CSS background-image
property is used to add a background image on an element or the webpage. For example,
body {
background-image: url("girl-avatar.png");
}
Browser Output
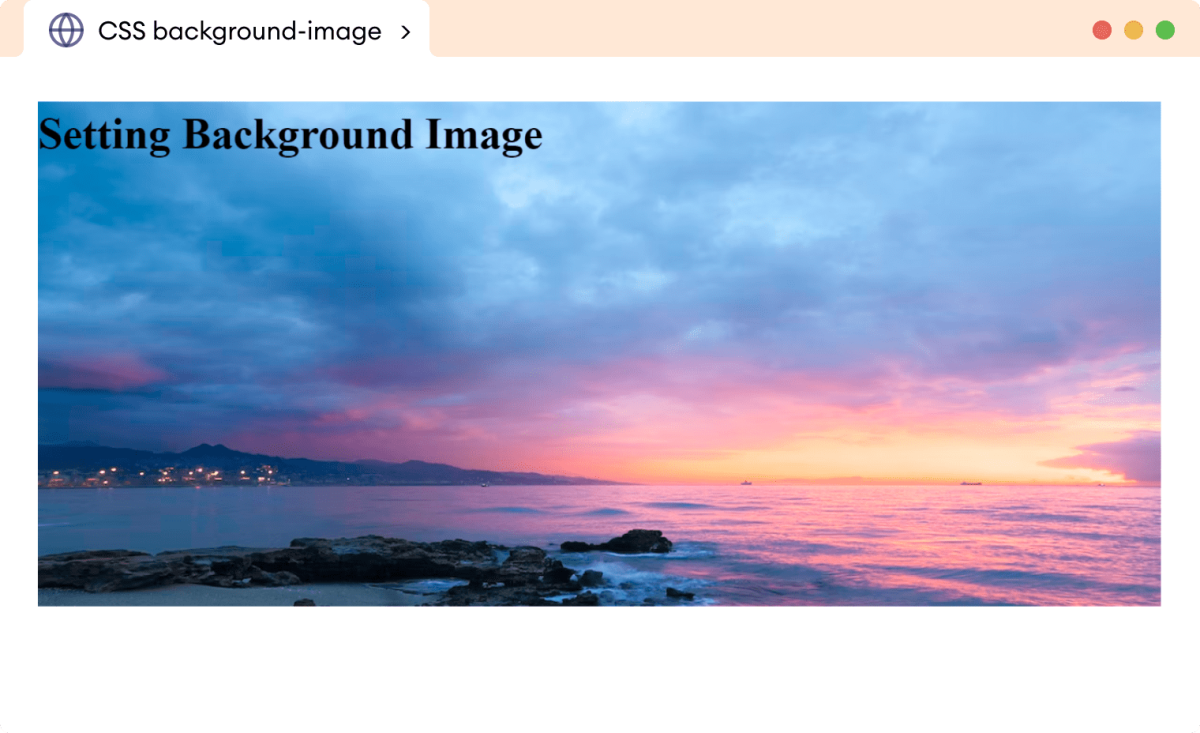
Here, the background-image
property sets the specified image in the background of the body
element.
Syntax of Background-Image
The syntax of the background-image
property is as follows,
background-image: url(path-to-image) | none | initial | inherit;
Here,
url(path-to-image)
: specifies the URL of the image,none
: no image is displayed (default value)initial
: sets the property to its default valueinherit
: inherits the property from its parent element.
