CSS 3D transform property allows to rotate, translate, and scale elements in a three-dimensional plane. For example,
Here, we can see the differences between rotation in a two-dimensional and three-dimensional plane.
CSS 3D Transforms Methods
CSS 3D transform
property allows us to use the following transformation methods:
translate3d()
rotate3d()
scale3d()
To visualize the effect of 3D transform methods, we need to use a perspective
property. This property allows us to define the virtual distance between the viewer and 3D transformed elements.
The syntax of the perspective
property is:
perspective: length_value;
The perspective
property takes a length value
, which indicates the distance from the viewer to the 3D plane containing the transformed element.
We will look at each of the methods briefly.
translate3d() Method
The translate3d()
method moves an element in a three dimensional space along the x, y, and z-axes.
The syntax of translate3d()
method is:
transform: translate3d(x-value,y-value,z-value)
Here, the x-value
, y-value
, and z-value
represent the distance that the element moves along x, y and z axes from the original position.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 3D Translate</title>
</head>
<body>
<div class="original">
<div class="translate"></div>
</div>
</body>
</html>
/* styles original div */
div.original {
width: 100px;
height: 100px;
border: 4px solid black;
perspective: 800px;
}
/* styles div with translate class */
div.translate {
width: 100px;
height: 100px;
background-color: orange;
transform: translate3d(50px, 20px, 30px);
}
Browser Output
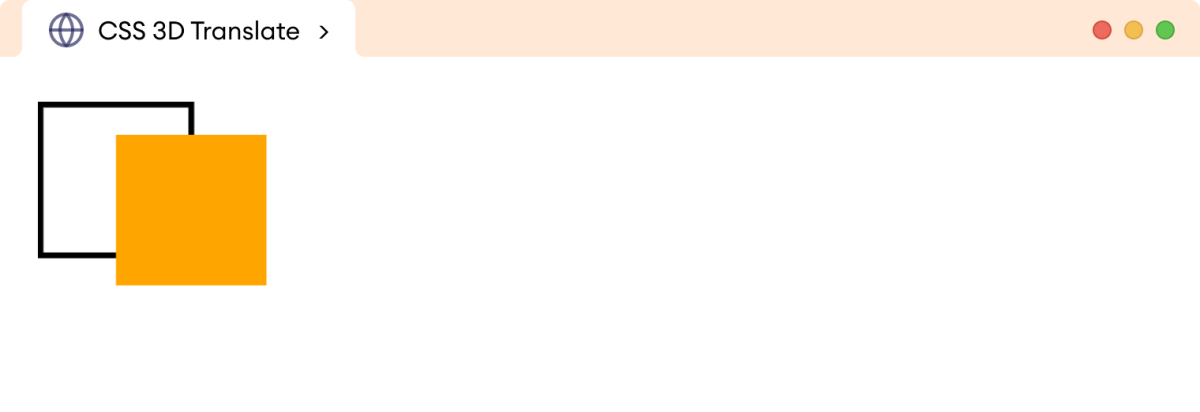
In the above example,
transform: translate3d(50px, 20px, 30px)
moves the inner div
element by 50
pixels horizontally, 20
pixels vertically and 30
pixels outward from the initial position.
Note: The negative value moves the element in the opposite direction.
