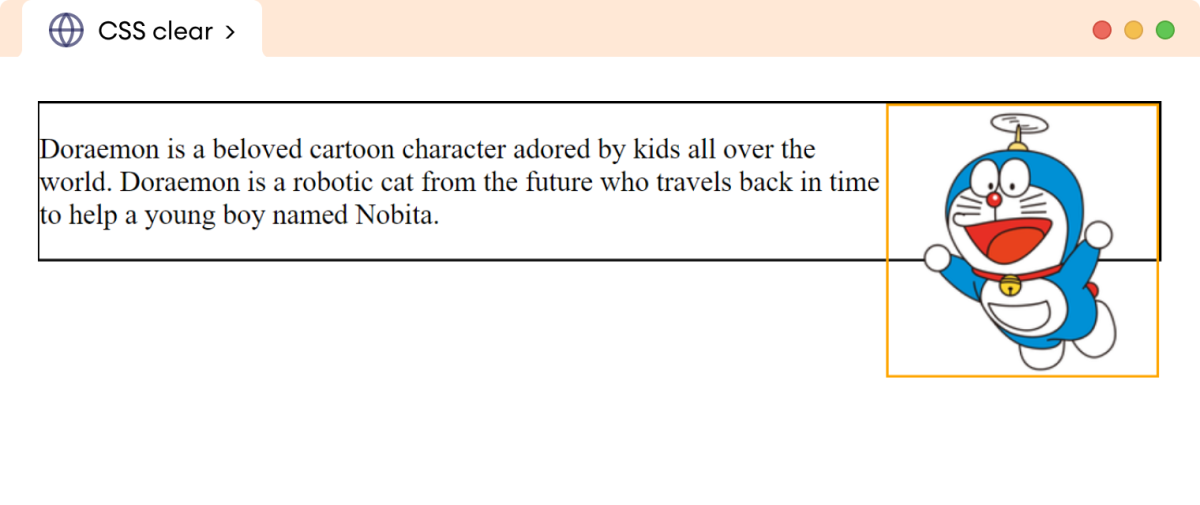
In the above example, the height of img
is greater than the height of the parent div
element causing it to overflow the parent.
The issues of collapsing and overflowing the parent element can be solved using the empty div method, CSS overflow property, and using the Clearfix hack.
Let's look into each of them.
Using Empty Div Element
An empty <div>
element is commonly used to solve the collapsing and overflow issues.
The empty <div>
element is positioned after the floated element, and the clear: both
style is applied.
Let's see an example of the overflow issue,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS clear</title>
</head>
<body>
<div class="parent">
<img
src="https://freepngimg.com/thumb/doraemon/35051-9-doraemon-transparent-thumb.png"
alt="Doraemon Photo"
/>
<p>
Doraemon is a beloved cartoon character adored by kids all over
the world. Doraemon is a robotic cat from the future who travels
back in time to help a young boy named Nobita.
</p>
<div class="empty"></div>
</div>
</body>
</html>
img {
/* moves the image to the right of its parent */
float: right;
width: 170px;
height: 170px;
border: 2px solid orange;
}
/* styles for empty div element; solves the overflow issue */
div.empty {
clear: both;
}
/* styles the div element */
div.parent {
border: 2px solid black;
}
Browser Output
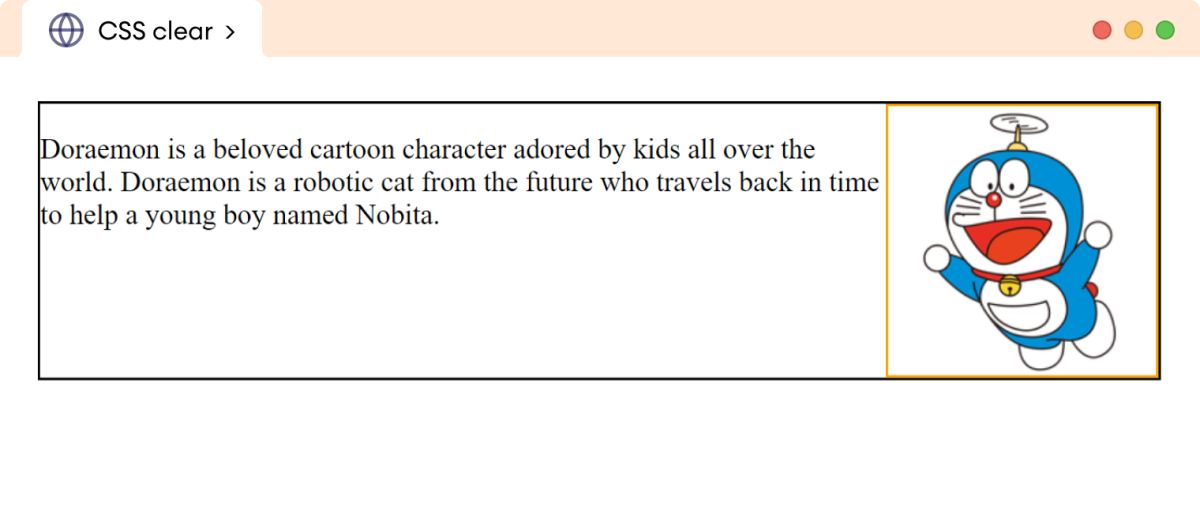
In the above example, the empty div
element with clear: both
expands the parent div
element fit the image.
Using CSS overflow Property
The CSS overflow
property with the value of auto
or hidden
in the parent element is used to solve the collapse and overflow issue. The parent element expands to contain the floating elements.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS clear</title>
</head>
<body>
<div class="parent">
<img
src="https://freepngimg.com/thumb/doraemon/35051-9-doraemon-transparent-thumb.png"
alt="Doraemon Photo"
/>
<p>
Doraemon is a beloved cartoon character adored by kids all over
the world. Doraemon is a robotic cat from the future who travels
back in time to help a young boy named Nobita.