CSS Background-Image Example
Let's see an example of the background-image
property,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS background-image</title>
</head>
<body>
<!-- Using CSS background-image property to set the background image of body -->
</body>
</html>
body {
background-image: url("https://www.programiz.com/blog/content/images/2022/03/Banner-Image-1.png");
}
Browser Output
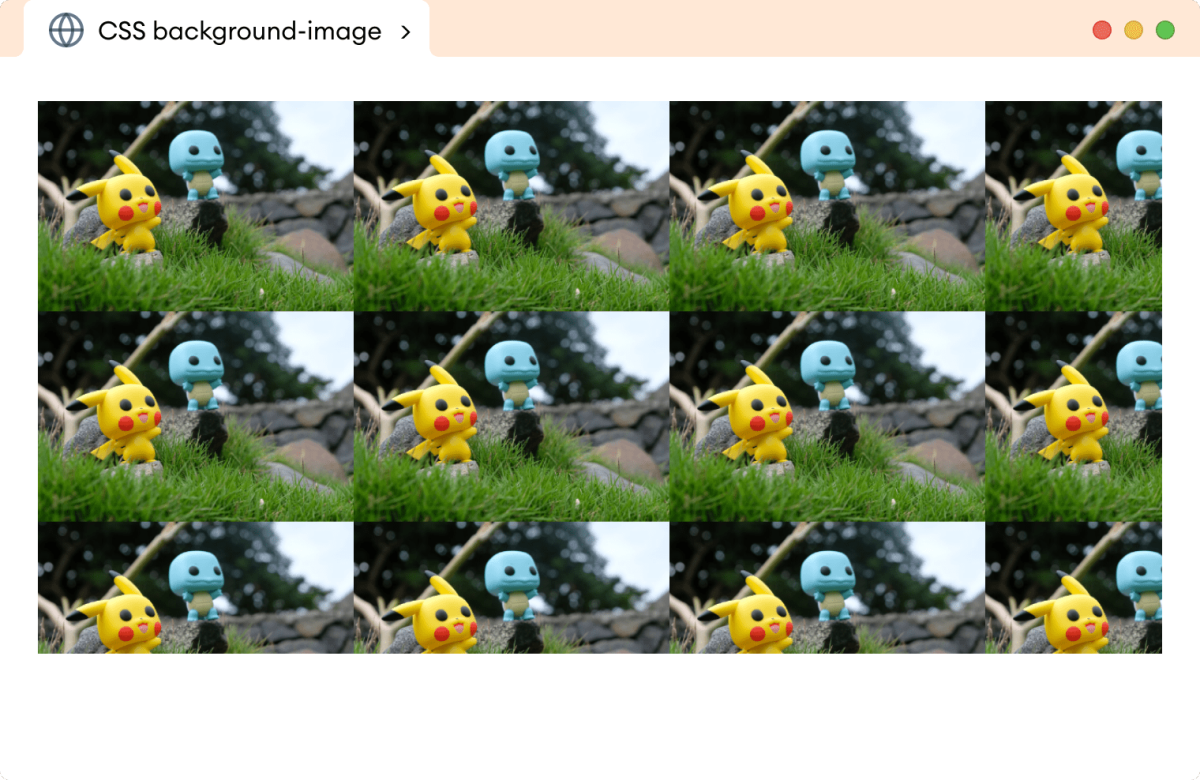
In the above example, the background image is repeated. If the image is smaller than the container element, then the image is repeated in horizontal and vertical directions. And the remaining image is clipped from the edge of the container.
