The CSS float
property is used to specify how an element floats within a parent element. The element can float to the right
, left
, or none
within the container. For example,
div.box2 {
float: right;
background-color: greenyellow;
}
Browser Output
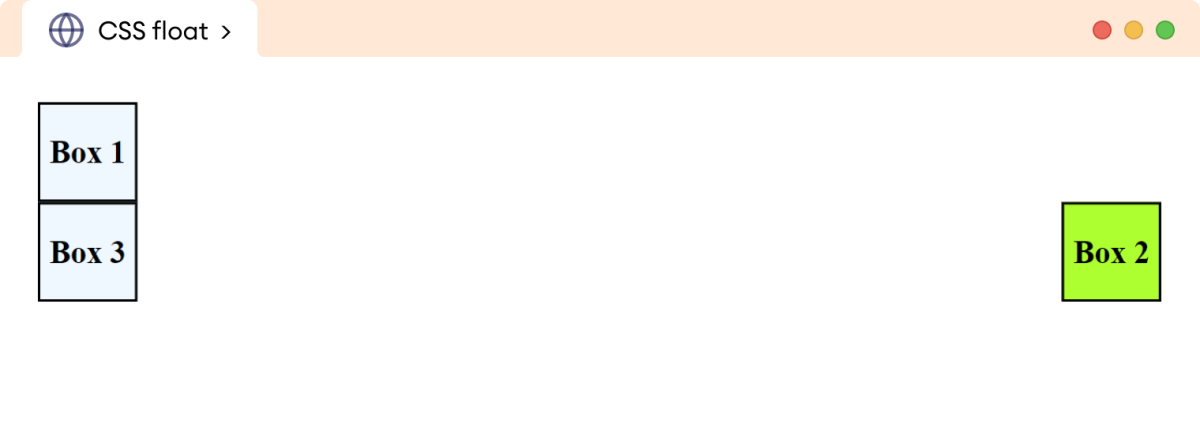
Here, the float
property moves the second div
element to the right
side of the document.
CSS float syntax
The syntax of the float
property is as follows,
float: none | left | right | initial | inherit;
Here,
none
: element doesn't float (default value)left
: element floats on the left side of its containing blockright
: element floats on the right side of its containing blockinitial
: the value is set to the default valueinherit
: inherits floating property from its parent element
Note: The float
property is different from the position
property.
The float
property is mainly used for text wrapping around elements. The position
property is used to position the element with precise control within the document.
Example: CSS float none Value
Let's see an example of the float
property with the none
value,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS float</title>
</head>
<body>
<div class="parent">
<img
src="https://www.seekpng.com/png/detail/109-1093815_doraemon-png-png-images-doraemon-png.png"
alt="A flying Doremon image"
/>
<p>
Doraemon is a beloved cartoon character adored by kids all over
the world. He is a robotic cat from the future who travels back
in time to help a young boy named Nobita. With his magical
gadgets and clever solutions, Doraemon always manages to turn
Nobita's troubles into exciting adventures.
