The CSS box-shadow
property is used to add shadows to elements. For example,
h1 {
box-shadow: 12px 12px 8px purple;
background-color: greenyellow;
}
Browser Output
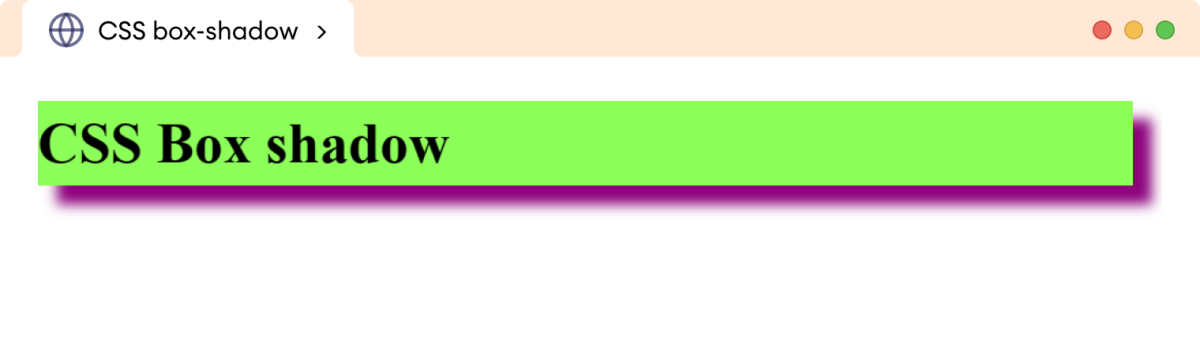
Here, the box-shadow
property adds a purple
shadow to the content of the h1
element.
The box shadow is also known as the drop shadow.
CSS box-shadow syntax
The syntax for the box-shadow
property is as follows,
box-shadow: horizontal-offset vertical-offset blur-radius spread-radius color | inset | initial | inherit | none ;
Here,
horizontal-offset
: specifies the horizontal position of the shadow relative to the elementvertical-shadow
: specifies the vertical position of the shadow relative to the elementblur-radius
: specifies the value to control the blurriness of the shadowspread-radius
: specifies the value to expand or contract the shadowcolor
: specifies the color of the shadow, which can be in any format such as named color, hexadecimal color,RGB
, orHSL
inset
: puts the shadow inside the element, the shadow is created outside of the element by defaultinitial
: sets the value to the defaultinherit
: inherits the value from its parent elementnone
: specifies the default value, no shadow
Note: The box-shadow
property needs the value of horizontal-shadow
and vertical-shadow,
while others are optional.
CSS box-shadow: Adding Horizontal Shadow
The horizontal shadow can be adjusted using the horizontal-offset
value in the box-shadow
property. The horizontal-offset
value can take a positive and negative value.
In the horizontal-offset
value,
- positive value creates a shadow in the right direction
- negative value creates a shadow in the left direction
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS box-shadow</title>
</head>
<body>
<h1>Horizontal Shadows</h1>
<p>
The positive value of the horizontal offset creates a shadow in the
right direction.
</p>
<h2 class="positive-value">box-shadow: 20px 10px orange;</h2>
<p>
The negative value of the horizontal offset creates a shadow in the
left direction.
