CSS selectors are used to select the HTML elements that are to be styled by CSS. For example,
h1 {
color: red;
}
Browser Output
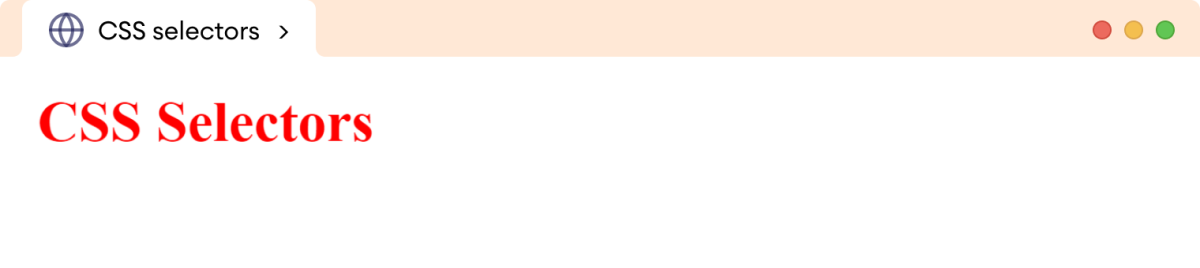
Here, the h1
is the selector that selects all the h1
elements of our document and changes their color
to red
.
Types of Selector
There are the following different types of selectors in CSS.
- Element selector
- Id selector
- Class selector
- Universal selector
- Group selector
- Attribute selector
Now, let's learn about them in detail.
Element Selector
The element selector selects HTML elements (p
, div
, h1
, etc) and applies CSS to them. For example,
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS selectors</title>
</head>
<body>
<h1>Heading</h1>
<p>This is a paragraph.</p>
</body>
</html>
h1 {
color: red;
}
p {
color: orange;
}
Browser Output
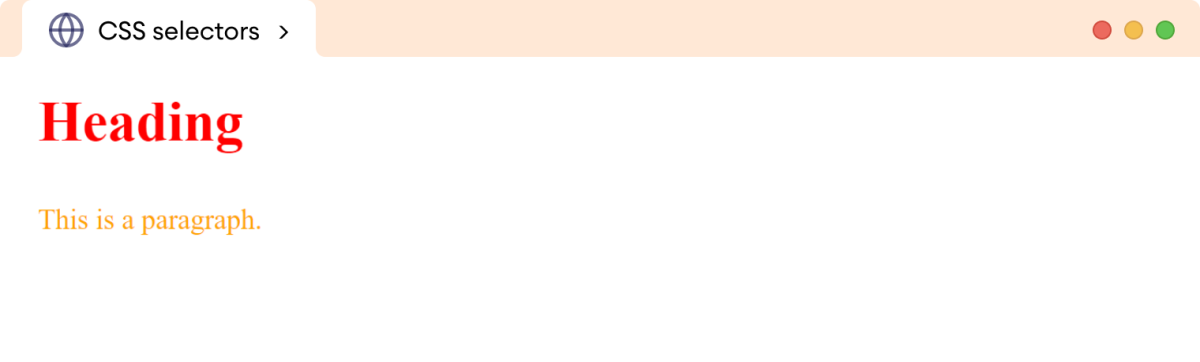
In the above example, the element selector
h1
selects allh1
elements and styles theircolor
tored
p
selects allp
elements and styles theircolor
toorange
Note: The element selector is also referred to as a tag selector because it selects HTML elements based on their tag names.
ID Selector
The id selector selects the HTML element with a unique identifier (id) and adds CSS to it.
The id selector is specified using the hash (#
) character, followed by the id of the element.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS selectors</title>
</head>
<body>
<p>First Paragraph</p>
<p id="second-paragraph">Second Paragraph</p>
<p>Third Paragraph</p>
</body>
</html>
#second-paragraph {
color: red;
}
Browser Output
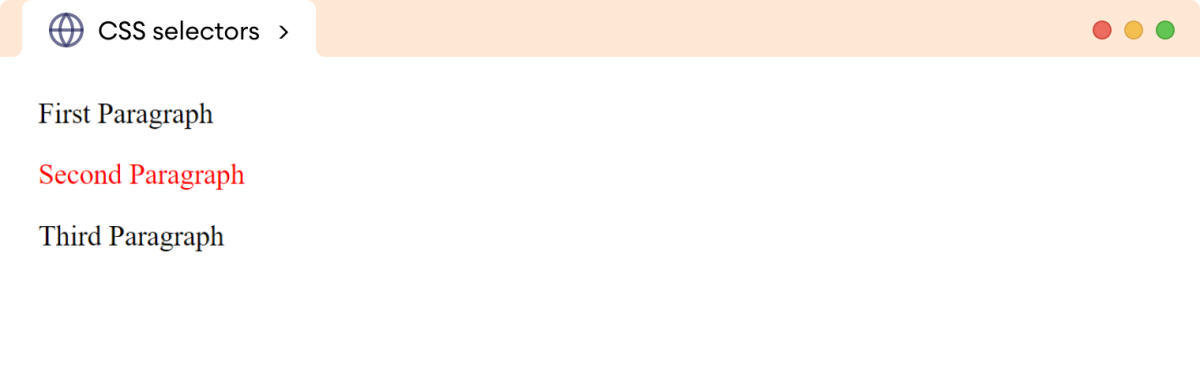
Here,
#
- id selectorsecond-paragraph
- the name of the id
The id selector #second-paragraph
selects the second paragraph and styles the text color to red
.