CSS Background-Image Properties
We can customize the background image using different background image properties. Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS background-image</title>
</head>
<body>
<!-- Using CSS background-image property to set the background image of body -->
</body>
</html>
body {
background-image: url("../assets/background-image.png");
background-image: url("https://www.programiz.com/blog/content/images/2020/11/intro-c-banner-1-1.png");
/* doesn't allows the image to repeat */
background-repeat: no-repeat;
/* scales the image proportionally to cover the background */
background-size: cover;
/* centers the image in the center of the background */
background-position: center;
/*sets the height of the body to 100viewport height */
height: 100vh;
}
Browser Output
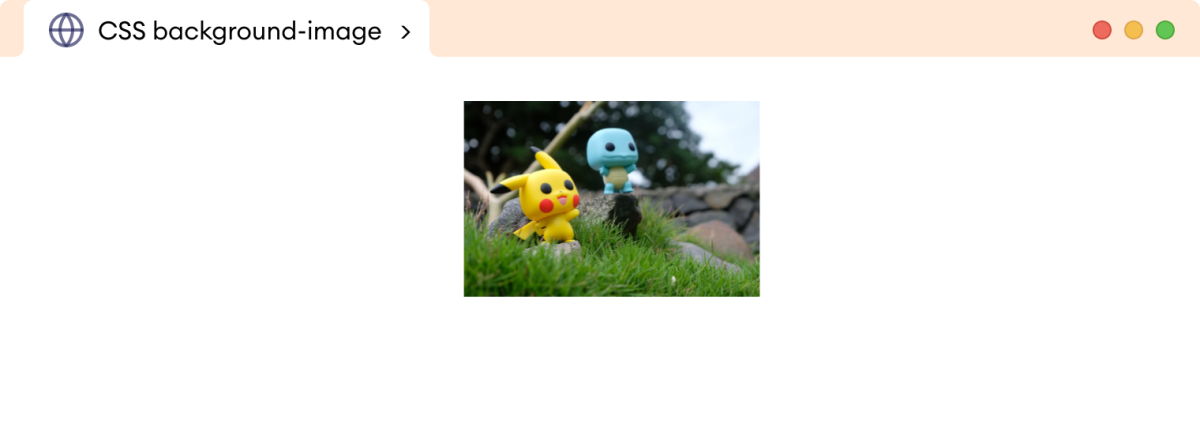
In the above example, we have used background-repeat
, background-size
, and background-position
properties. Some of the commonly used properties to customize background images are as follows:
Property | Description |
---|---|
background-repeat | specifies whether a background image should be repeated or not |
background-attachment | specifies whether the background image should be fixed or scroll with the rest of the content of the page |
background-size | specifies the size of the background image |