</p>
<h2 class="negative-value">box-shadow: -20px 10px orange;</h2>
</body>
</html>
h2 {
background-color: greenyellow;
}
h2.positive-value {
/* horizontal-offset | vertical-offset | shadow color */
box-shadow: 20px 10px orange;
}
h2.negative-value {
/* horizontal-offset | vertical-offset | shadow color */
box-shadow: -20px 10px orange;
}
Browser Output
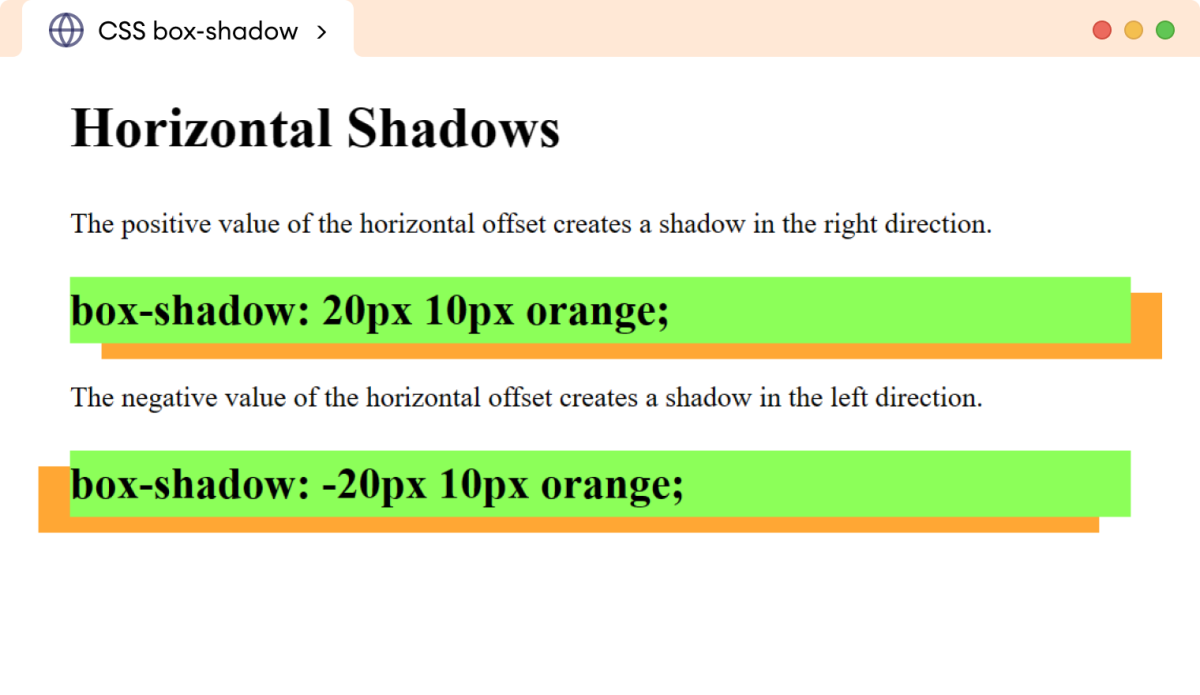
CSS box-shadow: Adding Vertical Shadow
The vertical shadow can be adjusted using the vertical-offset
value in the box-shadow
property. The vertical-offset
value can take a positive and negative value.
In the vertical-offset
value,
- positive value creates a shadow in the bottom direction
- negative value creates a shadow in the top direction
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS box-shadow</title>
</head>
<body>
<h1>Vertical Shadows</h1>
<p>
The positive value of the vertical offset creates a shadow in the
bottom direction.
</p>
<h2 class="positive-value">box-shadow: 10px 10px orange;</h2>
<p>
The negative value of the vertical offset creates a shadow in the
top direction.
