CSS pseudo-classes selectors select the HTML elements based on their state or position. For example,
button:hover {
background-color: red;
color:black;
}
Browser Output
Here, hover
pseudo-class changes the styles of button while
placing the mouse over it.
Syntax of Pseudo Class Selector
The syntax of a pseudo-class selector is as follows:
element:pseudo-class {
/* CSS styles */
}
Here,
element
: specifies the HTML element-
pseudo-class
: specifies the specific state of the element that we want to target
Pseudo-class keywords are added to the selectors and preceded by a colon
(:
).
Types of Pseudo-Classes
There are the following types of pseudo-classes in CSS:
-
Structural pseudo-class: Selects elements based on
their position in document such as
:first-child
,:last-child
, etc.
-
Link pseudo-class: Selects the links based on their
state such as
:link
,:visited
, etc.
-
UI pseudo-class: Selects the form elements based on
their state such as
:enabled
,:disabled
, etc.
Let's learn each of them in detail.
Structural Pseudo-Classes
Structural pseudo-class selects elements based on their position in the document. There are following structural pseudo-classes.
CSS first-child Pseudo-Class
The first-child
pseudo-class selector styles the first child of
its parent element. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>CSS first-child</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<div>
<p>This is the first paragraph.</p>
<p>This is the second paragraph.</p>
<p>This is the third paragraph.</p>
</div>
</body>
</html>
div p:first-child {
color: red;
}
Browser Output
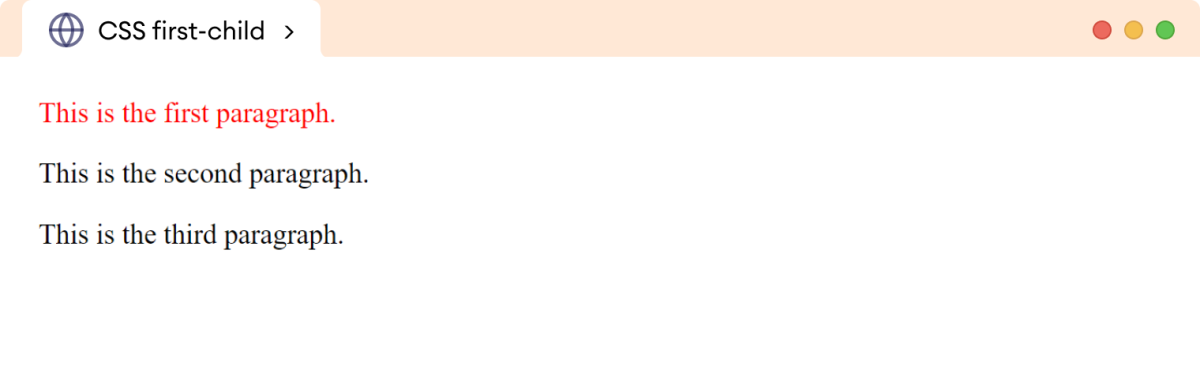
Here, the div p:first-child
selector selects the first
paragraph element that is a direct child of the div
element and
changes its color to red
.
CSS last-child Pseudo-Class
The last-child
pseudo-class selector styles the last child of
its parent element. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>CSS last-child</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<div>
<p>This is the first paragraph.</p>
<p>This is the second paragraph.</p>
<p>This is the third paragraph.</p>
</div>
</body>
</html>
div p:last-child {
color: red;
}
Browser Output
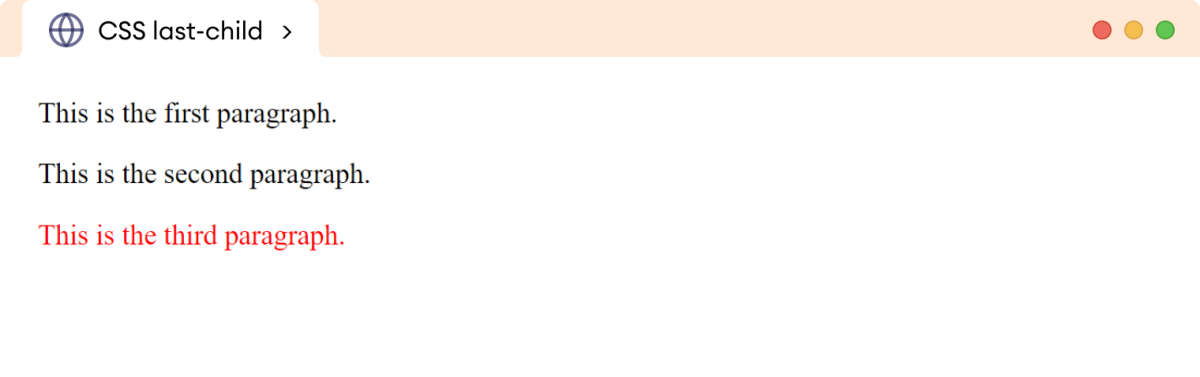
Here, the div p:last-child
selector selects the last paragraph
element that is a direct child of the div
element and changes
its color to red
.
