The CSS padding
property controls the space between an element's content and border. For example,
h1 {
border: 4px solid black;
padding: 20px;
}
Browser Output
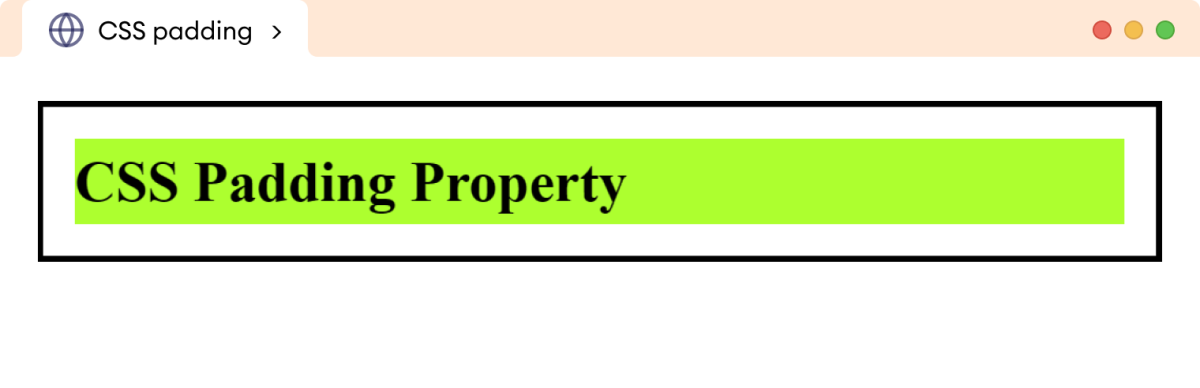
Here, the space between the content (represented by green color) and the border is padding.
The padding
property is different from the margin
property. The padding
property adds extra space within an element, while the margin
property adds extra space around the element.
CSS padding Syntax
The syntax of the padding
property is as follows,
padding: length | percentage | inherit;
Here,
length
: defines the padding in length units such aspx
,pt
,em
, etcpercentage
: defines the padding in percentage (%
)inherit
: inherits the value from the parent element
The padding
property does not accept the negative value.
Example: CSS padding Property
Let's see an example of the padding
property,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS padding</title>
</head>
<body>
<p class="first">This paragraph has a padding of 15px.</p>
<p class="second">This paragraph has a padding of 30px.</p>
</body>
</html>
p.first {
/* add a 20px padding */
padding: 20px;
}
p.second {
/* add a 40px padding */
padding: 40px;
}
p {
border: 4px solid black;
background-color: greenyellow;
/* clips the background color to content only */
background-clip: content-box;
}
Browser Output
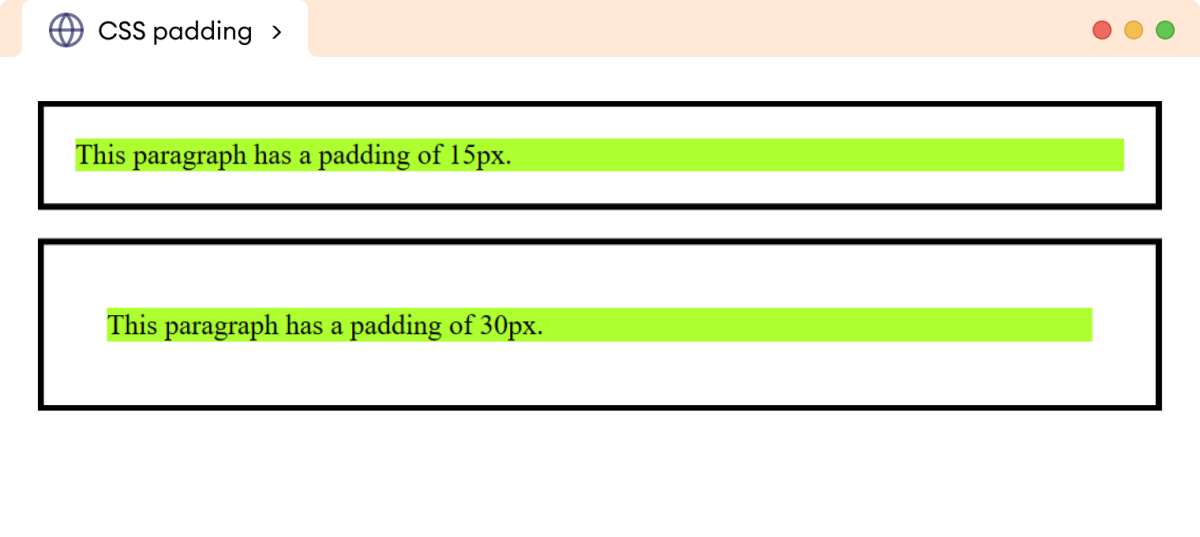
The above example shows the working of different padding
values using the px
unit.