</p>
<h2 class="negative-value">box-shadow: 10px -10px orange;</h2>
</body>
</html>
h2 {
background-color: greenyellow;
}
h2.positive-value {
/* horizontal-offset | vertical-offset | shadow color */
box-shadow: 10px 10px orange;
}
h2.negative-value {
/* horizontal-offset | vertical-offset | shadow color */
box-shadow: 10px -10px orange;
}
Browser Output
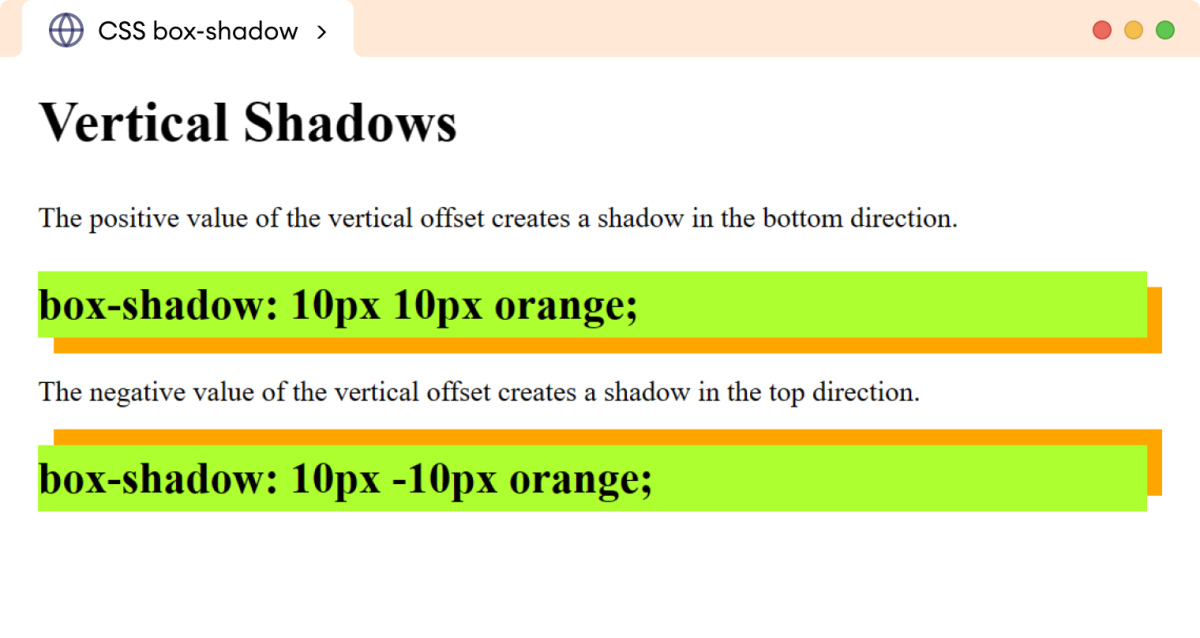
CSS box-shadow: Adding a blur radius
The blur-radius
value of the box-shadow
property is used to blur the shadow. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS box-shadow</title>
</head>
<body>
<h1>Blurry Shadows</h1>
<p>This shadow has a blur value of 5px.</p>
<h2 class="blur-5px">box-shadow: 10px 10px 5px orange;</h2>
<p>This shadow has a blur value of 15px.</p>
<h2 class="blur-10px">box-shadow: 10px 10px 15px orange;</h2>
</body>
</html>
h2 {
background-color: greenyellow;
}
h2.blur-5px {
/* horizontal-offset | vertical-offset | blur-radius | shadow color */
box-shadow: 10px 10px 5px orange;
}
h2.blur-10px {
