Icons are images or symbols that provide a visual representation of links, buttons, and other interactive elements. For example,
The above example shows icons in the navigation bar.
Icons add visual interest to a website and make it more engaging. Icons can be added to our website using different icon libraries.
Let's see how we can add icons using various available icon libraries and style them using CSS.
Font Awesome Icons
The Font Awesome icons can be added by linking the Font Awesome library in
the <head>
section of HTML. For example,
/* including font awesome library */
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.0.0-beta3/css/all.min.css">
The above code allows us to access the icons from the Font Awesome library.
We can then use different predefined classes in our inline elements, such as
span
and i
, to access icons on our webpage.
Let's see an example,
/* adding font awesome icons */
<i class="fas fa-home"></i>
<i class="fas fa-cloud"></i>
<i class="fas fa-heart"></i>
<i class="fas fa-car"></i>
<i class="fas fa-file"></i>
Browser Output
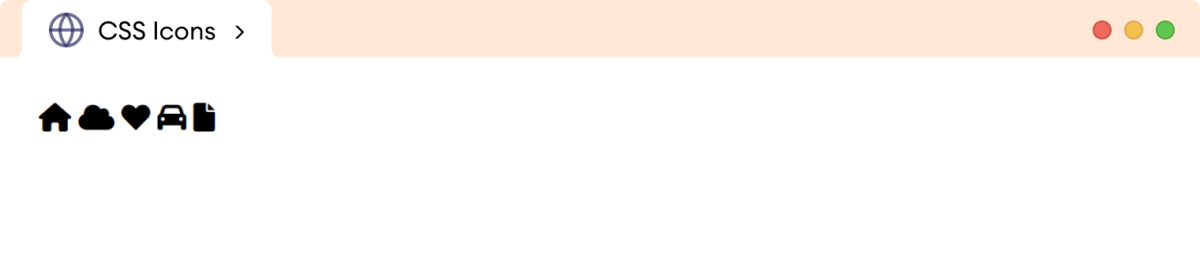
The above example shows the default font awesome icons.
Bootstrap Icons
The Bootstrap icons can be added by linking the Bootstrap Icons library in
the <head>
section of HTML. For example,
/* including bootstrap icon library */
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
The above code allows us to access the icons from the Bootstrap Icons library.
We can then use different predefined classes in our inline elements to access the icons. For example,
/* adding bootstrap icons */
<i class="glyphicon glyphicon-user"></i>
<i class="glyphicon glyphicon-cloud"></i>
<i class="glyphicon glyphicon-remove"></i>
<i class="glyphicon glyphicon-envelope"></i>
Browser Output
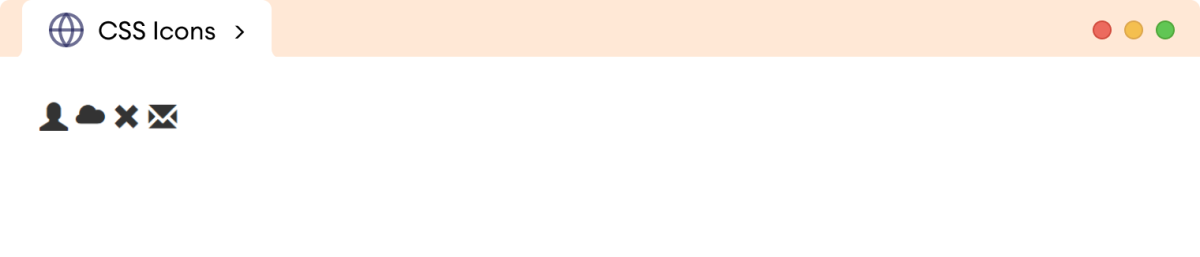
The above example shows the default bootstrap icons.
Google Icons
The Google icons can be added by linking the Google Material Icons library
in the <head>
section of HTML. For example,
/* including google material icon font */
<link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons">
The above code allows us to access the icons from the Google Material Icons library.
We can then use different predefined classes in our inline elements to access the icons. For example,
/* adding google icons */
<i class="material-icons">cloud</i>
<i class="material-icons">favorite</i>
<i class="material-icons">attachment</i>
<i class="material-icons">computer</i>
<i class="material-icons">traffic</i>
Browser Output
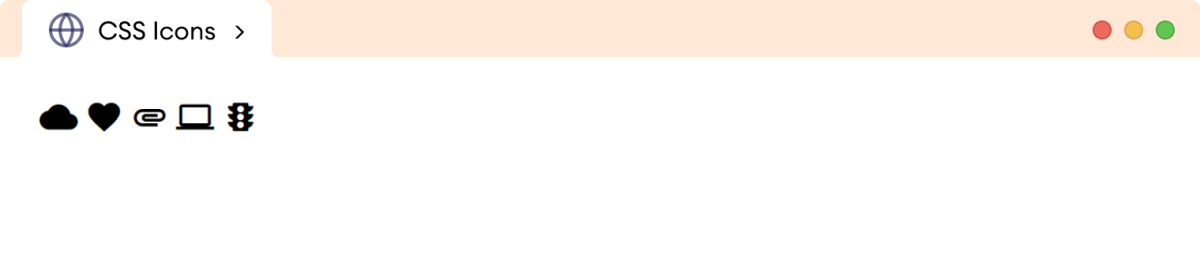
The above example shows the default Google Material Icons.
