The CSS position
property is used to define the position of an
element on a webpage.
The location of the positioned element is set with the four properties:
top
, left
, right
, and
bottom
. These properties only work when the
position
property is set and have different positioning
behaviors.
The position
property has the following five values:
- static (default value)
- relative
- absolute
- fixed
- sticky
We will look into each of them.
CSS static Position
The static
value of the position
property allows
elements to be positioned accordingly to the normal flow of the document.
The static
position is not affected by the top
,
right
, bottom
, and left
values.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS position</title>
</head>
<body>
<p>This is a normal paragraph.</p>
<p class="main">This paragraph is positioned using the static value.</p>
<p>This is a normal paragraph.</p>
</body>
</html>
p {
border: 1px solid black;
padding: 4px;
}
p.main {
position: static;
top: 50px; /* doesn't work */
right: 50px; /* doesn't work */
bottom: 50px; /* doesn't work */
left: 50%; /* doesn't work */
background-color: greenyellow;
}
Browser Output
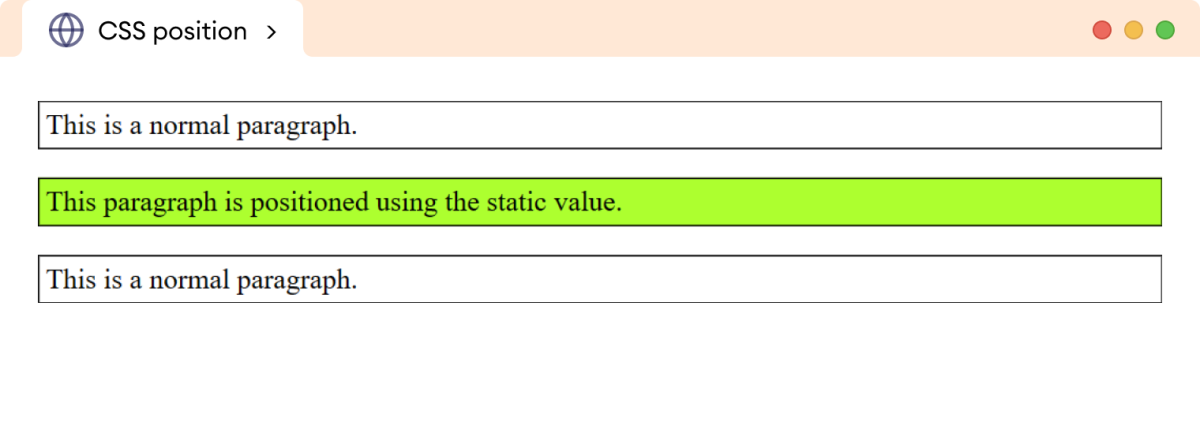
Note: The static
value is the default value
for the position
property.
CSS Relative Position
The relative
value positions the element relative to the
original position in the document. The element is positioned with the
top
, right
, bottom
, and
left
values.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS position</title>
</head>
<body>
<p>This is a normal paragraph.</p>
<p class="main">
This paragraph is positioned with a relative value of top 50px and
left 40px.
</p>
<p>This is a normal paragraph.</p>
</body>
</html>
p {
border: 1px solid black;
padding: 4px;
}
p.main {
position: relative;
/* positions 90px from the top */
top: 90px;
/* positions 40px from the left */
left: 40px;
background-color: greenyellow;
}
Browser Output
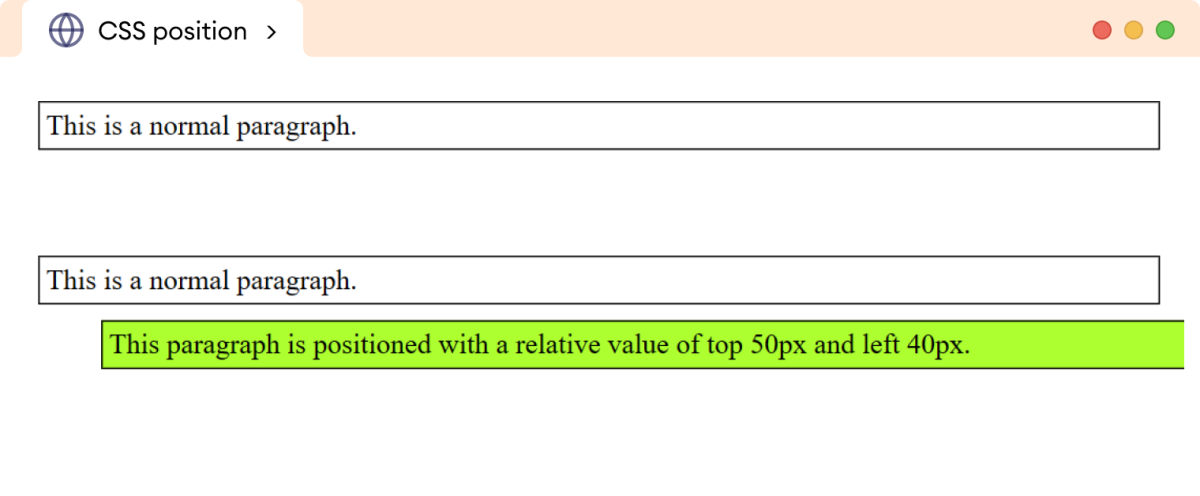
In the above example, the second paragraph is positioned relative to its
normal flow i.e. 90px
from the top and 40px
from
the left of the original position.