/* horizontal-offset | vertical-offset | blur-radius | shadow color */
box-shadow: 10px 10px 15px orange;
}
Browser Output
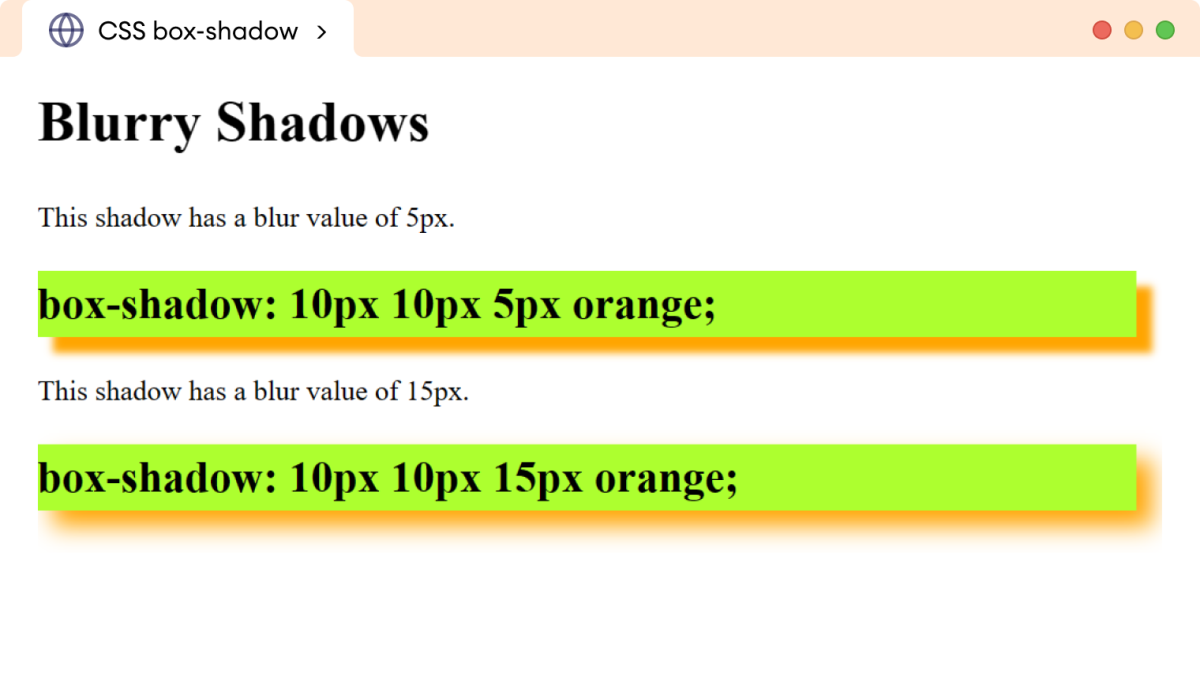
Note: The default value of blur-radius
is 0 which means no blur. The larger the value, the more blurred the shadow appears.
CSS box-shadow: Adding spread radius
The spread-radius
value of the box-shadow
property is used to increase or decrease the size of the shadow.
The shadow size,
- increases with the positive value
- decreases with the negative value
- remains the same size at 0 (default value)
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS box-shadow</title>
</head>
<body>
<h1>Adding spread radius</h1>
<p>This shadow has a 5px value of blur-radius and spread-radius.</p>
<h2 class="spread-5px">box-shadow: 10px 10px 5px 5px black;</h2>
<p>This shadow has a 5px blur-radius and 10px spread-radius.</p>
<h2 class="spread-10px">box-shadow: 10px 5px 10px 10px black;</h2>
<p>This shadow has 5px value of spread-radius and no blur-radius.</p>
<h2 class="no-blur">box-shadow: 10px 5px 0px 5px black;</h2>