CSS first-of-type Pseudo-Class
The first-of-type
pseudo-class selector styles the first
element of a particular type within the parent. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>CSS first-of-type</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<h2>Section one</h2>
<div>
<p>This is the first paragraph.</p>
<p>This is the second paragraph.</p>
</div>
<h2>Section two</h2>
<div>
<p>This is the first paragraph.</p>
<p>This is the second paragraph.</p>
</div>
</body>
</html>
p:first-of-type {
color: red;
}
Browser Output
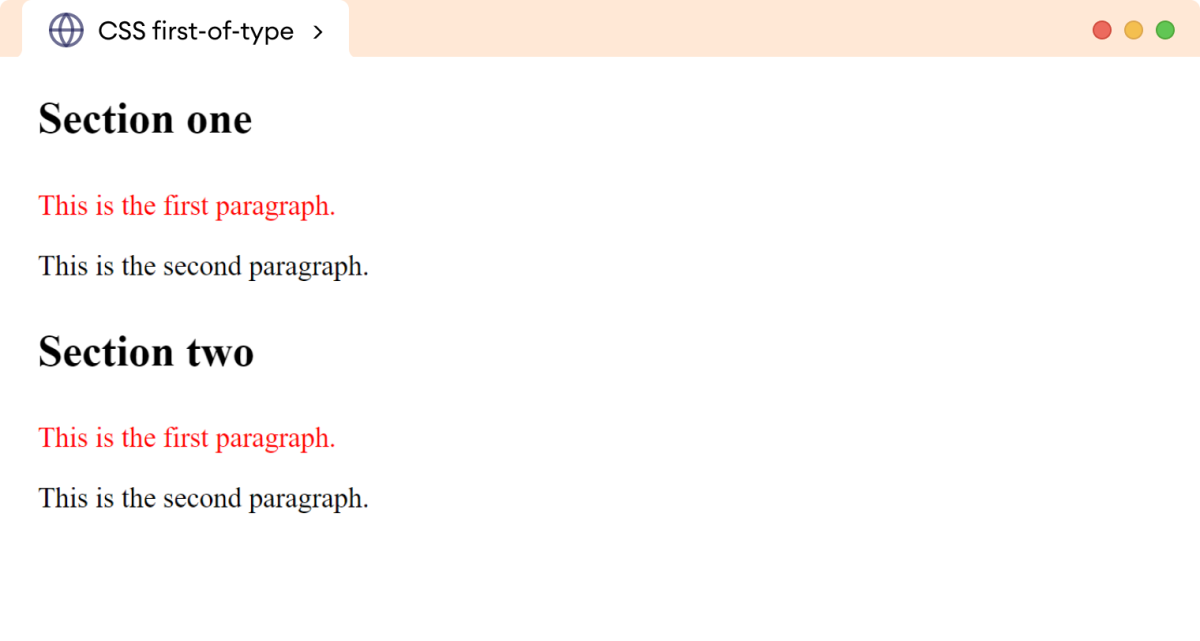
Here, the first-of-type
pseudo-class selects the first
p
element within the parent elements and changes the text color
to red
.