background-position | specifies the position of the background image within the element |
background-clip | specifies the background area to be either border-box, content-box, or padding-box |
background-origin | specifies the starting position of the background image within the element |
Multiple Backgrounds
The background-image
property also allows us to have multiple background images for an element. We can provide multiple url
separated by a comma in the background-image
property.
The images are layered on top of each other.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS background-image</title>
</head>
<body>
<!-- Using CSS background-image property to set multiple background images of body -->
</body>
</html>
body {
height: 100vh;
/* url for two images respectively */
background-image: url("https://www.programiz.com/blog/content/images/2022/03/Banner-Image-1.png"),
url("https://img.freepik.com/free-vector/leaves-background-color-year_23-2148380575.jpg?size=626&ext=jpg&ga=GA1.2.1929559493.1681795349&semt=ais");
/* sets first image width to 400px and scales the second image to
cover background area */
background-size: 400px, cover;
/* both images stopped from repeating */
background-repeat: no-repeat;
}
Browser Output
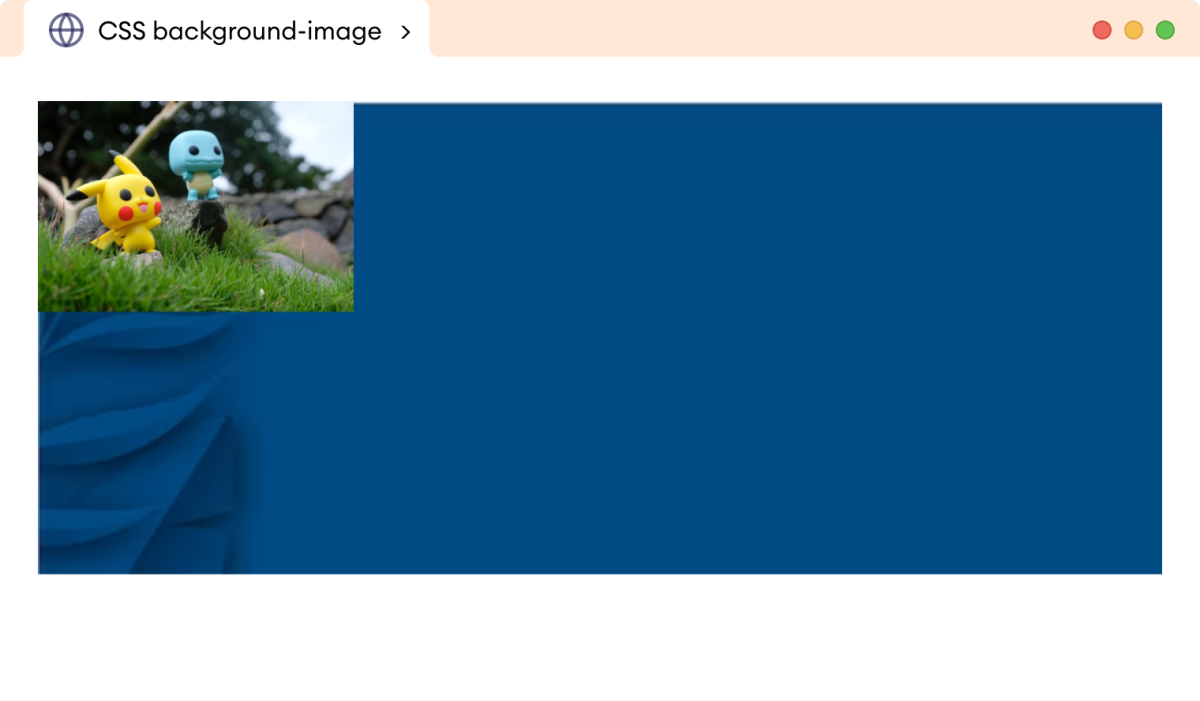