The translateX() , translateY(), and translateZ() Methods
The translateX()
method moves the element along the x-axis in three-dimensional space. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 3D TranslateX</title>
</head>
<body>
<div class="original">
<div class="translate"></div>
</div>
</body>
</html>
/* styles original div */
div.original {
width: 100px;
height: 100px;
border: 4px solid black;
perspective: 800px;
}
/* styles div with translate class */
div.translate {
width: 100px;
height: 100px;
background-color: orange;
transform: translateX(60px);
}
Browser Output
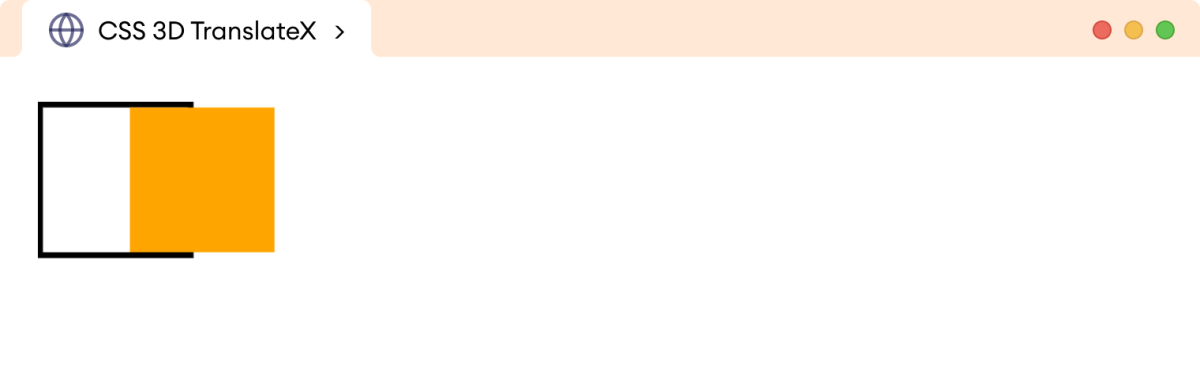
In the above example,
transform: translateX(60px)
moves the inner div
element by 60
pixels to the right horizontally in three-dimensional space from its original position.
The translateY()
method moves the element along the y-axis in three-dimensional space. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 3D TranslateY</title>
</head>
<body>
<div class="original">
<div class="translate"></div>
</div>
</body>
</html>
/* styles original div */
div.original {
width: 100px;
height: 100px;
border: 4px solid black;
perspective: 800px;
}
/* styles div with translate class */
div.translate {
width: 100px;
height: 100px;
background-color: orange;
transform: translateY(30px);
}
Browser Output
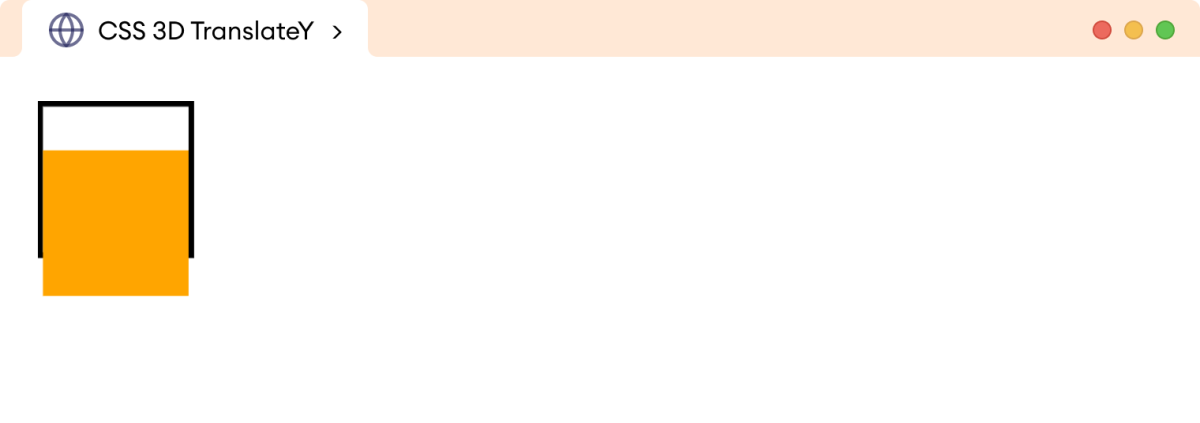
In the above example,
transform: translateY(40px)
moves the inner div
element by 40
pixels to the bottom vertically in three-dimensional space from its original position.