The space is preserved in the original position of the element.
CSS Absolute Position
The absolute
value removes the element completely from its
normal flow in the document.
The element is positioned relative to their closest positioned parent
element (an ancestor element with a position value other than
static
).
If there is no positioned ancestor, they are positioned relative to the document itself.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS position</title>
</head>
<body>
<p>This is a normal paragraph.</p>
<p class="main">
This is an absolutely positioned paragraph at 40px top and 60px left.
</p>
<p>This is a normal paragraph.</p>
</body>
</html>
p {
border: 1px solid black;
padding: 4px;
}
p.main {
position: absolute;
top: 70px;
left: 60px;
background-color: greenyellow;
}
Browser Output
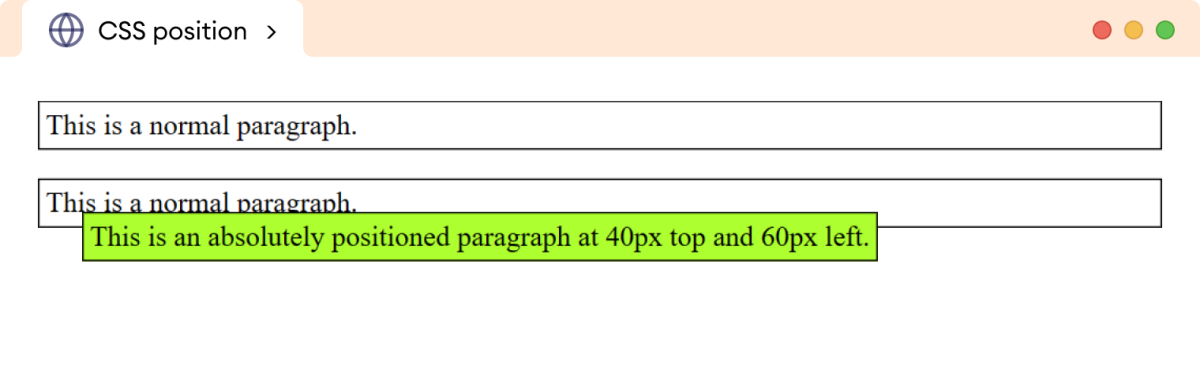
In the above example, the second paragraph doesn't have a positioned parent element, so it is positioned relative to the viewport (visible area of the browser).
Let's see an example to understand how the absolute
position
works with positioned parent element:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS position</title>
</head>
<body>
<p>This is a normal paragraph.</p>
<p class="main">
This is an absolutely positioned paragraph at 40px top and 60px
left.
</p>
<p>This is a normal paragraph.</p>
<!-- Creating an container -->
<div class="parent">
<p>This paragraph is inside the div element.</p>
<p class="main">
This is an absolutely positioned paragraph inside the div
element at 40px top and 60px left.