</p>
</div>
</body>
</html>
img {
/* default value */
float: none;
width: 100px;
height: 120px;
}
div.parent {
border: 2px solid black;
}
Browser Output
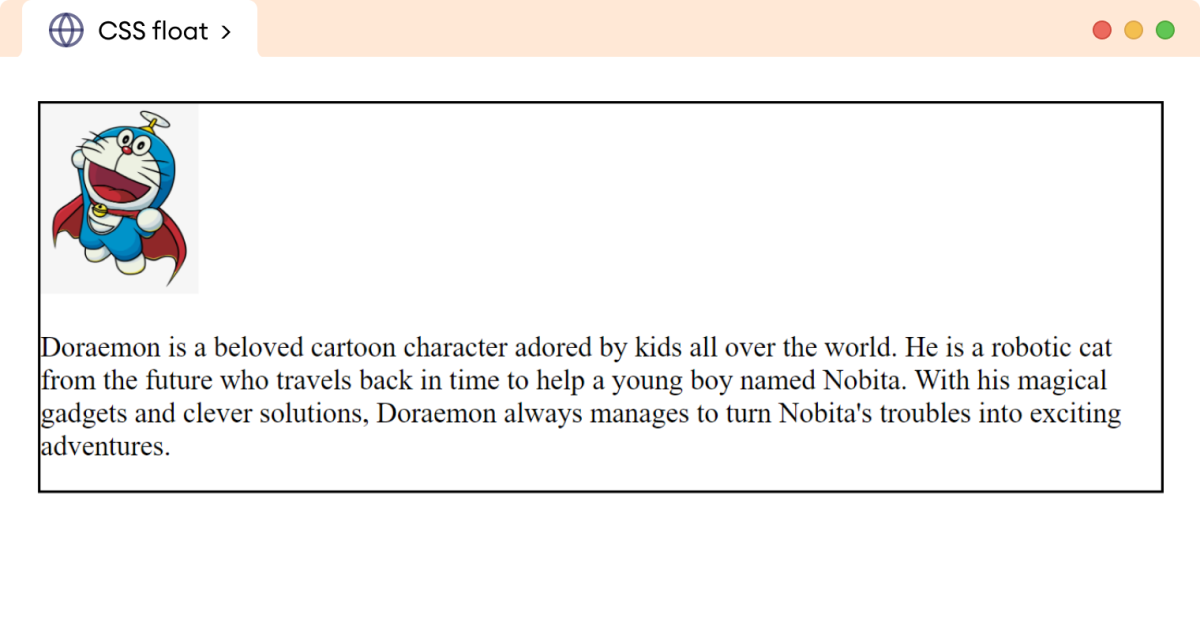
In the above example, we have used the none
(default value) of the float
property. This value does nothing to the existing layout.
Example: CSS float right Value
Let's see an example of the float
property with the right
value,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS float</title>
</head>
<body>
<div class="parent">
<img
src="https://www.seekpng.com/png/detail/109-1093815_doraemon-png-png-images-doraemon-png.png"
alt="A flying Doremon image"
/>
<p>
Doraemon is a beloved cartoon character adored by kids all over
the world. He is a robotic cat from the future who travels back
in time to help a young boy named Nobita. With his magical
gadgets and clever solutions, Doraemon always manages to turn
Nobita's troubles into exciting adventures. Whether it's
rescuing friends, solving problems, or teaching important life
lessons, Doraemon's kind heart and unwavering friendship make
him a true hero. Kids are captivated by Doraemon's cute
appearance, humorous antics, and the imaginative world he brings
to life, making him an endearing and unforgettable character in
their lives.
</p>
</div>
</body>
</html>
div.parent {
border: 2px solid black;
}
img {
/* pushes the image to the right of its container/parent */
float: right;
width: 100px;
height: 110px;
}
Browser Output
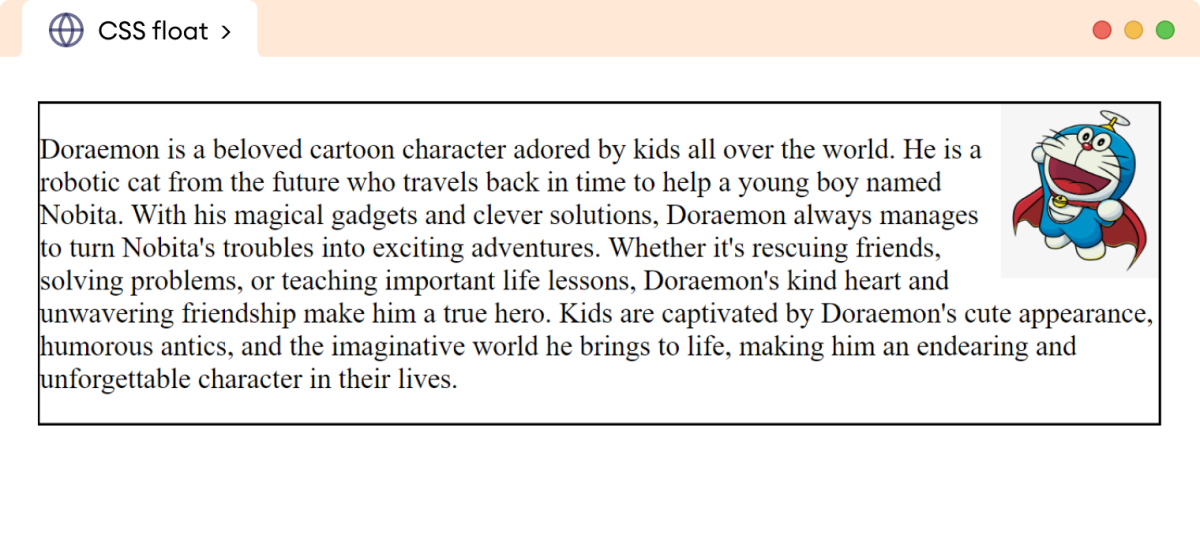
In the above example,
float: right;
pushes the image to the right
of the parent element div
. The surrounding text wraps the image.