The translateZ()
method moves the element along the z-axis in three-dimensional space. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 3D TranslateZ</title>
</head>
<body>
<div class="original">
<div class="translate"></div>
</div>
</body>
</html>
/* styles original div */
div.original {
width: 100px;
height: 100px;
border: 4px solid black;
perspective: 80px;
}
/* styles div with translate class */
div.translate {
width: 100px;
height: 100px;
background-color: orange;
transform: translateZ(-20px);
}
Browser Output
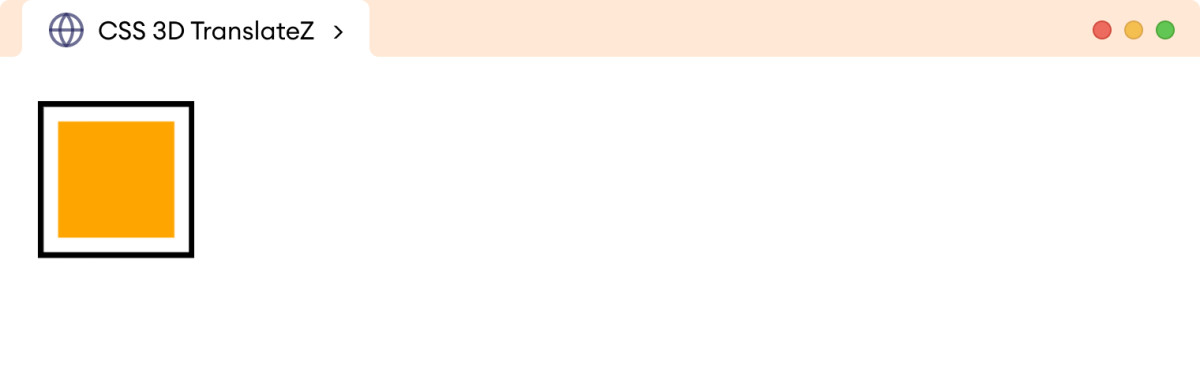
In the above example,
transform: translateZ(-20px)
moves the inner div
element by 20
pixels inward, away from the viewer in three dimensional space from its original position.
rotate3d() Method
The rotate3d()
method rotates an element in the three-dimensional plane to the clockwise or anti-clockwise direction.
The syntax of rotate3d()
method is:
transform: rotate3d(x, y, z, angle)
Here,
x
,y
, andz
represent the axis of rotation ; 1 means rotation occurs in that axis, and 0 means no rotation along that axisangle
represents the degree of rotation
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 3D Rotate</title>
</head>
<body>
<div class="original">
<div class="rotate"></div>
</div>
</body>
</html>
/* styles original div */
div.original {
width: 100px;
height: 100px;
margin: 50px;
border: 4px solid black;
perspective: 80px;
}
/* styles div with rotate class */
div.rotate {
width: 100px;
height: 100px;
background-color: orange;
transform: rotate3d(1, 1, 1, 30deg);
}
Browser Output
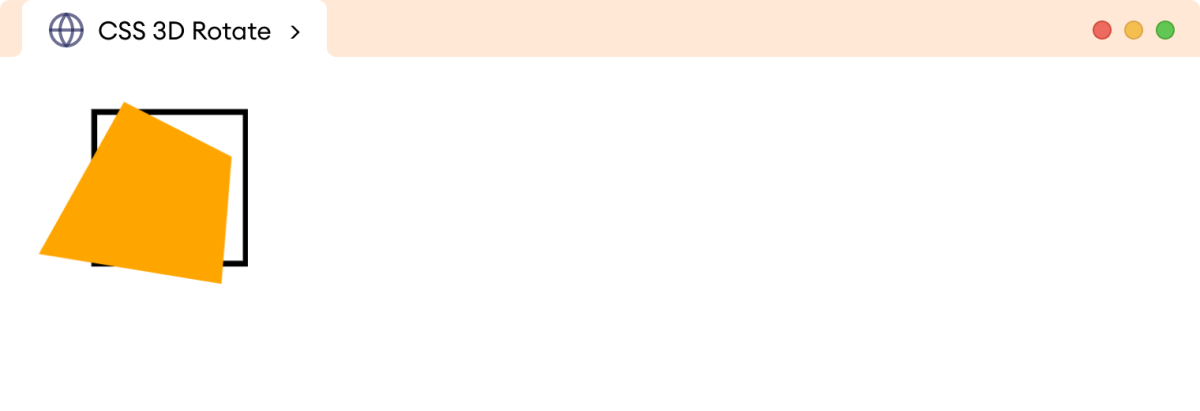
In the above example,
transform: rotate3d(1, 1, 1, 30deg)
rotates the inner div
element by 30
degrees along all three axes in clockwise direction.
Note: The negative angle rotates the element in anti-clockwise direction.