The percentage unit is not recommended for use for the padding. The percentage unit is a relative unit, and the change in the parent width causes unexpected changes in the padding size.
Note: The background-clip
property only adds background color to the content of an element using the content-box
value. We have used it to visualize the padding.
You can learn about background-clip.
CSS padding Constituent Properties
The padding property
constitutes the following CSS properties to add padding to individual sides of the element.
padding-top
: adds padding to the top sidepadding-right
: adds padding to the right sidepadding-bottom
: adds padding to the bottom sidepadding-left
: adds padding to the left side
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS padding</title>
</head>
<body>
<p>
This paragraph has a padding of 30px at the top, 80px at the right,
40px at the bottom, and 20px at the left.
</p>
</body>
</html>
p {
border: 4px solid black;
padding-top: 30px;
padding-right: 80px;
padding-bottom: 40px;
padding-left: 20px;
background-color: greenyellow;
/* clips the background color to content only */
background-clip: content-box;
}
Browser Output
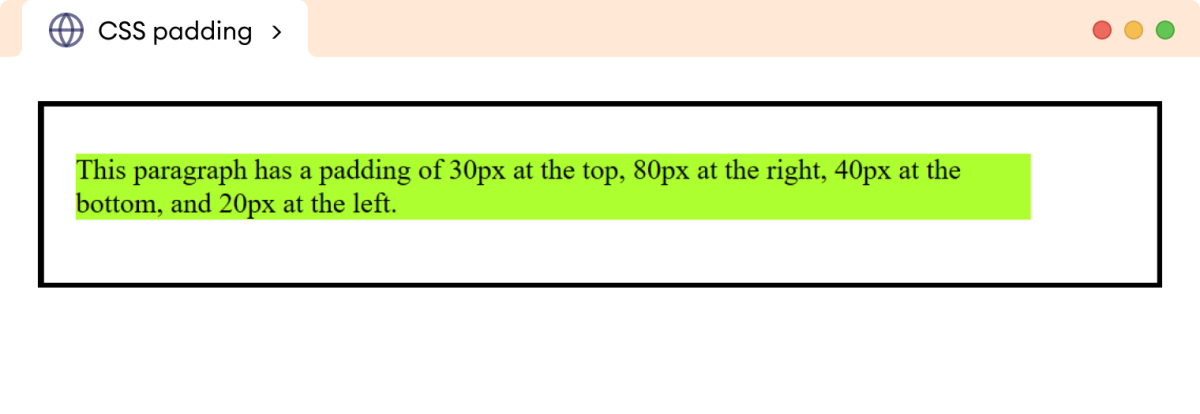
CSS padding as shorthand Property
The padding
property can be used as shorthand to specify the padding of one to four sides of an element.
The padding
property can have,
- One value: applies to all four sides
- Two values: applies to top/bottom and the second to left/right respectively
- Three values: applies to the top, left/right, and bottom, respectively
- Four values: applies to top, right, bottom, and left, respectively
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS padding</title>
</head>
<body>
<p class="one-value">
This paragraph has a padding of 30px on all sides.
</p>
<p class="two-value">
This paragraph has a padding of 10px at the top/bottom and 40px at
the left/right sides.
</p>
<p class="three-value">
This paragraph has a padding of 10px at the top, 80px at the
left/right, and 5px at the bottom.