Style Icons
We can style icons using different CSS properties. For our reference, we will style icons from Font Awesome Library.
Let's see an example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>CSS Icons</title>
<link
rel="stylesheet"
href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.0.0-beta3/css/all.min.css" />
<link rel="stylesheet" href="style.css" />
</head>
<body>
<p>Unstyled icons</p>
<i class="fas fa-home"></i>
<i class="fas fa-car"></i>
<i class="fas fa-file"></i>
<i class="fas fa-heart"></i>
<p class="label">Styled Icons</p>
<div class="styled-icons">
<i class="fas fa-home"></i>
<i class="fas fa-car"></i>
<i class="fas fa-file"></i>
<i class="fas fa-heart"></i>
</div>
</body>
</html>
/* adds right margin inside all icons of .styled-icons class*/
.styled-icons .fas {
margin-right: 12px;
}
/* styles the home icon */
.styled-icons .fa-home {
color: lightskyblue;
font-size: 24px;
}
/* styles the car icon */
.styled-icons .fa-car {
color: brown;
font-size: 28px;
}
/* styles the file icon */
.styled-icons .fa-file {
color: green;
font-size: 24px;
}
/* styles the heart icon */
.styled-icons .fa-heart {
color: red;
font-size: 28px;
}
Browser Output
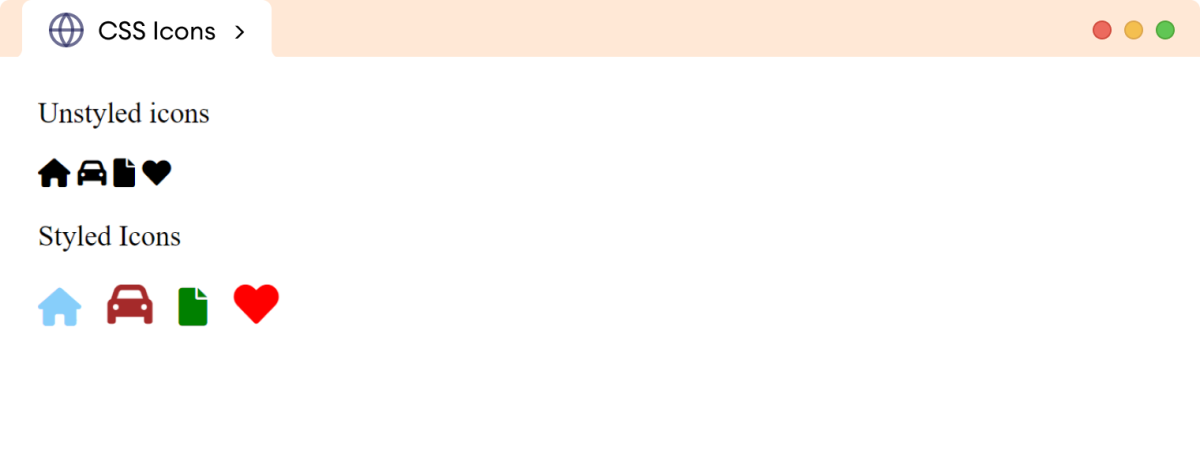
In this way, the icons can be made elegant using various CSS properties.
Note: Setting the width and height of icons within inline elements directly using CSS can be challenging. Instead, it is common practice to adjust the icon size using the font-size property.
Hover an Icon
The hover
pseudo-class selector is used to add styles for
hovering over the icon. For example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Hover Effect on Icons</title>
<link
rel="stylesheet"
href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.0.0-beta3/css/all.min.css"
/>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<h2>Hover over the icons</h2>
<div class="icons">
<i class="fas fa-home"></i>
<i class="fas fa-car"></i>
<i class="fas fa-file"></i>
<i class="fas fa-heart"></i>
</div>
</body>
</html>
/* styles all icons */
.fas {
margin-right: 24px;
/* font size increases the size */
font-size: 28px;
}
/* styles the home icon on mouse hover */
.fa-home:hover {
color: gray;
}
/* styles the car and heart icon on mouse hover */
.fa-car:hover,
.fa-heart:hover {
color: red;
}
/* styles the file icon mouse hover */
.fa-file:hover {
color: purple;
}
Browser Output
Hover over the icons
Here, the background color and the color of each icon change while hovering over them.