Example: CSS float left Value
Let's see an example of the float
property with the left
value,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS float</title>
</head>
<body>
<div class="parent">
<img
src="https://www.seekpng.com/png/detail/109-1093815_doraemon-png-png-images-doraemon-png.png"
alt="A flying Doremon image"/>
<p>
Doraemon is a beloved cartoon character adored by kids all over
the world. He is a robotic cat from the future who travels back
in time to help a young boy named Nobita. With his magical
gadgets and clever solutions, Doraemon always manages to turn
Nobita's troubles into exciting adventures. Whether it's
rescuing friends, solving problems, or teaching important life
lessons, Doraemon's kind heart and unwavering friendship make
him a true hero. Kids are captivated by Doraemon's cute
appearance, humorous antics, and the imaginative world he brings
to life, making him an endearing and unforgettable character in
their lives.
</p>
</div>
</body>
</html>
img {
/* pushes the image to the left of its container/parent */
float: left;
width: 100px;
height: 120px;
}
div.parent {
border: 2px solid black;
}
Browser Output
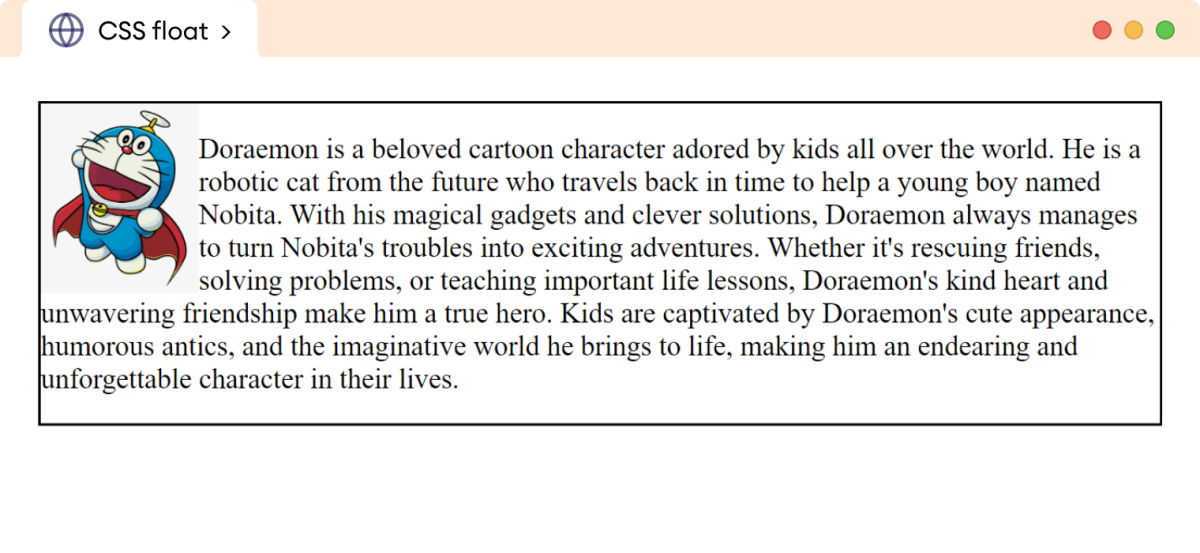
In the above example,
float: left;
pushes the image to the left
of the parent element div
. The surrounding text wraps the image.