</p>
<p class="four-value">
This paragraph has a padding of 40px at the top, 15px at the right,
5px at the bottom, and 30px at the left.
</p>
</body>
</html>
p.one-value {
/* applies to all sides */
padding: 30px;
}
p.two-value {
/* adds 15px to top/bottom and 40px to left/right */
padding: 10px 40px;
}
p.three-value {
/* adds 10px to top, 60px to left/right, 5px to bottom */
padding: 10px 80px 5px;
}
p.four-value {
padding: 40px 15px 5px 30px;
}
p {
/* add border of solid 4px */
border: 4px solid black;
background-color: greenyellow;
/* clips the background color to content only */
background-clip: content-box;
}
Browser Output
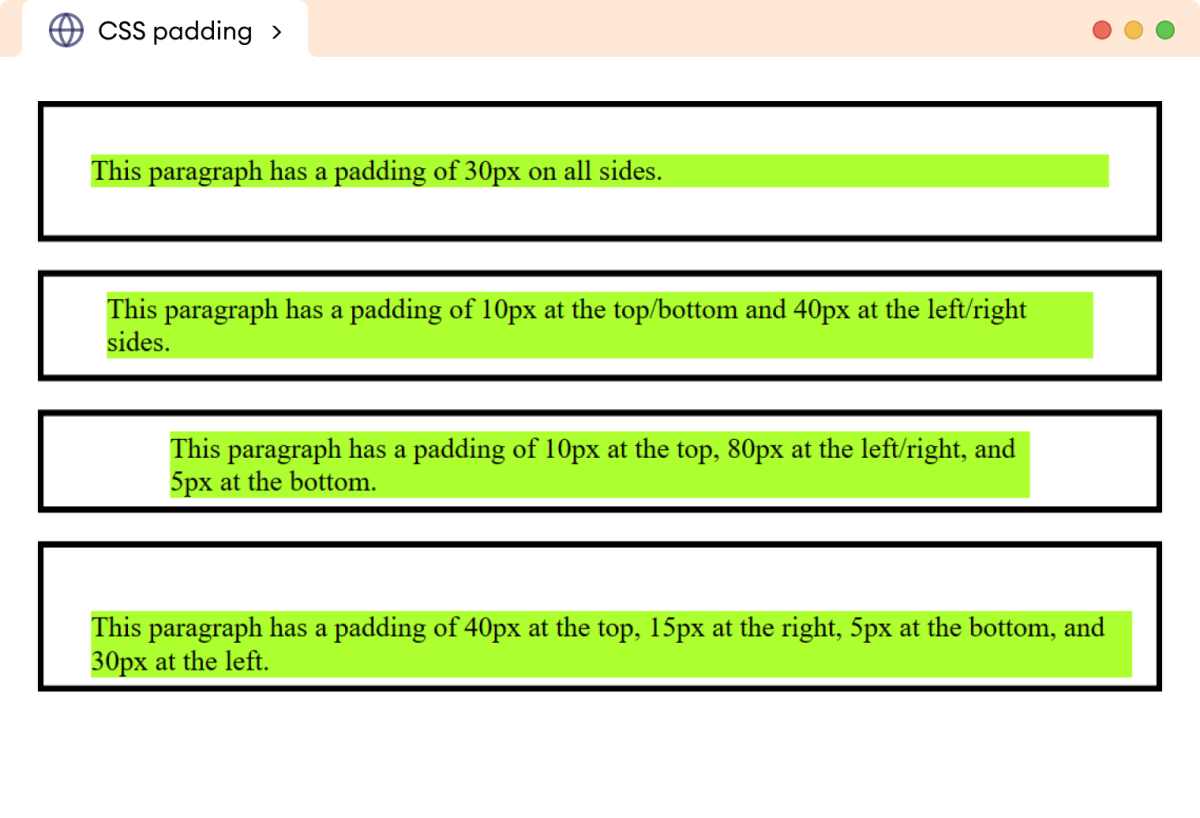
Note: The padding
value is not included in the width
and height
of an element by default. Adding a padding
value changes the actual width
and height
, leading to unexpected layouts.
This issue can be solved using the box-sizing property.