</p>
<p>This paragraph is inside the div element.</p>
</div>
</body>
</html>
p {
border: 1px solid black;
padding: 4px;
}
p.main {
position: absolute;
top: 70px;
left: 60px;
background-color: greenyellow;
}
div.parent {
position: relative;
background-color: orange;
border: 4px solid red;
}
Browser Output
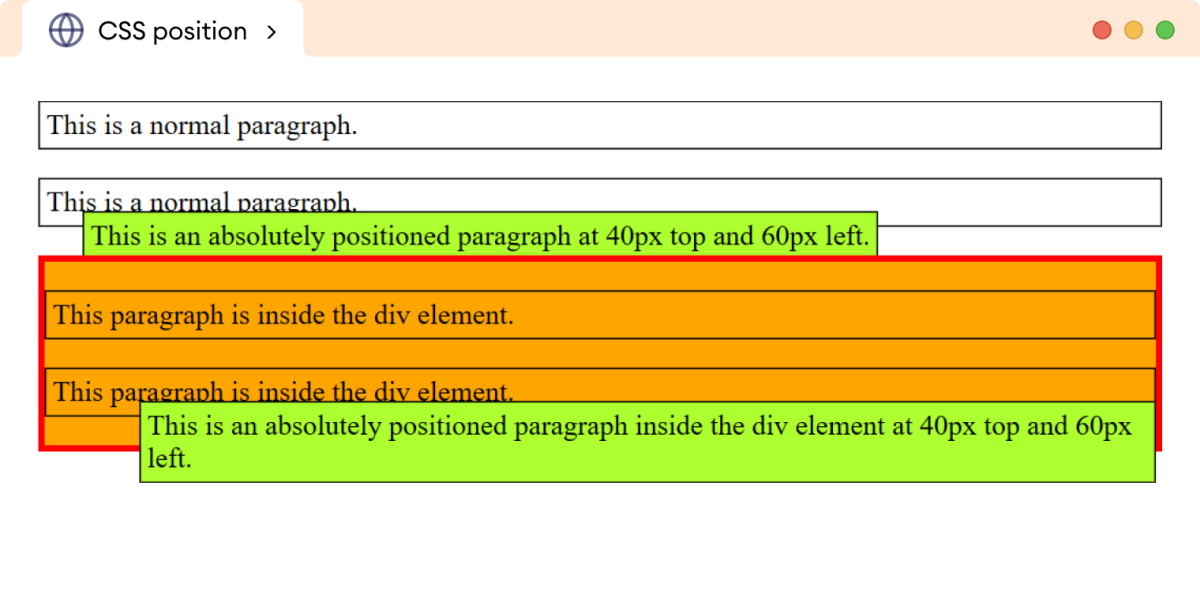
Here, even though both paragraphs of the main
class are given
the same styles, their positions are different. This is because the first
paragraph doesn't have a positioned parent element, so it is positioned
relative to the document (viewport).
On the other hand, the second paragraph has a parent
div
element with a relative
position value and is
hence positioned relative to the div
element.
Note: An absolutely positioned element loses its size and original space in the document flow.
CSS fixed Position
The fixed
value positions an element to remain fixed in the
same position, even when the page is scrolled. It is similar to the
absolute
value, but it remains relative to the viewport at all
times.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS position</title>
</head>
<body>
<p class="main">
This paragraph has a fixed position value at 10px top.
</p>
<p>This is a normal paragraph.</p>
<p>This is a normal paragraph.</p>
<p>This is a normal paragraph.</p>
<p>This is a normal paragraph.</p>
<p>This is a normal paragraph.</p>
<p>This is a normal paragraph.</p>
<p>This is a normal paragraph.</p>
</body>
</html>
p {
border: 2px solid black;
padding: 4px;
}
p.main {
position: fixed;
top: 10px;
background-color: greenyellow;
}
Browser Output
This parapraph has a fixed position value at 10px top.
This is a normal paragraph.
This is a normal paragraph.
This is a normal paragraph.
This is a normal paragraph.
This is a normal paragraph.
This is a normal paragraph.
This is a normal paragraph.
In the above example,
position: fixed;
top: 10px;
fixes the paragraph at the 10px
distance from the
top
in the webpage. The paragraph doesn't scroll with the other
content in the document.
CSS sticky Position
The sticky
value positions the element as a combination of
relative
and fixed
values.
The sticky
position behaves like
relative
positioning until the element reaches a certain scroll
point on the screen. After that, the element sticks to the top of the
viewport like a fixed
element.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS position</title>
</head>
<body>
<p class="main">
This paragraph has a fixed position value at 10px top.
</p>
<p>This is a normal paragraph.</p>
<p>This is a normal paragraph.</p>
<p>This is a normal paragraph.</p>
<p>This is a normal paragraph.</p>
<p>This is a normal paragraph.</p>
<p>This is a normal paragraph.</p>
<p>This is a normal paragraph.</p>
</body>
</html>
p {
border: 2px solid black;
padding: 4px;
}
p.main {
position: fixed;
top: 10px;
background-color: greenyellow;
}
Browser Output
This is a normal paragraph.
This parapraph is set sticky position at top 10px.
This is a normal paragraph.
In the above example, the paragraph remains scrollable until it reaches to
10px
distance from the top
of the viewport. After
that, it remains in the sticky
position.