</body>
</html>
h2 {
background-color: greenyellow;
}
h2.spread-5px {
/* horizontal-offset | vertical-offset | blur-radius | spread-radius | shadow color */
box-shadow: 10px 10px 5px 5px black;
}
h2.spread-10px {
/* horizontal-offset | vertical-offset | blur-radius | spread-radius | shadow color */
box-shadow: 10px 5px 10px 10px black;
}
h2.no-blur {
/* horizontal-offset | vertical-offset | blur-radius | spread-radius | black default color */
box-shadow: 10px 5px 0px 5px;
}
Browser Output
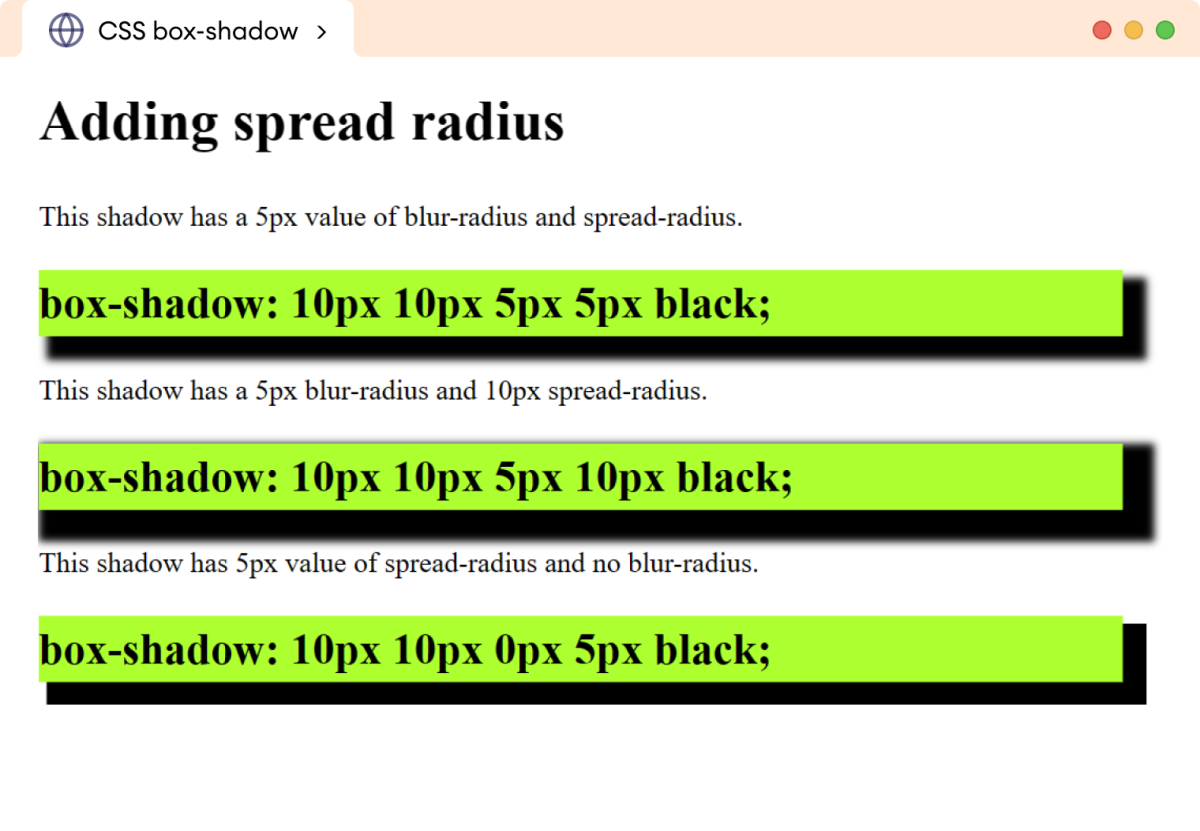
Note: It's important to specify the value for the blur-radius
before providing the value for the spread-radius
. Otherwise, the spread radius will be mistakenly interpreted as the blur radius value.
If we don't want to include a blur effect, we can simply set the blur-radius
to 0px.
Create even shadows
The shadows can be evenly distributed across all sides of the element. For this, we need to set the horizontal-offset
and vertical-offset
values to 0 and provide the blur-radius
and spread-radius
values.