Note: The CSS clear
property is used to control the behavior of elements that are adjacent to floated elements. It allows to turn off the wrapping of the text and helps to maintain the normal flow in the document.
CSS Float with Inline Elements
Let's see how floating works with inline elements like span
,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS float</title>
</head>
<body>
<p>
<span
>Doraemon is a beloved cartoon character adored by kids all over
the world.
</span>
Doraemon is a robotic cat from the future who travels back in time to
help a young boy named Nobita. With his magical gadgets and clever
solutions, Doraemon always manages to turn Nobita's troubles into
exciting adventures. Whether it's rescuing friends, solving
problems, or teaching important life lessons, Doraemon's kind heart
and unwavering friendship make him a true hero. Kids are captivated
by Doraemon's cute appearance, humorous antics, and the imaginative
world he brings to life, making him an endearing and unforgettable
character in their lives.
</p>
</body>
</html>
span {
/* pushes the span element to the right of its container */
float: right;
width: 190px;
border: 1px solid black;
background-color: greenyellow;
margin-left: 12px;
}
p {
padding: 8px;
border: 2px solid black;
}
Browser Output
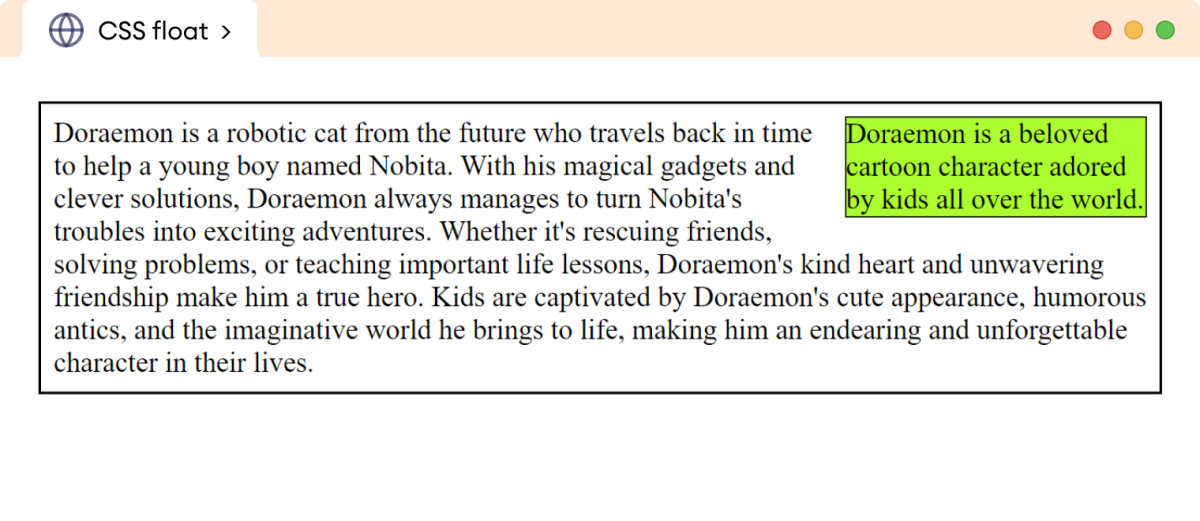
In the above example, the float: right
moves the inline element span
to the right of the parent
.
Note: Important points with inline float
- The floated inline text elements should always be assigned a width value. Otherwise, they will expand to fit their content, which may not be an issue for smaller content. This can become problematic for larger content.
Images have their inherent width and height, so adding width and height is not mandatory.
- The floated inline elements behave as block elements. We can add width, height, margin, and padding on all sides to a floated inline element.
- The margin in the floated element does not collapse. In the normal flow, the adjacent top and bottom margins collapse and take the value of the larger margin.