The rotateX() , rotateY(), and rotateZ() Methods
The rotateX()
method rotates an element along the x-axis in three-dimensional space. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 3D RotateX</title>
</head>
<body>
<div class="original">
<div class="rotate"></div>
</div>
</body>
</html>
/* styles original div */
div.original {
width: 100px;
height: 100px;
border: 4px solid black;
perspective: 80px;
}
/* styles div with rotate class */
div.rotate {
width: 100px;
height: 100px;
background-color: orange;
transform: rotateX(30deg);
}
Browser Output
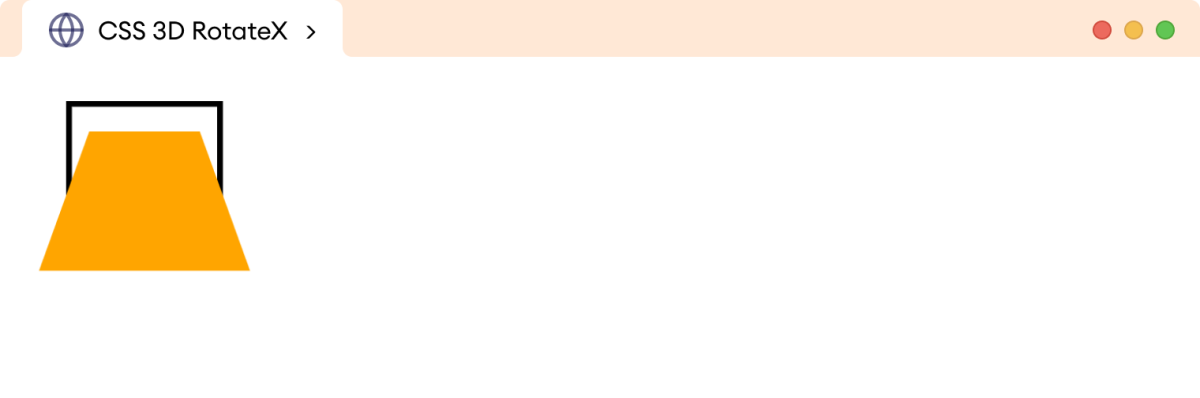
In the above example,
transform: rotateX(30deg)
rotates the inner div
element by 30
degrees in clockwise direction along the x-axis in 3D space.
The rotateY()
method rotates an element along the y-axis in three-dimensional space. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 3D RotateY</title>
</head>
<body>
<div class="original">
<div class="rotate"></div>
</div>
</body>
</html>
/* styles original div */
div.original {
width: 100px;
height: 100px;
border: 4px solid black;
perspective: 80px;
margin: 50px;
}
/* styles div with rotate class */
div.rotate {
width: 100px;
height: 100px;
background-color: orange;
transform: rotateY(30deg);
}
Browser Output
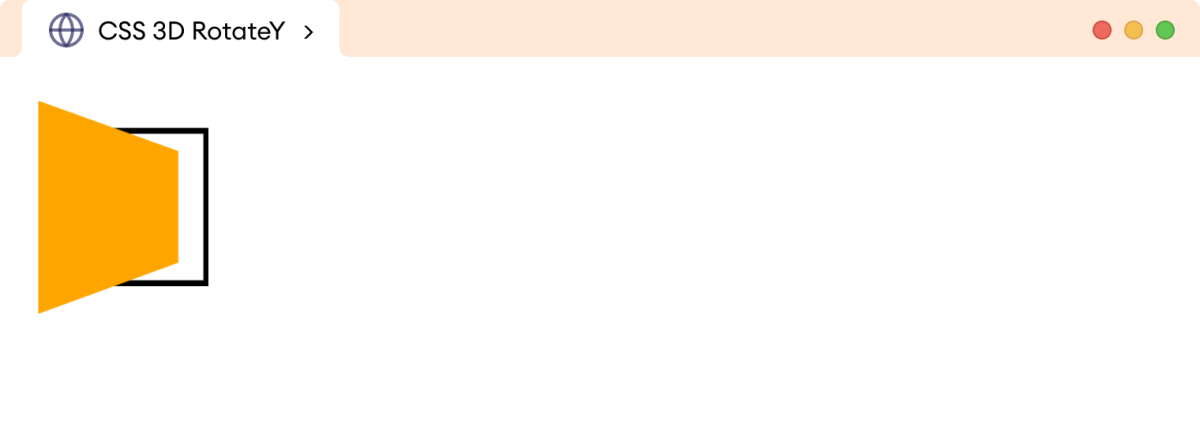
In the above example,
transform:rotateY(30deg)
rotates the inner div
element by 30
degrees in a clockwise direction along the y-axis in 3D space.