This causes the shadow to be spread out evenly on all sides of the element.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS box-shadow</title>
</head>
<body>
<h1>Evenly Distributed Shadows</h1>
<h2 class="first-shadow">box-shadow: 0px 0px 5px 2px purple;</h2>
<h2 class="second-shadow">
box-shadow: 0px 0px 10px 5px purple;
</h2>
<h2 class="third-shadow">box-shadow: 0px 0px 15px 8px purple;</h2>
</body>
</html>
h2 {
border: 2px solid black;
margin-bottom: 50px;
}
h2.first-shadow {
box-shadow: 0px 0px 5px 2px purple;
}
h2.second-shadow {
box-shadow: 0px 0px 10px 5px purple;
}
h2.third-shadow {
box-shadow: 0px 0px 15px 8px purple;
}
Browser Output
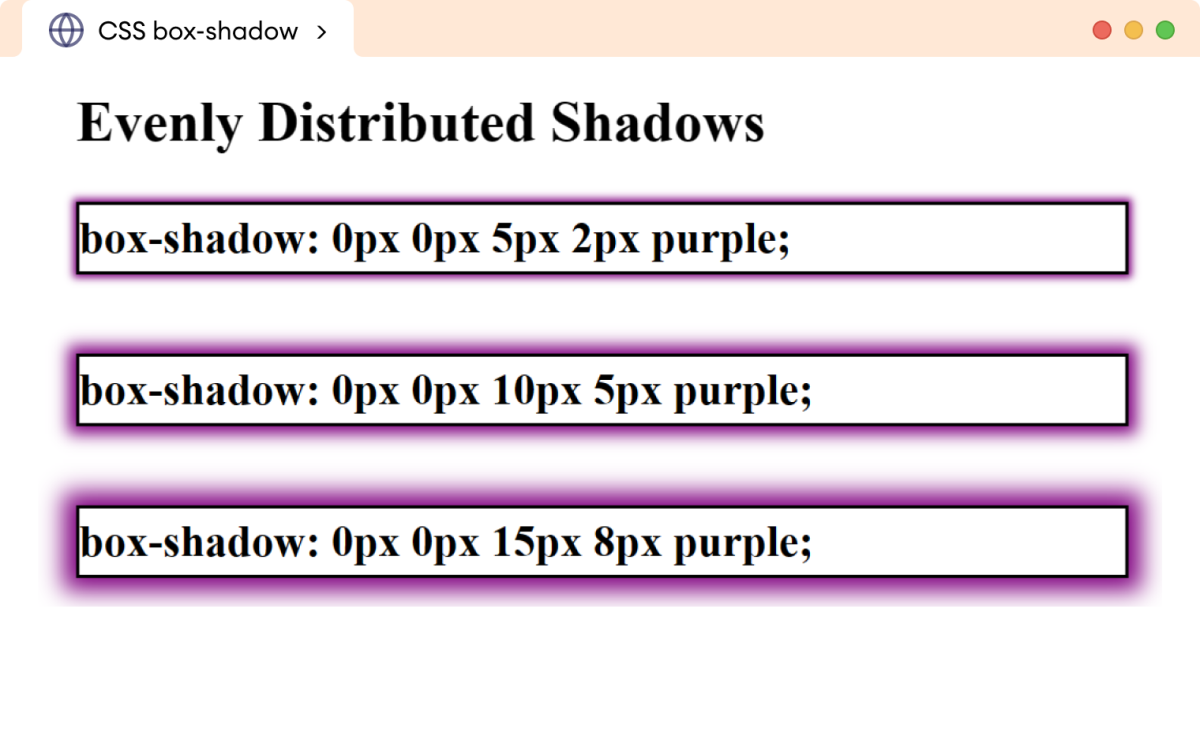
Create Inner Shadow
The inset
value of the box-shadow
property is used to create an inner shadow. By default, the shadow is created outside of the element.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS box-shadow</title>
</head>
<body>
<h1>The inset keyword</h1>
<h2 class="shadow-one">box-shadow: inset 0px 0px 5px 2px purple;</h2>