CSS not Pseudo-Class
The not
pseudo-class selector styles the elements that do not
match the user defined selector. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>CSS Pseudo-Classes</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<div>
<p>This is the first paragraph.</p>
<p class="special-paragraph">This is a special paragraph.</p>
<p>This is the third paragraph.</p>
</div>
</body>
</html>
p:not(.special-paragraph) {
color: red;
}
Browser Output
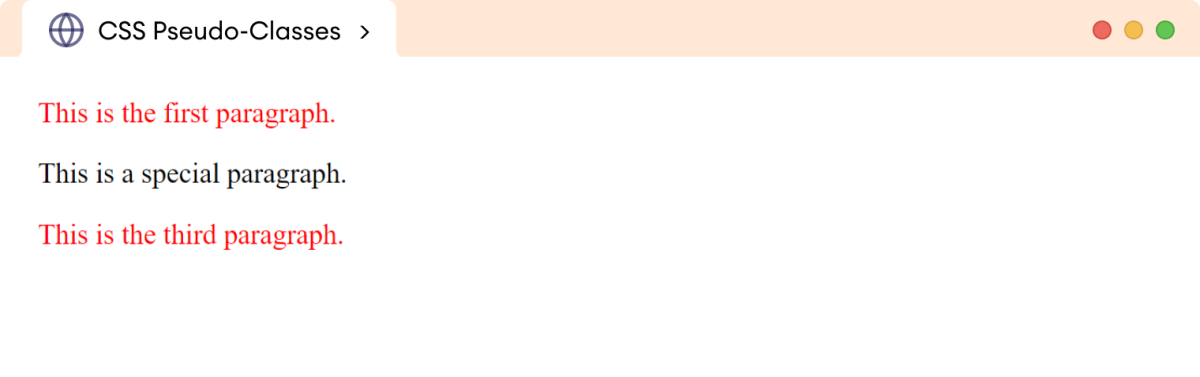
In the above example,
p:not(.special-paragraph) {
color: red;
}
select all p
elements that are not of the class
special-paragraph
.
This means that the first and third paragraphs will be red
, but
not the second paragraph.
CSS empty Pseudo-Class
The empty
pseudo-class selector styles every element that has
no children. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>CSS Pseudo-Classes</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<!--empty div-->
<div></div>
<!--div having child elements-->
<div>
<p>This is a paragraph.</p>
<p>This is a paragraph.</p>
</div>
</body>
</html>
div:empty {
width: 100px;
height: 20px;
background-color: red;
}
Browser Output
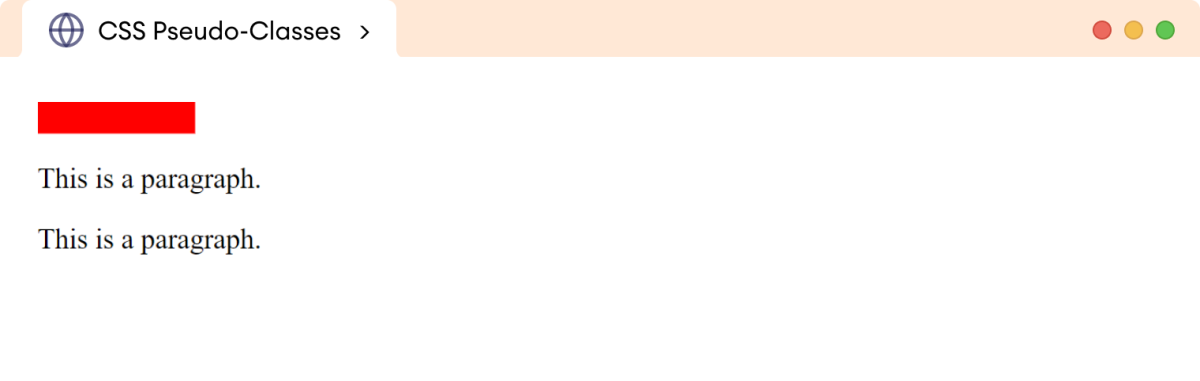
Here, the empty
pseudo-class selects and styles only the empty
div
element.
Link Pseudo-Classes
Link pseudo-classes select links based on their state. There are following link pseudo-classes.
