CSS @font-face
property allows us to specify a custom font for our webpage. For example,
@font-face {
font-family: "Roboto";
src: url("path/to/roboto-regular.woff2") format("woff2");
}
Here,
@font-face
: refers to the CSS property that allows custom fontsfont-family
: set the font-family toRoboto
src
: specifies the path and format for our custom font
Now we can use custom fonts in our webpage.
CSS @font-face Rule
The CSS @font-face
rule allows us to load custom fonts on a webpage. The custom font can be loaded from a remote server or a locally-installed font from the user's computer. For example,
@font-face {
font-family: "My Custom Font";
src: url("path/my-custom-font.woff") format("woff"),
url("path/my-custom-font.woff") format("woff");
}
Here,
font-family
: refers to thefont-family
to be loadedsrc
: specifies the URL and format for custom fonturl
: specifies the remote or locally hosted font pathformat
: refers to the format of the font file
Now, we can use My Custom Font
on our HTML page like the normal font.
h1{
font-family: "My Custom Font", sans-serif;
}
We use the custom font in the font-family
property only when we have defined the font using the @font-face
rule.
In the above example, we have defined multiple url
for our @font-face
property. If the first url
fails to load then the second url
will be rendered.
@font-face Example
Now, let's see an example of how @font-face
works,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>Custom font in CSS</title>
</head>
<body>
<h1>Custom fonts in CSS</h1>
<p>
We can use the @font-face property for using the custom fonts in
CSS.
</p>
</body>
</html>
/* allows to set Helvetica font*/
@font-face {
font-family: "Helvetica";
src: url("https://fonts.gstatic.com/s/helvetica/v1/7Auop_0qiz-aVz7u3PJLcA.eot?#iefix")
format("embedded-opentype"),
url("https://fonts.gstatic.com/s/helvetica/v1/7Auop_0qiz-aVz7u3PJLcA.woff2")
format("woff2"),
url("https://fonts.gstatic.com/s/helvetica/v1/7Auop_0qiz-aVz7u3PJLcA.woff")
format("woff");
}
h1, p {
/* sets the text to Helvetica font */
font-family: Helvetica;
}
Browser Output
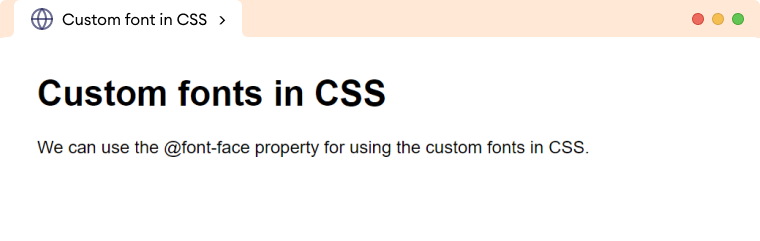
Alternative Techniques for Custom Font
We can also use the custom font in the following ways:
- using CSS
@import
rule - using HTML
<link>
tag
Using @import rule
We can use the CSS @import
rule to import the other remotely hosted fonts. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>Using import rule</title>
</head>
<body>
<h1>Using CSS @import rule</h1>
<p>
We can use the CSS @import property for loading the custom fonts in
CSS.
</p>
</body>
</html>
@import url("https://fonts.googleapis.com/css2?family=Roboto+Mono:wght@400;700&display=swap");
h1, p {
font-family: "Roboto Mono", monospace;
}
Browser Output
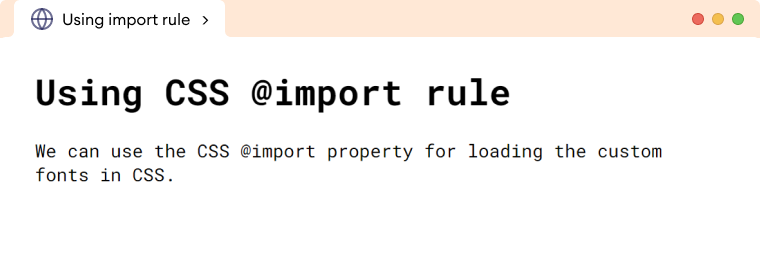
In the above example, we are using @import
to load the Roboto Mono
font from the Google Fonts.
Using <link> tag
We can use the <link>
tag to load the font just the way we used the @import
rule. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<link href="https://fonts.googleapis.com/css2?family=Roboto+Mono:wght@400;700&display=swap"
rel="stylesheet"/>
<title>Using Link tag</title>
</head>
<body>
<h1>Using link tag</h1>
<p>
We can use the HTML link tag for loading the custom fonts as well.
</p>
</body>
</html>
h1, p {
font-family: "Roboto Mono", monospace;
}
Browser Output
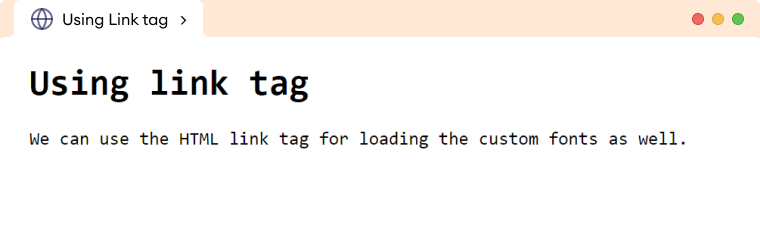
In the above example, we use <link>
tag to load Roboto Mono
font in the web page and use it with h1
and p
elements.
Different Font Format Types
We have different font format types having different features and capabilities.
WOFF/ WOFF2 (Web Open Font Format)
WOFF is a compressed font format that is commonly used on the webpage. It provides faster loading and is supported by most modern browsers.
SVG/SVGZ (Scalable Vector Graphics)
SVG is commonly used for graphics and logos that can be easily resized without losing quality. It can be used for fonts but it is not recommended as it lacks advanced typographic features. SVG fonts are not currently supported by major modern browsers.
EOT (Embedded Open Type)
EOT font format is used as a way to embed fonts on web pages. EOT was primarily used on the older versions of Internet Explorer and is currently not supported by modern browsers.
OTF/TTF (OpenType Font/ TrueType Font)
OTF and TTF fonts are the most commonly used fonts on web pages as they are supported by most modern web browsers.
Note:
- The custom fonts can impact the performance of web pages as the fonts need to be downloaded before they can be rendered, which can increase the page load time.
- The larger font sizes can also impact web performance due to longer download and render times.