Note: The id selector is unique and selects one unique element.
Class Selector
The class selector selects the HTML element using the class
attribute and applies CSS to it.
The class selector is specified using the period (.
) character, followed by the class name.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS selectors</title>
</head>
<body>
<h2>Section First</h2>
<p class="first-paragraph">This is the first paragraph.</p>
<p>This is the second paragraph.</p>
<h2>Section Second</h2>
<p class="first-paragraph">This is the first paragraph.</p>
<p>This is the second paragraph.</p>
</body>
</html>
.first-paragraph {
background-color: orange;
}
Browser Output
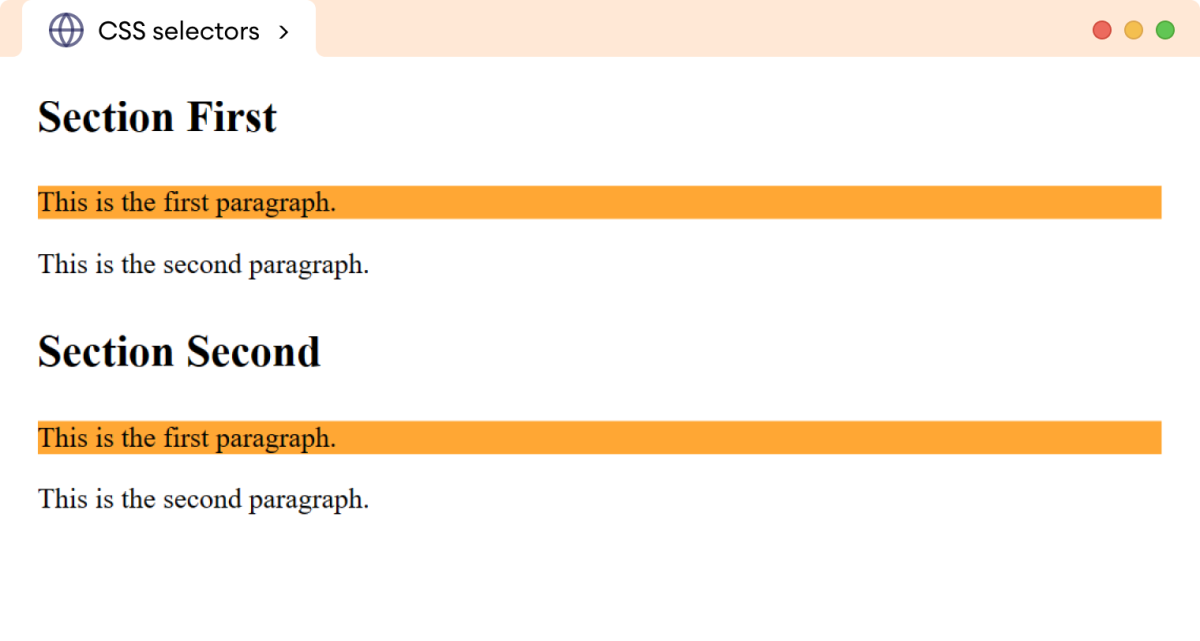
Here,
.
- class selectorfirst-paragraph
-name of the class
The class selector .first-paragraph
selects all the paragraphs having the first-paragraph
class name and styles background-color
to orange
.
Universal Selector
The universal selector selects every single HTML element on the page. It is written using the asterisk ( *
) character.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS selectors</title>
</head>
<body>
<h1>Heading</h1>
<p>First Paragraph</p>
<p>Second Paragraph</p>
</body>
</html>
* {
color: red;
}
Browser Output
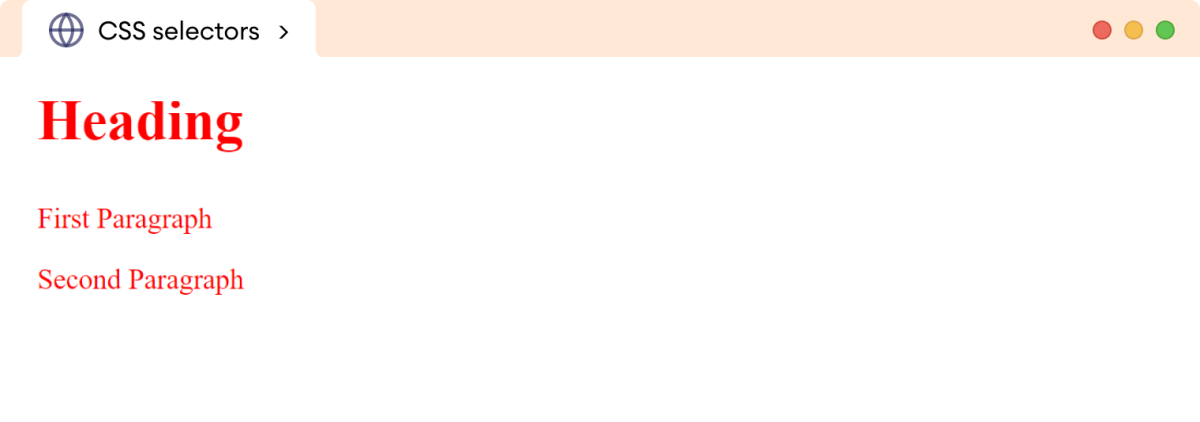
In the above example, the universal selector *
selects all the HTML elements and applies the red
color.
