CSS is the language for styling web pages, and it has a few essential concepts that are important to understand.
These concepts includes:
- Inheritance
- Rule Order
- Style Rule Hierarchy
- Specificity
- Box Model
By learning these concepts, we can build a strong foundation for understanding and using CSS effectively.
Let's look at each of these in detail.
Inheritance
In CSS, inheritance passes the styles directly from the parent element to its child elements.
The child elements normally take the same styles that have been assigned to the parent, unless they are provided their own styles.
This mechanism ensures a consistent design across a webpage.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Inheritance</title>
</head>
<body>
<section>
<h2>This is a heading.</h2>
<p>This is a paragraph.</p>
<p>This is a paragraph.</p>
<p>This is a paragraph.</p>
</section>
</body>
</html>
section {
color: blue;
}
Browser Output
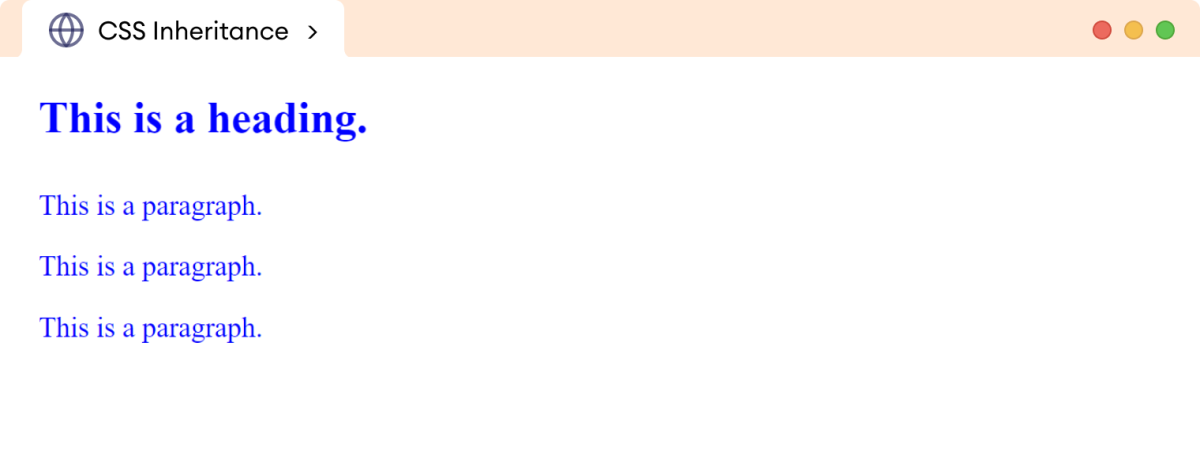
In the above example,
section {
color: blue;
}
sets the text color of all child elements of section
i.e h2
and p
to blue
.
This minimizes the need for repetitive declaration of styles, hence maintaining uniformity across the multiple elements.
Note: The inheritance behavior in CSS is property-specific, and not all properties are inherited by child elements.
Rule Order
Rule order refers to the sequence in which CSS rules are applied to the HTML elements.
The order of CSS rules determine the priority for the styles. The later rules overrides the earlier ones and ensures that the most recent styles are applied.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Rule Order</title>
</head>
<body>
<p>This is a paragraph.</p>
</body>
</html>
p {
color: red;
}
/* overrides color previous color value */
p {
color: blue;
}
Browser Output
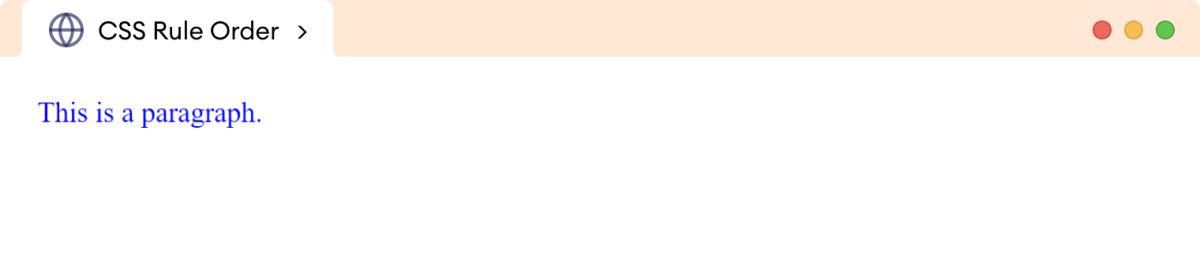
Here, the paragraph color is blue
because it is declared last in the stylesheet, overriding all previous values.
Style Rule Hierarchy
The style rule hierarchy determines the priority of CSS rules when multiple rules target the same element.
In CSS, the following hierarchy of style priorities applies:
- Inline styling: Styles applied directly within HTML element.
- ID selectors: Styles elements with specific ID.
- Class and attribute selectors: Styles elements with certain class or attribute.
- Element selectors: Styles elements with specific tag name.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Style Rule Hierarchy</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<p class=""paragraph"" id="unique">
This text will be red as the ID selector takes precedence over other
selectors.