The rotateZ()
method rotates an element along the z-axis in three-dimensional space. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 3D RotateZ</title>
</head>
<body>
<div class="original">
<div class="rotate"></div>
</div>
</body>
</html>
/* styles original div */
div.original {
width: 100px;
height: 100px;
margin: 50px;
border: 4px solid black;
perspective: 80px;
}
/* styles div with rotate class */
div.rotate {
width: 100px;
height: 100px;
background-color: orange;
transform: rotateZ(30deg);
}
Browser Output
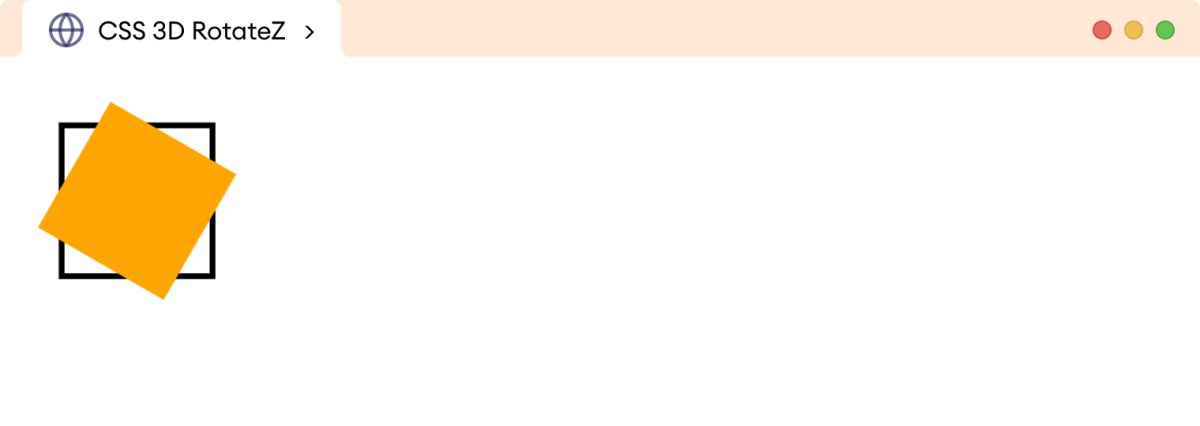
In the above example,
transform: rotateZ(30deg)
rotates the inner div
element by 30
degrees in a clockwise direction along the z-axis in 3D space.
scale3d() Method
The scale3d()
method scales the element i.e increases or decreases the size of an element in three-dimensional space along all the axes. It changes the width, height, and depth of the element.