Note: The universal selector is also referred to as the wildcard selector.
#group-selector Group Selector
The group selector allows you to select multiple elements and apply the same style to all of them.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS selectors</title>
</head>
<body>
<h1>Heading</h1>
<p>This is a paragraph.</p>
</body>
</html>
h1, p {
color: blue;
}
Browser Output
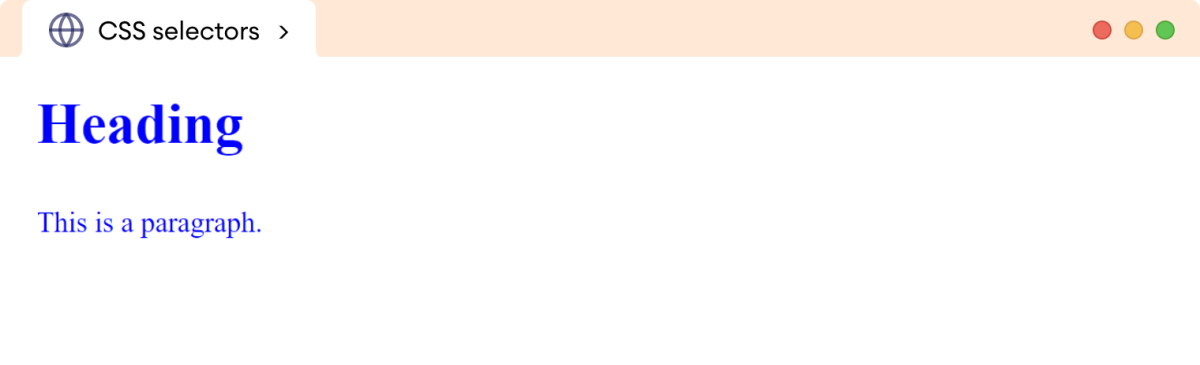
Here, the code applies CSS styling to all <h1>
and <p>
elements. Notice that we have used ,
to separate the HTML elements..
Attribute Selector
The attribute selector selects elements based on specific attribute values.
The syntax for the attribute selector is:
Element[attribute]
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS selectors</title>
</head>
<body>
<p class="first">This is a first paragraph.</p>
<p>This paragraph doesn't have a class attribute.</p>
<p class="third">This is a third paragraph.</p>
<p class="fourth">This is a fourth paragraph.</p>
</body>
</html>
p[class] {
background-color: orange;
}
p[class="third"] {
color: blue;
}
Browser Output
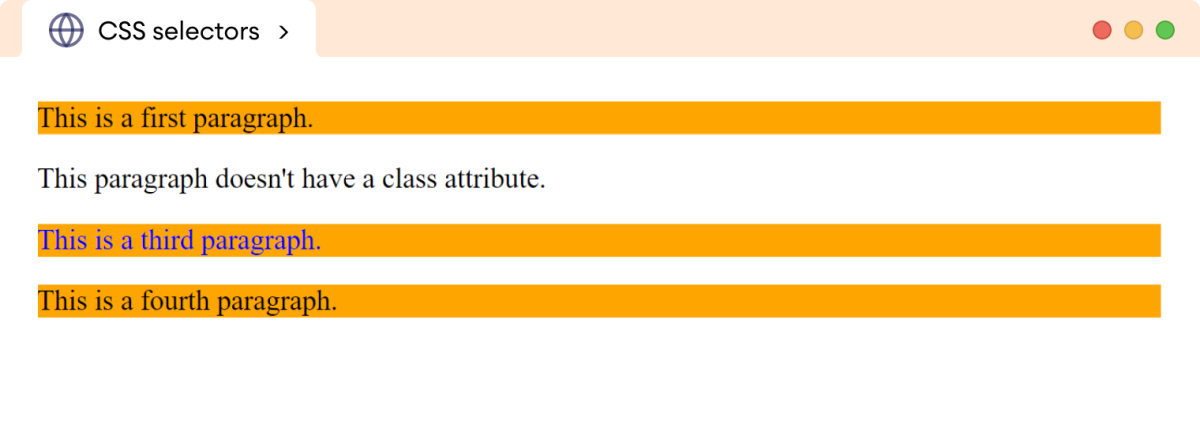
In the above example, the attribute selector
p[class]
selects allp
elements having theclass
attribute and styles their background color tored
.p[class="third"]
selects allp
elements with the.third
class name and styles their color toblue
.
Note: This selector only selects an element if a specified given attribute exists.