CSS link Pseudo-Class
The link
pseudo-class selector styles all the unvisited links.
For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Pseudo-Classes</title>
</head>
<body>
<p>Find additional information from <a href="#">here</a>.</p>
</body>
</html>
a:link {
text-decoration: none;
color: blue;
font-weight: bold;
background-color: greenyellow;
}
Browser Output
Find additional information from here.
Here, the link
pseudo-class selects and styles the link prior
to it is clicked or visited.
Note that as soon as we click on the link, the color changes to
purple
. This is the default behavior of the link.
CSS visited Pseudo-Class
The visited
pseudo-class selector styles the links that are
visited by the user. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Pseudo-Classes</title>
</head>
<body>
<p>Find additional information from <a href="#">here</a>.</p>
</body>
</html>
/* styles the default state */
a:link {
text-decoration: none;
color: blue;
font-weight: bold;
background-color: greenyellow;
}
/* styles the visited state */
a:visited {
color: red;
}
Browser Output
Find additional information from here.
.
In the above example, when the user clicks on the link, the color changes to
red
.
CSS focus Pseudo-Class
The focus
pseudo-class selector styles the element that is
focused by the user. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Pseudo-Classes</title>
</head>
<body>
<p>Find additional information from <a href="#">here</a>.</p>
</body>
</html>
/* styles the focused state */
a:focus {
background-color: skyblue;
}
Browser Output
Find additional information from here.
In the above example, the a:focus
selector styles link during
focus with a skyblue
background color.
CSS hover Pseudo-Class
The hover
pseudo-class selector styles the elements when the
mouse is placed over it. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Pseudo-Classes</title>
</head>
<body>
<p>Find additional information from <a href="#">here</a>.</p>
</body>
</html>
/* styles the link state */
a:link {
text-decoration: none;
color: blue;
font-weight: bold;
}
/* styles the visited state */
a:visited {
color: purple;
}
/* styles the hover state */
a:hover {
background-color: greenyellow;
}
Browser Output
Find additional information from here.
In the above example, the a:hover
selector adds a background
color to link while hovering.
Note: The hover
pseudo-class can be used with
any element, not only with the links.
CSS active Pseudo-Class
The active
pseudo-class selector styles the elements when the
user clicks on it. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Pseudo-Classes</title>
</head>
<body>
<p>Find additional information from <a href="#">here</a>.</p>
</body>
</html>
/* styles the link state */
a:link {
text-decoration: none;
color: blue;
font-weight: bold;
background-color: greenyellow;
}
/* styles the visited state */
a:visited {
color: purple;
}
/* styles the hover state */
a:hover {
text-decoration: underline;
}
/* styles the active state */
a:active {
color: red;
}
Browser Output
Find additional information from here.
In the above example, the a:active
selector styles link when it
is active with red
color.
Note: The order of providing link pseudo-classes is
important. The correct sequence is link
, visited
,
hover
, and active
to ensure that styles are
applied correctly.
UI Pseudo-Classes
A UI pseudo-class selects elements based on their state or interaction with the user.
CSS enabled Pseudo-Class
The enabled
pseudo-class selects and styles any enabled
element.
An element is enabled if it can be selected, clicked on, typed into, etc., or accepts focus.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Pseudo-Classes</title>
</head>
<body>
<input type="text" placeholder="Enter your username" />
<button>Submit</button>
</body>
</html>
input:enabled {
border: 2px solid blue;
}
Browser Output
Here, the enabled
pseudo-class selects the
input
element and adds a blue solid border of 2px
.
CSS disabled Pseudo-Class
The disabled
pseudo-class selects and styles elements that are
disabled. It can form elements like input fields or buttons that the user
cannot interact with.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Pseudo-Classes</title>
</head>
<body>
<input type="text" placeholder="Username..." />
<button disabled>Submit</button>
</body>
</html>
button:disabled {
cursor: not-allowed;
}
Browser Output
Here, the cursor
property sets the cursor to a
not-allowed
when the user hovers over the disabled button.
Note: To use the disabled
pseudoclass
selector, we need to add the disabled keyword to the HTML element.