The syntax of scale3d()
is:
transform: scale3d(x, y, z)
Here, x,
y
, and z
are the scaling factors to scale the element along the x-axis, y-axis, and z-axis, respectively.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 3D Scale</title>
</head>
<body>
<div class="original">
<div class="scale"></div>
</div>
</body>
</html>
/* styles original div */
div.original {
width: 100px;
height: 100px;
margin: 50px;
border: 4px solid black;
perspective: 800px;
}
/* styles div with scale class */
div.scale {
width: 100px;
height: 100px;
background-color: orange;
transform: scale3d(1, 2, 5) rotateX(45deg);
}
Browser Output
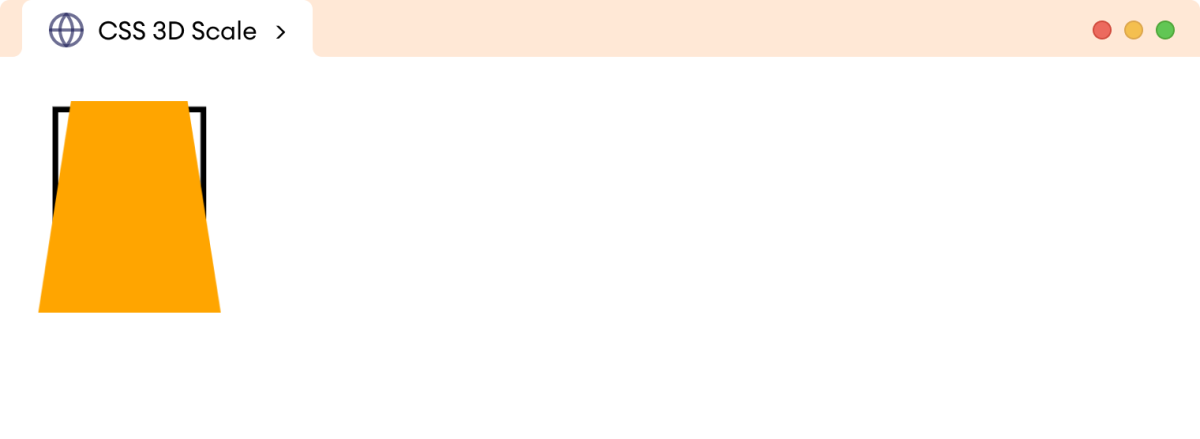
In the above example,
transform: scale3d(1, 2, 5)
scales the inner div
element by keeping its width the same, doubles the height and increases the depth by five times.
Note: The rotateX()
function enhances the visual effect of 3D scaling.
The scaleX() , scaleY() ,and scaleZ() Method
The scaleX()
method scales the element horizontally keeping all other axes constant. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 3D ScaleX</title>
</head>
<body>
<div class="original">
<div class="scale"></div>
</div>
</body>
</html>
/* styles original div */
div.original {
width: 100px;
height: 100px;
margin: 50px;
border: 4px solid black;
perspective: 80px;
}
/* styles div with scale class */
div.scale {
width: 100px;
height: 100px;
background-color: orange;
transform: scaleX(2);
}
Browser Output
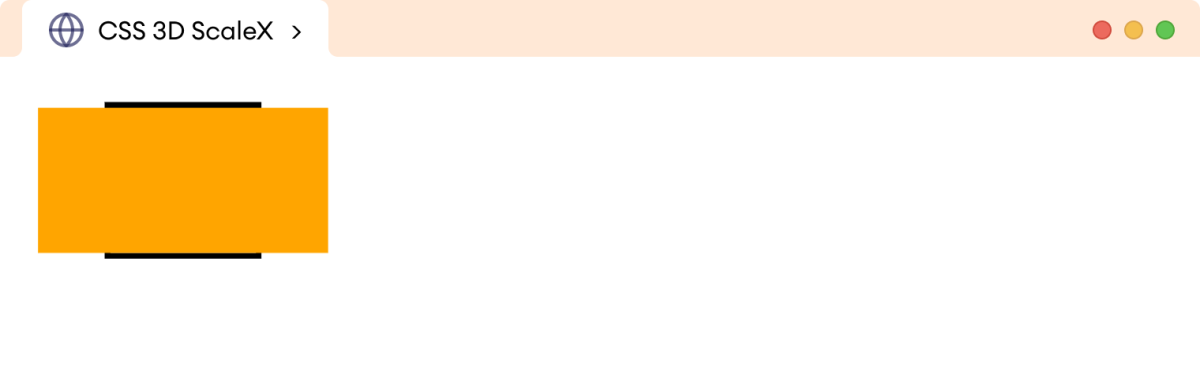
In the above example,
transform: scaleX(2)
scales the inner div
element to twice its width along x-axis keeping the height and depth unchanged.