<h2 class="shadow-two">box-shadow: inset 0px 0px 10px 5px purple;</h2>
<h2 class="shadow-three">
box-shadow: inset 0px 0px 15px 8px purple;
</h2>
</body>
</html>
h2 {
border: 2px solid black;
margin-bottom: 50px;
}
h2.shadow-one {
box-shadow: inset 0px 0px 5px 2px purple;
}
h2.shadow-two {
box-shadow: inset 0px 0px 10px 5px purple;
}
h2.shadow-three {
box-shadow: inset 0px 0px 15px 8px purple;
}
Browser Output
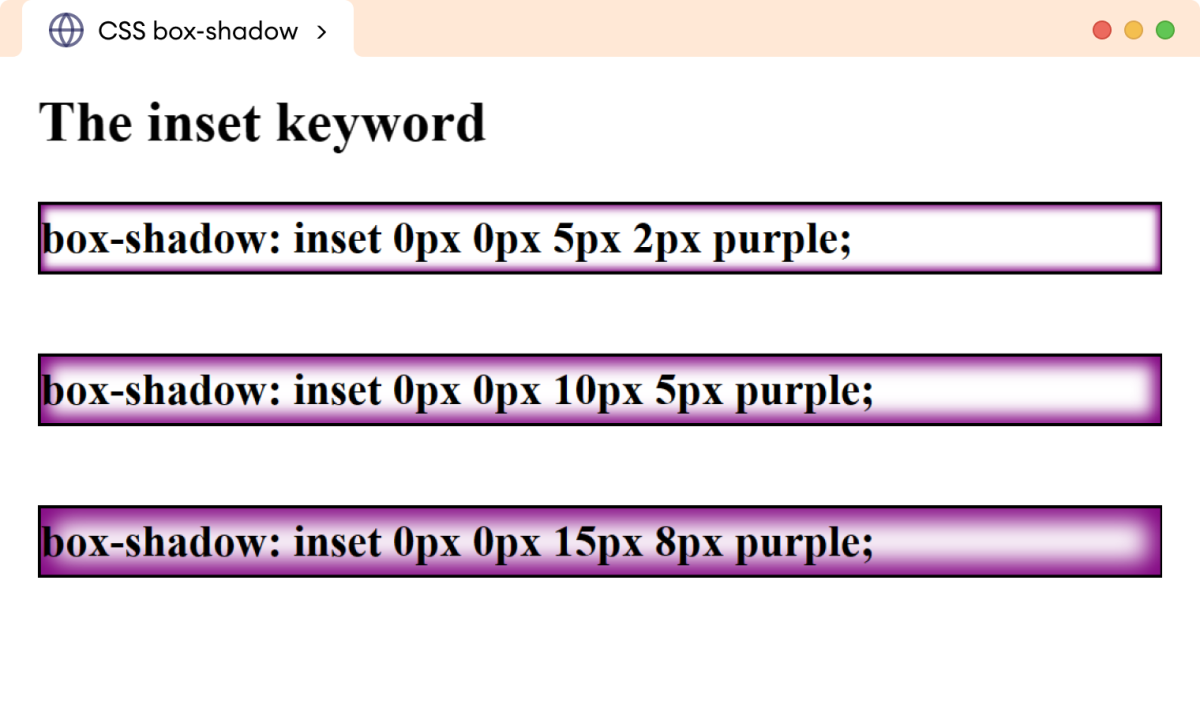
Adding Multiple Box Shadows
Multiple shadows can be applied to an element by separating them with commas. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS box-shadow</title>
</head>
<body>
<h1>Multiple Box Shadows</h1>
<p>box-shadow: 8px 8px purple, 16px 16px yellow, 22px 22px green;</p>
</body>
</html>
p {
box-shadow: 8px 8px purple, 16px 16px yellow, 22px 22px green;
background-color: greenyellow;
padding: 20px;
}
Browser Output
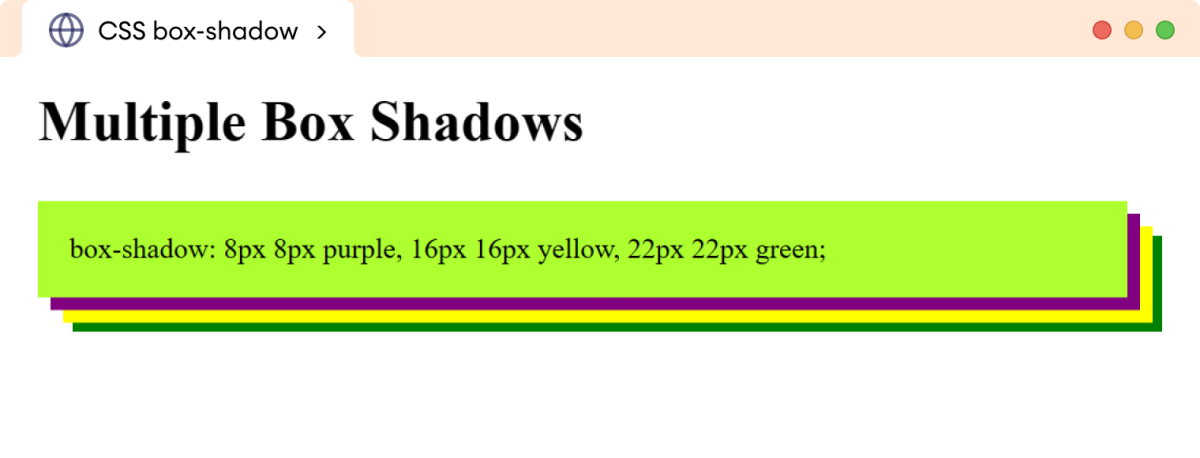
In the above example, each shadow represents a separate shadow layer and is stacked on top of each other as specified.