</p>
</body>
</html>
/* id selector */
#unique {
color: red;
}
/* class selector */
.paragraph {
color: green;
}
/* element selector */
p {
color: blue;
}
Browser Output
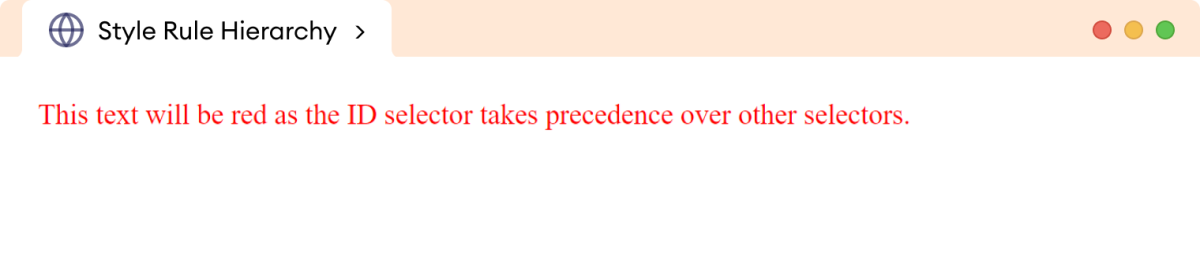
In the above example, the id selector overrides the styles of class and element selector. This is because the id selector has more priority than the class and element selectors.
Specificity
Specificity in CSS determines which style rules take precedence when multiple rules target the same element.
This helps the browser prioritize and apply the most relevant styles.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="style.css" />
<title>CSS Specificity</title>
</head>
<body>
<div>
<p id="para">This is a paragraph.</p>
</div>
</body>
</html>
div p {
color: blue;
}
p {
color: orange;
}
Browser Output
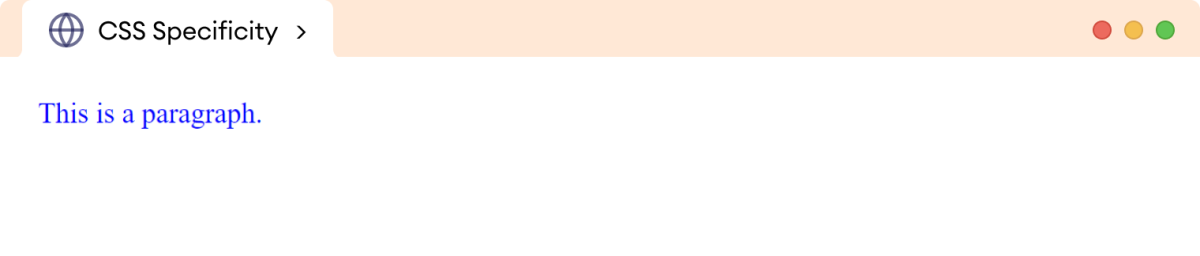
In the above example, the element selector p
sets the color of all p
elements to orange
.
However, the selector div p
is more specific for selecting paragraph so the color of paragraph is colored blue
.
Note: The more specific a CSS selector is, the higher its priority. This means that if two selectors match the same element, the more specific selector will be used to style the element.
Box Model
The box model specifies that every element in HTML is represented as a rectangular box. It helps to understand how elements are structured and interact with each other on a webpage.
Let's look at an example.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>CSS Box Model</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<h2>CSS Box Model</h2>
<div>
<p>This is a <span>paragraph</span> inside div element.</p>
</div>
</body>
</html>
h2, div, p, span {
border: 1px solid;
}
Browser Output
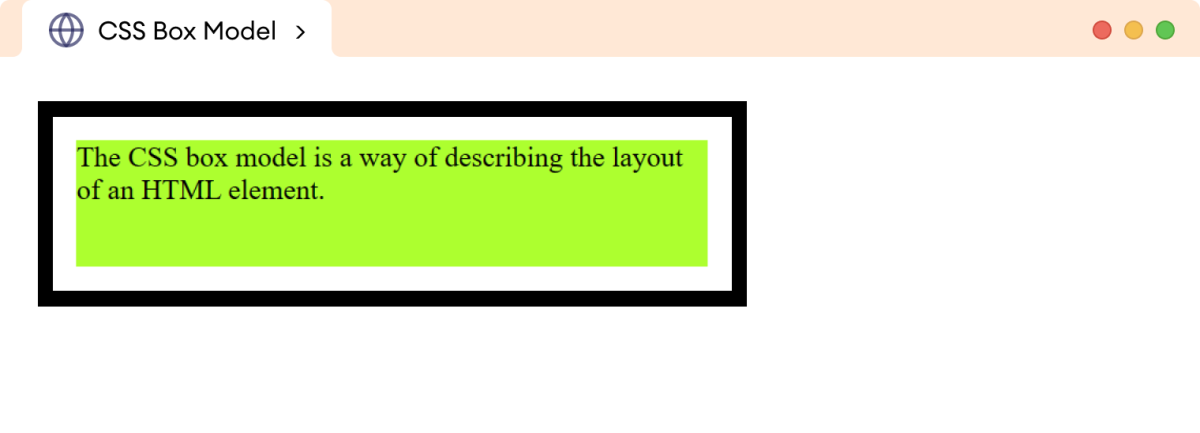
Here, the styles cause all h2
, div
, p
, and span
elements to have a 1px
solid border. This shows that all elements are rectangular in nature.
These essential concepts allow us to understand how CSS rules behave and help to design layouts more effectively.