The scaleY()
method scales the element vertically keeping all other axes constant. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 3D ScaleY</title>
</head>
<body>
<div class="original">
<div class="scale"></div>
</div>
</body>
</html>
/* styles original div */
div.original {
width: 100px;
height: 100px;
margin: 50px;
border: 4px solid black;
perspective: 80px;
}
/* styles div with scale class */
div.scale {
width: 100px;
height: 100px;
background-color: orange;
transform: scaleY(1.5);
}
Browser Output
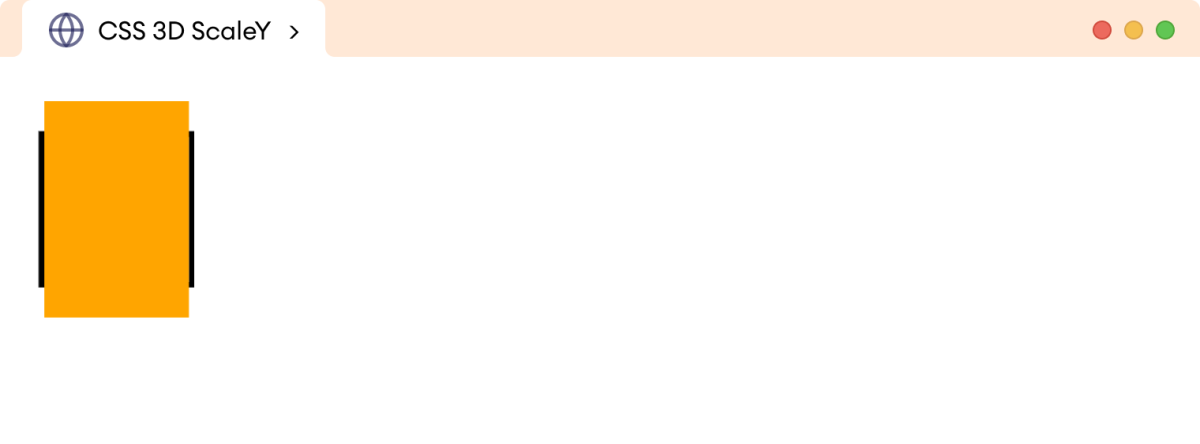
In the above example,
transform: scaleY(1.5)
scales the inner div
element to 1.5
times of its height along the y-axis keeping the width and depth unchanged.
The scaleZ()
method scales the element along the z-axis keeping all other axes constant. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS 3D ScaleZ</title>
</head>
<body>
<div class="original">
<div class="scale"></div>
</div>
</body>
</html>
/* styles original div */
div.original {
width: 100px;
height: 100px;
margin: 50px;
border: 4px solid black;
perspective: 800px;
}
/* styles div with scale class */
div.scale {
width: 100px;
height: 100px;
background-color: orange;
transform: scaleZ(10) rotateX(45deg);
}
Browser Output
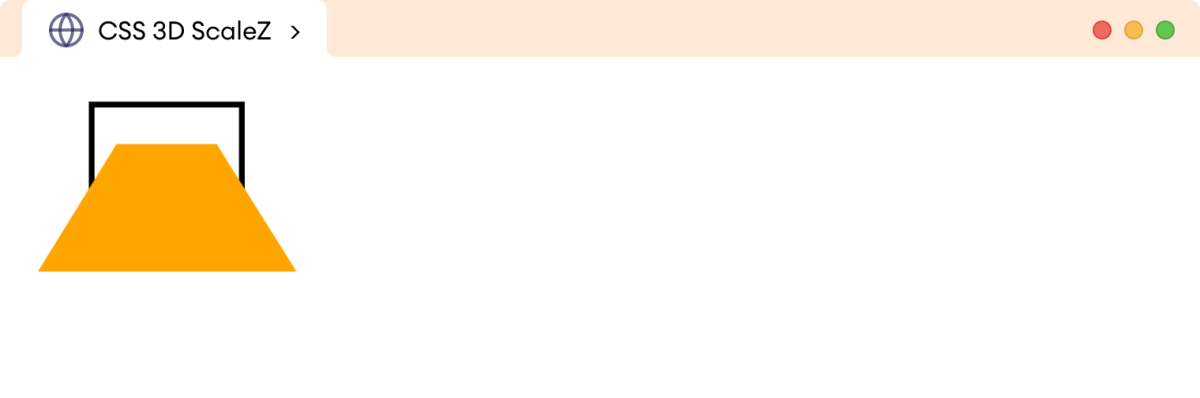
In the above example,
transform: scaleZ(10)
scales the inner div
element to 10
times
its depth along the z-axis keeping the width and height unchanged.
Note: The scaleZ()
function scales along the z-axis only. Hence, we need to combine it with other transformation methods to visualize the complete effect of transformation.