In the above example, we have used two background images (girl avatar image and dark blue background image) to set the background of the body
element. The first added image (girl avatar) is stacked at the top of the second added image (dark blue background image).
Additionally, we have used background-size
, background-postion
, and background-repeat
properties to customize the background.
Using Background Color as Fallback
It is common practice to specify a background-color
along with a background-image
as a fallback. This ensures that the website maintains a consistent look in case the image fails to load.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS background-image</title>
</head>
<body>
<h1>Using background color as fallback for image</h1>
</body>
</html>
body {
background-color: lightblue;
background-image: url("https://www.programiz.com/blog/content/images/2020/11/intro-c-banner-1-1.png");
background-repeat: no-repeat;
background-size: cover;
background-position: center;
height: 100vh;
}
Browser Output
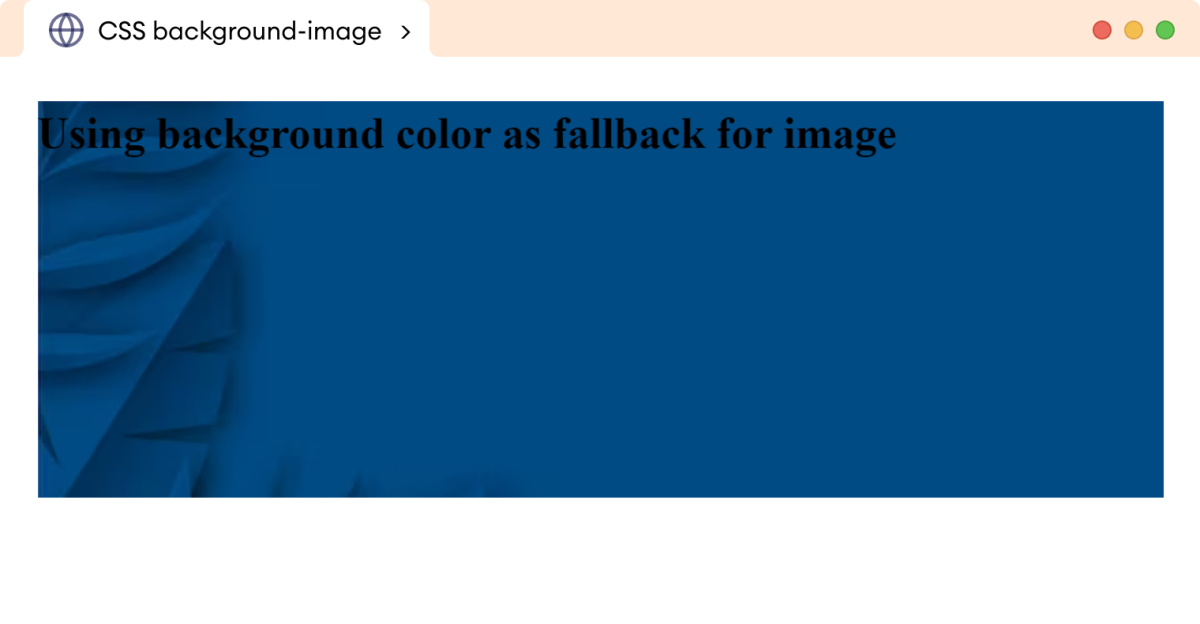
In the above example, if the background-image
fails to load then background-color
will be set to lightblue
, and our text will be still visible and consistent with the design.
Let's assume our background-image
fails to load, then we will have the following browser output,
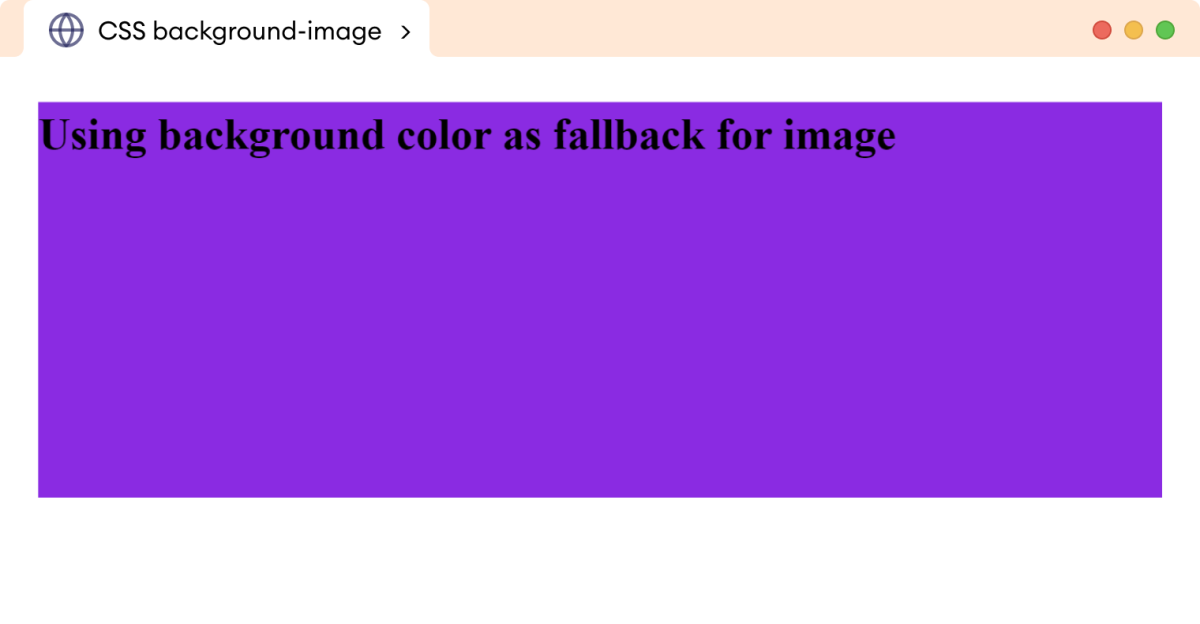