</p>
</div>
</body>
</html>
img,
p {
/* moves both image and p to the right of its parent */
float: right;
border: 2px solid orange;
}
img {
width: 100px;
height: 100px;
}
div.parent {
/* expands the div to contain the floating elements */
overflow: auto;
border: 2px solid black;
}
Browser Output
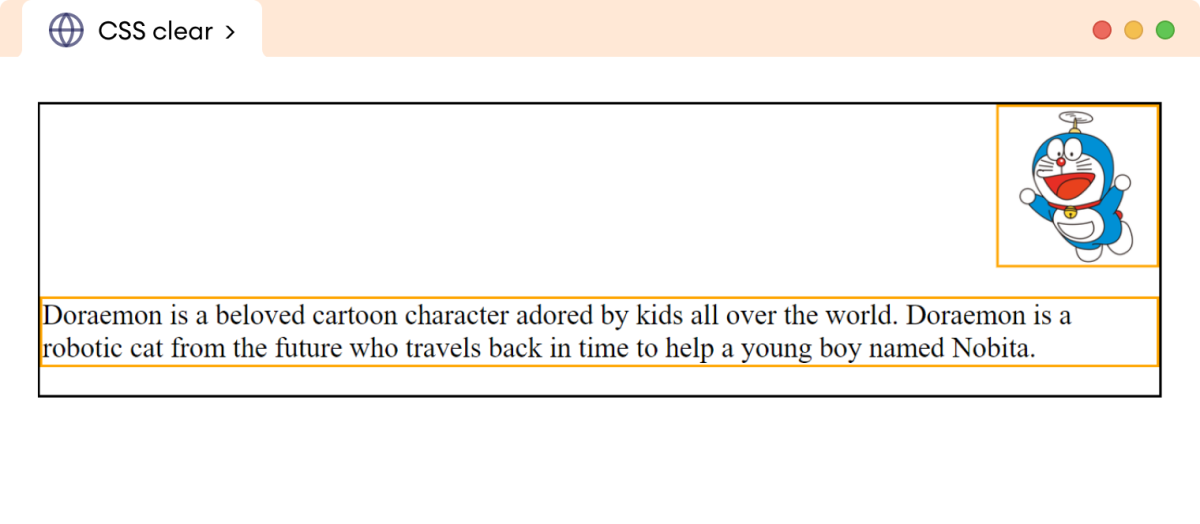
In the above example, the overflow property expands the parent div
element to contain both the floating p
and img
elements, preventing the collapse of the parent element.
The Clearfix Hack
The clearfix method is also commonly used for preventing collapsing and overflow issues. In this method, a CSS pseudo selector (::after
) is used on the parent element to clear floats.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS clear</title>
</head>
<body>
<div class="clearfix">
<img
src="https://freepngimg.com/thumb/doraemon/35051-9-doraemon-transparent-thumb.png"
alt="Doraemon Photo"
/>
<p>
Doraemon is a beloved cartoon character adored by kids all over
the world. Doraemon is a robotic cat from the future who travels
back in time to help a young boy named Nobita.
</p>
</div>
</body>
</html>
img,
p {
/* moves both image and p to the right of its parent */
float: right;
border: 2px solid orange;
}
/* expands the div element having clearfix class to prevent collapsing */
.clearfix::after {
content: "";
display: block;
clear: both;
}
img {
width: 100px;
height: 100px;
}
/* styles the div element */
div {
border: 2px solid black;
}
Browser Output
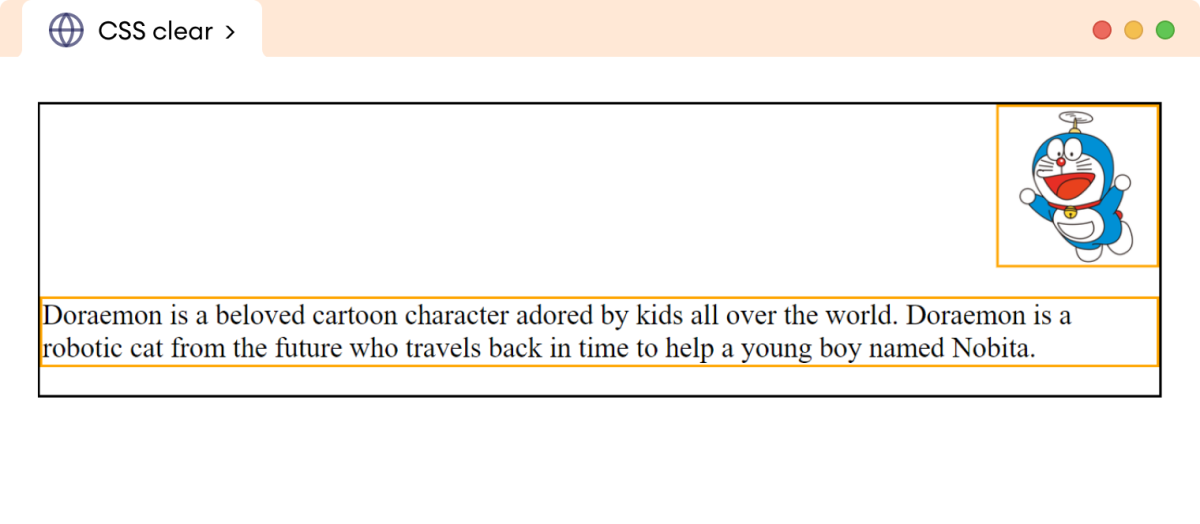
In the above example, the clearfix method prevents the parent div
element from collapsing and contains both the floating p
and img
elements.