CSS float with the Block Elements
Let's see an example of how the float
property works with the block elements like div
,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS float</title>
</head>
<body>
<div class="parent">
<p class="float">
Doraemon is a beloved cartoon character adored by kids all over
the world. Doraemon is a robotic cat from the future who travels
back in time to help a young boy named Nobita.
</p>
<p>
With his magical gadgets and clever solutions, Doraemon always
manages to turn Nobita's troubles into exciting adventures.
Whether it's rescuing friends, solving problems, or teaching
important life lessons, Doraemon's kind heart and unwavering
friendship make him a true hero. Kids are captivated by
Doraemon's cute appearance, humorous antics, and the imaginative
world he brings to life, making him an endearing and
unforgettable character in their lives.
</p>
</div>
</body>
</html>
p.float {
/* pushes the p element to the left of its container */
float: left;
/* width is necessary */
width: 300px;
border: 2px solid black;
background-color: greenyellow;
}
/* styles the div element with parent class */
div.parent {
padding: 4px;
border: 4px solid black;
background-color: yellow;
}
Browser Output
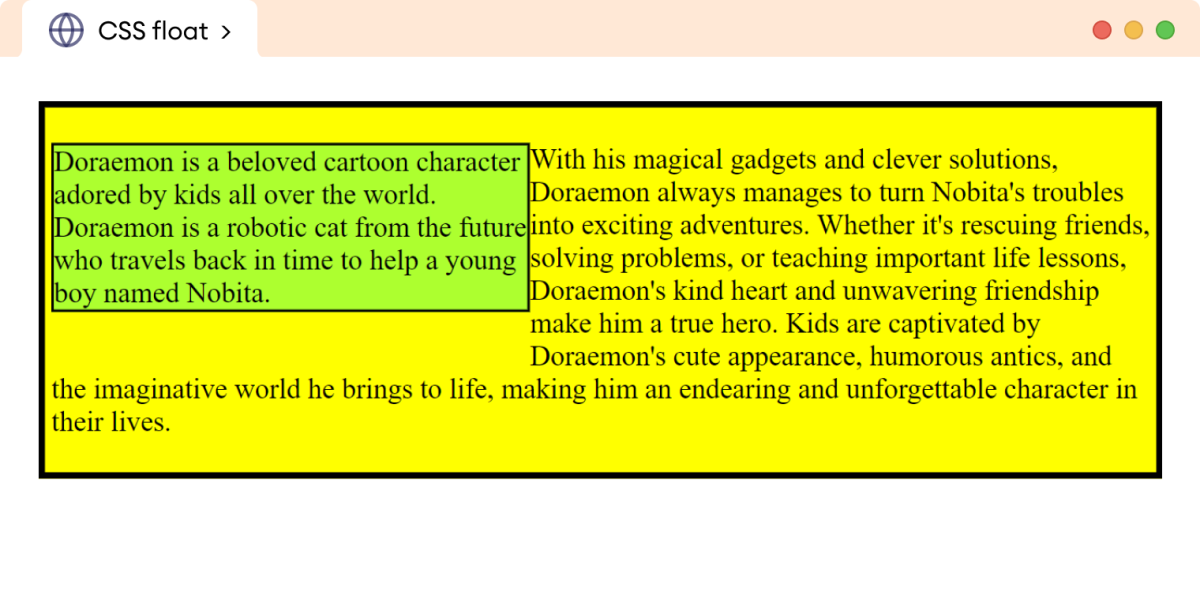
Here, the float: right
declaration moves the block element p
to the left of the parent div
element.
Similarly, the other non-floating paragraph after the floated block element maintains the normal flow in the document.
In the above example, the paragraph with a yellow
background occupies the entire width as expected while also accommodating the floated paragraph element. It wraps the text around the floated paragraph element, acknowledging its presence.
Note: Important points to remember when floating block elements:
- The block-level element should be assigned a
width
value when using thefloat
property. Otherwise, the default width value isauto
, which causes it to fill the entire width of the parent element.
Therefore, the effect of using the float
property becomes invisible.
- The floating block element does not float higher than its original reference in the document.